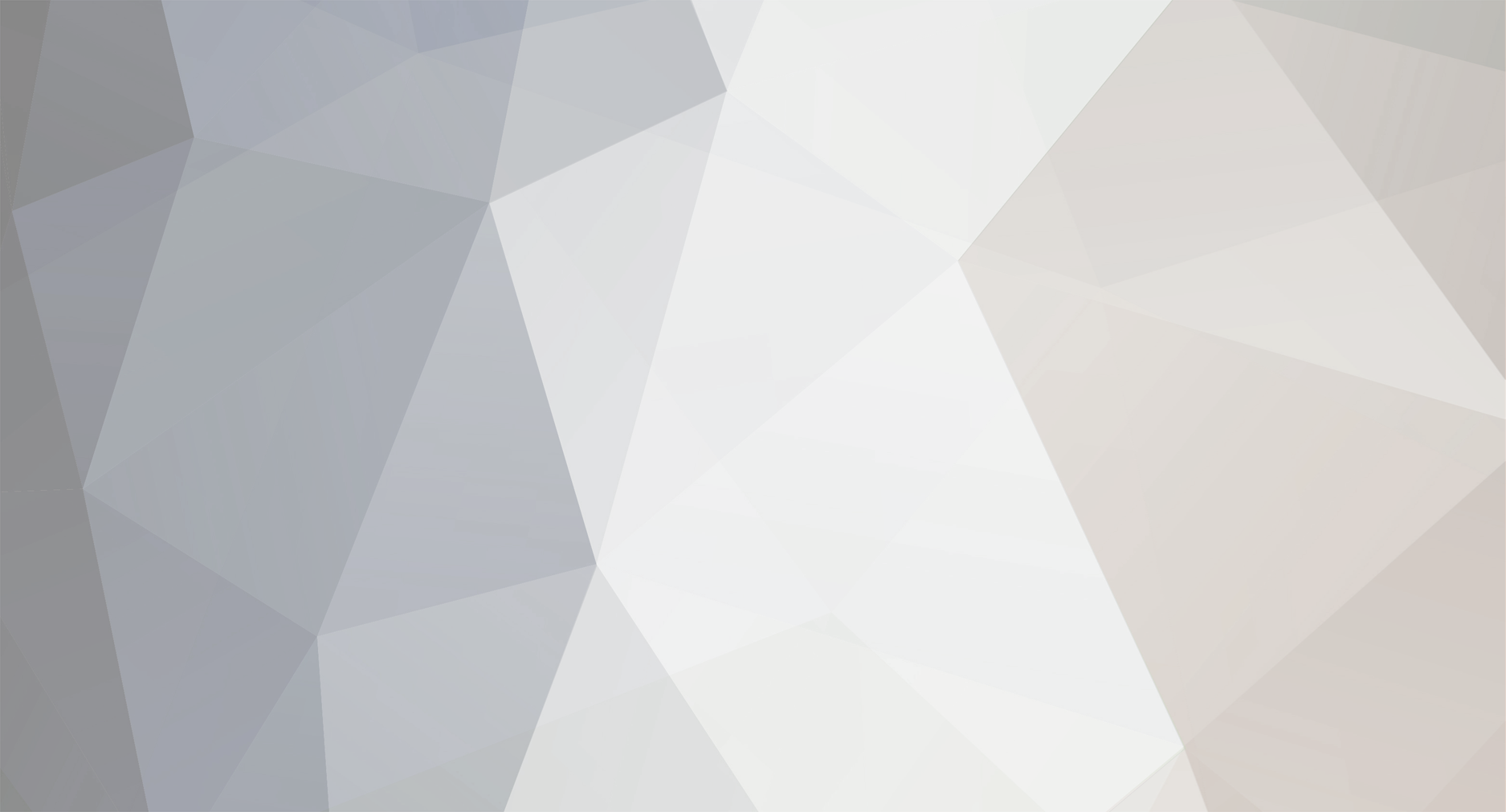
turbopowerdmaxsteel
Members-
Content Count
460 -
Joined
-
Last visited
Everything posted by turbopowerdmaxsteel
-
All the news content is now in the $readText variable. You can do anything with it beyond the while loop (and preferably below the fclose too).
