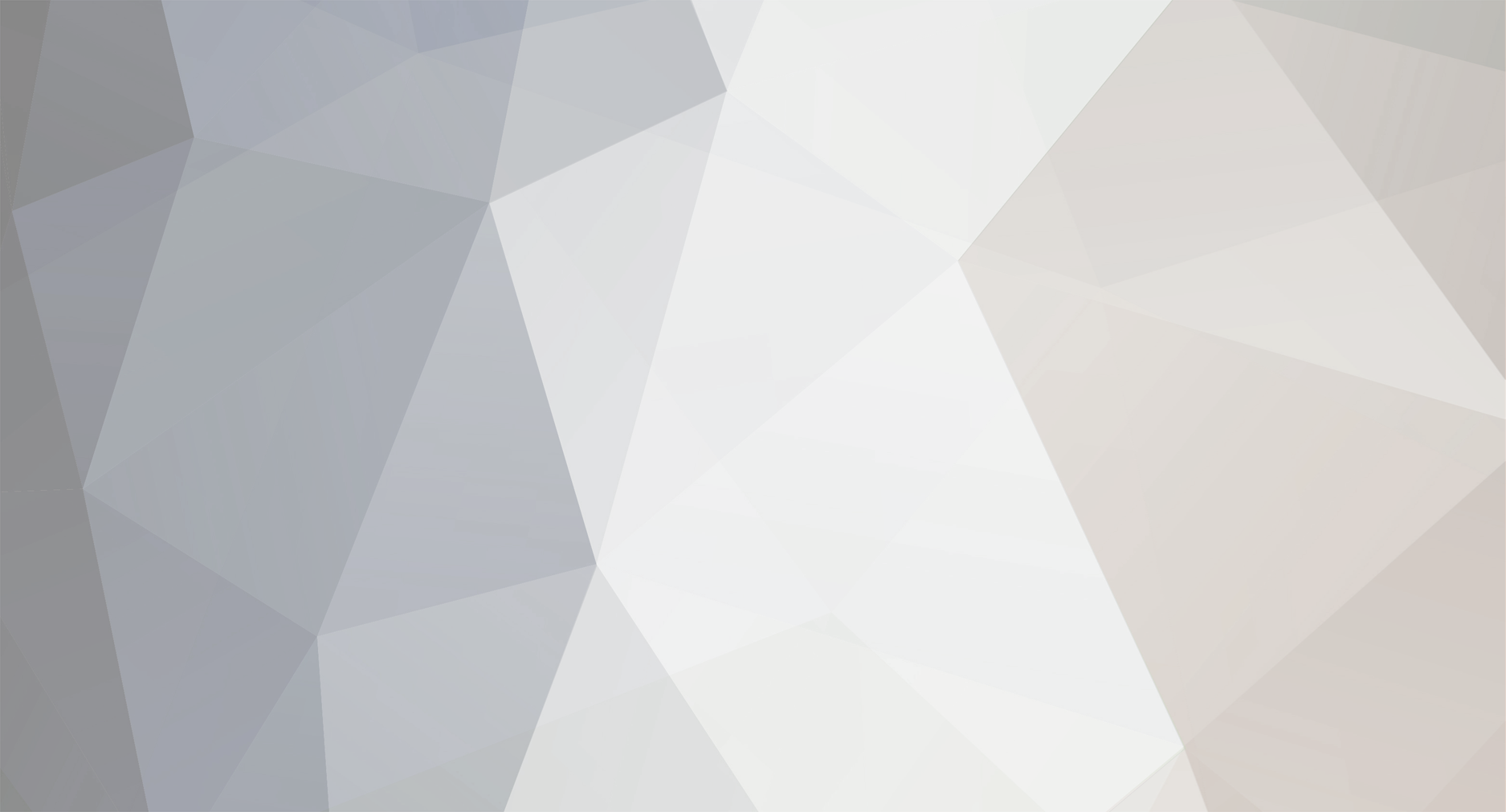
turbopowerdmaxsteel
Members-
Content Count
460 -
Joined
-
Last visited
Everything posted by turbopowerdmaxsteel
-
I didn't know that. Now I can finally format my system without the fear of my long time friends being wiped out. It seems like they use pre-defined messages for similar issues. I wonder what good are the so-called tech support personnel. Powerful atomated scripts would do better job than this. That whole feedback reminds me of our mobile service provider's customer care representatives who say "We are undergoing server updation. Please try again later." for every issue you bring in to their notice.
