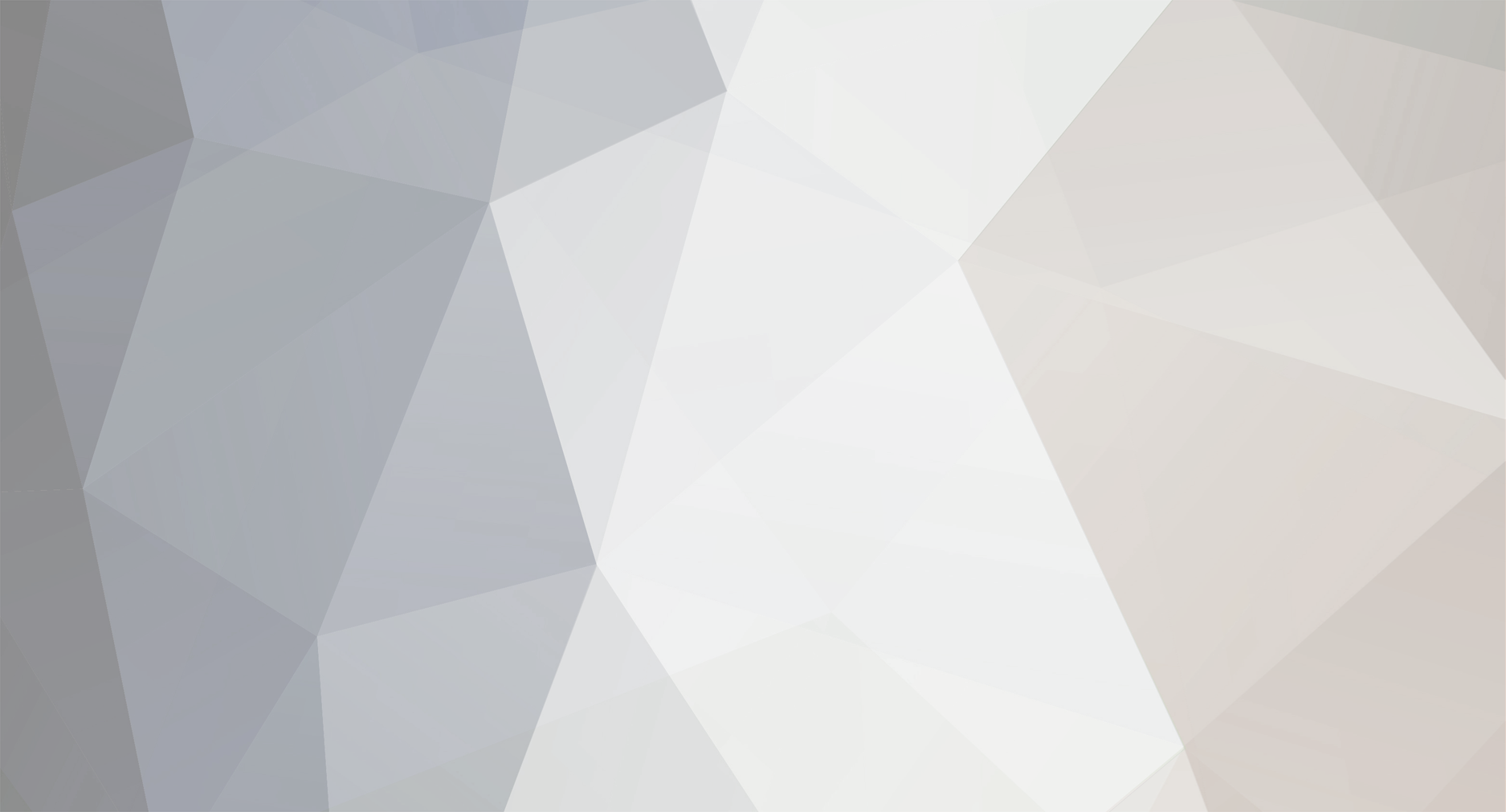
pointybirds
Members-
Content Count
12 -
Joined
-
Last visited
Everything posted by pointybirds
-
Data Structures -- Linked List -- Reverse Reverse a linked list
pointybirds replied to varalu's topic in Programming
There aren't too many simpler iterative algorithms than the one you've given. I would perhaps have the function return a result: Node *reverse(Node *head) { Node *curr=head, *prev=NULL, *next; while (curr != NULL) { next = curr->next; curr->next = prev; prev = curr; curr = next; } return prev;} -
Yes, there's a test and if you fail you'll be sent to Siberia. Unless you're from Siberia, then you'll just be hit with a cricket bat. How about: for (0 to halfway through string) { push letter onto stack}is_palindrome = truefor (halfway through string to end of string) { if letter != (pop letter from stack) is_palindrome = false end for loop }} Or, if you want to save your typing fingers and know some perl, use this comparison: (substr($str,0,length($str)/2) eq reverse(substr($str,-length($str)/2)))
-
Yep, just like the poor man's patent. However, the security of the digital signature (and the non-repudiation it provides others too) is superior to an envelope, mainly because with physical post there's no tying together of the postal stamp with the actual contents of the envelope, so it's more open to forgery. On the plus side, the postal service is recognised and trusted by far more people ... if only we had a large scale "standard" digital timestamper out there!
-
Hi all, If you've ever been in a position of needing to prove that you wrote something on or before a certain date, here's a relatively easy way of going about it: Create a crypto hash of the data in question using ripemd-160, sha-1 etc. Submit the md5sum to a 3rd party timestamping service (e.g. http://www.signedtimestamp.org/) You will receive a digital signature of what you submitted, containing your original data and a bunch of timestamp info Now, if you need to prove to someone the date you submitted the data, you can show them the result of the hash in step (1), then recover the original data from the timestamp server's email using its public key, and compare the values. If they match, you have your proof. If they differ, then you either don't have the same original data or you've gone awry in the digital signature recovery process. Note that in the case of the above timestamp server, you could submit the data itself, however there's a 16kb size limit so it's best to send a hash instead for any largeish data.
