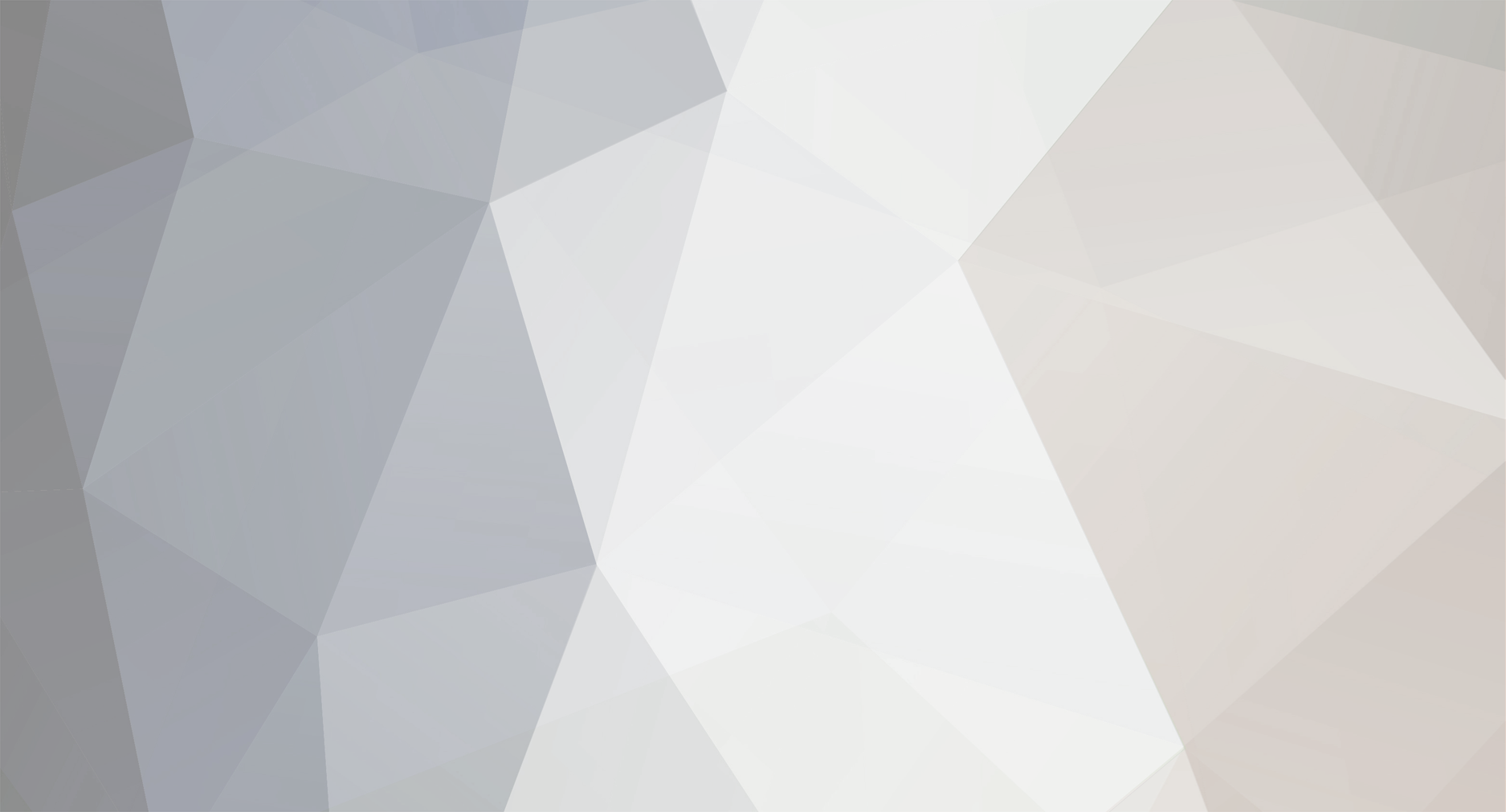
turbopowerdmaxsteel
Members-
Content Count
460 -
Joined
-
Last visited
Everything posted by turbopowerdmaxsteel
-
I have manged to get the job done. What I did was inherit the class fron System.Windows.Forms.Control. This allows Invoke to be called from within the class and thus removes the overhead of having to do so everytime. This comes at a cost, though. The Socket control is no longer the design time only visible thingy. So, I had to handle the Paint messages for the control and it is now quite similar to the Winsock control.
