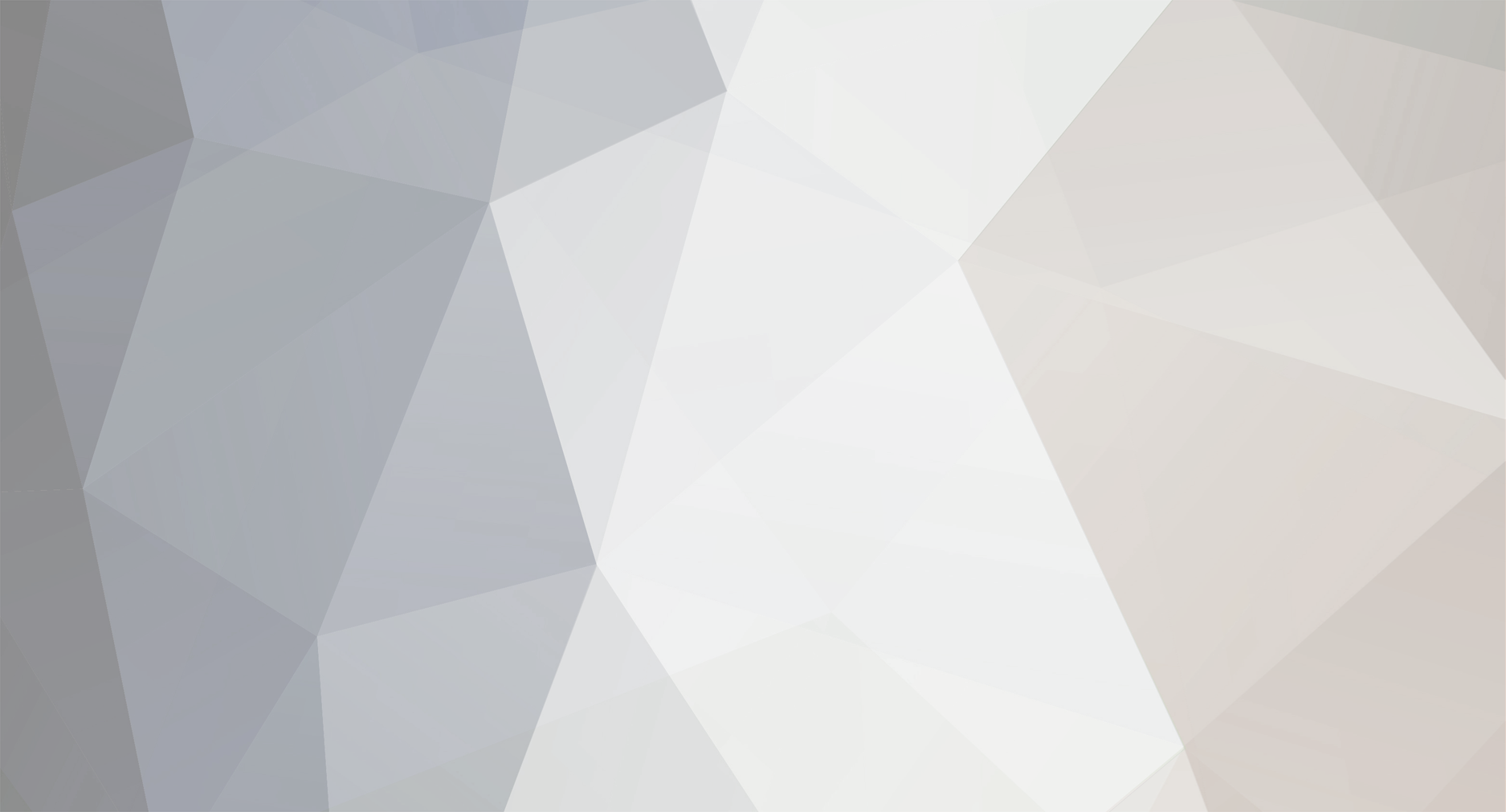
HannahI
Members-
Content Count
351 -
Joined
-
Last visited
About HannahI
-
Rank
Super Member
Contact Methods
-
Website URL
http://
Profile Information
-
Location
Northeast, United States of America
-
Welcome to chapter to of my tutorials. If you are reading this you should have also read [a]http://forums.xisto.com/topic/97893-topic/?findpost=1064410842[/a] If you did not, you should have a working knowledge of the registers ax, bx, cx, dx, instructions: add, sub, mul, div, and mov. In chapter 2, you will learn about arrays and the stack instructions. Lets start with arrays. In order to understand how arrays work, you have to understand how computer memory works. In Diagram 2.1 is an example of an array called foo and a variable called bar. In the example foo starts at memory address 675, and the variable bar starts at address 680. The assembler knows that when you refer to the word foo, it means the memory address 675, and when you reference the word bar, you are reffering to the memory address 680. If you wanted the access the nth element of foo, the equation the computer executes is 675 (address of foo) + n (index). If n was 3, the answer would be 678, which is a memory address. If you were to refer to that in the register side of the move instruction, and putting 5 on the other side, the assembler would say, "Okay, put the value 5 at memory address 678". This is the indepth explanation of the move instruction. But unfortunately, in assembly it isn't that easy. Diagram 2.1 0 0 0 0 0 5675 676 677 678 679 680foo foo foo foo foo bar In assembly it is actually pretty easy, but it requires a few steps. The first step would be to put the memory address 675 (or just type foo) into ax, lets say. Then put the element you want to access into bx, lets say. Now add bx to ax. Now you would use a special move instructiont to put the value in a specified memory address that you precalcualted when you set ax. This is shown in Example 2.1. Example 2.1 mov ax, 675;or you could just