Sign in to follow this
Followers
0
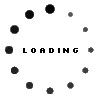
How To Put An Active Shoutbox On Your Website Using Ajax And Mysql
By
Rigaudon, in General Discussion
By
Rigaudon, in General Discussion
Terms of Use | Privacy Policy | Guidelines | We have placed cookies on your device to help make this website better. You can adjust your cookie settings, otherwise we'll assume you're okay to continue.