-
The main difference between a programming language and a scripting language is that the former may be compiled into binary code and executed while the latter needs to be interpreted each time. I guess that makes, PHP a scripting language, no matter how powerful or object oriented it is. Source: Wikipedia
-
I am creating a wrapper component for the System.Net.Sockets.Socket class which would resemble the Winsock control. The basic idea is to do operations such as Connect & Receive asynchronously. The component has events such as Connected, PacketArrival in response to these. To do this, I use the following code:- Socket Code Imports System.Net.SocketsPublic Class MySocket Dim Sck As Socket ' Socket Object ' Connect Method Sub Connect(ByVal Host As String, ByVal Port As Integer) Sck = New Socket(AddressFamily.InterNetwork, SocketType.Stream, ProtocolType.Tcp) ' Connect Multithreaded Sck.BeginConnect(Host, Port, New AsyncCallback(AddressOf ConnectCallBack), Sck) End Sub ' CallBack For System.Net.Sockets.Socket's asynchronous Connect Operation Private Sub ConnectCallBack(ByVal ar As IAsyncResult) Sck.EndConnect(ar) RaiseEvent Connected() End Sub ' The event to Notify the Owner Form Public Event Connected()End Class The problem here is that the Connected event is raised on the thread of the asynchronous Connect method of the Socket. So, if I try to access any control from this event's handler, a Cross-thread operation not valid exception is generated. Window Code Public Class Form1 Private Sub Form1_Load(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles MyBase.Load mSck.Connect("microsoft.com;, 80) End Sub Private Sub mSck_Connected() Handles mSck.Connected ' Set the Window Text to Notify this Event Me.Text = "Connected" End SubEnd Class I am able to get past this by following the process stated in MSDN for making thread safe calls. Public Class Form1 Private Sub Form1_Load(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles MyBase.Load mSck.Connect("microsoft.com;, 80) End Sub Delegate Sub ConnectedCallBack() Private Sub mSck_Connected() Handles mSck.Connected Dim D As New ConnectedCallBack(AddressOf SocketConnected) Me.Invoke(D) End Sub Sub SocketConnected() ' Set the Window Text to Notify this Event Me.Text = "Connected" End SubEnd Class While, this works, I am not satisfied with having to create callbacks for these routine events. Is there a way to raise the event in the same thread as that of the Form?
-
Please Review - Maxotek
turbopowerdmaxsteel replied to turbopowerdmaxsteel's topic in General Discussion
The site is pretty much up and running. As of now, I've released two products and in the comming weeks more will be added. Please drop by and have a look on the site at http://maxotek.net/ Regards, Max. -
Unofficial Astahosted Members Directory
turbopowerdmaxsteel replied to turbopowerdmaxsteel's topic in Programming
Yeah v6 is up and running. With v 5, I modified the core code to tackle various issues. The number of sites has certainly decreased, but I thought it was down to the fact that invalid links are not listed. I will look into this matter, right away. Thanx for reporting! Update This issue has now been fixed. After finishing v 4 I started working on the Xisto version. The current versions are modified forms of the same. In Xisto, 30 links are listed per page whereas over here its 20. This is what led to some accounts being skipped. -
Unofficial Astahosted Members Directory
turbopowerdmaxsteel replied to turbopowerdmaxsteel's topic in Programming
After hours of brain-storming, where I had written custom Decimal-To-Binary, Binray-To-Decimal, Bitwise XOR Calculator functions and what not. I figured it was pretty simple to do as all that was required was to make the numbers overflow to the otherside. This flow function is what saved the day:- function flow($num){ // Adjust Overflow if($num > UPPER_BOUND) { $num = LOWER_BOUND + ($num - UPPER_BOUND) - 1; } // Adjust UnderFlow if($num < LOWER_BOUND) { $num = UPPER_BOUND - (LOWER_BOUND - $num) + 1; } return $num;} Here UPPER_BOUND & LOWER BOUND are defined as :- define('LOWER_BOUND', -2147483648);define('UPPER_BOUND', 2147483647); With this, I want to announce the release of v 6 which includes a Page Rank Checker and congrats to vujsa for topping the page rank list with his site http://forums.xisto.com/no_longer_exists/ Script: http://forums.xisto.com/no_longer_exists/ -
Unofficial Astahosted Members Directory
turbopowerdmaxsteel replied to turbopowerdmaxsteel's topic in Programming
