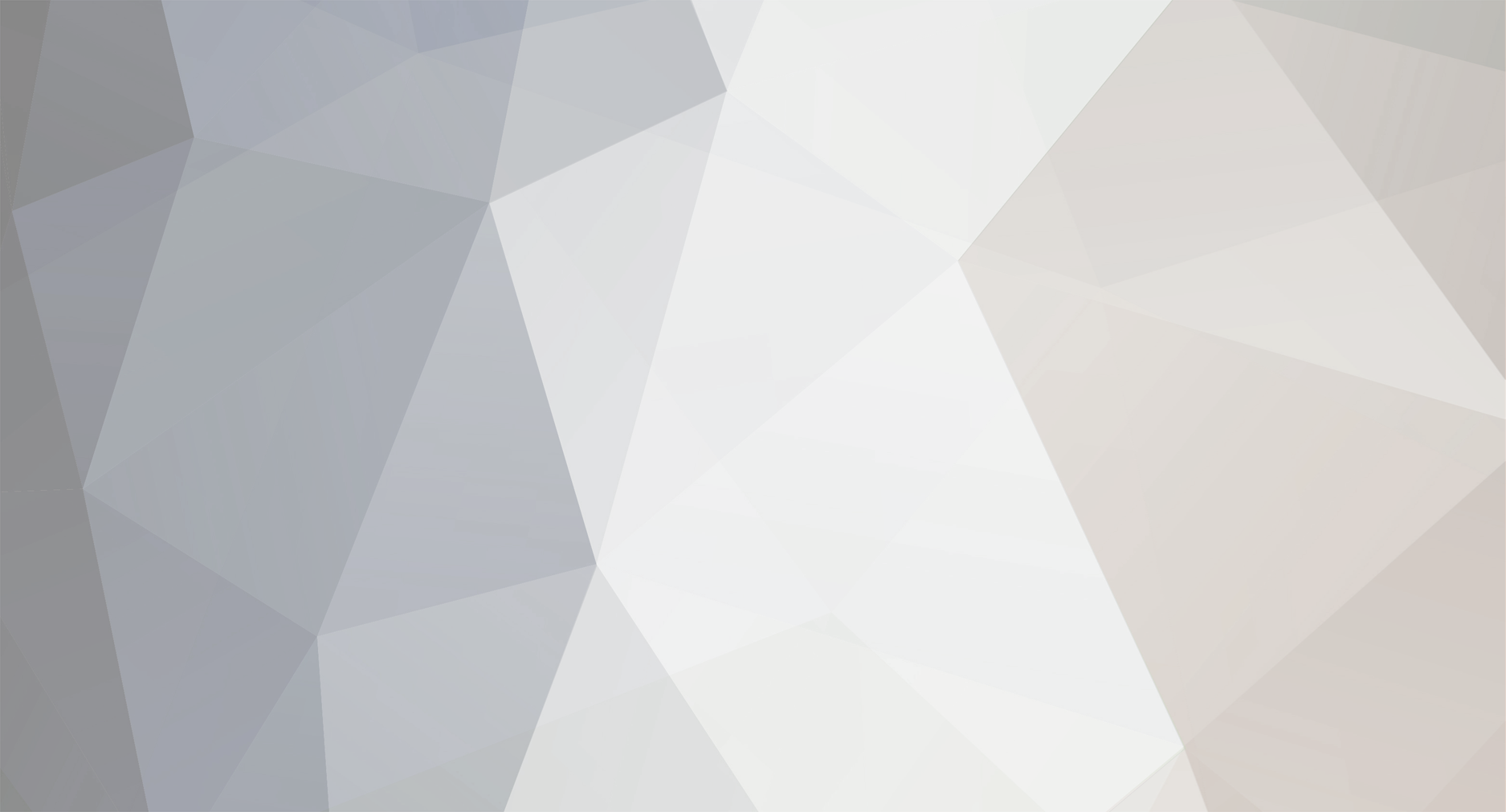
niran
Members-
Content Count
190 -
Joined
-
Last visited
Everything posted by niran
-
Hi all.. This the Section 2 for my previous tutorial about storing values in background using ajax! Please have a look at here: Xisto.com/ajax-php-sql-simply-superb-visitor-tracking-t15502.html Introduction! We are going to create 2 background script files. One to get values from the main page input field and store all values including visitor details ( IP Address, Input Value, Visitor Agent etc) to database and the 2nd one for retrieving all values from database and display it in the screen! We are going to 2 separate pages first and then going to integrate all those 2 pages to the main user interface page! So, let us start guys!! Stage 1: Getting values from the database and displaying the same We already inserted some values to the database as described here Now we have to retrieve all values by using query and to display them in screen!! So, here is the query to retrieve values: SELECT * FROM ajax ORDER BY slno DESC This will retrieve all values from the database and will be stored in some buffer! We have to show each and every field values in some table cells! So, here comes the main code for that! $query="SELECT * FROM ajax ORDER BY slno DESC"; $result=mysql_query($query); echo "<table> <tr> <th>Sl Num</th> <th>Text Msg</th> <th>IP Address</th> <th>Hostname</th> <th>Visitor Agent</th> <th>Time</th> </tr>\n"; while($row=mysql_fetch_array($result)) { echo "<tr bgcolor=#2F2F2F>\n"; echo "\n<td align=center>". $row['slno'] . "</td>"; echo "\n<td>". $row['test'] . "</td>"; echo "\n<td>". $row['ipaddr'] . "</td>"; echo "\n<td>". gethostbyaddr($row['ipaddr']) . "</td>"; echo "\n<td>". $row['agent'] . "</td>"; echo "\n<td>". date("D, d M y g:i:s a",$row['timer']+45000) . "</td>"; echo "\n</tr>"; } echo "\n</table>\n"; Here we already stored the visitor ipaddress. We can get hostname from the ip address by using the function: gethostbyaddr(); The above while loop will execute for the n number of times (* n = number of records retrieved) And we can get the timestamp and we can format it using php date() function! More details about Date function available here Ok, I think, now the idea is clear! This will display all values in some table format! Now we have to place some checkbox in each and every row and clicking the delete button should delete the selected values! ( Got this checkbox deletion idea from here ) So we are midifying the script page like this: if($_POST['perform']) { foreach($_POST as $id) { // This will loop through the checked checkboxes mysql_query("DELETE FROM ajax WHERE slno='$id' LIMIT 1"); // Change yourtable and id. This deletes the record from the database } } Thats over in the display part! So finished page will look like this: <html> <head> </head> <body> <form name="action" id="action" method="post" action="<?=$_SERVER['PHP_SELF']?