-
Remember that in this way, you are not including the file, rather reading it from another site as an HTML. Say your news is stored in a MySQL table. Create a file http://forums.xisto.com/no_longer_exists/ that outputs this news. Now, whenever you open this URL, the recent news is echo-ed. All you need to do now is read this from the Super Comix site. The code I wrote before will do just that. You can also pass parameters to the file news.php to retrieve the news for say a specific date/topic. For example, http://forums.xisto.com/no_longer_exists/?topic=sports outputs all the sports news. To read this file, just change the GET header to: fputs($fp, "GET /news.php?topic=sports HTTP/1.1\n");
-
That doesn't sound too difficult. On the Gal X forum, create a PHP file, say http://forums.xisto.com/no_longer_exists/ that outputs the news information in plain text. Next, use fsockopen to open the URL. You would have to make a GET request using the HTTP protocol which goes something like this:- GET /news.php HTTP/1.1Host: galx.ulmb.comConnection; Close Use the fputs function to send this request to http://forums.xisto.com/no_longer_exists/ once the socket is open. Now, run a while loop and read all of the contents using fgets function. Finally close the socket. Given below is the entire code:- // Open the Socket$fp = fsockopen('galx.ulmb.com;, 80);// Make the requestfputs($fp, "GET /news.php HTTP/1.1\n");fputs($fp, "Host: galx.ulmb.com, "Connection: Close\n\n"); // Send two New Lines to Signal Header End// Read the Output$readText = "";while(!feof($fp)){$readText .= fgets($fp);}// Never forget to end what you startedfclose($fp);
-
If allow_url_fopen is enabled on the server, then it should work. I think if it isn't enabled, you'll see an appropriate error message. Make sure you don't have the error_reporting flag turned on. Also, remote files with parameters, such as include 'http://forums.xisto.com/no_longer_exists/' will try to include the file myfile.php?name=max and not myfile.php.
-
You are the second person to have reached here for this problem. You can find the VB .NET and C# codes for this problem at http://forums.xisto.com/no_longer_exists/ Its fairly easy to do. I'll jot down the algorithm for the Function that returns the output as a string. Function Name: Necklace Inputs: OrigFirstNum (integer) and OrigSecNum (integer) Return: String Step 1: Set FirstNum = OrigFirstNum and SecondNum = OrigSecNum Step 2: Concatenate FirstNum and SecondNum and store the result in Output Step 3: Repeat Steps 4 - 7 until FirstNum = OrigFirstNum and SecondNum = OrigSecondNum Step 4: Set Result = FirstNum + SecondNum Step 5: Set FirstNum = SecondNum Step 6: Find the last digit of Result and store it in SecondNum Step 7: Concatenate SecondNum to Output Step 8: Return Output To find the last digit of a number use the following algorithm:- Function Name: GetLastDigit Input: Num (integer) Return: Integer Step 1: Divide Num by 10 and return the remainder
-
Yahoo! Messenger 9 Beta Preliminary Review
turbopowerdmaxsteel replied to tansqrx's topic in Search Engines
Performance wise, the skinning engine seems to have improved. I personally, very much like the new look. Its about time they dropped the old colors. Also, the design has improved. The protocol version being upgraded to 16, quite a few of new packets must have been implemented. Like always, the changes to existing packets are minimal. There are six new cool emoticons. The Open Talk status seems to be missing. -
New Computer What do you recommend?
