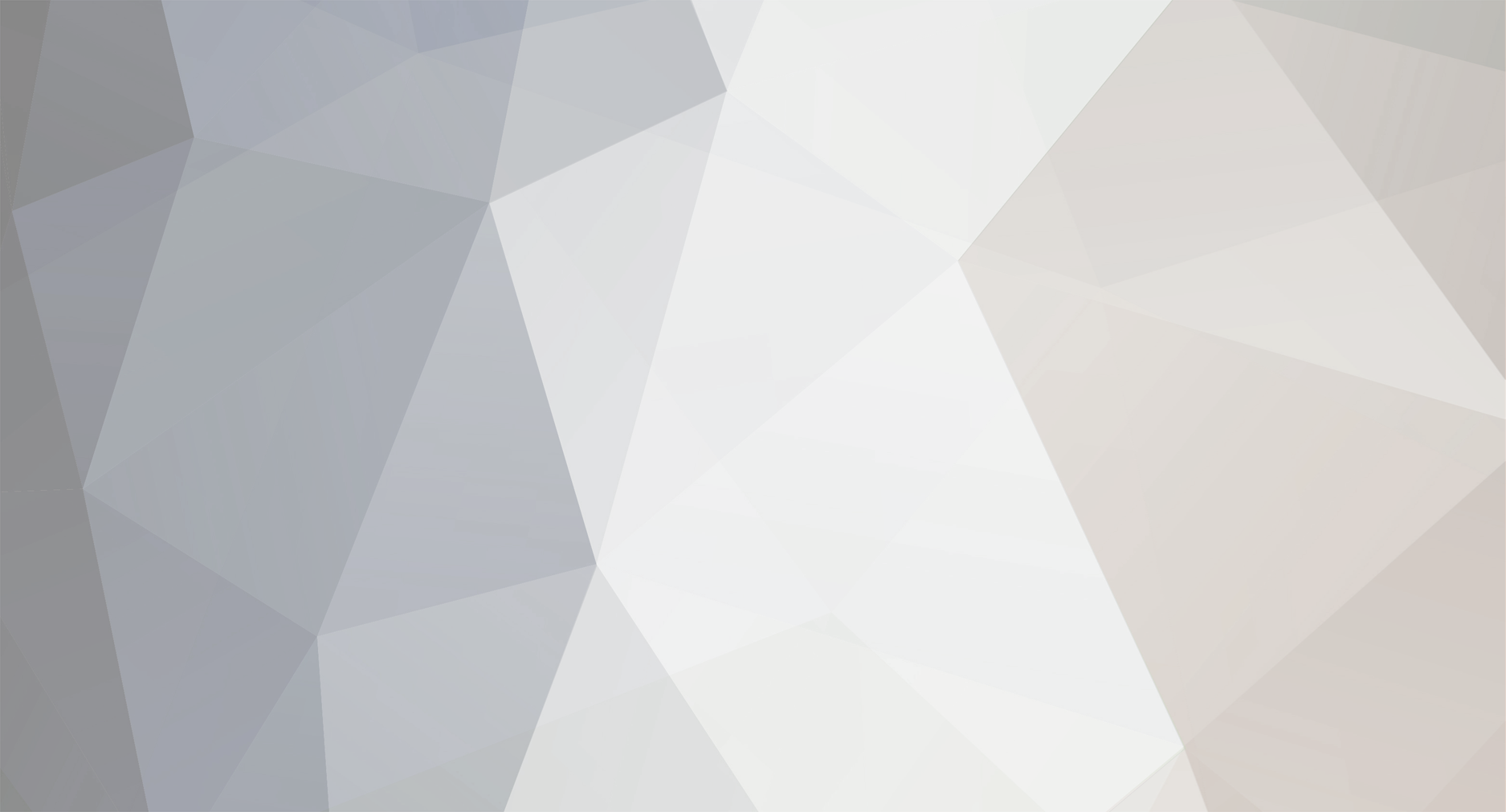
de4thpr00f
Members-
Content Count
77 -
Joined
-
Last visited
About de4thpr00f
-
Rank
Member [Level 2]
-
My account was suspended few minutes ago, i have 30 days left.My login is newengWhat happened?If i can't get my acc back i'm asking you to let me get all my data before closing it.I have a lot of important stuff in there.
-
The HTML <script> tag is used to insert a JavaScript into an HTML page. Examples Write text with Javascript - http://www.w3schools.com/js/tryit.asp?filename=tryjs_text The example demonstrates how to use JavaScript to write text on a web page. Write HTML with Javascript - http://www.w3schools.com/js/tryit.asp?fileryjs_formattext The example demonstrates how to use JavaScript to write HTML tags on a web page. How to Put a JavaScript Into an HTML Page <html><body>[color=red]<script type="text/javascript">document.write("Hello World!");</script>[/color]</body></html> The code above will produce this output on an HTML page: Hello World! Example Explained To insert a JavaScript into an HTML page, we use the <script> tag. Inside the <script> tag we use the "type=" attribute to define the scripting language. So, the <script type="text/javascript"> and </script> tells where the JavaScript starts and ends: <html><body>[color=red]<script type="text/javascript">...</script>[/color]</body></html> The part document.write is a standard JavaScript command for writing output to a page. By entering the document.write command between the <script> and </script> tags, the browser will recognize it as a JavaScript command and execute the code line. In this case the browser will write Hello World! to the page: <html><body>[color=red]<script type="text/javascript">document.write("Hello World!");</script>[/color]</body></html> Note: If we had not entered the <script> tag, the browser would have treated the document.write("Hello World!") command as pure text, and just write the entire line on the page. HTML Comments to Handle Simple Browsers Browsers that do not support JavaScript will display JavaScript as page content. To prevent them from doing this, and as a part of the JavaScript standard, the HTML comment tag can be used to "hide" the JavaScript. Just add an HTML comment tag <!-- before the first JavaScript statement, and a --> (end of comment) after the last JavaScript statement. <html><body>[color=red]<script type="text/javascript"><!--document.write("Hello World!");//--></script>[/color]</body></html> The two forward slashes at the end of comment line (//) is the JavaScript comment symbol. This prevents JavaScript from executing the --> tag.