>"> <? $MyUsername="username"; $MyPassword="password"; $MyDatabase="database"; mysql_connect(localhost,$MyUsername,$MyPassword); @mysql_select_db($MyDatabase) or die( "Unable to select database"); if($_POST['perform']) { foreach($_POST as $id) { // This will loop through the checked checkboxes mysql_query("DELETE FROM ajax WHERE slno='$id' LIMIT 1"); // Change yourtable and id. This deletes the record from the database } } $query="SELECT * FROM ajax ORDER BY slno DESC"; $result=mysql_query($query); $num=mysql_numrows($result); //echo date("D M d, Y g:i a"); //echo time(); //echo date("D, d M y g:i:s a",time()+45000); echo "<table width=100% border=0 align=center cellpadding=4 cellspacing=1 bordercolor=#333333 bgcolor=#FCFCFC class=sample id=sortTable> <tr bgcolor=#990000> <th height=30 align=center><span class=tab_head>Sl Num</span></th> <th height=30><span class=tab_head>Text Msg</span></th> <th height=30><span class=tab_head>IP Address</span></th> <th height=30><span class=tab_head>Hostname</span></th> <th height=30><span class=tab_head>Visitor Agent</span></th> <th height=30><span class=tab_head>Time</span></th> </tr>\n"; while($row=mysql_fetch_array($result)) { echo "<tr bgcolor=#2F2F2F>\n"; echo "\n<td align=center>". $row['slno'] . "<input type=checkbox name=". $row['slno'] . " id=". $row['slno'] . " value=". $row['slno'] . " /></td>"; echo "\n<td>". $row['test'] . "</td>"; echo "\n<td>". $row['ipaddr'] . "</td>"; echo "\n<td>". gethostbyaddr($row['ipaddr']) . "</td>"; echo "\n<td>". $row['agent'] . "</td>"; echo "\n<td>". date("D, d M y g:i:s a",$row['timer']+45000) . "</td>"; echo "\n</tr>"; } echo "\n</table>\n"; mysql_close(); ?> <input type="submit" name="perform" id="perform" value="Delete Selected" /> </form> </body> </html> And the finished page is available here: Fun.niranvv.com/test/php_ajax_output.php Stage 2: Embed the page inside main background script file We can view the results in separate as seen in the stage 1! But we have to display the table inside the main page immediately after inserting the values! So, we can copy and paste the script to the main background script page called by clicking the enter button! But, That will complicate the pages! So, Im going to include the script file inside the main background script file! We can include like this: echo include("php_ajax_integrated_output.php") here "php_ajax_integrated_output.php" is used to output the values ( created in stage 1 ) So the main Background script file will be like this: <?php $dbhost = "localhost"; $dbuser = "username"; $dbpass = "password"; $dbname = "database"; //Connect to MySQL Server mysql_connect($dbhost, $dbuser, $dbpass); //Select Database mysql_select_db($dbname) or die(mysql_error()); if (isset($_SERVER['HTTP_X_FORWARDED_FOR'])) { $ClientIP = $_SERVER['HTTP_X_FORWARDED_FOR']; } else { $ClientIP = $_SERVER['REMOTE_ADDR']; } //$ClientHost = gethostbyaddr($ClientIP); $ClientAgent = $_SERVER['HTTP_USER_AGENT']; $MyTimeStamp = time(); // Retrieve data from Query String $word = $_GET['word']; // Escape User Input to help prevent SQL Injection $word = mysql_real_escape_string($word); //build query $query = "INSERT INTO ajax(ipaddr, agent, test, timer) VALUES('$ClientIP', '$ClientAgent', '$word','$MyTimeStamp')"; //Execute query // Exit if calling directly the script file! if ($word != "") { $qry_result = mysql_query($query) or die(mysql_error()); echo "Updated Successfully with values IP :$ClientIP<br>Visitor Agent: $ClientAgent<br>Input Word: $word<br>Current Time Stamp: $MyTimeStamp"; } else { echo '<b>Hacking Attempt!! or No Input Values</b><br><br>'; } // Add Output Table echo include("php_ajax_integrated_output.php") ?