Sign in to follow this
Followers
0
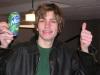
Php Form Data And Conditional Statements My third tutorial
By
ghostrider, in General Discussion
By
ghostrider, in General Discussion
Terms of Use | Privacy Policy | Guidelines | We have placed cookies on your device to help make this website better. You can adjust your cookie settings, otherwise we'll assume you're okay to continue.