-
Variables A Variable is a named location that stores a value. Although variables are physically stored in the RAM, their actual memory address is not known to the programmer. We can use the variables via the name we give them. These are the rules for naming a variable:- The name must begin with a letter or an underscore & can be followed by a sequence of letters (A-Z), digits (0-9) or underscore (_) Special Characters such as ? - + * / \ ! @ # $ % ^ ( ) [ ] { } , ; : . cannot be used in the variable name. A variable name must not be the same as a reserved keyword such as using, public, etc. The name can contain any number of the allowed characters Variables with the same scope cannot have the same name Note: C# being a case-sensitive language, two variables such as var1 and Var1 would be different. Any Variable has a type associated with it, which basically restricts the type of value it can store. The table below shows the Data types available in C#, the size they occupy in the memory and the values they can contain. Variable Declaration Before we can use a variable, we need to declare it. Declaration of variables are done using the syntax:- <data type> <variable name>; For Example:- int Age;char Sex;string Name;bool IsMarried;Variables of the same data type can also be declared using the syntax:-<data type> <variable 1>,<variable 2>, .. and so on; Example:- int Age, RollNum; InitializationWhile declaring a variable, it is possible to assign an initial value to it. This operation is called Initialization and it has the following syntax:- <data type> <variable name> = <value>; Example:- int Age = 20;char Sex = 'M';string Name = 'Max Steel';bool IsMarried = false;Multiple variables of the same data type can also be initialized in a single line of code using the syntax:-<data type> <variable 1> = <value>, <variable 2> = <value>; int Age = 20, RollNum = 69; Value TypesValues can be stored in variables by two methods which are: by value and by reference. Value types store the supplied value directly in the memory whereas reference types store a reference to the memory where the actual value is located. The benefit of the reference type is that changes made to any alias of the variable will reflect across all of its aliases. Accepting Values in Variables To input values from the user at runtime, we use the Console.ReadLine() function. For Example:- string FirstName = Console.ReadLine(); The above statement would declare a string variable FirstName and store the value retrieved from the user in it. Note: Console.ReadLine() returns value as a string. Therefore, one must use type casting to convert the value to the required type. We use the member functions of the Convert class to do this. For Example: int Age = Convert.ToInt32(Console.ReadLine());. Similarly, there are other functions such as ToChar(), ToString, etc for conversion to other data types. Operators Similar to mathematics, every programming language has its own set of operators, be it arithmetic or logical. Operators enable us to perform operations on 1 or more values (operands) and calculate the result. Usage Depending on the number of operands they take, operators can be either unary or binary. The use of an operator takes one of the following form:- Binary Usage <Operand 1> <Operator> <Operand 2> Unary Usage <Operator> <Operand> <Operand> <Operator> Classification The operators in C# can be classified as follows:- Arithmetic operators - Just what the name suggests, these operators are used to perform arithmetic operations. Arithmetic Assignment Operators - These can be considered as shortcuts as they are used to perform both arithmetic and assignment operations in a single step. Increment / Decrement Operators - These operators are used to increment or decrement the value of an operand by 1. Difference between Pre & Post Increment/Decrement Consider the following code snippet:- int A = 0;int B = A++;int C = ++A;At the very first line, we have used the Arithmetic Assignment operator, = to assign the value of 0 to A. In the proceeding lines we have used both Pre & Post increment. At line 2 int B = A++; the operation being post increment, the value of A i.e 0 is assigned to B followed by the increment of Aâs value. At line 3 int C = ++A; the current value of A, i.e. 1 is incremented by 1 following which the new value of A is assigned to C. Therefore, the value of A = 2, B = 0 and C = 2. Comparison Operators - These operators are to compare two values and return the result as either 'true' or 'false'. Logical Operators - These operators are used to perform logical operations and return the result as either 'true' or 'false'. Displaying Variables We us the WriteLine method to display the value stored in variables. Displaying Single Variable int A = 6;Console.WriteLine(A); Displaying Multiple Variables int A = 6, B = 9;Console.WriteLine("{0},{1}", A, B);Notice the code "{0},{1}". This