> And I'm going to modify the included page like this for easy understanding: <? $MyUsername="username"; $MyPassword="password"; $MyDatabase="database"; mysql_connect(localhost,$MyUsername,$MyPassword); @mysql_select_db($MyDatabase) or die( "Unable to select database"); $query="SELECT * FROM ajax ORDER BY slno DESC"; $result=mysql_query($query); $num=mysql_numrows($result); echo "<table width=100% border=1> <tr> <th align=center>Sl Num</th> <th>Text Msg</th> <th>IP Address</th> <th>Hostname</th> <th>Visitor Agent</th> <th>Time</th> </tr>\n"; while($row=mysql_fetch_array($result)) { echo "<tr>\n"; echo "\n<td align=center>". $row['slno'] . "</td>"; echo "\n<td>". $row['test'] . "</td>"; echo "\n<td>". $row['ipaddr'] . "</td>"; echo "\n<td>". gethostbyaddr($row['ipaddr']) . "</td>"; echo "\n<td>". $row['agent'] . "</td>"; echo "\n<td>". date("D, d M y g:i:s a",$row['timer']+45000) . "</td>"; echo "\n</tr>"; } echo "\n</table>\n"; mysql_close(); ?> We are dynamically updating the main page with updated database field values! Now the this is the process flow: User enter information and clicking the enter button ( php_ajax_integrated.php) Entered values will be send to background script (php_ajax_integrated_script.php) using query string The values will be inserted to the database and the background script will call another php page (php_ajax_integrated_output.php) to display updated values using echo include("file path") In that php_ajax_integrated_output.php , retrive all values from the database and display the values Inrtegrate all files and display output in the main page ( Please have a look at here for more info So, here is our final integrated page: Fun.niranvv.com/test/php_ajax_integrated.php Thats all guys!! If you have any doubt, Please reply here! I will try to help you! ( Im a beginner only .. Yes guys!! U have to believe my words!! Just went through some online tutorials about php and ajax, and got some ideas! And tried to integrate all.. This page is the result of that!! And it took only 1-2 days to study all these functions! I Started from a big '0' only!! So, if you dont have any ideas, dont worry!! If you know pragramming concepts, u can also come up with this! all the best ) regards, Niru
-
You can get Fully Integrated Shoutbox from here:http://www.phpbbhacks.com/download/1255 It can be displayed on your index page! Its having many features like templates,censored words, smilies, BBcode,languages, and it will only reg users yo shout on the shoutbox! For any phpBB related help, you can contact me!! Im totally addicted to phpBB!! I Love phpBB!! Its the SIMPLEST forum software!