-
Saint_Michael started following de4thpr00f
-
On this tutorial we will see how to draw a circle on the screen and move it around using the arrows of the keyboard. (Don't worry if you don't get anything, the code will be analysed line by line) #!/usr/bin/env python import pygamefrom pygame.locals import * if not pygame.font: print 'Atention, there are no fonts.' if not pygame.mixer: print 'Atention, there is no sound.' pygame.init() red = (255, 0, 0)black = (0, 0, 0) window_width = 640window_height = 480 window = pygame.display.set_mode((window_width, window_height)) ray_circle = 10 xpos = 50ypos = 50 circle = pygame.draw.circle(window, red, (xpos, ypos), ray_circle) movement_x = 5movement_y = 5 pygame.display.flip() pygame.key.set_repeat(1000, 100) while True: for event in pygame.event.get(): pass key_pressed = pygame.key.get_pressed() if key_pressed[K_LEFT]: xpos -= movement_x if key_pressed[K_RIGHT]: xpos += movement_x if key_pressed[K_UP]: ypos -= movement_y if key_pressed[K_DOWN]: ypos += movement_y window.fill(black) circle = pygame.draw.circle(window, red, (xpos, ypos), ray_circle) pygame.display.flip() Ok, let's start explaining line by line. #!/usr/bin/env python This line is only for people who have Linux, and it shows where to find the executable of Python. There are two versions of this line, "#!/usr/bin/env python" and "#!/usr/bin/python". This line need to be the first of the file (in this case, the whitespace is revelant). There are some diferences between both, but they are irrevelant on day by day job, and they doesn't fit this tutorial. import pygamefrom pygame.locals import * The Pygame is a module for Python (i assume you already have this installed, otherwise you should find on the repositories of your Linux distro, or move into the oficial website at http://www.pygame.org/download.shtml where you'll find the downloads for Windows and Mac, or the sources if you want to compile your own). Being Pygame a module, you need to import it, using the command "import pygame" The pygame.locals has things like keyboard key codes, and to be faster accessing them, you import the module directaly. if not pygame.font: print 'Atention, there are no fonts.' if not pygame.mixer: print 'Atention, there is no sound.' This two blocs are optional, but it's always handy. In case that the program can't load the fonts of pygame, or can't access the sound, this prints an error on the console. pygame.init() This command starts all the modules that are imported when we use "import game". If you want, it's possible to start modules one by one, but normally, that is not necessary. If you are using one interpretre like IDLE, you should receive a tuple, that shows the modules that have been started with success, and those who are not. (At normal conditions this command should return (6,0), 6 modules started correctaly, 0 not started) red = (255, 0, 0)black = (0, 0, 0) I think when your doing a tutorial, there should not be numbers out of nowhere, so i started the variables red and black containing the RGB color to that color. (RGB means Red, Green, Blue, the max value for R/G/B is 255, so definig a color is easy, take a look at my red, 255 of red, 0 of green and 0 of blue, so it's red definetly, my black 0 red, 0 green, 0 blue, no color = black ) The red will be the color of the circle, and the black will be the color to clean the screen after each frame (this will be explained right away). window_width = 640window_height = 480 AS i told before, numbers shouldn't appear out of nowhere, so i created a variable with the numbers needed for the width and height of the screen, 640x480 will be the size of the window. If you want to change the size of the window, just change those values ;D window = pygame.display.set_mode((window_width, window_height))This line creates a window, with the size of 640x480 (because of the variables we created before) Ok, those brackets are really needed, the info should come in a tuple, window = pygame.display.set_mode(window_width, window_height) wouldn't work. ray_circle = 10 xpos = 50ypos = 50 The variable ray_circle will define the ray of the circle in pixels. The variables xpos and ypos will define the position where the circle will be shown on the first frame. circle = pygame.draw.circle(window, red, (xpos, ypos), ray_circle)Now we will create the circle, using the module draw of pygame. With this we can create the images without using the sprites created on other programs (obviously the draw only draws basic geometric figures). The draw can also create rectangles. To see everything that it can create, go to the oficial documentation of the module that can be found here. The pygame.draw.circle needs the next arguments: name of the window variable where it will draw the circle, color of the circle, a tuple with the center position of circle (x, y), and the ray of the circle. ok, so this circle will be created on our window, with red color, at the position (50,50) (because we defined those variables before), and a ray of 10. movement_x = 5movement_y = 5 This will define the number of pixels that the circle will move on the "x" axle and "y" axle. pygame.display.flip() This command will refresh the window, so if any movement happens, this command "sticks" everything on the window to be seen. (There is another way to do this but it's more difficult ;D ) pygame.key.set_repeat(1000, 100)At normal conditions, Pygame doesn't "accept" a key pressed as a loop. So, if you press the left key and "glue" the finger on it, it will only move the circle to left 1 time. To "fight" this we use the "set_repeat". The first value is the time that we have to "un-glue" the finger untill the Pygame accepts the first loop. The second value, is the interval that exists between each loop. Every values will be given in milliseconds (1000 milliseconds = 1 second). In this example, when you press left key, and "glue" the finger, the circle will move 5 pixels to the left, after 1second it will move 5 pixels to left every 100 milissenconds (50 pixels per second). This is a little difficult to explain in a foreign language, the best way is to change the values and see how the program will react. while True: Every game has to have a main loop, to process the input during frames. for event in pygame.event.get(): pass The module "event" of Pygame is the one that gets all the events that occur. The function get() will return a list with everything that passes determined moment (to try this, try to change "pass" with "print event"). This list ocupies a lot of memory space if runned for a long time, the best way is to clean it up with "pass". key_pressed = pygame.key.get_pressed()This variable will store the keys that are pressed, so after it can compares with the "if"'s that we have later in the code, and if the key pressed is found on the "if" it will execute the code. if key_pressed[K_LEFT]: xpos -= movimento_em_xIn the case that the pressed key is the left arrow, we change the position of the circle. The "grid" will be something like this: y^ | | | ---------> |0 x | | To move the circle to the left, we need to subtract the x value, to move it to right, we need to add the x value, to move it to top, we need to add the y value, to move it down, we need to subtract the value of y. That is what the next blocs will do. if key_pressed[K_RIGHT]: xpos += movement_x if key_pressed[K_UP]: ypos -= movement_y if key_pressed[K_DOWN]: ypos += movement_y Now that the program knows where to move, it's time to place it on the window. screen.fill(black) But, before we show the next circle, we need to clean the one before, we do this filling the screen withour black color. (Clean this line to see what happens) We haven't done this at the beggining cause the screen is already black. circle = pygame.draw.circle(window, red, (xpos, ypos), ray_circle) Now that the window is cleaned, it's time to place the new circle at it's new position. pygame.display.flip() Like before, to show the new circle we need to refresh the window, and like we've seen before, this is made using the command pygame.display.flip(). Ok, now you have your first game, a ball that moves around a black window. (Fine it's not unreal or starcraft, but we need to start somewhere, right?) Play with this code to see what happens. Any doubt, post here. Cheerz
