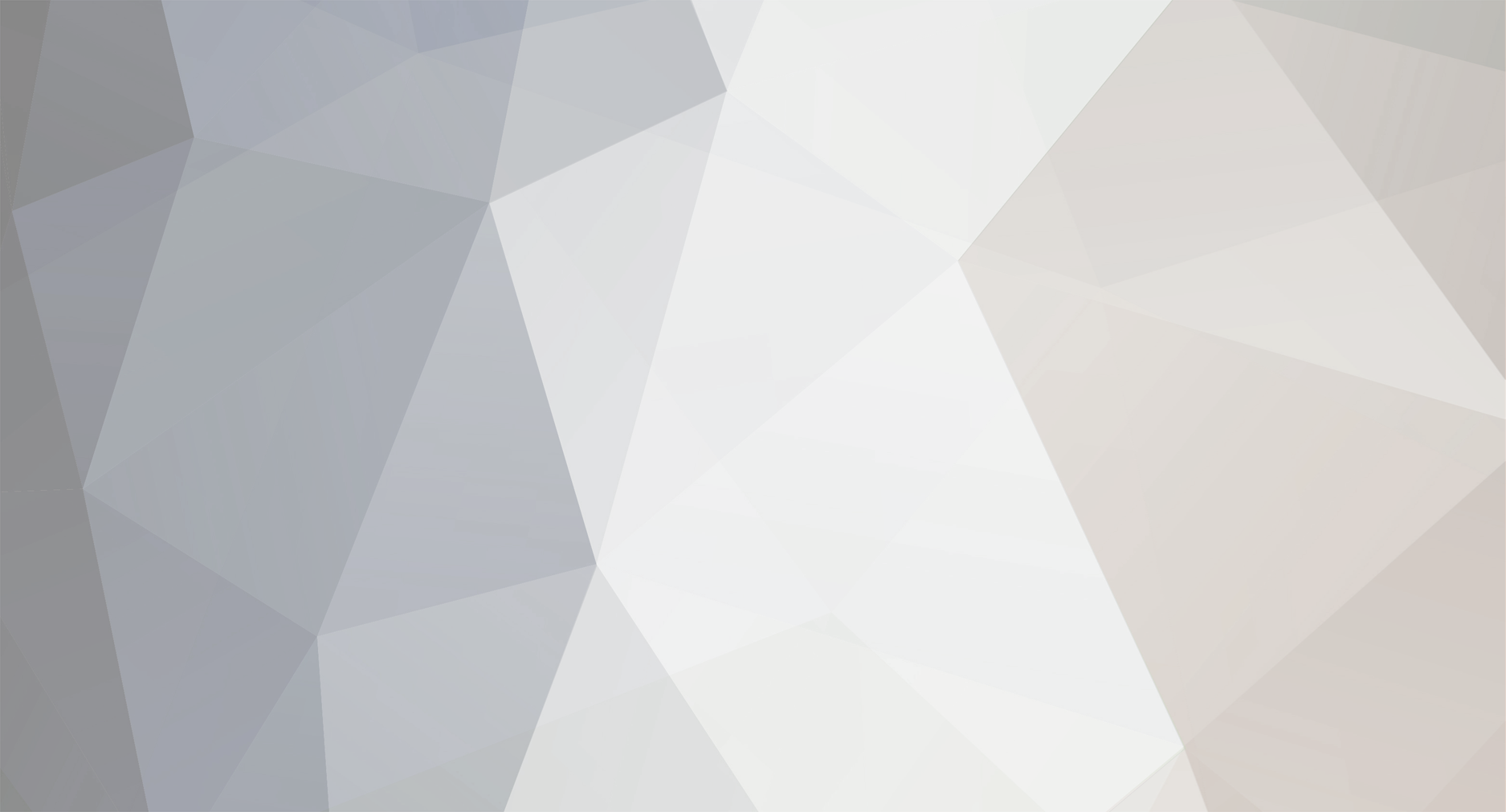
iGuest
Members-
Content Count
72,093 -
Joined
-
Last visited
-
Days Won
5
Everything posted by iGuest
-
Hi everyone, I'm going to tell you how to make a simple shoutbox using PHP and MySQL. To start off, open up mysql in the command line, or phpmyadmin, and create a database called shoutbox. Next, enter the following sql into the command line, or the phpmyadmin sql box, while using the shoutbox database: create table messages(author varchar(30), message text, time timestamp, mid int auto_increment, primary key(mid));This creates the table we need to store the messages, note the "mid" column, this gives each message a seperate id number, we'll see why that's useful later. Next, create a new PHP page on your server, lets call it shoutbox.php. Copy this code into it: <html><head><title>My Shoutbox</title></head><body><center><b>My Shoutbox</b></center><center><table width="80%"><!-- Shoutbox update --><!-- Shoutbox update --><!-- Shoutbox posts --><!-- Shoutbox posts --><!-- Shoutbox form --><!-- Shoutbox form --></table></center></body></html>Ok, now we start on the actual shoutbox. In between the "<!-- Shoutbox form -->" lines, type the following text, we're going to make the shoutbox form. <tr><td><form name="shoutbox" action="?action=shout" method="POST"><label for="name">Name:</label><input type="text" name="name"><br><textarea name="message" cols="20" rows="2"><br><input type="submit" value="Shout!"></form></td></tr>Ok, this gives us a place to write a name, and a message. Note that in the "action" attribute of the form, I've put "?action=shout", if the page is called shoutbox.php, it will take us to shoutbox.php?action=shout. This uses a GET variable, which we will talk about below. Now, we're going to make the section that updates the shoutbox. This is where we get into some actual PHP coding! Between the "<!-- Shoutbox update -->" lines, type the following text: <?phpmysql_connect("hostname","username","password");mysql_select_db("shoutbox");if ($_GET["action"]=="shout"){$update = "insert into messages values('".$_POST["name"]."','".$_POST["message"]."',NULL,NULL);";mysql_query($update) or die("Failed to update database. The error returned was:<br>".mysql_error());}?>Not much, is it? But it does what we need. First, it connects to mysql. Change the text in bold to your own mysql hostname, username and password. Then, it selects the database. If your database isn't called shoutbox, change this bit. Then, it checks to see if the GET variable action is set to "shout." Get variables are those things you sometimes see in urls, you know, when there's a ?something=something&hello=whatever after the address. These are get variables. In this example, submitting the form takes you to shoutbox.php?action=submit, so the action GET variable is set to "shout." Next, it performs the MySQL query, which inserts the data from the form into the shoutouts table. There's also a bit of code to show us whet the problem is if it doesn't work. Ok, now for the final bit, the posts. In between the "<!