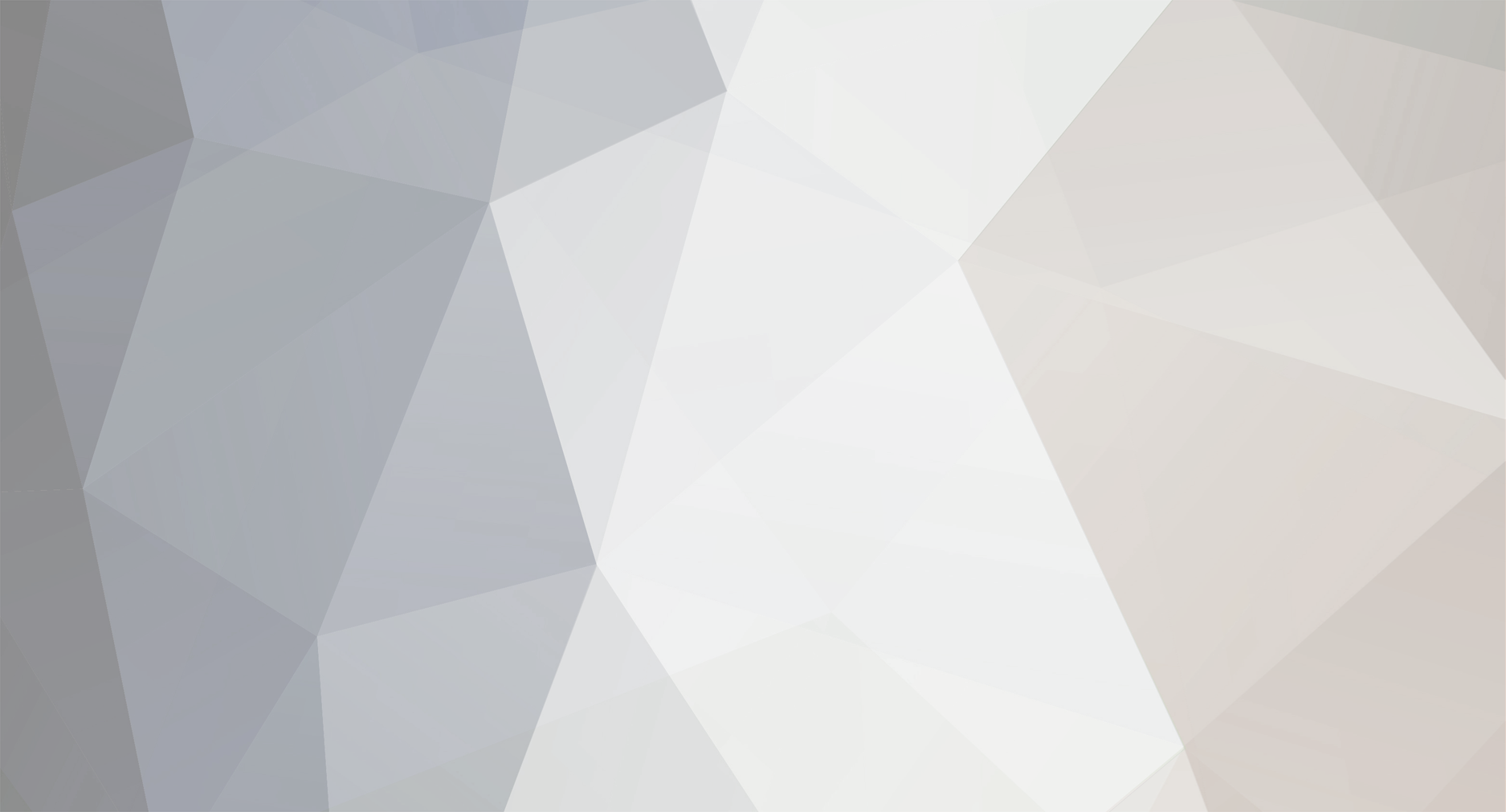
k_nitin_r
Members-
Content Count
1,467 -
Joined
-
Last visited
-
Days Won
5
Everything posted by k_nitin_r
-
Asp.net & Oracle Connectivity Can I connect ASP.NET with Oracle 11g
k_nitin_r replied to Nik's topic in Programming
Sure you can! I hooked up my ASP.NET application to an Oracle 11g database and it's heavily integrated with the Oracle database such that I can't pull it apart and hook it up to another database right now. What? ASP.NET doesn't work with Oracle? OMG! Hang on, I've got to go cancel my order for a new pair of JBL speakers... I'm not getting my paycheck till the application I built works and this is going to take a whole re-write of my code.... No, not really, just kidding! ASP.NET does work with Oracle and my paycheck is on its way. So, anyway, back to hooking up ASP.NET to Oracle. When I installed the Oracle database (I didn't settle for the Express edition cheapness... I went all the way and installed the real deal, since we had a development license lying around someplace), I got the database server and all the client libraries to go with it, and that includes the ODP.NET (Oracle Data Provider for .NET) and ODAC (Oracle Data Access Components?). After installing all the needful (the Oracle database, ODAC, and ODP.NET), you have to add a reference to the Oracle data access library (Add Reference through Visual Studio and select Oracle.DataAccess... if it is not in the Global Assembly Cache, browse for it and select Oracle.DataAccess.dll). Within your code, create a new instance of the OracleConnection class (located within the Oracle.DataAccess.Client namespace) and pass in the connection string as a parameter (the connection string would look something like "Data Source=ORCL; User ID=scott; Password=tiger". Then, call the Open method on the OracleConnection object and there you go! You have a connection to the Oracle database. Then, create an OracleCommand object by calling the CreateCommand method of the OracleConnection object, set the CommandText property to something like "DELETE FROM dept WHERE deptno = 20" and set the CommandType to CommandType.Text (an enumeration; these are a kind of class constants that are used to specify options to methods instead of using true-false flags or integers that are codes to represent values). Then, call the ExecuteNonQuery method on the OracleCommand object and watch department number 20 disappear from the database! Make sure you call the Close method on the OracleConnection object and if you figure out that the DML (Data manipulation language, a subset of the Structured Query Language) isn't taking effect, make sure that you've set the AutoCommit property on the connection to True. That's all there is to it! -
If you have worked with the StreamWriter object in .NET, you probably know how much of a pain it is when getting started. The StreamWriter is a buffer writer and it was built to improve the performance of your application, but if not used corrently, it can lead to data loss (and I am still trying to figure out where in the StreamWriter-sprinkled code do I have something that isn't writing to a file I'm expecting output in).Let's suppose that you have a FileStream object, perhaps created through File.OpenWrite or maybe even the FileStream constructor. When you call the constructor of the StreamWriter class, you would pass in the FileStream object like this: "New StreamWriter(ObjFileStream)". Getting an instance of the StreamWriter class is as simple as that - you don't have to tell the StreamWriter what kind of stream it is - Is it a file? Is it a network stream? Is it a line printer stream... (Reminds me of - is it a bird, is it a plane, no, it's superman!)Writing with the stream writer object is as simple as writing to Console because you have the Write and WriteLine methods that the Console object has and the Console object is one