-
Outline for Tutorial 2: a)Pseudo code for Program 2 b)Writing Program 2: the if statement c)Enrichment:creating readable output d)Relational operators,compound assignment operators e)Data types float, double, char f)Enrichment: debugging -Summary and Exercises Problem #2: A program is required that determines if or if not a person can register for courses based a specific formula. The person's GPA is plugged into the formula result = formula(GPA) and tested if or if it is not greater than zero. If it is greater than zero, the program will print "Admit". Construct a chart showing various values for grade point average and formula.The formula is as follows: result = GPA3+7GPA-1 GPA2-GPA + 5 3 a) Since we have to construct a chart showing various values, we should use a loop structure. So... for values of gpa, from 0.00 to 4.00, increasing by increments of 0.50 compute result = formula(GPA) print GPA, result. if result is greater than or equal to 0 print "Admit" * The 0.50 increment is only an example of the increment. Any increment can be used in this program example but for teaching purposes 0.50 was chosen. b)Writing Program 2: The if Statement: The first few lines in our program will be exactly the same except for the comment. Which will look like this: //Problem #2://creates a table to evaluate a formula based on values of//GPA in the range from GPA = 0.00 to 4.00 in units of 0.50#include <iostream>using namespace std;int main(){ Now what we need is a declaration of the variables and their data types. The problem already tells us to use the variables GPA and result so all that is left is the data types. So we examine what values GPA and result hold in this program. The GPA values start at 0.00 and go up in increments of .5 but the only data type we have seen so far is int. Declaring GPA as int will not work because data such as 1.5 will have the .5 dropped and be stored as 1.So now the data type double will be introduced. The data type double values to hold a real number, one that may contain decimal places. Clearly since result comes from computation using GPA, the data type is bound to contain decimals and therefore is also of data type double. So, here is our declaration: double GPA, result;Next is our for loop which should look like this: for (GPA = 0.00; GPA <= 4.00; GPA = GPA + 0.50)After our for loop we compute the result. For now we'll simply just write the assignment statement as result = formula Since translating the formula to code can be tricky we'll cover it a little later.After this we print the GPA and the result with this: cout<< GPA << " " << result << endl;Last is checking if the result is greater than or equal to zero. In C++, a conditional or if statement is used to ask a question or make a decision. If the result is greater than or equal to zero then we print "Admit". If the result is less than zero then we don't print. In either case we want to go to a new line to continue processing the next value of GPA. So our code for it should look like this: if (result >= 0) cout << " Admit"; cout << endl;The general form of an if Statement is this: if(condition) // no semicolon here stmt-1; //end of if statement stmt-2; //next statementSo for our example if the result were to be less than zero; it skips statement 1 and moves onto the next statement. Now onto writing the formula in C++ result = GPA3+7GPA-1 GPA2-GPA + 5 3 By straightforward translation our formula comes out to this: result = GPA * GPA * GPA + 7 * GPA - 1 / GPA * GPA - GPA + 5 / 3; //This is incorrectThe straightforward translation ignores precedence of operations therefore is incorrect. The Arithmetic Precedence Along with precedence there is the concept of associativity which has priority if two operators with the same precedence are next to each other. So an expression such as a-b+c where both operations have the same precedence, the one on the left is done first because of associativity. The C++ Arithmetic Precedence Rules with Associativity Rules: Associativityhighest precedence(done first) unary minus unary plus ++ -- right to left * / % left to rightLowest precedence(done last) + - left to right So, our translation for the formula is to be changed based on precedence and parenthesis just like in math has higher precedence than multiplication and division. The changed formula is as follows: result = (GPA * GPA * GPA + 7 * GPA - 1) / (GPA * GPA - (GPA + 5) / 3); //correct one Our entire program for Problem 2 so far is: //Problem #2://creates a table to evaluate a formula based on values of//GPA in the range from GPA = 0.00 to 4.00 in units of 0.50#include <iostream>using namespace std;int main(){ double GPA,result; for(GPA = 0.00; GPA <= 4.00; GPA = GPA + 0.50) { result = (GPA * GPA * GPA + 7 * GPA - 1) / (GPA * GPA - (GPA + 5) / 3); cout << GPA << " " << result << endl; if(result >= 0) cout<< " Admit" << endl; cout <<endl; } cout << "The Chart is Finished" <<endl return 0;} ***section c-f & exercises coming later***
-
My tutorial, assuming I stick with it will go like this: NOTE: These tutorials are for educational purposes don't ask me how to create botting programs or anything of that sort. Book I'm teaching from: Problem Solving With C++ by Jacqueline A. Jones and Keith Harrows. Compiler used for assignments and programs will be dev-c++. Tutorial 1: First C++ Program a)Pseudo code for Program 1 b)Basic Concepts of a C++ Program c)Declaration, Assignment, and Print Statements d)The for loop e)A Better version of Program 1 f)More details: comments,arithmetic operations, identifiers, for loops -Summary and Exercises Tutorial 2: Evaluating an Expression a)Pseudo code for Program 2 b)Writing Program 2: the if statement c)Enrichment:creating readable output d)Relational operators,compound assignment operators e)Data types float, double, char f)Enrichment: debugging -Summary and Exercises Tutorial 3: Reading a set of data a)Pseudo code for Program 3 b)reading data from cin c)The while loop d)Writing Program 3 e)Enrichment: Improving the input/output, Prompts and printing f)The if-else statement g)File I/O -Summary and Exercises Tutorial 4:Summation, Stepwise Refinement, and Nested Loops a)Pseudo code for program 4 b)Program 4 (first version) c)Enrichment: Other versions of program 4--declaring a loop index, Defining a constant, and reading data d)Enrichment: using a nested loop -Summary and Exercises Tutorial 5: Functions a)Function subprograms b)Programmer defined functions c)Program 5; Location of functions d)void and parameterless functions e)Reference parameters -Summary and Exercises Tutorial 6: More on control structures Tutorial 7: Arrays Tutorial 8: Strings Tutorial 9: Mix and Match Tutorial 10: Simple classes -Tuts 6-10 will be more detailed if 1-5 works out.