is the format for the output to be generated by WriteLine. Since, we are displaying two variables, we need to specify the format accordingly. Here {0} and {1} represent where the proceeding variables A & B will be displayed. Now take a look at the following code snippet:- int A = 6, B = 9;Console.WriteLine("The value of A = {0} and B = {1}", A, B);The output of these lines would be: The value of A = 6 and B = 9. As you can see, the {0} and {1} are replaced by the variables A and B. 0 and 1 correspond to the index of the variables following the format, starting from 0. Comments Software applications are made by a group of coders. Each one of them handles various aspects of the application. Its important to write descriptive texts in order to help other coders understand, the mechanism of the codes you have written. We use comments denoted by // or /* and */ for this very purpose. Example usage:- // This is a comment /* These are two linesof comments */Note: The compiler ignores all comment entries. // is used for single line of comment while /* and */ are used for multiple lines. What's in a Semicolon? Whitespace except when used inside quotes, is ignored in C#. All statements in C# are terminated by using the semicolon. This allow spanning of codes across multiple lines. The following lines of code are functionally equivalent. int A = 6, B = 9; Console.WriteLine("A + B = {0}", A + B); Console.ReadLine(); int A = 6, B = 9;Console.WriteLine("The sum of {0} and {1} = {2}", A, B, A + B);Console.ReadLine(); Previous Tutorial - Lesson 1 - Hello World Program Next Tutorial - Lesson 3 - Programming Constructs Lesson: 1 2 3 4 5 6 7
-
This is the second installment of my attempt to put together what I have learnt from the MCAD/MCSD self paced learning kit. Web Programming is the process of creating Internet Applications. Any application that uses the Internet in a way, can be considered an Internet application. They can be classified into four common categories:- Web Applications - Applications based on the Client/Server architecture over the Internet. The Client/Server architecture is composed of a server, which is responsible for providing services to the other computer systems - Clients. Typically, there is a single server which handles requests from multiple clients and responds to these requests by providing the client with the appropriate information. In a Web Application, the server is the machine where the web page is stored and the clients employ web browsers to view the application. Such a server is called a Web Server. Web Services - Web Services are components that expose processing services from a server to other applications over the Internet. The services themselves are executed remotely in the server hosting them. Internet Enabled Applications - Any stand-alone application that uses the Internet falls into this category. Such an an application uses the Internet for online Registration/Activation, Help, Updates, etc. Peer-to-Peer Applications - These are stand-alone applications that use the Internet to communicate with other users running their own instances of the application. They use decentralized network architecture where there is no central server, rather individual nodes. Examples of such applications can include the famous Bit Torrent client. Note:- In this tutorial, we would be involved with Web Application only. Working of the Web Applications As mentioned before, the client side of the Web Application includes a web browser, which interprets Hypertext Markup Language (HTML) transferred by the server and displays the user interface. The server itself runs the web applications under Microsoft Internet Information Services (IIS) which is responsible for managing the application, passing request from clients to the application and returning the application's responses to the client. The intricate communication involved in this process is done by using a standard set of rules (Protocols) known as the Hypertext Transfer Protocol (HTTP). The responses generated by the Web application is made from the resources (Executable code running on the server, Web Forms, HTML pages, images and other media files) found on the server. These responses are similar to traditional Web sites with HTML pages, except that they are dynamically generated. Consider a university's web site which releases the exam results. Do they take the pain of creating different HTML pages for each of the student's mark sheet? No, they use web applications to retrieve data (marks, subjects, student name, roll no, etc) from a database and dynamically generate the HTML output which is then sent to the client's browser. The executable portion of the Web application is responsible for overcoming the limitations of static web pages. They can be used to:- Collect information from the user and store the information on the server (in a database). Performing