of the first things you work with when developing for the command line, so I'm assuming that's something we all know like the back of our hands. To write using the stream writer, we would have a call that looks like this: "StrWrite.WriteLine(MyStringHere)". Just as with the Console.WriteLine class, you would expect the text to appear in the file, right?Well, apparently it isn't so. My little console application simply open a file stream, creates a streamwriter object, and writes using the streamwriter class, then closes the file. When I close the application and actually look at the file, it is zero bytes in length. That's right, I've got nothing there, zilch, nada, you get my point! So, I look back at the code and try to perform a not-so-forensic analysis of what may have gone wrong. Considering the possibility of a buffer in the FileStream, I add in a Flush call to the FileStream object and run the application again, but there's absolutely no difference in the output of the application, so I decide that I'm barking up the wrong tree - I've flushed the filestream object before closing it and I did make sure that the FileStream object was appropriately closed with the call to the Close method so there's absolutely nothing wrong in there. I then turn my attention to the StreamWriter object, which seems to be the prime suspect in this case, and boy, was it really really guilty! Behind the scenes, when nobody is around, and when there are no eyes or ears around to figure out what's really going on, the StreamWriter object takes your string and goes off to sleep. Yes, that's write - it does not write to the stream. Imagine that, a lazy bugger slouching off while on-duty! So, the sloth (read: instance) of a StreamWriter class just leaves your string there on the work table, untouched, till it actually thinks that there's so much on the table that there's no space left there at all and it just has to go on there and get some work done lest the table itself collapses under the weight. In other words, the StreamWriter object maintains a buffer so that it does not have to write to the stream each time you make a call to the Write or the WriteLine method. The advantage of this is the application does not have to wait on the stream to finish writing each time it makes a call to the Write or the WriteLine method and therefore the application feels quicker. Why is it quicker? Think about your recent shopping trip to the grocery store. When you did have to line-up in a queue to get a bottle of Coke (sorry, Pepsi, they've got a shorter name and so its easier to type), you would have to wait in the queue for about 2 minutes, pay for the Coke, and head out... just then, you figure out that the Coke bottle needs a bottle opener so you head back into the store, get the bottle opener, wait in the queue for another 2 minutes, pay for the bottle opener, and you head out. See the problem there? Because you had to get into the queue twice, you had to waste a good 4 minutes of your time that would otherwise have been spent watching the game on television (or something equally useless and unproductive), but the point here is that it's simply inefficient. Instead, if you got both the Coke and the bottle opener the first time, you would have spent only 2 minutes in the queue. Anyway, so that's exactly what the StreamWriter object does.Okay, now that we know why the StreamWriter is a celebrity in these parts, how do we get it to actually write the output to our file? Simple - we call the StreamWriter object's Flush method. That's write, just as the FileStream object has a Flush method to call, the StreamWriter has a Flush method too! The Flush tells StreamWriter to get off it's lazy bu*t and get the job done before it has to get shipped off to someplace else (shipping off the work product, not the StreamWriter object!)Anyway, having done all that, I now have my application writing output to the file just as I expect it to and I hope we've all learned a lesson out of all of this - don't trust that sloth of a StreamWriter to ever do it's job. You're supposed to get back in there and Flush out all of the data in the end!