-
World's Smallest Website the smallest in the word
niran replied to iGuest's topic in Websites and Web Designing
Yop! that was a really nice one!!Thanks for posting here dude! Really liked that one!!I'm going to buy one glass for my eyes!!can't see anything and I can realize how SMALL is this world -
I personally recommend you to use PhpBB for ur forum! https://www.phpbb.com/ You can get many customization options by using mods! http://www.phpbbhacks.com/ is the best site to get MODs for ur phpBB forum! Fully MODed phpBB forum is available to download from various sites! A fully Moded phpbb software called as PhpBB Plus is available free to download! Its having many security options! http://www.phpbb2.de/ is the site to download phpBB Plus! You can download phpBB2 Plus 1.53a Full Package from here: http://www.phpbb2.de/dload.php?action=file&file_id=828 Installation is easy task!! Please have a look at my old tutorial to install phpBB forum ( for phpbb plus also) http://forums.xisto.com/topic/92495-topic/?findpost=1064365430 It will be helpfull to you for the installation and configuration of the forum! But phpbb does not have that much having any security like paid forums! A fully MODed phpbb forum is available here: http://www.funlokam.com/ ( My personal forum ) Thnaks, Niru
-
Very interesting work dude!! Good use of Ajax Technology! Its interesting to see checking the accounts and site status dynamically!! But, For me, its showing as site not working! But I can able to open my site! All the best for your works! Thanks, Niru
-
Site is working fine now!!!! But there is some probs with the database!!!! Showing some error message as can't connect to database server in regular intervals!!! And the cPanel is not looking good as before !!!! While checking cPanel, the database size is displaying as NA Megabytes! and showing number of databases as: NA / 99 Also, phpMyAdmin is not working Hope, the guys will fix the issues soon!! Niru
-
I can open the cPanel for my site! But its not looking nice and shows the version info as "cPanel X v2.6.0" And my disk usage is showing as 0 MB ( It was around 60 MB) and MySQL size is showing as 0.02 MB ( It was greater than 30 MB) If I try to open the site, then its showing like this Will I get the files back?? and I don't have any database backup!! How much time it will take to restore the accounts??