tasks for the user such as placing an order for a product, performing complex calculations, or retrieving information from a database. Identify a user and present customized user interface. These are just a few uses. The actual possibilities with Web applications are endless. ASP .NET ASP .NET is the platform that allows us to create Web applications and services that run under IIS. One must note that ASP .NET is NOT the only platform to develop Web applications. Other platforms such as Common Gateway Interface (CGI) can also be used to create Web applications. ASP .NET is unique in the way it is tightly integrated with Microsoft server, programming, data access and security tools. It forms a part of the Microsoft .NET suite of products and is composed of:- Visual Studio .NET Web Development Tools - These are Graphical User Interface (GUI) based tools to facilitate easy designing of Web pages using What You See Is What You Get (WYSIWYG) editors, project management and deployment tools. The System.Web namespaces - These form a part of the .NET Framework Base class libraries and include the programming classes that deal with Web specific items such as HTTP requests and responses, browsers and e-mail. Server and HTML Controls - The user interface components such as Text Box, label, Button, ListBox, etc. that are used to gather information from and provide to users. Microsoft ADO.NET database classes and tools - Database access is one of the key components of modern Web applications. These tools provide methods to access and use Microsoft SQL Server and ODBC databases. Microsoft Application Center Test (ACT) - Testing environment for Web applications. Note:- ASP .NET requires a Windows Server with IIS in order to run. Why Choose ASP .NET? The following are the advantages that ASP .NET has over other platforms:- Faster execution - Executable portions of Web applications are compiled to facilitate faster performance. On the fly updates of deployed Web applications thus preventing the need to restart the server. The amount of code to be written is greatly reduced because of the access to .NET Framework base class libraries which includes classes and methods to perform common operations. Language independent - Developers have the choice to write codes in the friendly Visual Basic programming language or the type safe C# language. Other third party .NET compliant languages can also be used. Automatic state management for controls on a web page (server controls) makes the controls behave more like the Windows controls. New controls can be created and existing controls can be extended. Built in security through the Windows server or through other authentication/authorization methods. Integration with ADO .NET to provide database access and database design tools from within Visual Studio .NET Full support for Extensible Markup Language (XML) and Cascading Style Sheets (CSS) Automatic intelligent caching of frequently requested Web pages, localizing content for specific languages and cultures and detecting browser capabilities. In the next tutorial we will be venturing into the world of coding with our first Web application. We will also take a look at the fundamental differences between the two heavweight languages VB and C#. Previous Tutorial - The .NET Framework & CLR : Basic Introduction
-
This is the first installment of my venture to explain the bits and pieces of C# that I have managed to learn at NIIT. In this lesson we would be creating the Hello World application. STEP 1: Create a New Console Application in Visual C# The IDE automatically adds the following code:- using System;using System.Collections.Generic;using System.Text; namespace HelloWorld{ class Program { static void Main(string[] args) { } }}Lets take a look at the first 3 lines. All of these lines begin with the keyword using. The .NET Framework has a huge set of classes. They are grouped under namespaces for easy accessibility and better categorization. For Example, the System.IO namespace contains all the classes, functions & methods required for Input Output operations, thus making life easier for us human beings. While the namespaces have remarkable benefits, they tend to bring in some potential inconveniances too. Take the System.Convert.ToString() method for example. The method has to be called along with its complete path in the hierarchy, a really tiresome process to do repeatedly. This is when the using keyword comes into aid by importing the namespaces. When imported, the classes inside the namespace can be accessed just by their names. Any .NET application that we make, has to reside in a namespace of its own, so we write the statement namespace HelloWorld. All the classes you create under this namespace can be accessed as NameSpaceName.ClassName. Next we have the class declaration itself. Inside you'll find the static void Main function. This is the entry point for