-
PHP programming is pretty easy to learn and is the programming language of choice for most web designers. PHP code fits in neatly within regular HTML code and is therefore really simple to build, just as was the case with classic ASP (ASP.NET, however, uses a different programming model with a clear separation between code and HTML markup - a move that has been welcomed by many a developer). Having code within the HTML markup is neither a bad thing nor is a good thing. Think of the case when you want to simply add in the server time or the user name into the HTML markup... you could just put in a:<?= $username ?> and the username would appear right then and there! However, when you have to write complex logic into the HTML markup, that's when you should consider having to move some of the code into a separate file. There's a pretty good balance that most PHP development frameworks achieve between putting code into the HTML markup and moving it back into a separate code file. Template engines, such as Smarty, help you in achieving such separation between the HTML markup and the backend coded logic.Learning to program with PHP is as simple as getting a PHP-capable web server (for most people, it is Apache with the PHP modules installed though you can also use IIS for PHP development and testing) or signing up for a PHP hosting account. You can build your webpages as you have been building them with Adobe DreamWeaver, Bluefish, or Notepad++ and can put in the 'magical' PHP code to make the web page content change dynamically. A lot of websites out there make use of content management systems developed in PHP, which means that they do not have to re-create the HTML files whenever they want to add additional pages or articles, or change the layout of elements on their sites. They can simply log in to a backend administrative interface that provides the administrator with the ability to select where a component should appear, add in a couple of plugins, add or edit articles, and publish articles for them to be visible on the site. Commenting is a feature present on most content management systems and provides a great way to obtain feedback from visitors to the site.You can get yourself a good book on PHP that tells you about the basics, on how to get started, what type of editor to use, and provides you with a quick reference for the functions that are available in PHP. You can also get yourself a good editor, once you are familiar with the basics of the language, such as the NuSphere integrated development environment. The advantage of getting an editor is that they provide you with the autocomplete that you need to quickly develop code without having to go back to the PHP function reference. Iniyila has provided a good set of web references for you to get started with PHP programming and those are pretty comprehensive enough for you to build some real applications with. You can find sample chapters from the PHP Cookbook online and can get you a feel of what is in the book.I got started with PHP programming while looking at existing programs. They helped in understanding the structure of the programs and the norms that are followed within the PHP development community. As a great programmer once said, "Read more code than you write." I believe he said he would read twice or thrice as much code as he would write.I hope I have left you with something useful in getting started on your path to PHP. My advice to you is to keep a schedule for your learning and stick to it, lest you fall along the path of many a traveller who started but never entered the world of PHP development.Happy programming!