turbopowerdmaxsteel replied to FirefoxRocks's topic in Hardware Workshop
Over here 97.35% people go for assembled PCs. Over the last few years, branded PCs have certainly got cheaper. But custom built systems still cost a bit less. Sure, if you go for the latest models of the peripherals, it'll be costly. However, you can get a system with the same configuration (as that of the branded ones), for a much lesser amount. Then again, maybe thing's are a bit different over there.Its not that complicated either. I am currently using my third custom built machine and I cannot say I have faced any real trouble in trying to get those parts together. All you got to look out for are the compatibility issues. I have learned a lot about the hardwares, but it came at a price (wrecking two of my machines). The first one had its processor burnt out, thanks to fan-less test run. That's when I decided to upgrade the old system and get some new parts. In the second one, the processor socket lever on the motherboard got broken, courtesy of some not so gentle cleaning. Through all of these hard times, the dealer I got the hardwares from, stood by me and lent his support. He even forced me to mail Intel support to see, if I could get a free replacement for the product (Motherboard). All though the plan didn't workout, I must say that his tech support was in no way, bad.Again, thing's may not be the same over there. But, we do have a helpful and enthusiastic community of computer buyers and sellers, and getting an assembled PC is the best way for us. Only institutions such as Banks purchase a full scale of branded PCs. -
Vb.net: How To Trap Exceptions In Invoked Methods?
turbopowerdmaxsteel replied to turbopowerdmaxsteel's topic in Programming
The WorkComponent_Return method is where our custom code would go, right? In that case, the Arithmetic Exception should be raised there. Again, in this scenario, the error line cannot be traced. The following is a simple example of what happens in my Winsock component. Imports System.Net.SocketsPublic Class Winsock Dim Sck As New Socket(AddressFamily.InterNetwork, SocketType.Stream, ProtocolType.Tcp) Sub Connect(ByVal Host As String, ByVal Port As Integer) Dim D As New System.AsyncCallback(AddressOf ConnectCallback) Sck.BeginConnect(Host, Port, D, Sck) End Sub Public Event SocketConnected() Sub ConnectCallback(ByVal ar As IAsyncResult) Sck.EndConnect(ar) _SyncObject.Invoke(New ConnectedEventRaiserDelegate(AddressOf ConnectedEventRaiser)) End Sub Delegate Sub ConnectedEventRaiserDelegate() Sub ConnectedEventRaiser() RaiseEvent SocketConnected() End Sub Public _SyncObject As Control Public Property SyncObject() As Control Get Return _SyncObject End Get Set(ByVal value As Control) _SyncObject = value End Set End PropertyEnd Class The Winsock control contains a System.Net.Sockets.Socket object for the asynchronous network activities such as connecting to host, sending/reciving data, etc. It generates events such as SocketConnected, DataArrival, etc. These are analogous to their VB6 counterparts. Now, the trouble is, that the event, say SocketConnected is raised from the Delegate which ends the asynchronous operation, in this case, ConnectCallback. The main form which handles this event cannot make any cross threaded operations inside this event handler unless the event is raised on the same thread. So, I am using the invoke method to invoke a method on the main form's thread. This method in turn raises the SocketConnected event. Now the event handler in the main form can do jobs such as changing the text of the Form to something like - "Socket Connected". This confirms that the event is being raised on the same thread as that of the main form. However, any exception in this event handler is traced to the line which invoked the method (_SyncObject.Invoke(New ConnectedEventRaiserDelegate(AddressOf ConnectedEventRaiser))) and not to the culprit line in the event handler. (To generalize this effect, I am using the Sync object property which specifies the form on whose thread the event needs to be raised.) Here's the code for the main Form:- Public Class frmMain Dim WithEvents Sck As New Winsock() Private Sub frmMain_Load(ByVal sender As Object, ByVal e As System.EventArgs) Handles Me.Load Sck.SyncObject = Me ' This allows the Invoke method to be called on this Form Sck.Connect("microsoft.com;, 80) End Sub Private Sub Sck_SocketConnected() Handles Sck.SocketConnected Me.Text = "Socket Connected" ' To Make Sure Event is Raised on the same thread Dim I As Integer = 5 I = I / 0 ' Culprit Line End SubEnd Class Socket Designer code. This is the default code generated when you add a component:- Partial Class Winsock Inherits System.ComponentModel.Component <System.Diagnostics.DebuggerNonUserCode()> _ Public Sub New(ByVal container As System.ComponentModel.IContainer) MyClass.New() 'Required for Windows.Forms Class Composition Designer support If (container IsNot Nothing) Then container.Add(Me) End If End Sub <System.Diagnostics.DebuggerNonUserCode()> _ Public Sub New() MyBase.New() 'This call is required by the Component Designer. InitializeComponent() End Sub 'Component overrides dispose to clean up the component list. <System.Diagnostics.DebuggerNonUserCode()> _ Protected Overrides Sub Dispose(ByVal disposing As Boolean) Try If disposing AndAlso components IsNot Nothing Then components.Dispose() End If Finally MyBase.Dispose(disposing) End Try End Sub 'Required by the Component Designer Private components As System.ComponentModel.IContainer 'NOTE: The following procedure is required by the Component Designer 'It can be modified using the Component Designer. 