the application. Think of it as the outer door of your house. Any one comming inside has to first pass through it. The entry point must be declared as static because that way the main function can be invoked even without creating an object of the Program class. Also the name of the entry function should be Main, the capital M counts too. Any application can take parameters, when run from the command prompt. Say you ran the Notepad application as follows Notepad.exe MyFile.txt. The text MyFile.txt is the parameter passed to it, Notepad reads this parameter and opens the file. Similarly, we may need to work with arguments passed to your application. This is why we use the string[] args inside the Main function. Up until this point I have described the auto generated code. Now let us type in some code of our own. Keeping with the tradition of the Hello World program we type in Console.WriteLine("Hello World"); inside the body of the Main function. The Console class resides in the System namespace and represents the standard input, output and error streams for console application. The WriteLine method displays the supplied argument(s) on the console. Press F5 to run the application. You'll see a console window with the text Hello World appear. Don't worry if the window closes on its own. Its because the application reaches the end point and exits. You can add the following line to make it halt for an input before exiting: Console.ReadLine(); This is how our final code looks like:- using System;using System.Collections.Generic;using System.Text; namespace HelloWorld{ class Program { static void Main(string[] args) { Console.WriteLine("Hello World"); Console.ReadLine(); } }} Escape Sequences Much like C/C , C# has a list of escape sequences. Escape Sequences or Escape Characters are some special characters which cannot be displayed directly. For Example, the newline character or the Double Quotes. White space being ignored by C#, the newline escape sequence is the only way to display the newline character in the output. The double quotes are used to enclose strings hence they can’t be used directly. To display the special charcters such as these, we use escape sequences. All escape sequences begin with the backslash character \ Example Usage:- Console.WriteLine(”This is a \n multiline text”); Output:- This is a multiline text Console.WriteLine(”C# is a \”Programming Language\”"); Output:- C# is a “Programming Language” Here’s the list of the escape characters in C# Note: Equivalent for Visual Basic’s vbCrLf is \n\r Next Tutorial - Lesson 2 - Variables & Operators Lesson: 1 2 3 4 5 6 7
-
Having cockroaches inside the cabinet is really weird. Even though my room has quite a few of them lurking all around the corners and one of the PCI slot latch being open, I have never seen any of them inside the cabinet. The other common bugs that generally dwell in electronics such as TVs have stayed out of the PC as well. Perhaps, the heat factor attributes to it or maybe the daily usage keeps them at bay.If I were in your position, I would do probably anything to get rid of those bugs. The thought of them eating up the wires, although unlikely, stirs me off. You should certainly try on m^e's formula.
-
Best Download Manager what download manager do you use? and why?
turbopowerdmaxsteel replied to m107's topic in Software
Whatever be the name, Download Accelerator Plus, Internet Download Manager, Flashget, Get Right, Free Download Manager, I've tried it all. Each of them have their benefits and shortcomings. Here's my take on a few of them. Download Accelerator Plus - It is the most popular Download Manager/Accelerator on the planet. The User Interface is neatly laid out. Although, some minor details have been left out. I remember the time I had the 32 kbps Airtel GPRS internet connection with extremely slow speeds; downloads were aborted during limited or no connectivity even though always retry option was selected. It was really annoying to find out huge unattended downloads stuck at low percentages due to this very bug. Internet Download Manager - The fastest Download Manager with intelligent segmenting during download. The User Interface is no match for the likes of Download Accelerator Plus or Free Download Manager, but is workable. Internet Download Manager (IDM) does what it says - download faster. It is stated that it can recover from download errors, however, download errors due to computer crash aren't recoverable. Flash Get - The smoothest of all download managers. With speeds comparable to Internet Download Manager and comparitively better User Interface, flashget heads my list of the best download manager. Free Download Manager - It is quite similar to flashget in terms of UI, has decent download speeds but trails a bit on smoothness. Get Right - With loads of features packed into it, Get Right does have great intentions but seems to be a bit buggy. -