-
While building WordPress widgets, I use the widget API that was introduced in WordPress 2.8. I have always wondered, however, what the parameters passed to the WP_Widget constructor are and have only been passing in a false followed by the name of the widget. My curiosity got the better of me today and I finally decided to go figure out what the other parameters to the WP_Widget constructor were.I looked up Google, the all-knowing search engine that documents almost all of the knowledge known to man, but all I could find were tutorial sites that explained how to build a widget and all they would say is along the lines of "pass in the name of the widget as the second parameter". As my search went on, I reached the definition of the WP_Widget class and I noticed that the WP_Widget class does indeed take four parameters, which are the Base ID, the widget name, options for the widget, and other control options. The default value for the Base ID is taken as false, which is the argument being passed to the $id_base parameter by most tutorials and widgets out there. The name is something for the plugin developer to specify and is self-explanatory. The widget options are values that are passed on to the wp_register_sidebar_widget function (I hope you still remember that function from the pre-WordPress 2.8 days) and can take a class name and a description through an associative array. The control options, which are the last parameter to the WP_Widget constructor, can take parameters to pass to the wp_register_widget_control function that can specify the width and height for the widget. The width parameter can be specified but the height parameter has been document as not currently used. Both the width and the height control options are passed in through an associative array.
-
If you've still got the laptop with you, you could do a couple of less-destructive things with it too. I'd run a web server on it and have it run my personal website. I run WordPress on my personal website and even an old laptop should be able to handle the load from a small number of concurrent users. WordPress may be CPU intensive, but if you do enable caching, it can run on older hardware too.You can also use old laptops for hooking up webcams and using them instead of CCTV cameras. You can write up a script to record video footage to a file and periodically upload the footage to an FTP server. If you are less concerned about security, you can simply take photographs and send them across periodically instead of a video - you may not catch a burglar in the act, but you would know that something is wrong out there.If you can hook up a couple of extra network cards to the laptop (either PCMCIA expansion cards or USB), you can turn the laptop into a router too.If you've got a computer that you would like multiple users working on together, an old laptop can be used as a terminal for connecting to the other computer. You can hook up the old laptop to the network, run a terminal server, telnet, or SSH server on the other computer and connect to the computer via the laptop. All of the execution would be through the other computer and the old laptop would only be sending input and receiving output. Think of it as a wireless keyboard and monitor. You can also do this with old smartphones. If you have an iMate Jasjar, you can connect to a computer and you have a QWERTY keyboard on the phone to be able to do stuff that you would normally do from the computer.You can also use old computers to share hard disk drives across a network. You can hook up your USB external hard disk to it, plug it into the network, and access the root of the drive as an administrative share or create a shared folder on the USB drive.Using an old laptop with a webcam for security, as a router, or for sharing external hard disk drives may seem like a patchy solution because if you were to get dedicated devices to perform the same function, they would be in a much more compact form and would use much less electricity. However, using an old laptop is a cost-saving measure as buying a network CCTV camera would cost you much more than a simple webcam, a router that provides all of the functionality as the old laptop would cost much much more than a simple network card, and getting a cheap external USB hard disk drive (the large ones with an external AC power supply adapter) can give you more bang for the buck than a passport USB external hard disk drive or even an external hard disk drive with a network interface.