say foomov bx, 3;to access the third elementadd ax, bx;get the destination memory addressmov byte ptr [ax], 5;put five into the address pointed by ax - we'll talk more with this in the next tut on what this meansThat is basic arrays. Not to complicated. But now for stacks. The stack is actually just a very special array in assembly. When you call a c function, you put its parameters on the stack. This way you are able to hold multiple things in the same, global, array that every program has access to. There are two main stack instructions - push and pop. The push instruction puts something on the top of the stack. The pop instruction takes the thing at the top of the stack. The only problem with this is that everything is read in reverse order. You have to be very careful when dealing with stacks. The syntax for push is shown in Example 2.2. The syntax for pop is shown in Example 2.3. The register or value part of push is where you put what you want to put on the top of stack. The register part of pop is the destination of where the popped element will be stored. Thats all to basic stacks. But there are also 4 more stack instruction - pusha, popa, pushf, popf. For pushf and popf, the only differences from these registers and the other stack regster they originate from is the destination/register or value type. They use something called flags instead of registers. Pusha and popa go on their own line and do not have any parameters unlike all of the other instructions I've showed you. Pusha puts these register on the stack in the following order: AX, CX, DX, BX, SP, BP, SI, DI. Popa does the same thing as pop except for that it pops its values off the stack in the following order: DI, SI, BP, SP, BX, DX, CX, AX. Example 2.2push [i]register of value[/i]Example 2.3pop [i]register[/i] Excersises1. What did we compare the stack to?Answer: 2. What are all the stack instructions?Answer: 3. How do you compute the memory address to write to when using arrays?Answer: Thanks for learning about Assembly's Arrays and Stacks!