Preferred Forum System So many choices...its overwhelming
turbopowerdmaxsteel replied to FirefoxRocks's topic in Software
I have tried my hands on a few forum systems, namely - phpBB, VB, IPB & SMF. The new IPB rules the list, while phpBB is the best of the free ones. Being Open Source and backed by a huge community, the possibilities with phpBB are endless. While the Gold version of phpBB2 lacks quite a few necessary features, most notably - Subforums and Attachments, you could be rest assured that there is a MOD available for every such feature. The upcoming version 3 of phpBB - Olympus will include most of the lacking features. Although, it has been stated that not all of the features will be included. After all, they are not trying to mimic other systems. Olympus focuses on improved modularity. While the MODing of phpBB2 is pretty powerful, it does involve significantly high amount of complexity. Traditionally, MODs were installed manually rendering the process extremely time consuming. However, with the introduction of EasyMOD, the inconveniance was greatly reduced. Huge MODs such as Subforum and Attachments leads to growing chance of collision between existing MODs. Olympus aims at resolving this issue by implementing a better modular approach. Coding styles have been altered and it is being said that none of the phpBB2 MODs will work with Olympus. This will hinder the initial spread of Olympus. As the developers start to chip in and start MODing it, Olympus will surely improve on the reputation, some amount of which was lost when the Santy worm which exploited old phpBB installations came to the surface. The worm exploited the security hole by overwriting PHP and HTML pages. Olympus is also aimed at minimizing such vulnerablities. Other features include, the debuting AJAX @ phpBB. However, the extent of AJAX usage will be limited and it is hinted that AJAX support will not be used for quick replies, perhaps due to security concerns. Adding Custom BBCode was quite difficult as the users had to rely on MODing. With Olympus such custom BBCodes can be added through the admin panel itself, much like what IPB allows. Users can now send Private messages to multiple recipients. Jabber support will allow notification via Instant Messaging. From what I saw of phpBB3 Beta 5, the installation has become much more user-friendly. The control panel seems better organized and the number of settings have increased. For a wider list of introductory features in phpBB3, check out http://forums.xisto.com/no_longer_exists/ All in all, if you are looking for a free discussion board for your site, there's nothing better than phpBB. -
New Yahoo! Messenger Protocol Changes?
turbopowerdmaxsteel replied to tansqrx's topic in Search Engines
Whatever the motives are, major protocol changes would render many third party tools (including my Pika Bot )useless. Not that we've got the right to be complaining; after all, its their game and their rules.But, I sincerely doubt that it will happen. They are more concerened on the advertising part as you mention. Engaging work on the protocol wouldn't be ideal for them. However, I do think that they should do something for the bot problem. Its hard to find real people in chat rooms these days. On an average there are 2-4 bots residing in every room, which spam the chat room based on a timer circuit. Then there are the cycler bots, which keep on moving across rooms & posting their advertisements. I've always wanted bots to be able to replicate human chit chat with smart answers. Yet, these days, they are primarily used for no good advertisements. -
Well, I am not an expert at the .htaccess magic. But it does point like that. See this page for example:- http://forums.xisto.com/no_longer_exists/ You'll notice the following code:- <link rel="shortcut icon" href="favicon.ico" />The favicon.ico does not exist in the root folder rather in the wip folder itself. The favicon is shown in both IE7 and Firefox in this case. While check out another page:- http://forums.xisto.com/no_longer_exists/ The code in this case is:- <link rel="shortcut icon" href="findicon.ico" />The favicon exists in the root folder as http://forums.xisto.com/no_longer_exists/. Yet, the favicon is not shown. Now check out this page:- http://forums.xisto.com/no_longer_exists/ The code in this case is:- <link rel="shortcut icon" href="http://ntek.astahost.com/findicon.ico" /> Using the abosule URL solves all hierarchy problem.
-
They revamped the whole look after the major disk failure forced them to shut the site down. Signs are really encouraging for phpBB3. Lets see when it goes gold. The wait has been intriguingly long though. Yes, the coding style has been greatly altered. I have heard that no phpBB2 MOD will work with phpBB3. The new version focuses on modularity which will allow easy Modding and lesser conflicts between existing ones.