-- Shoutbox posts -->" lines, write the following text: <?php$query = "select * from messages order my mid desc";$result = mysql_query($query);while ($array=mysql_fetch_array($result)){echo "<tr><td>".$array["message"]."<br><font size=\"small\">Posted on ".date("D j/n/Y g:i:s a",$array["time"])." by ".$array["name"]."</font></td></tr>";}?>Ok, what this does, is it starts off by getting the data from the database, and ordering it by it's unique id, descending. It then writes the information into a table cell once for every entry in the table. The date function makes a readable date out of the time from the database. By now, the page should look like this: <html><head><title>My Shoutbox</title></head><body><center><b>My Shoutbox</b></center><!-- Shoutbox update --><?phpmysql_connect("hostname","username","password");mysql_select_db("shoutbox");if ($_GET["action"]=="shout"){$update = "insert into messages values('".$_POST["name"]."','".$_POST["message"]."',NULL,NULL);";mysql_query($update) or die("Failed to update database. The error returned was:<br>".mysql_error());}?><!-- Shoutbox update --><center><table width="80%"><!-- Shoutbox posts --><?php$query = "select * from messages order my mid desc";$result = mysql_query($query);while ($array=mysql_fetch_array($result)){echo "<tr><td>".$array["message"]."<br><font size=\"small\">Posted on ".date("D j/n/Y g:i:s a",$array["time"])." by ".$array["name"]."</font></td></tr>";}?><!-- Shoutbox posts --><!-- Shoutbox form --><tr><td><form name="shoutbox" action="?action=shout" method="POST"><label for="name">Name:</label><input type="text" name="name"><br><textarea name="message" cols="20" rows="2"><br><input type="submit" value="Shout!"></form></td></tr><!-- Shoutbox form --></table></center></body></html>And there you have it! Your very own shoutbox! Feel free to style is, and modify it anyway you want. You can add validation code, and functions to delete posts! - Jay
-
Also, on most servers, you cannot put php scripts in .htm/.html files, although that can be changed. If you wish, you can make one .php file, and include other .php files in it, if you have a lot. E.g. <html><head><title>Test Page</title></head><body><p>There's some included scripts below this!</p><?php include("[b]script.php[/b]"); ?></body></html>Replacing script.php with the filename of your .php file.