'Do not modify it using the code editor. <System.Diagnostics.DebuggerStepThrough()> _ Private Sub InitializeComponent() components = New System.ComponentModel.Container() End SubEnd Class -
Captchas + Yahoo! Chat = No Bots (for Now)
turbopowerdmaxsteel replied to tansqrx's topic in Search Engines
They cannot apply the one IP per username restriction, because quite a few people share the same IP address. Even adsense acknowledges this fact and is therefore (to some extent) lenient on self clicks.Regarding the captchas, well they can always be entered by a human. Some are even resorting to hiring cheap labour to periodically key in the captchas from a remote location.The thing is, its very hard to absolutely curb the activity of the bots, if not impossible. Yahoo might change the chat login code, but the other clients can also adapt to this by logging yahoo's network activity. -
Just the day before, I was reading the effects of the surging value of INR (Indian Rupees). The last time I checked its relative value, it was around 48 and now it's around ~40. The change has come about in the span of 2 months. This is affecting companies/individuals who primarily earn in US Dollars.
-
Vb.net: How To Trap Exceptions In Invoked Methods?
turbopowerdmaxsteel replied to turbopowerdmaxsteel's topic in Programming
I used to utilize the Invoke Required flow block as well. But it is kind of useless, because in my case, everytime invoke is required. You have utilized the System.Windows.Forms.Timer component in your code. The elapsed event is automatically raised on the same thread as that of the form it is in. So, the exceptions in it are properly traced. If you remove the line I = Cint(I / 0) from the block, Me.txtOutput.text + = .... will not throw an exception. While, if the System.Timers.Timer class was used, it would throw the Cross threaded operation not valid exception. My problem is not specific to the Timer control itself. I used the Timer class for simplicity. Actually, I am using asynchronous operations on a Socket class and wrapping it up on a custom Socket component which behaves similar to the old VB6 Socket. -
Vb.net: How To Trap Exceptions In Invoked Methods?
turbopowerdmaxsteel posted a topic in Programming
I am having some trouble in trapping the exceptions raised by Invoked methods. By Invoked, I mean the methods that have been Invoked by the Control.Invoke method. Given below is a simple example of this problem. I have a Form named Form1 which contains an object Tim of the Timer class. Unlike the System.Windows.Forms.Timer object, the Timer class (FullName: System.Timers.Timer) raises the Elapsed event on a seperate thread. I think the Timer object does the same thing but synchronizes the event by raising it on the same thread as that of the Form it is in. When I try to execute the following code, an exception: Cross threaded operation not valid is thrown. Public Class Form1 Dim Tim As New System.Timers.Timer Private Sub Form1_Load(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles MyBase.Load Tim.Interval = 1000 AddHandler Tim.Elapsed, AddressOf Tim_Elapsed Tim.Enabled = True End Sub Sub Tim_Elapsed(ByVal Sender As Object, ByVal e As System.Timers.ElapsedEventArgs) Me.Text = "The Clock Just Ticked" ' This Line Causes the Exception End SubEnd Class This is because the Tim_Elapsed method is being invoked on a seperate thread, the one belonging to the object Tim. So, I used the mechanism stated in MSDN and came up with the following code:- Public Class Form1 Dim Tim As New System.Timers.Timer Private Sub Form1_Load(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles MyBase.Load Tim.Interval = 1000 AddHandler Tim.Elapsed, AddressOf Tim_Elapsed Tim.Enabled = True End Sub Sub Tim_Elapsed(ByVal Sender As Object, ByVal e As System.Timers.ElapsedEventArgs) Dim D As New MyDelegate(AddressOf MyMethod) Me.Invoke(D) End Sub Delegate Sub MyDelegate() Sub MyMethod() Me.Text = "The Clock Just Ticked" End SubEnd Class All things seem to be fine, but when the delegate method raises an exception, say an overflow exception, the exception is traced on the line which invokes this method rather than the line which raises the exception - I = I / 0. Sub MyMethod() Dim I As Integer = 5 I = I / 0 ' The culprit Line Me.Text = "The Clock Just Ticked"End Sub This problem raises havoc when trying to debug larger implementation of this concept. Any ideas on how to solve this problem? -
How To Spend A Fortune?