-
I did some Testing on this matter & this is what I came up with:- No Link tag in Page:- Firefox - Doesn't display the favicon. IE7 - Looks for favicon.ico in the current folder and displays it if found. Using rel="shortcut icon" Firefox - Displays the favicon when the href points to the correct location or the favicon.ico exists in the current folder. IE7 - Displays the favicon when the href points to the correct location or the favicon.ico exists in the current folder. Using rel="icon" Firefox - Displays the favicon when the href points to the correct location or the favicon.ico exists in the current folder. IE7 - Displays the favicon when the href points to the correct location or the favicon.ico exists in the current folder. Eitherways, the href attribute MUST point to the correct image/icon. Use rel="icon" only when you want an animated favicon (GIF) to be shown. rel="shortcut icon" will work in most browsers as the standard compliant browsers ignore shortcut and use icon, while IE interprets "shortcut icon" as a single name.
-
The problem is that the href attribute for the tag - 'favicon.ico' exists in http://chaos-laboratory.com/favicon.ico and not in http://forums.xisto.com/no_longer_exists/favicon.ico Using the full link http://chaos-laboratory.com/favicon.ico would do the job. <link rel="icon" href="http://chaos-laboratory.com/coding/favicon.ico" type="image/x-icon" /> <link rel="shortcut icon" href="http://chaos-laboratory.com/coding/favicon.ico" type="image/x-icon" /> Although I must say, IE7 is smart and it shows the favicon at http://forums.xisto.com/no_longer_exists/ too.
-
Onward To Domination!
turbopowerdmaxsteel replied to xboxrulz1405241485's topic in General Discussion
Great Job, xboxrulz. Still, 727 is too huge a gap. That would take about 4 years or so on my part. Good Luck on your venture. -
Well, it has been out of beta for a while now. I've been sticking with WMP since v 7 (the one bundled with Windows ME). That was the time, when they really revamped the player. The way it sits on top of the taskbar (WMP taskbar toolbar) comes in real handy, as the entire player doesn't need to be focused for the basic jobs. It has improved album based management, instant search, album info finder and the vista black look, enough to make it my favorite media player. Personally, I don't have any problem with downloading the codecs for out of the block media formats.It does crash once in a while. But one could attribute that to the growing complexity in the software. And let me tell you, Winamp isn't perfect either. I remember, v 3 not starting at all, on many occasions. Re-installing was the only option back then. The last Winamp I tried was v 5 which was a great improvement to the already impressive Winamp.
-
Asp With Astahost How do you get it working?
turbopowerdmaxsteel replied to FirefoxRocks's topic in General Discussion
Astahost does not support ASP, as yet. I use the ads free IPDZ server for hosting my ASP pages. However, there site seems to be down quite frequently. -
Ok I am on it. Making the Find Next & Back work, was such a pain in the *bottom* that I really didn't give enough thought to the replace all thingy. In the testings that I have been doing, it pops up with Replaced (0) Instances after a complete REPLACE ALL. I presume the replace text you are using creates another instance of the find text. For Example:- Find Text: Adam Replace Text: Madam Source Text: Adam ate the apple. Final Text: Madam ate the apple. It would be easier for me if you could attach the files you were testing on. Backups are stored in a new directory 'Backup' which in turn is created in the folder the executable resides. Next release will have location customization feature. I'll include the filters to stop it from doing that.
-
Ok here goes the Work in Progress version. http://forums.xisto.com/no_longer_exists/ Hopefully, this would serve your purpose. Do mention the features you would like to be added/ripped off and any bugs that you encounter (I had to rush things 'cause of the classes). For now, the backups will be stored in the backup directory of the application with a timestamp prefix added to the filename.
-
Logo Trouble Image just shows an x
turbopowerdmaxsteel replied to lonebyrd's topic in Graphics, Design & Animation
Even though I believe your problem has been solved by now. The 'x' mark represents that the image can't be found as per the src tag. The value from the alt tag is used in such cases to describe the purpose of the image. Although, I can see the logo on your page. I reckon you opened the remote page before uploading the image and the browser is using the cached version of your page. Refreshing the page would be enough in most cases. -
That sure is an accurate pie, that it is.Happy Pi Day to you too.