-
Hi all, Daily we are receiving countless mails, showing pictures of Indian cricketers as barber, milkman, coolie, cook, butcher etc etc. Just answer these questions before we go ahead… 1. We go to temples, make lots of wishes to god, some of them come true, some don't…will you start burning effigies of gods and godess..if god can fall short of our expectations…they are just human beings!!!!!!! 2. India is still a developing nation, most of us come from average middle class families. tell me if tomorrow..Microsoft,google or sun comes to you and offer you ten times what infy is paying..what will u do????Every one of us is striving for more money..these cricketers are not the family members of TATA n Birlas…like us they do ve every right to make money..they do so cuz they have the stuff..like ur here in infy cuz ur gud enough for it. 3. India ranked no.6 prior to start of wc-2007…!!!!!!!!!!if a no 6 team can so easily win the wc….guys n gals…be ready all of u r going to onsite tomorrow..as a PM/GPM/SPM or who knows Nandan might nominate you as the next ceo of ITL tomorrow!!!!!!!!!!!!!!!!!!! 4. How many among us are IITians or have come even from top 10 engg colleges of India…(????)but be honest haan…you must have taken all these exams n entrance..after class 12 th but u didn't succeed!!!rite as simple as that!!accept ur failure!!!what ur parents did…they started mourning over the dummies kept as your dead bodies..or they also burned your effigies..did ur mother stop giving you food or father stopped giving u pocket money!!!!haan..they backed you..always supported you paid heavy donations and fee to let you complete ur engg and beared all your other expenses till u got the right job!!!!!!these cricketers are our fellow Indians our very own blood…they are going through a rough patch of time so instead of backing them up we are abusing them.. 5. Your mom n dad calls u late in the night ur still at ur office..they say ..son ..go n have ur dinner..work hard..do your best….when u will get salary hikes..promotion..or remember your college days…dad calls u..son why r u getting less marks in every subject…YOUR REPLIES::::::::::"oh common…dad,mom u don't understand..it really tough,only I can understand bla bla ….guys….same is the agony of these cricketers…no one can understand how it feels to be in the middle..n burdened with expectations of a billion ppl….shame on us we hardly live up to expectations of merely two persons(our parents!!!!)!!!! 6. We produce false certificates,claims to get posted at our desired locations..or if not we will run away to our hometown..on a leave…saying "I am feeling homesick"!!!Remember Sachin…wc-1999 his father died in India…while he was in England he came n rushed back to support and play for his team n country!!!!!!how many of us can even think of this !!! 7. Lastly it's the mistake made by ourselves that we don't consider a game..just as a game…its we the ppl of India who made these cricketers dummy gods..simply fanatic..thank god fans in Zimbabwe,Canada and Netherlands are not like us or imagine what they would have done to their teams if expecting them to win world cup!!!Try to live up within the spirit of the game…take a game like the way it should be. 8. Frnz…this is a tough time for our cricketers and their families..stand beside em' support them they badly need it..they are already upset don't add up to their agony n pain.. N lets get together n say…HOO-HAA INDIA…..PHIR "AAYEGA" INDIA!!!!!!!!!
-
Gmail Paper :: Free Physical Copy Of Any Message With The Click Of A Button
niran replied to niran's topic in Search Engines
Hehehe!! Funny guys at Google!! I was searching for that "Paper Archive" button in my GMail!! Didn't expected this from Google -
Google's Annual April Fools Pranks
niran replied to nightfox1405241487's topic in Websites and Web Designing
hahaha!~! I was also a small victim of that GMail paper!! Started one topic here at Xisto itself!! -
Hi all... One new news from Google GMail Team!! GMail Paper Get your Physical copy of any message by Mail ( Not eMail ) with the click of a button!! Yes its true guys!! Its completely FREE only ( Its not 'April Fool' Special Announcement ) You will get it within 2-4 business days as parcel! You can You can read, touch it. Or even move it to your Original Trash Can! Here is the Image from Google: Check this page: http://forums.xisto.com/no_longer_exists/ Regards,Niru
-
Hi.. I was using IP Country Flag 2.9.2 for my phpBB2 board at: http://www.funlokam.com/ Problem 1: I'm facing one issue with the mod. Each and every time, when an user goes to "Edit Profile" page, The user country will be reseted to the "Non Selected" And the drop down will be shown like "Select your ISO.... flag" How can I get the default selection in the dropdown as user previously selected flag? Problem 2: Yesterday I had applied the two fixes for 2.9.4 as mentioned here: http://forums.xisto.com/no_longer_exists/ and here: http://forums.xisto.com/no_longer_exists/ ( The SQL fix has been applied manually by going to phpMyAdmin) Note: I changed all those default field values to "in" where "wo" was used! Also changed lang file as: Actual IP to Country >> Current Location User Country select >> User Country Now I'm facing one new problem friend! Inside one topic itself, for some users ( with this zz.png flag) its showing User Country select: as zz.png in one page and some other flag in the next page! I'm posting the screenshots here! Page 1: Page 2: What will be the problem with the MODing?? Can anybody help me to fix the issue? Thanks, Niru
-
Favicon Shows Only On Main Domain Url ?