-
Colour-coded Html Editors What do you use?
iGuest replied to iGuest's topic in Graphics, Design & Animation
Hey guys, thanks for the editors.I had a look at some of them, and I think I'll use EditPlus. It has all the color-coding i need, and I especially like the sort of tab-system.I just looked at the homepage, and it turns out you have to pay. Didnt see that .I think I'll try out HAPEdit. It also has tabs, and seems to have the right colour-coding I need! -
Yes, I recently tried out my website in Safari (The Windows version) and it wouldn't work with filter or -moz-opacity, so I tried Opacity like you suggested, and now it works. Thanks!
-
And as for your website, if it's hosted somewhere, on a webhost, it'll have a server (obviously) and PHP/mysql is usally installed. Some webhosts don't have PHP however, so choose one that does, like Xisto for instance!
-
It has several online multiplayer features, but I'd recommend downloading a DLL for online connection. Like 39DLL.I use it to make games, it's really good. It can do pretty much anything with the right DLLs and Extensions. It's not worth using unregistered though, so if you want to use it, register it!
-
I installed Apache myself
-
Good list! Another thing I might add, is don't use too bright colours for backgrounds, and if you're going to use an image for the background, use content boxes, unless the image is just a faint outline. Also, if you're going to have the site in a certain font, don't use <font> tags around the whole thing, use CSS.
-
Thanks for the list! I've often thought when I'm testing my site in Firefox that there ought to be a few more validators, debuggers etc. These will really help! thanks!
-
Hey everyone, I'm looking for a new HTML colour-coded editor. The one I use at the moment is called Vim, and it's mainly text-based, which can get annoying sometimes, so I need a different one. What I need is a simple editor, that will color-code PHP, HTML and JavaScript. It has to recognise functions/tags, e.g, color-code "<br>" bot not "<afds>." And it needs to be small. I'd prefer not to use One built-in to a WYSIWYG editor, Because those programs take a while to load. I need something quick that i can just right-click -> Open with and will pop up immediately, because with some of the larger WYSIWYG programs, once you've got past the splash screen, and the tip-of-the-day, it's usually been at least 20 seconds. What do you use? Any suggestions?
-
My guess would be, MySQL isn't configured properly with Apache. try looking on the Apache site, and the Mysql site, to see if there's any additional configuration you need to do. Have you been able to use Mysql with anything else on your Apache Server?
-
I'd use PHPBB3. They have a great sub-forum system. It's all based off forums now, no categories. You can create a forum, and it can either be a category, link, or forum, and you can give it a parent, which is how you use sub-forums
-
Anyone here use google Apps? If you don't you should. Some of the features are: Email Use Gmail with your domain. Your email address is yourname@yourdomain.com, and you get to use the GMail WYSIWYG editor to create emails. It has powerful Spam protection also. In all my time of using Gmail (With and without google Apps) I've never had any Spam go unfiltered. Another feature of Gmail is conversations. If you've replied to an email, and someone's relied back, and so-on and so-forth, you can view all emails in one window, and they appear as one email, instead of cluttering your inbox. Also, if you're replying to an email, or reading it, if the person replies, a message will popup and you can view their reply right away. Chat You can also use Google chat to chat to people with email accounts at your domain. Google Chat is a Instant Messenger program that usually allows you to chat with other Gmail users. In this case however, you chat with other people from your domain. Google Chat also has the additional feature of notifying you when you have new mail on Gmail, and allowing you to access it on-the-spot. Pages Google also have their own WYSIWYG Web Editor, you can choose from a variety of templates, then create, move, edit and delete elements such as text, images etc. anywhere on the page. Great for beginners at HTML who want their site to look professional! Docs & Spreadsheets Pretty much Google's online answer to Microsoft Word and Excel! You can create advanced online documents and spreadsheets, and save them! Great when you need to create Documents/spreadsheets on-the-go, on computers that don't have any good office programs, or in internet cafes What's even better, is you can either access them via google, or point them at your own domain/subdomain! E.g. for your email, you can access it via mail.google.com/a/yourdomain.com, or email.yourdomain.com! If you're having trouble configuring it to work with your website, don't worry! Google has specific walkthroughs for many different webhosts! To sign up, go to https://www.google.com/enterprise/apps/business/ Note: It takes some time for Google's servers to update, and get your domain working 100%, but this should only take a few hours if you configured it correctly.