This is the range of integers in PHP +2147483647 to -2147483648. When any bitwise operation yields a result beyond these boundaries (more than +2147483647 or less than -2147483648), an overflow/underflow occurs. For example:- The bitwise operator ^ which does the operation XOR on the operands, should return the first number when the second number is 0 (Order is not important). So, the following code should output the number 2147483647 which is what the value of $t1 is. <?$t1 = 2147483647;$t2 = 0;$t3 = $t1 ^ t2;echo $t3;?> Now, if we make $t1 greater than the integer boundary for PHP by substituting the value to 2147483648 (which is one greater than the upper limit), the output is -2147483648. As you can see, an overflow occured and the result changed sign turning to the other side. If, $t1 had a value of 2147483649 (two more than the limit), the output would be -2147483647. This is ok and desired by the Page Rank script during the cheksum calculation. But, cosider the underflow scenario:- <?$t1 = -2147483648;$t2 = 0;$t3 = $t1 ^ t2;echo $t3;?> Here, $t1 is on the edge of the lower limit and the output would be -2147483648. When we set $t1 to -2147483649 (one less than the limit) an underflow should happen and the sign should again change causing the output to be 2147483647. This happens on my localhost running on Win XP x86, but not on the Xisto servers. There the result is -2147483648 (the lower bound), no matter how much underflow occurs. Does anybody have an idea on how to tackle this problem? -
Unofficial Astahosted Members Directory
turbopowerdmaxsteel replied to turbopowerdmaxsteel's topic in Programming
By Historical Page, do you mean a change log? I haven't kept any such log for this script except for my posts here on Asta. Right now, I am trying to Integrate a Page Rank Checker into the script. The integration has been done. The page rank calculator needs to calculate a kind of cheksum for the website. This works on a 32 bit system (like my localhost) but on the Xisto server (which I presume is 64 bit), the cheksum is different because there is no overflow there. I have pin pointed the problem to the XOR (^) operator. The following code yields different results:- $t1 = -4738698913;$t2 = 20;$t1 = $t1 ^ $t2; Localhost: -443731637Xisto Server: -2147483628 It is certainly a case of overflow, because if I reduce the value of $t1 by 1 digit the results are equal. I am trying to learn as to how, the 32 bit machine compensates for the overflow in such cases. I wish I had taken the Computer Architecture classes more seriously. -
Unofficial Astahosted Members Directory
turbopowerdmaxsteel replied to turbopowerdmaxsteel's topic in Programming
Check out the latest version 5 of the script: http://forums.xisto.com/no_longer_exists/ Changes:- > New & Improved Progress Bar. > Profile Override - If found, the site address is taken from the member's profile. Note: Database saving/loading is currently disabled. -
The programming model has gone through many refinements, each change improving upon the model. One of the early changes was the inclusion of Subroutines or Subprograms. As the names suggest, these are fragments of code within a program. They offer the following advantages:- Reduction of code duplication by offering re-usability Simplifying program logic by decomposing the program into smaller blocks Improving readability of the program Why do we need functions? Consider the following program:- using System;namespace ConsoleApplication1{ class Program { static void Main(string[] args) { int JoinDay; Console.WriteLine("Enter the day of Joining:"); JoinDay = Convert.ToInt32(Console.ReadLine()); switch (JoinDay) { case 1: Console.WriteLine("You Joined on a Sunday"); break; case 2: Console.WriteLine("You Joined on a Monday"); break; case 3: Console.WriteLine("You Joined on a Tuesday"); break; case 4: Console.WriteLine("You Joined on a Wednesday"); break; case 5: Console.WriteLine("You Joined on a Thursday"); break; case 6: Console.WriteLine("You Joined on a Friday"); break; case 7: Console.WriteLine("You Joined on a Saturday"); break; } int ResigDay; Console.WriteLine("Enter the Resignation Date:"); ResigDay = Convert.ToInt32(Console.ReadLine()); switch (ResigDay) { case 1: Console.WriteLine("You Resigned on a Sunday"); break; case 2: Console.WriteLine("You Resigned on a Monday"); break; case 3: Console.WriteLine("You Resigned on a Tuesday"); break; case 4: Console.WriteLine("You Resigned on a Wednesday"); break; case 5: Console.WriteLine("You Resigned on a Thursday"); break; case 6: Console.WriteLine("You Resigned on a Friday"); break; case 7: Console.WriteLine("You Resigned on a Saturday"); break; } Console.ReadLine(); } }}This program displays the weekday of joining and resigning based on the day code entered by the user. While, there are no errors