-
Outline for Tutorial 1 a)Pseudo code for Program 1 b)Basic Concepts of a C++ Program c)Declaration, Assignment, and Print Statements d)The for loop e)A Better version of Program 1 f)More details: comments,arithmetic operations, identifiers, for loops -Summary and Exercises Problem #1: Write a complete C++ program to do the following: Display a list of numbers from 4 to 9. Next to each number display it's square.(The number multiplied by itself) a) Pseudo code for Program 1: First of all, what exactly is PseudoCode? -Well Pseudo code is used by most programmers to help translate an English-language description of a problem to a program written in C++. To start writing the program to solve Problem 1, look at the statement. Read it through carefully to comprehend what is being requested. -Basically the problem requests that we Start with the first number and compute its square. Print the number and its square. Then do the same for each of the other numbers. Our Pseudocode will look like this: start with the number 4 compute its square print the number and its square do the same for each of the other numbers from 5 to 9 (Writing each step of the program on separate lines makes it easier on the eyes.) Basic concepts of a C++ Program We will start program 1, and every other program taught in the tutorials with a comment The comment provides a short description of what the program is suppose to do. Comments are not necessary for programs to work, but they provide detailed description for anyone reading the code. A possible comment for problem 1 might be this: // First Program // Print the numbers from 4 to 9 // and their squares *Note that each line of the comment begins with " // " Once the // symbols are seen by the C++ compiler everything to the right is ignored. If the comment is too long to fit on one line, break it down into multiple smaller comments. Next is the program header. After the comment, we can start writing the program. The next three lines of our C++ program looks like this: #include <iostream> using namespace std; int main() Any line in a program that starts with # is an instruction to the compiler, not an actual statement in the C++ language. The line containing #include tells the compiler to allow our program to perform standard input and output operations. The #include directive tells the compiler that we will using parts of the standard function library. A function is a building block of a program, typically each function performs one particular task. Infformation about these functions is contained in a series of header files. iostream is short for input/output stream and putting the name inside < and > tells the compiler the exact location of this header file. The line using namespace std; allows us to use certain shortcuts when specifying the names of items from C++ libraries. The third line int main () is called the main program header. It tells the C++ compiler that we are starting to work on the main function of this program. The word int says that this program will return an integer, and the empty set of parenthesis indicates the main program will not be sent any information from the outside. Last of portion b is the body and action of the program. Just as English is composed of sentences, a C++ program is composed made up C++ statements. Inside every C++ main program, after the header is a set of braces " { " and " } " that hold the C++ statements which comprises the body. This is call the action portion of the program. Here is the outline of our C++ program //... #include <iostream> using namespace std; int main () { //action portion of the program goes here ... } c) Declaration, Assignment, and Print Statements The first thing that will be introduced is a declaration statement. The declaration tells the computer which storage locations or variables to use in the program. Variables are used for declarations. A variable is a name for a physical location within the computer that can hold one value at a time. Variables can change its value as the program is running. Variables can be called bob[u/] , charlie[u/] , x , y , or just about anything you like. But generally for good style, meaningful names that suggest how the variables are being used us recommended. That is why for Problem 1 the variables number and sqnumber will be chosen. The declaration for Problem 1 will look like this: int number, sqnumber;Or can be written like this: int number; int sqnumber; *notice that we have int before number and sqnumber. Int is our data type. The variables we are using are used to hold numbers, specifically integers. Also notice the semicolon ( at the end of the declaration. A semicolon terminates every complete statement in C++. Think of it like a period that ends a sentence in English but for C++. Semicolons are not needed for comments and complier directives which contain #include. After out declaration statements we have our assignment statements. We must remember that declarations simply set aside space for variables. It does not give them values. (Another type of declaration statement that gives a value to a variable will be discussed in Tutorial 3) The way to give a value to a variable is through an assignment statement. For Problem 1 we want to start with the number 4 so we will have this as our assignment statement: number = 4;Now, in this assignment statement we use the symbol =. This is the assignment operator in C++. A proper way to read the statement is "number is set to 4" or "number is assigned to 4" or "number takes the value of 4" but if you read it as "number is equal to 4" you confuses the assignment operator with the word "equals". The variable number does not necessarily equal 4 it just starts at 4 in our case.The general form of an Assignment statement is: varname = expr;the name of the variable followed by the assignment operator then the value of the expression. Problem 1 says that we need to print the number and square number. It is not necessary to print every calculation just ones that you want to know about. In this case mainly the initial and the result. The printed or displayed results of running a program is called the output. So, our Pseudo code for printing will be this: output number and sqnumberThe simplest way to print in C++ is using the standard output stream cout as follows: cout << "My first program." << endl; We can also use cout to print the value of a variable or an expression as follows: cout << number; For Program 1 we will need this: cout << number << " " << sqnumber << endl; Now, I will go into detail what is happening or what is used in the printing statements.The << is call an insertion operator. It follows cout and then by the item whose value we want to print. Each value we want to print is preceded by the insertion operator. The "endl" is the command for a newline. Basically it says to end the line and move to the beginning of a new line. If endl is not printed then the printed items will all be on one line. The quotes and empty space allows us to print an empty space between number and square number. This is called a literal string and more on strings will come later in Tutorial 8. Now for out line of code: cout << number << " " << sqnumber << endl; we have previously declared number = 4 but we have not set anything to sqnumber. Therefore we have to set it before we can use it or else a totally different number that what you want will be printed. A square number is a number multiplied by itself. So sqnumber should be assigned like this: sqnumber = number * number; Thus we have printed the number 4 and its square. We have to continue the process until we finish with 9. After we finish the process for 9 we formally end the proram with a return statement which brings about a logical end to the program. Its execution terminates the