-
Firefox 4 has been released earlier this week and it definitely is a significant improvement over previous releases. The Firefox 4 web browser now provides the ability to have the tabs open in the title bar of the web browser, thus extending the web page display area within the browser, just as Google Chrome does. If you disable the menu bar, add-on bar, and search bar, Firefox gives you more web page viewing area than Chrome does! The only downside is that Firefox maintains a separate addressbar and search bar. If the two were integrated, it would totally rock! That's on my wishlist for Firefox 5.To download Firefox, point your browser at https://www.mozilla.org/en-US/firefox/new/?utm_source=getfirefox-com&utm_medium=referral
-
Php Bug Tracking System Free PHP Bug tracking scripts recommneded
k_nitin_r replied to annyphp's topic in Programming
Hi!My bug tracking system of choice is and always has been the MantisBT bug tracker. The MantisBT bug tracker is written in PHP and is very extensible through plugins. You can also find browser search providers for the MantisBT bug tracking system (navigate to the site and look at the menu items from the search provider dropdown to find it). I may be biased in my opinion because of my love for all things PHP. PHP-based software is available in source code form, enabling advanced users to make modifications to the code base and tailor the software product to meet their needsIf you are looking for other options to track bugs, you can use Jira (for a Java-based bug tracker), Trac (for a Python-based bug tracker) , and Visual Studio Team Suite (for a .NET based bug tracker). Visual Studio Team Suite is more than just a bug tracker. It is in fact a complete software development workflow management system that includes a source code repository (an enhanced version of the Visual Source Safe source code repository) and is integrated within Visual Studio.Sure, you will find people that will claim that one bug tracking system is a complete mess while another is one that was written by the Roman and Greek Gods themselves (For an example, perform a Google search for "trac bug tracking software is junk" and look at the first search result, which should be from designoplasty.com, unless Google decides to index this post and put me on top of the search result page). Everyone is entitled to his or her own opinions. I've once claimed that BugTracker.NET was too buggy to be used in a production environment due to the extended downtime (Side-note: I have no clue - it would just display the ASP.NET error page for no reason, and the error page would go away after waiting for an hour or two. The error would appear every morning and would go away on its own, without any intervention though it sometimes needed the IIS server to be restarted to get it working without the wait). All I can say is, "To each his own" (I don't really know who I'm quoting, but whoever said it phrased it aptly). -
Hi!Namespaces in .NET are analogous to packages in Java. They enable you to create classes with names that are similar and help in avoiding naming conflicts. They also help in maintaining consistency between class names. The concept of namespaces was probably borrowed from C++ and Java, so it isn't unique to the .NET platform.For example, the class OracleConnection is present within Oracle.DataAccess.Client, from the ODP.NET library provided by Oracle, as well as System.Data.OracleClient, the Oracle database access client library provided by Microsoft. By usin the appropriate namespace, such as by importing only one of the two namespaces or by using the fully-qualified name of the class, you can easily switch between the two classes.
-
@bojaxAlienWare is great! They even attach a customized engraved name plate to the base of the notebook to make you feel that you are getting your money's worth. But are you really? You can get a desktop that does what the notebook does for half the price but then I guess you are paying for the mobility and the convenience that having a notebook has to offer.Just as Dell sells AlienWare, there is the Qosmio from Toshiba, and there is the Thinkpad W-series from Lenovo. You don't get the bang for the buck, but you get the ultimate in notebook computing (back in the day, we would call that mobile computing, but the term applies to personal digital assistants, tablets, and cell phones now).My choice is something that lasts - the Thinkpad T-series is for me, till I can afford to get the Thinkpad W-series.