in the code, there is a problem - repetition of code. The switch block in the second case (Resignation Day) is more or less the same except for the prefix to the output message being You Resigned on a . Looping is meant to minimize code repetition, but it is not applicable everywhere. In scenarios such as these we should use functions to reduce code repetition. Below is the same program when done by using a function. using System;namespace ConsoleApplication1{ class Program { static void Main(string[] args) { int JoinDay; Console.WriteLine("Enter the day of Joining:"); JoinDay = Convert.ToInt32(Console.ReadLine()); Console.WriteLine("You Joined on a {0}", GetDayFromCode(JoinDay)); int ResigDay; Console.WriteLine("Enter the Resignation Date:"); ResigDay = Convert.ToInt32(Console.ReadLine()); Console.WriteLine("You Resigned on a {0}", GetDayFromCode(ResigDay)); Console.ReadLine(); } public static string GetDayFromCode(int Code) { switch (Code) { case 1: return "Sunday"; case 2: return "Monday"; case 3: return "Tuesday"; case 4: return "Wednesday"; case 5: return "Thursday"; case 6: return "Friday"; case 7: return "Saturday"; default: return ""; } } }}We have saved 28 lines by using the function for just 2 cases - Date of Joining & Resigning. Note: - We haven't added provision for handling invalid day codes i.e values not belonging to the range 1 - 7. The terms function and methods are used interchangeably. Functions are composed of statements that are grouped under a name and can be used repeatedly by just calling the function. Declaration Before we can use a function, we need to define its properties, what it does and the value that it returns. Any function declaration has two parts - Signature and Body. We will use this function as our example to study the two parts. public int SumOfTwoNumbers(int Num1, int Num2){ int Sum = Num1 Num2; return Sum;} Function Signature The function signature is composed of the Return Type, Function Name and the optional Parameters and Access Specifier. Return TypeThe return type determines the type of value that the function will return. It can be any of the atomic data types (int, char, string, bool, float, etc) or the user defined types (enums, objects, structures). A function that does not return any value should have a return type of void. Function NameThe function name can be anything that follows the naming rules:- The name must begin with a letter or an underscore & can be followed by a sequence of letters (A-Z), digits (0-9) or underscore (_) Special Characters such as ? - * / \\ ! @ # $ % ^ ( ) [ ] { } , ; : . cannot be used in the function name. A function name must not be the same as a reserved keyword such as using, public, etc. The name can contain any number of the allowed characters. Functions with the same class cannot have the same name ParametersFunctions take values as input, process it and return the output. These inputs are in the form of parameters. The parameters themselves are composed of the data type and the parameter name. The data type restricts the values that can be passed to the function (int only allows Integer values, bool allows Boolean values and so on) and the parameter name can be used like a regular variable under the function body. This variable would be initialized with the value that is passed to the function when it is invoked (called). A function can have any number of parameters each of which need to separated by a comma. Function Body This is where we define what the function actually does. In our case, the body is composed of the lineint Sum = Num1 Num2; which does the actual calculation and return Sum which returns the value to the calling function. Every function (except the constructor and destructor) which doesn't have a return type of void must return a value. The return value must comply with the data type specified in the signature. Calling/Invoking the function By calling/invoking the function, we imply using it - which results in the lines of code written inside it being executed. Calling a function is very simple. We do so by the following syntax:- FunctionName(param1, param2 ...); In our case, it would go something like - SumOfTwoNumbers(6, 9);. This line will pass the execution to the function SumOfTwoNumbers along with the values 6 and 9 which will get copied in the variables Num1 and Num2. After this, the line int Sum = Num1 Num2; will be executed and the sum of the two numbers will be stored in the variable Sum. Finally, this value will be returned to the calling function, which basically discards this value (as of now). We can store this result as int MySum = SumOfTwoNumbers(6, 9); or display it on screen