program. After the return statement we mark the physical end of the program with a closing brace to match the opening brace that appears immediately after the main program header. So, here's our first version of Program 1: //Print the numbers from 4 to 9//and their squares#include<iostream>using namespace std;int main (){ int number, sqnumber; number = 4; sqnumber = number * number; cout << number << " " << sqnumber << endl; number = number+1; sqnumber = number * number; cout << number << " " << sqnumber << endl; number = number+1; sqnumber = number * number; cout << number << " " << sqnumber << endl; number = number+1; sqnumber = number * number; cout << number << " " << sqnumber << endl; number = number+1; sqnumber = number * number; cout << number << " " << sqnumber << endl; number = number+1; sqnumber = number * number; cout << number << " " << sqnumber << endl; system("pause") return 0;} In this first version we increment the value of number by telling the computer to add 1 to the previous value of number. Every time we assign number it gets a new value. Also notice the system("pause") before the return statement. It is a statement in dev-C++ that lets you view your output on your computer monitor. If you run the program without it, then the program runs and closes without you seeing the output. d)The for loop Although the first version of Program 1 will work, it is an inefficient way to solve the problem. We are doing alot of repetitious work. Suppose the problem asked us to print from 4 to 786, the size of our program will increase tremendously plus we would have to count how many times we increment manually. So now we will have to find a better way of doing repetitions. Using a loop to repeat a series of instructions is such a way. A loop gives us the ability to write a statement once but have the computer execute it over and over. Before including a loop in program 1, lets look at a simple for loop and see how it works. Example: int i;for (i = 1; i <5; i = i + 1) //header of the loopcout << i <<endl; //body of the loop Note that the header of the loop does not end in a semicolon. That is because itself it is not a complete C++ statement just as the header of a main program is not one. The header is always followed by the body of the loop and together with the body it is a complete C++ statement.The for loop header has three specific things each separated by semicolons. An initial value, a control variable and an increment. For our initial value we set it to 1. Then for our control variable we have "as long as i is less than 5". And our increment is i plus 1. So lets see what our example does. Our example states that i starts at 1 and as long as i is less than 5 we will do the body of the loop. Our body of the loop prints out i. So our example will go like this. i is 1, then it checks if i is less than 5. 1 is less than 5 therefore we move to the body which says print i. Thus we print out 1. Next we do the increment which is i plus 1. So 1 plus 1 is 2. We do not move back to the initial anymore because it is only done once. (If we did the initial again it would set i back to 1 and we'd have an infinite loop. ) So we have 2 and we check if it is less than 5. If yes then we proceed to the body and so forth until the control variable proves false. In our example we are only allowed to execute a single statement. However a pair of braces is used in C++ whenever we want to execute a series of statements where normally only one is allowed. The entire series is called a compound statement. e) A better version of Program 1 Now that we've learned the for loop, lets use it to shorten Program 1. Doing so will change the main as follows: //Print the numbers from 4 to 9//and their squares#include<iostream>using namespace std;int main(){ int number, sqnumber; for(number = 4; number <= 9; number = number + 1){ sqnumber = number * number; cout << number << " " << sqnumber << endl; } system("pause") return 0;} f) The other stuff: First thing that should be mentioned is the spacing. Although spacing doesn't matter to dev-C++ complier it does matter to the person reading the code. Indenting is important and it helps the reader identify what is what. Notice in the code that it's 5 spaces after the braces and then we start the statements. Also it's 5 spaces on the line after the for header. Then the brace that hold the for statement is also 5 spaces. This helps us identify what code is contained within the for loop. Arithmetic Operations in C++ there are a number of arithmetic operations available in C++. For addition we use "+" For subtraction we use "-" For multiplication we us "*" For division we use "/" But note that the fractional part of the answer is lost in integer division so we use a modulus or a remainder operator for any division that yields a remainder. The remainder operator is "%" There are also shorthand for increments. Instead of "number = number + 1" we can write "number ++" just as "number = number - 1" can be written as "number --" *Exercises* 1)For each of the following C++ statements, write what happens to the section of the code as it is run in a program. Show what value is stored in each variable as each step is executed. a. y = 2; x = y + 1; y = y + 1; x = 4; b. numb = 5; cbnumb = numb * numb * numb; sqnumb = numb * numb; c. w = 6; w = w + 1; q = 2 * w; d. rate = 7; time = 5; junk = rate - time; dist = rate * time; e. for (x = 6; x <= 10; x = x + 1) y = x * 2; f. s = 0; for (i = 1; i <= 5; i = i + 1) s = s + i; 2) For each of the following C++ programs, show what is printed. a. #include <iostream> using namespace std; int main () { int x, y; y = 2; x = y + 1; cout << x << " " << y << endl; y = y + 2; x = 5; cout << x << " " << y << endl; return 0; } b. #include <iostream> using namespace std; int main () { int numb,cbnumb; numb = 4; cbnumb = numb * numb * numb; cout << numb << " " << cbnumb << " " << numb*numb << endl; return 0; } c. #include <iostream> using namespace std; int main () { int w, q; w = 6; w = w + 3; q = 4 * w; cout << w << " " << q << endl; return 0; } d. #include <iostream> using namespace std; int main () { int rate, time, dist, junk; rate = 7; time = 3; junk = rate + time; dist = rate * time; cout << rate << " " << time << " " << junk << " " << dist << endl; return 0; } e. #include <iostream> using namespace std; int main () { int x, y; for (x = 6; x <= 10; x++){ y = x * 5; cout << x << " " << y << endl; } x = 8; cout << x << " " << y << endl; return 0; } f. #include <iostream> using namespace std; int main () { int i, s; s = 0; for (i = 1; i <= 7; i++) s = s + 1; cout << s << endl; return 0; } 3) Assume variables w , x , y , z are of type int. w = 4; x = 7; y = 6; z = 1; Show what is stored in each variable in each of the following: a. z = x / y; b. x = y / w; c. x = x + 1; d. w = y - w; w = x % y; z = y % y; y = x + 1; z = y / x; 4) The following program has many errors in it. An error in a program is called a bug.It is your job to fix the program, therefore you are debugging the program. (And NO I did not make typos) // comment is ok;#includ <iostream>;using myspace: standard;void main{ ints x,y; x + 1 = 4; y = y + 1; x = 3y + 5x cout << x,y) return 0; 5) Write a complete program that would print your last name, followed by a comma, followed by your first name. Do not print anything else (that includes blanks). 6) Given an int variable k that has already been declared, use a for loop to print a single line consisting of 97 asterisks. Use no variables other than k . 7) Given an int variable n that has already been declared and initialized to a positive value, and another int variable j that has already been declared, use a for loop to print a single line consisting of n asterisks. Thus if n contains 5, five asterisks will be printed. Use no variables other than n and j .