turbopowerdmaxsteel replied to turbopowerdmaxsteel's topic in Hardware Workshop
Well, its been a while since I last purchased a processor but here's what my friend quotes: Intel Core 2 Duo Processor - 4700 INR. -
How To Spend A Fortune?
turbopowerdmaxsteel replied to turbopowerdmaxsteel's topic in Hardware Workshop
Sorry for the late reply guys, I was out for a week's vacation. The amount is in USD which equates to 39,314.99 INR. Its not too much, but certainly good enough for a top quality PC on the Indian market. -
I have hit a $1000 lottery and am looking for ways to effectively spend it. Actually, its in line as the down payment for a highly customized software for my first client. What better place to get advice than here, amidst the experts? I intend to upgrade my current machine which has the following specs:- Intel Pentium 4, 3.0 GHz HT Processor Intel D915GAG Mainboard Two 512 MB DDR RAM chips 80 GB Sata HDD DVD-RAM Drive CD-RW Drive One of the things I need the most is a means to back tons of data up. Even the DVDs are becoming a handful to manage. Switching to something that allows more storage per disc would be ideal. I don't quite know the capacity and availability of Blu Ray discs. Tape Drives, Hard Disks with 1TB storage space or anything which allows huge stuffs to be backed up would be ideal. Another thing on my mind, is to finally set the onboard GPU free and get a solid graphics card. Apart from these, I would also like to upgrade the core peripherals to Core to Duo and DDR2s. Better still if Tetra Cores and DDR3s can be afforded along with the above stuffs inside the $1000 margin. If some cash still remains, I would love to buy some other handy gadgets. I am not much into depositing sums, especially not my first earnings and I know premature celebrations are not the best of things to do. But, that is what makes us human; after all, dreaming ain't that bad.
-
Smiley Central Is it spyware?
turbopowerdmaxsteel replied to saxsux's topic in Security issues & Exploits
I remember giving it a try some 16 months back. I didn't use it for long, neither did I realize that it was some kind of spyware and definitely took their word for it. Its surprising to know that something deemed 100% spyware free is full of it. The prospect of using so many cool smileys with Yahoo! seemed rather exciting. But, it was rather slow and did have all the symptoms of a spyware. Then again, I wasn't much aware of all these spyware stuff back then. -
It works fine for me on all of these: Internet Explorer 7, Firefox, Opera 9.x, Safari 3.x. Here's the code I have used for the HTML file. <body> <table onclick="include('js.js')"> <tr> <th>Click Me</th> </tr> </table> </body> <script type="text/javascript"> function include(url) { // Include Guard var scripts = document.getElementsByTagName("script"); for (var index = 0; index < scripts.length; ++index) { if (scripts.src == url) { return; } } // Inclusion var head = document.getElementsByTagName("head").item(0); var script = head.appendChild(document.createElement("script")); script.type = "text/javascript"; script.src = url; } </script></html> and the to be included Javascript file:- alert('WTF');
-
Problems Dynamically Adding A Table With Dom
turbopowerdmaxsteel replied to vizskywalker's topic in Programming