as follows Console.WriteLine(SumOfTwoNumbers(6, 9));. Special Cases A parameter can have two variations - ref and out. When these prefixes are applied to any parameter, its behaviour changes. Normally, the values passed to the function are just copies of the original value and any changes made to this value have no effect on the original value. By using the ref keyword we can force the value to be passed by reference. This creates an alias of the variable which might have a different name but points to the same address in the memory. Thus, changes made to this value reflect in the original value. The swap function is a trivial example of this difference:- using System;using System.Collections.Generic;using System.Text;namespace ConsoleApplication1{ class Program { static void Main(string[] args) { int A = 10, B = 20; Console.WriteLine("The value of A = {0} and B = {1}", A, B); Swap(A, B); Console.WriteLine("The value (after swapping) of A = {0} and B = {1}", A, B); Console.ReadLine(); } public static void Swap(int A, int B) { int Temp = A; A = B; B = Temp; Console.WriteLine("The value (during swapping) of A = {0} and B = {1}", A, B); } }}The output of the following program would be:- The value of A = 10 and B = 20 The value (during swapping) of A = 20 and B = 10 The value (after swapping) of A = 10 and B = 20 As you can see, the changes made to the value of A and B inside the Swap function have no effect on the values of A and B inside the Main function. This is because both pairs of variable belong to their scopes (the Swap function and the Main Function) and are hence separate. However, by using the ref keyword, we can point the variable A inside the Main and Swap function to the same memory location (same holds for the variable B ). The variable A inside the Swap function will be called an alias of the variable A inside the Main function. The alias doesn't need to have the same name either. The alias stores the memory address of the original variable and not its value. using System;using System.Collections.Generic;using System.Text;namespace ConsoleApplication1{ class Program { static void Main(string[] args) { int A = 10, B = 20; Console.WriteLine("The value of A = {0} and B = {1}", A, B); Swap(ref A, ref B); Console.WriteLine("The value (after swapping) of A = {0} and B = {1}", A, B); Console.ReadLine(); } public static void Swap(ref int A, ref int B) { int Temp = A; A = B; B = Temp; Console.WriteLine("The value (during swapping) of A = {0} and B = {1}", A, B); } }}Output:- The value of A = 10 and B = 20 The value (during swapping) of A = 20 and B = 10 The value (after swapping) of A = 20 and B = 10 By using the ref keyword, we have made the change persistent inside the Main function. Note: It is necessary to specify the ref keyword during function calling. A function can take multiple inputs but only return a single value. There might be situations when we need multiple values as output from the function. We can do this by using either the ref or the out keyword. Both of them result in references being passed. The only difference is that the out keyword passes along null values and is hence ideally suited for output purposes. Note: It is necessary to specify the out keyword during function calling. Previous Tutorial - Lesson 7 - Creating Value Types & Reference Types - Part II Lesson: 1 2 3 4 5 6 7
-
Unofficial Trap17 Hosted Members Directory
turbopowerdmaxsteel replied to turbopowerdmaxsteel's topic in Programming
The script now overrides the Website location based on the one specified in their profiles. This will resolve all domain change issues. All hosted members who have changed there domains are hereby requested to keep this information updated on their profiles.Thanx,Max. -
Unofficial Trap17 Hosted Members Directory
turbopowerdmaxsteel replied to turbopowerdmaxsteel's topic in Programming
Thanx for reporting it. By mentioning the double click you have cleared it all. This would cause the execution of two threads which is precisely what must have caused the problem. Actually, the button is supposed to get disabled after a click. But as of now, I haven't done that. Thanx once again. It is the continued feedbacks by the members that helps the script get better -
Unofficial Trap17 Hosted Members Directory
turbopowerdmaxsteel replied to turbopowerdmaxsteel's topic in Programming
I am working on overriding the members' site based on the one listed in their profile. The script is under constant development. You must have caught it during one of those buggy testing phases. As of now, the progress bar doesn't exceed its limits. But, the operation might get stuck once in a while because of the sheer number of hosting requests at Xisto. I am working on pinpointing the exact cause of the problem. -