-
Two solutions spring to mind. Firstly, you could pass the root nodes of both trees into a recursive function and compare their children. If they have an identical number & position of children, continue to recurse, otherwise unravel and report "different". If the function returns with no report of difference you have identical trees.Alternatively, if you don't have both trees at the same time to compare, you could create a model of a tree using bit strings to denote the presense or absence of nodes. E.g. a fully populated three-level tree would be represented as 1111111 ... leave off the bottom leftmost child and you get 1110111. Then run your final bit string through a hash (e.g. md5sum) and you have a fingerprint of your tree structure. This way you'd be able to store the structure of a tree in a few bytes for future use.
-
eval will run the contents of the string as code, however it interpolates variables too, so the above code will replace $name with "test", then uses $test in the eval.
-
Alex, I believe the evil eval could be your friend here: $test=array(1,2,5);$name="test";eval ("\$blah = $${name}[2];");echo $blah; Use it with care and good luck!
-
Hi,Certainly there are some things you can do adequately with good old procedural programming -- Turing even posited that you can simulate any possible computer with a few symbols -- it's just that certain types of programming problems lend themselves better to the OO way of doing things, and I'm thinking specifically of two of OO's main features: inheritance and polymorphism.To use your ball example, the code could become unwieldy when you start getting lots of different ball types, and you have to keep track of them all manually with a .type element in a struct, and select between them with IF statements. The OO folks would have you create an overarching "ball" class, with basic actions for a ball such as bounce(), rollI(), animate() etc. Then, for different types of ball, you subclass this into classes like Football and Tennisball and override the generic methods as required ... some balls bounce higher than others, that kind of thing. Now the code itself is taking care of what _particular_ subclass an object is, and you don't necessarily have to know or care. You can also code other objects with animate() methods, and call them in a loop:for object in (objects) { object.animate()}There's nothing invalid about the procedural way of doing it, it's just not as clean and maintainable for certain applications such as GUI programming where objects fall naturally into classes. Also, I think you'll find that if you do it procedurally you'll spend a lot of your time reinventing ways of dealing with objects that OO specifically sets out to solve.I would suggest reading up on some of the fundamentals of what makes OO OO (if that makes sense) before your next class so you don't get any more zingers. I've been there before and it's not comfortable. Good luck.
-
Data Structures -- Linked List Find the nth last element in linked list.
pointybirds replied to varalu's topic in Programming
Hmm, presuming a singly linked list where you can't start at the end and work your way back, you could use a circular buffer with 5 elements (e.g. an array that can store the contents of the LL nodes) and traverse the list until you reach the end ... then, the "next" element in your circular buffer is the fifth last. E.g.: for (i=0; (e = next element) != null; i++%5) { buf = e } fifth_last = buf Something like that I guess. You'd also need to initialise your array to null and check for this at the end in case your LL < 5 elements. -
That which we call a soul is merely the higher functions of our brains, brought about by electrochemical impulses shooting around our synapses. I don't support reductionism, and there's definitely a gestalt aspect to consciousness that can't (yet?) fully be explained by recourse to the sciences of chemistry and physics. However, without the active brain, we have nothing. Imagine if Shakespeare's plays (or Coldplay's songs, etc., whatever inflates your dinghy) were stored on hard drives round the world and nowhere else. They bring us indescribable thoughts and emotions. Now, a nasty virus wipes them all out. So all along, we were fundamentally dependent on microscopic, magnetically aligned grains for our art and culture; but by no means could we say they represented them at any "soulful" level.Decartes wrestled with all this and came up with the mind-body dualism. Since then, the idea of a ghost in the machine has come to seem a little quaint and old-fashioned, however we persist with an oxymoronic belief in life after death. Perhaps Decartes would have benefited from something like a spiritual OSI model to explain how the world might work at different levels.Why isn't it enough that this life is all we get? Does the thought of an eternity without existence fill us with dread? Do we think that we're unfairly treated in this life and hope for something better in the next, like all those earth-inheriting meek folk? You'd better enjoy it while you can; like a toilet roll, it gets faster as you get closer to the end ...
-
Does Practice Really Make Perfect? Heres my thought...
pointybirds replied to Seidhrith's topic in General Discussion
Well obviously the expression's not perfect, but we can always work on it ... On the other side of the coin is the expression my old boss used to recite to us: "If you aim at nothing, you'll reach it every time." -
I learnt C programming through a mixture of theory and practice, but it wasn't until I started tutoring in the language that my grounding in it became decently solid. There's nothing quite like needing to answer all manner of leftfield questions to really drum some of the fundaments of a language into you. Obviously this isn't possible or even desirable for everyone, but I believe the advantage came from the inquiring minds of those learning the language: If you can keep your level of curiosity up while you study a language, and follow up on unanswered questions you might have, you'll get a far better understanding of the language.Also, very importantly: Read other people's code. Not only for their successes and different ways of solving problems, but for the novel ways that they find to make mistakes. Nobody could write a decent novel without having read a bunch of them first; there's a difference between getting across the plot of a story and telling it well.Finally, I don't think I'd recommend C as a first language unless you have a compelling reason to start using it. The basic theories of programming are more prominent and educational in languages such as Pascal/Modula-2, if you have the time and inclination to study them. Have a read through the C FAQ to get an idea of how esoteric some of the fundamentals can get!