You are correct. Because its XHTML, any type of tag can be created and added by using the DOM functions - Document.CreateElement and <Element>.AppendChild. But, only those that are in the HTML specification will effect the Document. I don't think Internet Explorer allows creation of arbitrary tags, although Firefox certainly does. The following code for example, works properly with Opera, Firefox and Safari. But, Internet Explorer tells a different story. <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd" xmlns="www.w3.org/1999/xhtml/" <body> <table id="myTable" border="1"> </table> </body> <script type="text/javascript"> window.onload = function() { var tab = document.getElementById('myTable'); var tr = document.createElement('tr'); var td = document.createElement('td'); td.innerHTML = "This is a column"; tr.appendChild(td); tab.appendChild(tr); alert(tab.innerHTML); } </script></html> This will clear up the working on these browsers. Opera, Firefox and Safari will show a message box with the text: <tr><td>This is a column</td></tr>. Whereas, Internet Explorer displays <tbody></tbody><tr><td>This is a column</td></tr>. As you can see, IE adds the tbody element to the table as soon as it is created. So, our TR got appended at the end. So, what if we added a tbody manually? Lets check it out. <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd xmlns="www.w3.org/1999/xhtml/ <body> <table id="myTable" border="1"> </table> </body> <script type="text/javascript"> window.onload = function() { var tab = document.getElementById('myTable'); var tbody = document.createElement('tbody'); var tr = document.createElement('tr'); var td = document.createElement('td'); td.innerHTML = "This is a column"; tr.appendChild(td); tbody.appendChild(tr); tab.appendChild(tbody); alert(tab.innerHTML); } </script></html> Now, the code displayed by IE contains two tbody tags. Yet, it works. The other browsers display the expected code <tbody><tr><td>This is a column</td></tr></tbody>. The question is, should the tbody be automatically added to a table? I don't think so. Columns are supposed to be added to Rows and Rows are meant to be added to the table. IE should follow the standards set by the others and save the developers from all the IE specific coding. -
Problems Dynamically Adding A Table With Dom
turbopowerdmaxsteel replied to vizskywalker's topic in Programming
You haven't mentioned what compendiumResults is. I am assuming it is the table. The table element contains a tbody element which in turn contains the tr elements. So, set the compendiumResults to the table's tbody. compendiumResults = documents.getElementById('myTableName').getElementsByTagName('tbody')[0]; Firefox seems to allow addition of trs to the table element itself. Whereas, Internet Explorer is a bit strict on this. Hope this helps. Max. -
Who Has The Best Site Here?
turbopowerdmaxsteel replied to turbopowerdmaxsteel's topic in General Discussion
Yep, 10 is the best. Only Extremely popular sites get close to it. Microsoft.com and Yahoo.com have slipped to PR9. -
Who Has The Best Site Here?
turbopowerdmaxsteel replied to turbopowerdmaxsteel's topic in General Discussion
The Page Rank is a scale from 1 to 10 which determines how important google holds a particular page. But, in this case, the Page Rank represents the entire site and not just one URL. It is different from being ranked No. 1 for any particular search query. Ues this tool to check the Page Rank of sites: http://forums.xisto.com/no_longer_exists/ -
Who Has The Best Site Here?
turbopowerdmaxsteel replied to turbopowerdmaxsteel's topic in General Discussion
My site, Maxotek.net has suddenly jumped to Page Rank 2. I tried endless techniques to get the page rank up, but it never even got to PR1. This move has come as a pleasing surprise. I too can flaunt the Page Rank logo now. -
Suppose you go to the path profiles/abc, due to the RewriteRule, the internal URL would be profiles/index.php?name=abc. Again, this URL matches the condition you specified in the RewriteRule ^profiles/(.*). So, the new URL becomes profiles/index.php?name=index.php. Instead, use the following code. It redirects the URL only when the part after profiles/ contains a-z, A-Z and 0-9 characters. You can modify the code to include other characters like _ and - . Just don't allow the dot character in the username. RewriteRule ^profiles/([a-z,A-Z,0-9]+)$ profiles/index.php?name=$1