-
Chinese Student Jailed 10 Years For A Website
HannahI replied to marretas's topic in Websites and Web Designing
If you ask me, this guy is either doesn't know anything about marketing, or he is just a plain insane idiot. After all, what would that change his sales. Like others said, people who want to see nude aren't the kind of people who want to buy flowers. -
Man Jailed For Shouting At Parrot Its exactly how it sounds
HannahI replied to 8ennett's topic in Websites and Web Designing
Wow. Thats a pretty strange story.. and it's a bit awkard at the same time. I'm not sure if it would a very, well, correct story. (well, obviosly its true but its not the right decision for the grandmother or the court to take it that seriosly) But, then again, that is completely my person oppion.Sorry if I just wasted 10 seconds of your life XD -
Welcome to my Assembler language tutorial! Of course, this is going to be one of the longest tutorial because its the first one! I hope you'll continue learning after this tutorial. First off, let me show you a few basic things in assembly that will be very useful in later parts of my guide. Comments and sections. A comment, for those who don't know, is just a piece of English (well, actually it can be any language ) that will help support your code. The computer skips over this code, its just for human interaction. Now sections on the other hand are a bit different. The only section I'm going to talk about right now is the text section. This is where all your real code will go. The basic syntax for starting a section is shown in Example 1.1. You can change the text part to any section, not just text. They're pretty basic to use. Comments are even easier, though. You simply put a semi-colon after some code and the rest of the line is ignored by the assembler. This is shown in Example 1.2. Example 1.1 section .textExample 1.2 some code;a comment If you programmed in a higher a language before, you'll know what variables are. If you don't, you probbably remember them from algebra. They're just basicly a word that can store a value. But in assembly, they're called registers and have a slightly different purpose. If the purpose were the same, they would be called variables, not registers. Each register is assigned a name thats either 2 or 3 letters long. The reason why they're registers and not variables is that they have assigned names and purposes. In this part I'll talk about the four general purpose registers. These are registers that don't really have an assigned purpose, they're just for, well, general purpose. The four general purpose registers are ax, bx, cx, dx. The can be used for anything you want. Now you may be wondering how to access these registers. The answers is simple, you use the move instruction. When writing assembly, you write mov instead of move. It's a little confusing at first, but then you'll get the hang of it. The syntax for the move instruction is shown in Example 1.3. When the assembler reads the code in Example 1.3, it says, "Ok memory, please put 8 at the location of the AX register". Using the move instruction shouldn't be too, too, complicated. Wrapping this partion of chapter 1 up, I'll show a basic real world example using move and the registers in Example 1.4. Example 1.3 mov ax, 8Example 1.4 section .text;start the programmov ax, 5;this is a random commentmov bx, 64mov cx, 4mov dx, 61 Now that I have wrapped up basic usage of registers, I will now show something more, well, useful. Arithmetic. Since it is that complex, I'll explain all four at once. Their names are add, sub, mul, and div. They do addition, subtraction, multiplication, and division. The work exactly like the move instruction, but instead of putting values into registers, they add, subtract, multiply, and divide values from register. You will use the same exact syntax as move, except where you would put how much you want to put in the register, you put how much you want to add, subtract, multiply, or divide, depending on the instruction you are using. Example 1.5 shows some basic usage of each of these. Example 1.5: mov ax, 3add ax, 2mov bx, axsub bx, 1mov cx, axadd cx, bxmul cx, 2mov dx, 6div dx, 2 Exercises1. What is wrong in Exercise 1.1 Answer: mov dx, 5add dx 3sub dx, 1mul dx, 2 2. Try to write a program the puts 7 in the CX register, multiplies by three, and clears the cx register without the move instruction (Think )Answer: 3. How many general registers exist? Answer Hope you learned something..and that you will want to continue learning stuff about assembly!
-
Pretty awkward. I would really doubt that its real because of the fact that it had a bundled exe file. Since the exe was bundled, the spammer/viruser was trying to make you unzip it and have an unzipping program that would automatically run the document.exe, or you have a file browser that hides that file extension so it lookes like it is something like a Word document when it is really an executable.Don't know if thats any help, though.
-
I found the problem. Aparrantly, the quotes were real and were the issue Mods, please change the title to [solved] Thanks
-
I know, I know.
-
I half agree with yordan. It does make sense for them not to have their own category, but it would still make it harder to find games that run on linux. After all, linux is 'the gaming system'.
-
Yeah, most of this was basic stuff for me, so it was really no use.....
-
Looks neat, you motivated me into trying to write my own! I promise not to look at the source code