-
This tutorial will teach you how to login in gaiaonline through vb.net. I'll do it with a way that you don't need to leave vb.net to get/post (bad joke) anything. What you need in this tutorial is: Download and extract the Resources.rar file (i'll use 'c:\resources' in this project) This tutorial Now let's startOpen the VS.NET and create a new project: You now have something, that is called a form, let's rename it to GaiaLogin Easy, let's take a look at the form What?? Form1? But i renamed it... Ok, if you really said that just close this window right now. That's the title of the form, let's rename it Click on the form (anywhere) On the properties locate 'Text' and change 'form1' to 'GaiaOnline' Ok, now we are talking. Let's insert 1 textbox on the form (see example for details) Yes, simple as drag and drop, but i want this textbox to be multiline and to have a vertival scroll. (easier to see what you'll get from teh website) So, the way that i'm going to do this is to select the textbox and go to properties (like we did to change the form1 title to GaiaOnline) and select "multiline -> true" and "Scroolbars -> Both" (yes, i changed my mind) Now just resize the textbox, mine is like this: ok, all set. Did you extract the Resources.rar? Let's use it. Save your project Copy the c:\recources\SleepModule.vb and c:\recources\Stuff.vb and c:\recources\wrapper.* to your project path (mine is C:\vb.net\GaiaLogin\GaiaLogin\) All set?Let's move then. Go back to your project. Let's add the references Ok, it was easy. Now we need to insert them on the project. You should have a tab that says "Object Browser", if you don't have it press "F2" Ok, as shown in the picture, you select the Object to insert and click on the small button that is focused in the image. Do this to AxInterop.* and Interop.* Now let's add the modules to the project, select the gaionline project and follow the pictures. Press add and let's move to the code (press 2 times on the form): you should see this Now, let's make some changes to the code: After Public Class GaiaLoginAdd Dim wrap As New HTTPWrapperlike this Ok, we are ready to go. Let's see what gaia online has for us after this Private Sub GaiaLogin_Load(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles MyBase.Loadadd TextBox1.Text = wrap.GetWrapper("http://gaiaonline.com")and press F5What did you got? Your first error The reason for that is that you shouldn't trust me as much as you think, you'll get some errors during this tutorials (come on, it's part of the job) Note, you need to stop the script before continue (to do this you have a play and a stop button in a bar, yes press the stop one) To solve this problem let's replace the above code with TextBox1.Text = wrap.GetWrapper("http://gaiaonline.com/")That's it, the slash So, now press F5 Wow, what is that? Yes, it's a little bit confusing, but it's easier to get what we want. Let's take a look to the code in that textbox. Done? What i found interesting was this: <form method="post" id="loginForm" action="http://login.gaiaonline.com/gaia/login.php"> <fieldset> <label for="username">Username:</label><input type="text" id="username" name="username" tabindex="1" /> </fieldset> <fieldset> <label for="password">Password:</label><input type="password" id="password" name="password" tabindex="2" /> </fieldset>let's give it a tryClose the window. In the code replace TextBox1.Text = wrap.GetWrapper("http://gaiaonline.com/")with TextBox1.Text = wrap.PostWrapper("http://login.gaiaonline.com/gaia/login.php", "username=Zer0&password=mypassword") 'please replace with your dataand run the script again (F5)hmm, interesting. It's shorter HTTP/1.0 302 FoundDate: Tue, 11 Dec 2007 12:05:44 GMTServer: ApacheX-Powered-By: PHP/5.1.5Set-Cookie: gaia4_ano=13858786241197374744; path=/; domain=.gaiaonline.comSet-Cookie: gaia4_ano=13858786241197374744; path=/; domain=.gaiaonline.comSet-Cookie: gaia4_sid=0057f0c85e611139e4a9cf77d01986f9; path=/; domain=.gaiaonline.comSet-Cookie: gaia4_data=deleted; expires=Mon, 11-Dec-2006 12:05:43 GMT; path=/; domain=login.gaiaonline.comSet-Cookie: gaia4_ano=13858786241197374744; path=/; domain=.gaiaonline.comSet-Cookie: gaia4_sid=f5b0b6d0194b761d7d156ad280cfe74600002f258bec3d11; path=/; domain=.gaiaonline.comSet-Cookie: gaia4_data=a%3A1%3A%7Bs%3A11%3A%22autologinid%22%3Bs%3A0%3A%22%22%3B%7D; path=/; domain=login.gaiaonline.comLocation: gaiaonline.com/?login_success=1Content-Length: 0Vary: Accept-Encoding,User-AgentConnection: closeContent-Type: text/htmllet's see what info the next page will show.after TextBox1.Text = wrap.PostWrapper("http://login.gaiaonline.com/gaia/login.php", "username=Zer0&password=mypassword") 'please replace with your dataadd TextBox1.Text = wrap.GetWrapper("gaiaonline.com/?login_success=1;and press F5 againHmm, bigger again. But look what i found in the code src="http://s.gaiaonline.com/images/gaia_global/gaia_header/ic_shortcuts.gif" alt="Shortcuts"/>Shortcuts</div></li> <li id="messages"><a href="/profile/privmsg.php" class="newMail">(2)</a></li> </ul>I have new mail, interesting, let's see if i can read it through vb (note that i am logged already)so the link to my private messages is? I'll let you answer this one. Let's keep going with the code. I want to read my messages now. after TextBox1.Text = wrap.GetWrapper("gaiaonline.com/?login_success=1;I'll add TextBox1.Text = wrap.GetWrapper("gaiaonline.com/auth/?redirect=%2Fprofile%2Fprivmsg.php;and press F5 againAnd yes, after a while i found this <img src="http://s.gaiaonline.com/images/template/folder_new.gif" width="19" height="18" alt="New Message" title="New Message" /></td> <td valign="top"><span class="topictitle"><a href="/profile/privmsg.php?folder=inbox&mode=read&id=4537.1478364922" class="topictitle"><strong>You've received a special gift!