-
The solar powered boat is a thing of the future. You may mock at it now, but five decades from now, everyone is going to have one. Well, at least that is what they said about the Ford Edsel and nobody really got one but the fins on the back of cars became a mark of the yesteryears although no one back then would want to be seen in one. I guess the movie producers are to blame - they have weird fantasies about the past.The cheap solar panels are not as efficient as the ones that they mount onto satellites and send out to space, but at least it is better than nothing. Plus, it helps keep the heat away from your roof keeping the upper rooms cooler. If you have sloping roofs like I do, solar panels are the way to go (unless you happen to have hail storms that leave holes in your roof and car windshield).
-
@nirvamanWe're more than pleased to answer Ojaser's questions - we're getting paid MyCENTs to answer them :-)@deadmad7Oh, I never noticed that there's a Help link up there. I guess it's because I never sign out, let the browser take care of tossing away the cookies, and have the web server time out my session. If I had a desktop, I'd never sign out... my notebook doubles up as a workhorse to run databases for software development and that has me closing applications to save on memory (back when I got the notebook, 3GB was a lot of memory).
-
@chinuThe MyCENT system takes a while to kick in. From the looks of it, you are earning MyCENTs already - your personal message block shows a MyCENT earning of 13.26 right now - it is the number in red just above your current mood.As soon as the MyCENT count hits 100, the 100 cents go into your account and you can start making purchases. The absolute basic package that Xisto Hosting has to offer (the decade plan) is great for somebody who just wants to have a personal website, but move up to level two (logic plan) for up to five domains - that's a good choice in my opinion.BTW, where are you hosting your websites right now?
-
I just made my 10 posts of the day. It took a whole 3 hours!
-
-
-
@nnnoooooo: 6 months later, and the forum still lives!
@anwii: I have an on-and-off thing, so I can't really say I'm back. Whenever I can spare the time, I head back here and post.
@chini13: A lot of time and effort goes into making each post of enough length to count for some real content, so about a dozen is all an average person can manage in a day. I can help you with a couple of posts though - if anything comes up on your mind, start a new thread, and we...
- Show next comments 75 more
-
-
Earn money quickly with a small investment? Let's go rob a piggy bank. All the investment we need is in getting a hammer, which can probably be rented for the job, and BAM! A small loan of pocket change. If that was your own piggy bank to begin with, you now need to go out and buy a new piggy bank. If it wasn't, you would owe someone else a piggy bank and a pocket load of change.There's no such thing as a get-rich-quick scheme that is guaranteed to work. If you do hear of such a scheme, it would only make the guy who came up with the plan rich.However, you can put in some hard work and a couple of months to develop a network of contacts, pick up some contracts, and hire staff to get work done. There is a lot that can go wrong and there is a lot that needs to be taken care of, but in the end the rewards pay off assuming you did all that needed to be done. That's how much freelancers and independent contractors work.
-
All I can say is the same as what anyone else on the forum would say. Tell your boyfriend what really happened and if he hears it from you and he really loves you, he will forgive you. The night with the ex is a mistake you will have to live with. It happened and there is nothing you can do to change the past. To keep the present, all that you can do is go with the truth and hope it all goes well but expect that there is a good chance that it will not.As soon as you have set things right with your boyfriend, the threats from your ex will not seem threatening any more and he would only make a fool of himself if he eventually does end up telling your boyfriend about what happened on the night with ex.