Unofficial Trap17 Hosted Members Directory
turbopowerdmaxsteel replied to turbopowerdmaxsteel's topic in Programming
There are quite a few ways.> You could change the description text in your hosting request Topic.> Using the topic corresponding to the domain change request for obtaining the new domain.> Thirdly, a way to allow user's to override their sitename by specifying the correct one in the script.Out of these, only the first one will work at present as the others would require modifications. -
Unofficial Trap17 Hosted Members Directory
turbopowerdmaxsteel replied to turbopowerdmaxsteel's topic in Programming
PHP is used for the actual crawling and parsing while AJAX is used for the interactive interface, multi-threading and a bit of processing. Making the Xisto version was a bit difficult because although similar to asta, the Xisto forum does have significant differences. With this new version that has been coded from the scratch, I have solved the problem where the operation skipped a lot of sites. The entire process is now a lot more efficient. I had to! The process requires the crawling of many a pages in the forum and hence takes a bit of time. -
Unofficial Astahosted Members Directory
turbopowerdmaxsteel replied to turbopowerdmaxsteel's topic in Programming
The script does this by crawling the forum and looking for regular patterns to determine the fields - Member Name & Web Site. -
This is the Xisto edition of the Hosted Members Directory. A script initially written by me for the Xisto forums. The script has gone through many a changes and is currently in version 4 which includes the following features:- > Listing of hosted members. > Listing of the websites of the hosted members. > Validation of the websites (whether the site is suspended, working or not not working, etc) > Save/Load result to/from database. > Multithreaded for faster operation. > Status messages, images and progress bar to keep you informed of the process. Link to the Script: http://forums.xisto.com/no_longer_exists/ Here's a screenshot of the script:-
-
Unofficial Astahosted Members Directory
turbopowerdmaxsteel replied to turbopowerdmaxsteel's topic in Programming
Thanks mate. Not Setup means that the default index page for the site has not been replaced, thus signifying that the site has not been setup. I'll put a legend explaining the status colors and messages once I am done with the Xisto version. -
Unofficial Astahosted Members Directory
turbopowerdmaxsteel replied to turbopowerdmaxsteel's topic in Programming
Info on the latest Version -> 4 of the script:- The new version sports a new look (well, atleast the progress bars and the buttons are new). Additional features include:- > Saving/Loading the list to/from the database > More accurate version of the site verifier. Now shows Suspended, Not Setup, Error or OK as the status. http://forums.xisto.com/no_longer_exists/ -
Please Review - Maxotek
turbopowerdmaxsteel replied to turbopowerdmaxsteel's topic in General Discussion
Well, it was done in like 4 or 5 days. I guess I should thank m^e for throwing me the basics of AJAX. This is my second successful script after the Unofficial Astahosted Members Directory -
Please Review - Maxotek
turbopowerdmaxsteel replied to turbopowerdmaxsteel's topic in General Discussion
Pika Bot did have some problems responding to messages. Thanx for reporting it. It should work now. As I mentioned before, I am emphasizing on the design and aesthetics before porting my existing site located at http://forums.xisto.com/no_longer_exists/ to http://maxotek.net/ . So, only a few pages have been developed. For the last few days, I have been busy developing another one of my scripts that would come in handy - Sitemap Generator. It can be found at http://forums.xisto.com/no_longer_exists/ The following is the list of the features in the current version:- > Spider pages based on a site based on any starting page. > Multi-threaded for faster crawling. > Shares Processing Time between the client and server to maximize speed. > Live statistics to keep you informed. > Comprehensive Crawl Log with HTTP Status to allow debugging of Broken Links. > Allows saving/loading of the sitemap to/from the database. Features Comming Soon:- > XML Sitemap Output > Configurable Crawling > Custom Filtering of Web Pages -
Please Review - Maxotek
turbopowerdmaxsteel replied to turbopowerdmaxsteel's topic in General Discussion
Thanks for the review Jimmy. I changed the color for the labels to good ol' black. -