niran replied to miCRoSCoPiC^eaRthLinG's topic in Websites and Web Designing
I think, for IE and Firefox it will automatically search for the Favicon in the main page of the domain! For me, Im using the favicon (Animated one fore Firefox ) for the main page only at: http://niran.in/ but, its working fine for the inner directories also ( without specifying the favicon location ) I mean, you can see the same favicon for my inner folder: http://forums.xisto.com/no_longer_exists/ in both Firefox and IE 7May I know what browser you are using? Please check http://forums.xisto.com/no_longer_exists/, If you can see the FavIcon there, then that will be the problem with html codes in your site! -
Thanks a lot friend! I think, BartPE will be suitable for me! I will try to create one BartPE boot CD One more question: Is there anyway to get write access on NTFS partition while using Knoppix LIve CD?? Knoppix is the my fav Live CD ever Niru
-
Hi all... Did anybody checked the new cute face of phpbb.com It was back for some time and now back with cool amazing look! Just check phpbb.com (Bad news: they are working on MOD display and it wont be available to download for some time ) Niru
-
I recommend you to use Better captcha from here: https://www.phpbb.com/community/viewtopic.php?t=473222 one more is here: http://forums.xisto.com/no_longer_exists/ After installing the same, the spam bot registration on my forum has been reduced ( No its totally solved : ) You can see my custom captcha image here in my forum registration page: http://forums.xisto.com/no_longer_exists/ You can change the background properties of the captcha images and many more custom options will be there on admin control panel! You can use some new fonts and update the captcha system regularly! Niru
-
Best Host (free) To Host Audio,video And Image Files.
niran replied to richierich1m's topic in General Discussion
I think, all those above mentioned sites, is only for file sharing by uploading them to server and giving download links to user! Hot linking is not supported in those sites! The users need to go to the site and wait till the counter reaches 0 and they need to click the download link! But "richie" is asking for the server, where we can upload files like image, audio or video, wat ever it is, and we can simply embed them in to our websites! like, <img src="http://remotesite.com/imageurl> Many of those free file uploading sites wont support direct linking for video or audio files! Try to use http://www.tripod.lycos.com/ and upload your files there. they will allow hot linking of media files ( but with some bandwidth limitations ) Many of the free web hosting sites, wont support hot linking of files which is having a size greater than 100 kb or 250 kb etc -
Grand Theft Auto: San Andreas Come to talk about this game
niran replied to iGuest's topic in Computer Gaming
San Andreas is having biggerr city size while comparing to older versions like GTA Vice city and GTA 3. I'm a big fan of all the Rock Star City Games ( Including Maxpayne 2) GTA series is rocking with some realistic effects, and in short we can live in our Computer!! Its creating a virtual world inside our room! -
Hi all, Can anybody suggest me some good live CDs that can be run directly by using Bootable CDs without installing the particular OS in my system! I need to get read and write access on NTFS file systems! I need some good alternative to the Knoppix Live CD! Its not allowing me to write or edit files on my NTFS partitions! Thanks, Niru
-
Hi all.. Thanks for all your supports! I'm adding some CSS resources that can be useful to create nice looking menus and styles for your websites! ( Collected from various sites) ADxMenu : multiple menu examples at aPlus A drop-down theme : at CSS Play Bookend Lists: Using CSS to Float a Masthead : at WebSiteOptimization Bulletproof Slants : demo at Simplebits Create a Teaser Thumbnail List Using CSS: Part 1 : lists of items made up of a title, short description, and thumbnail. Creating Indented Navigation Lists : A multi-level indented list 11 CSS navigation menus : at Exploding Boy 12 more CSS Navigation Menus. : at Exploding Boy 14 