-
Solar energy is a great way to think about conservation. As long as the sun has enough Hydrogen to power the nuclear fusion that it has going up there, things are looking cool. The problem with using solar energy is that the energy comes to earth in a diffused form and therefore you would require a large area to capture the energy. One way to reduce the cost of producing electricity from solar energy is to use mirrors to help increase the concentration of sun light onto the solar panels.If you do find yourself using lots of 'AA' batteries and tossing them into the bin, think of how much you could save by using rechargeable cells and charging them using solar energy. Battery manufacturers need to do their part by making rechargeable batteries cheaper. There are tons of them all around in mobile computing devices, digial cameras, cellular phones, and other electronic devices so surely they would have achieved economies of scale. Apart from the energy needed in the production of batteries, such as in powering diesel trucks to transport chemicals and materials for the batteries, we also use traditional fossil fuels in disposing off the disposable batteries so rechargeable 'AA' batteries is what we should all be demanding.Nuclear fission reactions are 'dirty' in that they result in radioactive fuels that are hard to dispose off. Existing research has not provided us with the technology to successfully manage a sustained nuclear fusion reaction, let alone build a nuclear fusion reactor.Windmills are definitely a cheap source of energy when you've got plenty of wind blowing all around the year. As long as a hurricane doesn't bring the windmill tearing down onto your rooftop and crashing through the living room, they're the best way for the rest of us living by the country side to get some clean and cheap energy.
-
Hi!What you need to do is create an intermediary script that either outputs the header to redirect to index.php or include another file that contains the markup with the stylesheet or anything else that you choose to include. This approach will not only fix the problem that you are experiencing but will also make your code better organized (and I'm sure folks would want to debate on that bit... what constitutes a good design is something fairly subjective).If you do need more help with the problem, feel free to reply or send me a private message/email/instant message and I'll try to get back to you as soon as I can.
-
10 Posts Per Day Pledge - Save The Forum! Save the forum
k_nitin_r replied to deadmad7's topic in Web Hosting Support
@deadmad7, I'm glad you started this thread and I really do want to help with the Xisto resurrection. I've posted 7 posts so far today. It took me about two hours to type them up and send them across, minus a short tea break and the time I spent in thinking of stuff to write about.@Mahesh2k, offering reduced MyCENTs to older members who participate often is a kind of a penalty and would drive them away rather than keep them on the forum. Sure, they don't need the MyCENTs, but it's the feelings that such a system evokes. I'm sure a couple of members would come back if they were offered the same or higher MyCENT rates than other members.@Contactskn, I haven't had a Captcha or warning either, but I guess it is because I'm mostly responding to existing thread and am not creating new threads. I've always been more of a thread responder than a thread started, but I guess that's the kind of people that the forum needed back then since there were folks creating tons of new topics.@Iniyila, I have been inactive for quite a while but do occasionally come back to check on the forums. I've got an average of 1.36 postings per day but considering that I joined way back in May 2009, it isn't half bad. I don't manage to come up with posts as long as anwii did and I don't make as many posts as Saint Michael did but I think I did manage to come up with what I would guess to be the average across all the members of the forum if you don't count the inactive members with under a dozen posts to the forum.Apart from forum posts, I think we ought to get other parts of the forum active too - post your status updates, set your mood, share some links, hang out in the chat rooms (another thread responder suggested this too, if you scroll up a bit), and post pictures to the gallery. All it takes to get Xisto resurrected and buzzing with activity again. -
Do You Have A Mobile Phone? Then say how old are you
k_nitin_r replied to sader's topic in Mobile Phones
My nephew who is 7 years old has a cell phone. It's a Samsung but I can't remember which model it is. The oldest I know is an uncle who is about 63 years old... I don't know what phone he uses but I do call him up occasionally.I started off with an ancient Nokia 2050 while in high school, switched to a Nokia 6110, tried a couple of brands including a Siemens C35i, a Motorola V60, a Motorola V66, and an i-mate Jasjar (read as HTC Universal, since i-mate shut its doors during the economic recession), and had a Nokia 9210i Communicator (before the i-mate Jasjar). I had a Nokia 2600 as a spare phone that still works really great! I recently switched to a Nokia E72 and it offers pretty much everything that the i-mate Jasjar and the Nokia 9210i Communicator had to offer, but it all comes within a smaller and more practical package.Some things of the past still remain the best and I must say that the screen of the Nokia 2050 was really good for reading text messages with the green backlight for night reading.