</strong><br /><span class="gensmall" style="font-weight:normal;"></span></a></span></td> <td valign="top"><span class="name"> <a href="/profile/?view=profile.ShowProfile&item=4537" class="name" style="font-weight:bold;color:#D75252"><strong>[NPC] Rina</strong></a></span></td> <td NOWRAP><span class="postdetails"><strong>Thu Dec 06, 2007 5:36 am</strong></span></td> <td align="center"><input type="checkbox" name="mark[]" value="4537.1478364922" /></td> </tr> <tr> <td colspan="5"><img src="http://s.gaiaonline.com/images/template/s.gif" width="1" height="2"></td> </tr> <tr bgcolor=#FFFFFF> <td valign="top"><img src="http://s.gaiaonline.com/images/template/folder_new.gif" width="19" height="18" alt="New Message" title="New Message" /></td> <td valign="top"><span class="topictitle"><a href="/profile/privmsg.php?folder=inbox&mode=read&id=10566556.1476371945" class="topictitle"><strong>(no subject)</strong><br /><span class="gensmall" style="font-weight:normal;"></span></a></span></td> <td valign="top"><span class="name"> <a href="/profile/?view=profile.ShowProfile&item=10566556" class="name" style="font-weight:bold;color: ##2244A2"><strong>ICAN'T SHOW YOU THIS NAME</strong></a></span></td>Wow, ok, i'm not going to show you that message now that we are logged.I just want to finish this. Let's move to the design again. But first, take a look at the code that is parsed to the html, you should notice that you have one thing different that show us that we are logged. Did you find it? Good, let's move then Remove that stupid textbox1. Create something similar to this Ok, what you should know by now is how to set a name to a textbox or to a button (in the properties), so let's use our capabilities, i named the button as Loggin, the first textbox as username and the second as password. Let's move to the code (press 2 times on the form) Hmm, i forgot, you want to login using the button ( see the errors are coming to us ) Ok, let's go back to the form, now, instead of clicking 2 times on the form, let's do the same on the button. Good, you should see a new thing on the code Private Sub Login_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Login.Click End Sub haha, let's move it.Add this to the login button Dim strHtml As String strHtml = wrap.PostWrapper("http://login.gaiaonline.com/gaia/login.php", "username=" & username.Text & "&password=" & password.Text) strHtml = wrap.GetWrapper("gaiaonline.com/?login_success=1;that's it, press F5, insert your data and try now.Easy hein? What? Nothing showed up? Damn, i forgot again, the thing we need to know that we are logged is "Gold:" Let's see. add this code to the button If InStr(strHtml, "Gold") Then MsgBox("You are logged!") Else MsgBox("Loggin failed!") End IfPress F5 and insert your data Hehe, cool... OK goo lu... What? You want to know the amount of gold in the account? But that's a new function... OK ok, calm down. It's also easy Let's change the if thing. After the "If instr(strhtml, "Gold") Then" Let's add Dim gold As String = wrap.iB(strHtml, "Gold: </span>", "<") MsgBox("You have " & gold)The iB, is the function in between, if you had look to the code you would see that the amount of gold is between Gold: </span> and <, and that's what we want. Run the program. HEHE, we did it. Now it's done, (donate i'm poor ) Now good luck at your code. Resources.zip
-
As i said on other tutorial. This are tutorials from Gaianlurker on the New Engin3 forum. Alright, this tutorial was going to be Variables and Functions, but that would just be massive so I broke it down. Let's start with a basic program; #include <iostream.h> //cin and cout functions are hereint main(){ int a, b; cout<<"Welcome to GaianLurker's basic math thingy."<<endl; cout<<"Please enter a number."<<endl; cin>>a; cout<<".. and another?"<<endl; cin>>b; cout<<a<<"+"<<b<<"="<<a+b<<endl; cout<<a<<"x"<<b<<"="<<a*b<<endl; cout<<"The remainder of "<<a<<"/"<<b<<" is "<<a%b<<endl; return 0;}int a, b; This is a declaration line of variables "a" and "b". Both of these variables are integers (for now), so they are given the type "int". Other types include char(acter), string, double, and there are modifiers as well. For instance, you may want to use an "unsigned short integer", which would limit the size of the variable, and not allow it to hold a negative number. Since this is the variable tutorial, any questions regarding variable types can go here, but please don't rush with modifiers and such as we're not there yet, by far. Alright, anyway.. I rant a lot so.. I'll try to be more on-track as we go along. To declare a variable, the syntax is: type name; You can declare several variables of the same type as such: type name, name, name, name; So for example, we may have: int i, j; string username, password; char favletter; all within the same .. declaration area (trying to avoid the word scope for now). You can see some basic manipulation of variables in my example, but let's get more in-depth. Math things: There is a math.h header file that includes functions such as sqrt() (for square roots.. duh?) but for now, we're sticking to the basics. To add variables, simply type "a+b" (no quotes) To subtract variables, type "a-b" (no quotes) To multiply, a*b To divide, a/b To get the remainder of division (no long division is done): a%b (5%2 = 1) Order of Operations are followed, and you can use ()s to give priority, as PEMDAS dictates. Variables may be mixed with numbers, as long as the math makes sense. ex: if a = 5.. a/5 = 1, and 5/a = 1 New concepts (stuff you didn't use in algebra.. exactly..): C++ actually means "C +1" You can take a variable, and "increment" or "decrement" it with ++ or -- respectively. For example; a=5; a++; //a now equals 6 ++a; //a now equals 7 a--; //a now equals 6 --a; //a now equals 5 Also, you can assign variables on the fly. a=5; b=3; c=a; //c now equals 5 c=c+b //c now equals a+b.. or 5+3.. or 8 You can always make something equal to itself, plus another variable in that format. x = x+y <--- will make x now whatever x+y was at the time. Another syntax for this is x += y.. This is easily read as x additionally equals y (I made that up.. you may not see that anywhere else or it may be how it is said.. xD) .. but What else does this work for? x = x-y .. does this equal x -= y? .. Sure it does. What about x = x*y? .. Not necessarily, and as far as I know, not at all. I suggest sticking to x = x*y as opposed to x*=y. Only use the shorthand for + and -. Don't forget that to declare something, the left side of the equal sign must be a variable ALONE. No coefficient or exponent is allowed on the left side. 5*x = y is NOT allowed. y = 5*x is, however. I'm running out of things to remember, if you think I've missed something, please let me know.. or I'll explain it if/when it comes up. Typecasting. >.> What's that? Well, sometimes you're going to need to make a variable hold a different type. Say you want to use an operation that only double/float (numbers with a decimal) can perform... yet you want to perform this action on an integer value. Well, that's simple. An example: int a=5; //Yes, you can give them initial values when declaring variables double(a).. blah blah. That's typecasting. We may or may not see it later, but in case you ever find a need for it, simply put the new (temporary) type, and the variable in the syntax type(variable). This will behave exactly like the variable in the ()'s as if it was that particular type. Constants.. Oops, when I wrote this up I forgot about constants. Constants are variables that never change. For example, you want variable c to = a*b, no matter what a and b are. int a, b; const int c=a*b; a=5; b=3; //c=15 a=3; //c=9 now b=5; //c=15 again. Get the idea? It's not a hard concept to grasp, just something I forgot about until the last second.