Its been a while since I got a domain but I haven't managed to get the designs going; thanks to frequent unsatisfactory results. This time, though, I am taking it slow and building it from the scratch. I am focusing on consistency in the interface and logical arrangement of the back-end files. The site is aimed at being the hub for the distribution of my softwares. I am planning to build a customized CMS for my site so that I don't have to rely on Dreamweaver for the regular maintenance work. Right now, the main Dreamweaver template is complete. But I have only wrote the main Index page along with the AJAX back-ends for the Pika Bot widget. Another thing that I worked on, is the image previewer which can be seen by clicking on the images. The design is more-or-less to my satisfaction. But, I wouldn't like to head on creating other pages until I get the expert opinions from you guys. Here goes the link: http://maxotek.net/
-
foreach statement This statement is explicitly used to traverse through arrays. The benefit of using foreach over the normal for statement is that it is not needed to check the size of the array while using the former. Syntax:- foreach(type identifier in expression) { statement 1; statement 2; .... } Suppose we have an array StudentNames containing the name of all the students in a class. We need to display the name of each one of them on screen. First we will see how it can be done using the for loop. string[] StudentNames = new string[] {"Peter", "Tony", "Bruce", "Scott", "Clark", "Kenshin"}; for (int I = 0; I < StudentNames.Length; I++) { Console.WriteLine(StudentNames[I]); } Now we'll use the foreach statement to do this. string[] StudentNames = new string[] {"Peter", "Tony", "Bruce", "Scott", "Clark", "Kenshin"}; foreach (string StudentName in StudentNames) { Console.WriteLine(StudentName); } Param Arrays Remember the Console.WriteLine method which outputs the result using a string format followed by the additional values as input parameters. For example: Console.WriteLine("The sum of {0} and {1} is {2}", Num1, Num2, Num1 + Num2); would display The sum of 5 and 6 is 11 for values 5 and 6 for Num1 and Num2 respectively. What is worth noting here, is that WriteLine() can take any number of parameters after the string format. So, something like this would work as well:- Console.WriteLine("The Prime Numbers -> {0}, {1}, {2}, {3}, {4}, {5}, {6}, {7}, {8}", 2, 3, 5, 7, 11, 13, 17, 19, 23); This is done by the param array data type. Example:- public static int Sum(params int[] Numbers) { int Sum = 0; foreach (int Number in Numbers) { Sum += Number; } return Sum; } This is a function to return the sum of any number of numbers. The numbers to be summed are passed as parameters during the function call as: Sum(4, 69, 13, 99); Note: param array should be the last parameter in the function signature which also means that only one param array can be used in a function. Why is this done? Consider the following function signature: public static int MyFunction(params int[] A, int C). How would we call the function if this were to be allowed? MyFunction(1, 2, 3, 4, 5); How can the compiler determine where the values for the param array A finish? Actually, if a reverse scanning of parameters were to be done, this could be determined. But that would increase useless complexity of having to implement this along with the normal forward scanning of parameters. Multi Dimensional Arrays Up until now, we have only worked with Single Dimensional arrays. Now we shall see how the Multi Dimensional arrays are declared, initialized and manipulated. The pictures below show how the Single and Double Dimensional arrays are represented. While, the Single Dimensional arrays are represented as a single row of elements, Double Dimensional arrays are represented by multiple rows. Similarly, arrays with 3 dimensions would be represented in 3D space along the three axes. Declaration Declaration is done by the syntax:- datatype [ , ] VariableName; The number of commas ( , ) inside the square brackets [ ] should be one less than the number of dimensions required for the array. Example Usage:- int [ , ] Numbers; Initialization Initialization is done in a way where each row is treated like a single dimensional array. Example:- int[,] Numbers = new int[3, 3] { {1, 2, 3}, {4, 5, 6}, {7, 8, 9} }; Assigning Values Values can be assigned as:- Numbers[1, 2] = 7; Array Class The Array class defined in the System namespace serves as the base class for all the arrays in the Common Language Runtime. It can be used to create, manipulate, search and sort arrays. Some of the common Properties and methods are summarized below. Properties IsFixedSize - Returns a boolean value (True / False) indicating whether the array is of Fixed Size or not. IsReadOnly - Returns a boolean value (True / False) indicating whether the array is Read Only or not. Length - Returns a 32-bit integer that represents the total number of elements across all dimensions in the array. Rank - Returns the number of dimensions (also called the Rank) of the array. Methods BinarySearch - Performs the faster Binary Search algorithm on the array and returns the number if found. Clear - Resets the value of the supplied range of elements in the array to the default values of the datatype:- int - 0 bool - false Reference Data Types - Null Copy - Copies a range of elements to another array along with type casting and boxing as required CopTo - Copies all elements to the specified single dimensional array Find - Searches for the given value and returns its first occurrence in the array FindAll - Returns all occurrences of the searched element in the array GetLength - Returns the 32 bit length of the array GetValue - Returns the value of the specified item in the array IndexOf - Searches for the given value and returns the index of the first position its found in Resize - Resizes the array Reverse - Reverses the order of the elements. (Works only on a single dimensional array) SetValue - Sets the value at the specified location of the array Sort - Sorts the elements. (Works only on a single dimensional array) Collections An integer array can only store integers. Same goes for any other array type - string, boolean, etc. Collections overcome this limitation of arrays and can store elements of different types as items. This is possible because Collections actually store references and not values. We use the System.Collections namespace to work with collections. Boxing - No, not the Sport! Boxing is the automatic conversion of value types to reference types allowing them to be stored in collections. Unboxing - The reverse of the former, conversion of reference type to value type. Because Collections store references, it is necessary to do the above conversions while storing and retrieving values in Collections. List of classes under the System.Collection namespace. Array List - Array List is a better alternative to arrays because of the following advantages it offers over arrays. Resizing - Arrays cannot be resized natively. So, one has to create a new array and copy the elements of the existing one followed by rearrangement of references for this process. Array List on the other hand work on the concept of Linked List where memory is allocated and deallocated at runtime as and when required. Adding an element - To add an element we need to first create a new array, copy the values before the position where the new element is to be inserted, insert the new element and then insert the remaining elements. Again, a cumbersome process for arrays. Array Lists only need to allocate memory for the new element and resolve the references of the elements on both side of the new element. Deleting an element - All elements after the element to be deleted need to be shifted forward to fill up the vacancy created by the deletion. Array Lists rely on releasing the memory occupied by the element and resolving the references of the elements on both sides. Common Methods used by the Array List class:- Add - Adds an item at the end of the Array List Clear - Removes all the items from the Array List Insert - Inserts an element into the Array List at the specified position Reverse - Reverses the order of all the elements or a portion of the Array List Remove - Deletes the item at its first occurrence TrimToSize - Sets the maximum capacity to the number of elements currently in the the Array List Other Classes that the collection namespace provides are:- Queue - A Collection that works on the First-In-First-Out (FIFO) rule. Here, insertion of elements occur at the rear and deletion at the front. Stack - Works on the Last-In-First-Out (LIFO) rule. Both insertion and deletion occur at the top of the stack. Hash Table - It uses unique keys to access/store elements in the collection. Previous Tutorial - Lesson 6 - Creating Value Types & Reference Types - Part I Lesson: 1 2 3 4 5 6 7
-
I don't think it is meant to upgrade older dreamweaver versions. It certainly didn't upgrade my Dreamweaver 8 or even offer such a proposal. While the sheer number of files you stated is huge, I don't think even that can account to 3-4 hours of installation on today's low-mid range machines. @Niru: You might as well try to uninstall + install the software once again, so as to find out if that was a one time experience. Only this time ensure a clean enviorment.