-
You could try hosting it on your own computer. That's be slower though. I'd use sczone. 2.50/month is the best i've seen apart from Listen2myradio, which, is better not to look at at all
-
Yes, I use filter, for IE, and -moz-opacity for Firefox/Mozilla What browser is "opacity" for? I've never seen it.
-
How To Program A Counter? counter for clicks on picture
iGuest replied to darul0r's topic in Programming
You should use php with mysql. Make a table with a field for the image filename, and a number of clicks, then put something like this on the page that the image takes you to. <?php$connection = mysql_connect("host","username","password");mysql_select_db("database");$select = "select clicks from table where imagename = 'Image filename';";$sresult = mysql_query($select);$sarray = mysql_fetch_array($sresult);$clicks = $sarray+1;$query = "update table set clicks = '".$clicks."' where imagename = 'Image filename';";mysql_query($query);mysql_close($connection);?>That's assuming you know the basics of PHP and MySQL. If not, I can tell you in full. -
I recently found out that you can put transparency in websites using CSS, (I didn't know that!), and I'm making a website that contains transparent panels. With Internet Explorer you can only use transparency with absolute positions, which is a bit of a nuisance.Would/do you use transparencies? They lopok good, but if theyre too transparent, it can be hard to read text, and some form elements still appear opaque in IE, which makes it look weird.
-
Game Maker's great. You can do heaps. I've used it for about 3 years now, and I'm really good at it. I can make good games now. I went off it a bit last year, but Im making new games now. Its actually one of the easiest languages to learn. The action libraries are good to get you started, and then you can learn how to code. Its not just for games, you can create all sorts of things with it.There aren't any ads in it Sten, unless you count the bar up the top when you load games made with the unregistered version, and that's always been there.Definitely wort getting, and registering
-
Just thought I'd post about the differences between a serverside language, and a clientside language. Alot of people get them confused easily Clientside: Scripts are placed inside a <script> tag in the page. Visitors are able to see the scripts in the source code of the page. The scripts are run on your computer. Advantages: Can do more, e.g. make items move. Can perform actions without having to reload the page first. Can be used with HTML tags. (e.g. onLoad, OnMouseOver) Are used to make rollover buttons, which are an important part of many websites. Disadvantages: Scripts are interpreted at the visitors browser, if their browser is out-of-date the website will not display properly Not secure, anyone can look at the code in the page source Can slow down old computers Some browsers disable active content, such as clientside scripts and tell the user they may be harmful Some common uses: Button rollover effects Cursor trails Form verification Creating cookies Serverside:Scripts are placed inside a <? tag in the page. Visitors are not able to see the scripts. The scripts are run at the server, then the result is sent to your computer. Advantages: More secure, visitors aren't able to view scripts All scripts are performed at the server, it will work with any browser You are able to include external files, to save coding You can combine it with programs such as MySQL Disadvantages: Scripts can only be performed when the page loads/reloads When moving sites, some code may have to be modified, depending on the configuration and version of PHP In most languages, variables must be preceeded with a $ sign All lines must end in a semicolon ( Some common uses: User management File includes Database communication Finding $_GET variables (e.g. index.php?variable=value) I hope that helped you decide which is the best for you! So you're less and more !
-
What's your favourite forum software, and where do you prefer to host it? My fave is IPB, but it costs too much <_<I prefer to host them on my own site, so I usually only use free hosting. PHPBB3, or SMF is good. XMB's too complicated Anyway, what are your views?
-
Firefox's Bookmark manager will 'export' your list of bookmarks to an html (I think) file in order to save the current list of Bookmarks. And it will also 'import' them back from the same file format.Would this feature solve the problem you are facing?
-
add FireFTP to that list of Add-ons. An easily configurable FTP Client that allows you to retain several different FTP logins and will position the Local and Remote Folders according to the User you select for connection.Saves a whack of time in navigating around before uploading/downloading.
-
How To Limit The Number Of Pages In Ms Word?
iGuest replied to miCRoSCoPiC^eaRthLinG's topic in Software
Think about it this way... after they wrote the Operating Systems to be as bloated as they are, why would they allow you to limit the size of Word Documents?