-
This are not my tutorials, althought it's always nice to share. And i got the permition for these. (Thanks to GaianLurker for this) Enjoy and try to keep up. #include <iostream.h> // io = in/out stream.. I'll explain.int main(){ cout<<"Hello, Xisto."<<endl; return 0;}This is a very basic program. In fact, it's the program most tutorials start with in any programming language, so.. it's the one I'm starting with. #include <iostream.h> Let's start here. # is a signal used to say something should be done first. Yes, I know all the terminology, but I'm going to spare you. #include, #define, and others are to be done before your actual program. Right now, let's just deal with #include. Some of you may have seen Zero's work in vB. TONS of files come with his program, right? Well in C++, our dependant files get "included" in our main program. If you're familiar with DLLs, it's like that. The extension .h is for a header file. Most header files are really just where the compiler has taken several tasks that would be very difficult to code by hand, or are written in ASM for speed.. and make them easily accessible to you. iostream stands for in/out stream, which refers to accepting input, and expelling output. You may have noticed the comment syntax; //. Anything after // in a single line is commented out. This means that the compiler will ignore it and you may use the space to explain things for yourself and others. A good programmer leaves good comment. If you start out with 50:50 code:comments, you'll learn the proper balance as you learn to properly code. int main(){} Alright, let's ignore what's INSIDE our function for now. Anything you see that has () after it is a function. Functions are snippets of code that perform actions (usually, we'll assume they do for now). int stands for integer. Every function has to have a type. The types we will most likely deal with in these tutorials will be int, double, char, (maybe strings), and void. Right now let's focus with what we have. If something is an int function, it must return an integer. That's why we have the return 0; line. Hypothetically we could include THIS program could, for example, check if the fishing servers of Gaia Online are up. It could return 0 if all 3 connected successfully, or 1 if one of the servers failed. The other program could check if our main here returned 0 or 1. >.> Maybe I'm getting ahead of myself, so let's look at the syntax again. type name() {} Is a function's syntax. Every program must have a main(), which I'll explain when we get into scope (much later o.o).. Inside the ()'s we will put arguments, and inside the {}'s you put the code executed when the function is called. cout<<"Hello, Xisto."<<endl; (alternatively cout<<"Hello, NEF.\n"; or many others)Alright, let's start with the basics. cout is a command that is defined in iostream.h .. and you can see the syntax here. When you want to display something to the command prompt, the command is cout<<"RAW TEXT"; Every line of code must end in a ; .. for now. There are exceptions, but you can almost guarantee if there's a line of code, it'll need a ; after it. cout is very special in that it can be used several times in one line without repeating the command. For example, you can type cout<<"Hello, "<<name<<". How are you?"; if "name" is a variable with their name. One variable that is always declared, unless you declare it and give it a value (please do NOT do this to reserved words, such as cout. You'll just shoot yourself in the foot.) would be our friend "endl". endl stands for end line, and will move your text down to the next line. Think of it as an enter space. If you are in raw text string, you can also use \n to do the same. return 0; We had to return a value for our int main(). Although most interpreters will consider this "understood" if you omit it.. it's good practice to make sure it's there, especially since when we get into REAL functions, they'll rarely return 0.
-
To Automatically Run Command When Login / Logoff
de4thpr00f replied to magiccode9's topic in General Discussion
Hehe, sweet. Simple and without the need of tweak programs.By the way, do you have anything like this for windows vista?Thanks in advance.Greetz~Jo?o Lopes -
I test my scripts at my pc. It's easier if you want to make a lot of changes in the script. But from the topic i thought you wanted to know how to test variables, lol.The answer to test variables is echo them. (for me at least)Greetz~Jo?o Lopes
-
Can you guys tell me what this do? I can't get it.The topic is not very clear.You use this script in wich situations?Greetz~Jo?o Lopes
-
A domain normaly is a redirection. So if the host is Xisto and you'll only change the name, when yot go on the domain you'll be mirrored to your host. So no traffic lost. Both links will work. If Xisto is not your future host, create a new index for your Xisto homepage with the info and links for the new website.
-
I don't think it's an insentive to illegal download and maybe ms will make a special release to Singapore.Maybe Singapore is not with lack of money lol. I don't get why governament don't look on the bright side of the things. Why forbidd pixels?I bet they don't have Mtv...Anyway, nice post.Greetz~Jo?o Lopes
-
Install An Aef Forum Onto The Trap17 From a zip file
de4thpr00f replied to jlhaslip's topic in General Discussion
Really nice tutorial. I didn't know you could extract on server without ssh.Great job, keep up the good work.Greetz~Jo?o Lopes