Free Vertical CSS Menus : at Exploding Boy 2-level horizontal navigation : demo at Duoh Absolute Lists: Alternatives to Divs : An approach of using lists instead of divs at evolt Accessible Image-Tab Rollovers : demo at Simplebits Centered Tabs with CSS : at 24ways Clickable Link Backgrounds : A bulletproof unordered list of links, each with a unique (purely decorative) left-aligned icon that is referenced with CSS ; but that is also clickable. CSS-based Navigation : demo at Nundroo CSS: Double Lists : A single list that appears in two columns CSS Mini Tabs (the UN-tab, tab) : demo at Simplebits Creating Multicolumn Lists : at Builder.com cssMenus - 4 Level Deep List Menu : at SolarDreamStudios CSS and Round Corners: Build Accessible Menu Tabs : at SitePoint CSS-Based Tabbed Menu : a simple tabbed menu. CSS Tabs : tabs without any images CSS Tabs : list of various tab solutions CSS tabs with Submenus : at Kalsey. dTree Navigation Menu : Javascripts Tree at Destroydrop CSS only dropdown menu : at CSS Play CSS only flyout menus : at CSS Play CSS only flyout/dropdown menu : at CSS Play CSS only flyout menu with transparency : at CSS Play CSS only vertical sliding menu : at CSS Play CSS Swag: Multi-Column Lists : at A List Apart Flowing a List Across Multiple Columns : A table without using tables. Free Menu Designs V 1.1 : ready-to-download block menusat e-lusion FreeStyle Menus : XHTML compliant, CSS-formatted menu script at TwinHelix Definition lists - misused or misunderstood? : appropriate uses of definition lists Do You Want To Do That With CSS? - Multiple Column Lists : multi-column lists. Drop-Down Menus, Horizontal Style : at A List Apart Float Mini tabs : at Web-Graphics Hidden tab menu : at CSS Play How to Style a Definition List with CSS : at WebReference How to Style an Unordered List with CSS : at WebReference How to Use CSS to Position Horizontal Unordered Lists : at WebReference Hybrid CSS Dropdowns : at a List Apart Inline Mini Tabs : at Web-Graphics Intelligent Menus : CSS and PHP menu at PhotoMatt.net Listamatic : simple lists; various styles. Listamatic2 : nexted lists; various styles Menus galleries in CSS and XHTML : multiple examples and downloads at Alsacreations Mini-Tab Shapes : demo at Simplebits Mini-Tab Shapes 2 : demo at Simplebits More than Just Bullets : at W3.org Multiple Column Lists : at css-discuss Inverted Sliding Doors Tabs : at 456BereaStreet Light Weight Multi Level Menu : at CssCreator List Display Problems In Explorer For Windows : list hack for IE A Navbar Using Lists : A lightweight nav bar at WestCiv Navigation Matrix Reloaded : at SuperfluousBanter Remote Control CSS : examples of lists styled differently Remote Control CSS Revisited - Caving in to peer pressure : multi-column remote control Rounding Tab Corners : by Eric A. Meyer. Tabtastic : Gavin Kistner. Tabs Tutorial at BrainJar Taming Lists : at A List Apart Turning a List into a Navigation Bar : at 456BereaStreet Ultimate css only dropdown menu : at CSS Play Simple CSS Tabs : at SilverOrange Simplified CSS Tabs : demo at Simplebits Sliding Doors : at A List Apart Spruced-Up Site Maps : sitemaps as lists Styling Nested List : at SimpleBits Suckerfish Dropdowns : at HTMLDog Thanks, Niru
-
But site is not loading friend!! I can ping the server! and getting response from the server! But its not opening in the browser!! Its showing as Hope, you guys will fix the issue soon! Niru
-
Dynamic Background Server Hosted Sig
niran replied to master_nero's topic in Graphics, Design & Animation
Hey dude!! thats looking nice! How did you created this one?? If you dont mind, please share the code with us!! I know some sites, that provide some codes, using that, we can display visitor details on our Signature images! But I dont have any idea about the coding behind those scripts Niru -
Logo Trouble Image just shows an x
niran replied to lonebyrd's topic in Graphics, Design & Animation
Its working fine for me too!! Are you using any ad blocking services?? Some ad blockers will block with file names such as *logo* *banner* like that!! Just give some other name and test it! The image is loading correctly for all of us Niru