-
For the very fear of ruining the relations, I tried to play it safe & insisted that I didn't doubt the evaluation scheme. Instead I kept on emphasizing that it were my mistakes that I was trying to determine. Anyone could have noticed the lack of reason on their part, but yet, they tried to force it upon me. I kept my cool through the discussion and even left with a thank you, for I believe teachers are to be respected, no matter what happens. Lemme just quote some of the conversation extracts. Faculty 2: You must have rushed the exams and made mistakes. Some of the options are pretty close to each other. Me: I agree that the options were tricky. That's why I took the entire 80 minutes in an exam that should've been completed much earlier. I reviewed every answer time & again after I did logical elimination on the tricky ones. Faculty 2: Study harder and appear for the re-exam. Me: I am sorry sir, even if I were to devote 10 years from now on to learning C#, I would have banked on the same answers. For, I believe there were no mistakes on my part. Me: Afterall, an exam is meant for testing our skills and learning from our mistakes, isn't it? But, how am I supposed to learn from the mistakes, when I can't even find them out. Faculty 2: Did you get a printout of the marksheet? Use it to assess where you made mistakes. Me: We can only see the sections we lost out on, over there. I need to be able to review the wrong answers. Faculty 2: Try to remeber the questions and discuss them with your faculty. Me: How can I remember the case studies which were more than a page long? Faculty 2: I can't show you the answers. Were you shown your answers in the board exam? Me: No Sir, but now-a-days, we can obtain the entire evaluated papers on paying some nominal charges. Faculty 2: If I show you the answers, others would come asking for the answers as well. Me: I am not asking for a hardcopy of the paper. Just pointing the mistakes out would be enough. Faculty 2: I can't do that. That would be against the policy. Faculty 1: Didn't I tell you, that the answers can't be shown. Me: Yes you did sir, but I can't come to terms with the result. Faculty 1: If it were possible, I would surely show you. Friend: Could there've been some problem with the evaluation system? Something that mismatched the answers. Faculty 1: Appear for the re-exam. I'll oversee your answers this time and oversee your answers. If thing's go wrong again, their might be a problem with the system. Me: Alright then, Sir. I am on it. I complied to their terms at that time. But what if I picked the same answers and got more marks this time? They would simply say that I made mistakes the last time around. While an improvement in the marks would surely be good, it would still be mentioned as a supplimentary result. Right now, another friend has voiced his support and he insists we follow the procedures - drop a complaint letter, if it still doesn't serve the job, we should approach the higher authority. True, fluctuations can certainly be there, but within a bounded limit and that limit gets narrower with the amount of grasp you have on the paper. Besides there's more to prediction than just counting the ones you think are correct and calculating the marks accordingly. First you count the ones you are absolutely sure of, that way you determine the lower limit. You score close to this lower limit when the exam goes really bad and getting marks below that is certainly not feasible - as long as the prediction was not flawed in itself.
-
Sorry for the unclear clarification. I thought the example ought to make it clear. Anyways, what you've said is exactly what I want to do. I had no idea that variables are passed across files in such a fashion. Kinda, overrides the variable scope thingy we learn in the other programming languages, doesn't it? I was aware that such a thing could be done in the same file but still couldn't guess this comming. My appologies to you too. I prefer to have different header files, more so because they contain a lot of plain HTML codes and having to resort to echo is really trecherous. I wouldn't ask for server re-configuration either, just something that does the job. Thank You guys, you were of tremendous help.
-
As per the php documentation:- // Won't work; looks for a file named 'file.php?foo=1&bar=2' on the// local filesystem.include 'file.php?foo=1&bar=2';// Works.include 'http://forums.xisto.com/no_longer_exists/;; So, I used the following code to include the header.php file which I use to draw the top navigation menu on my site. <? include "http://" . $_SERVER['HTTP_HOST'] . "/includes/header.php?d=../../" ?> It worked before, but now I see the following errors on my page. I suppose disabling URL file access was meant for security purposes. Is there any workaround?