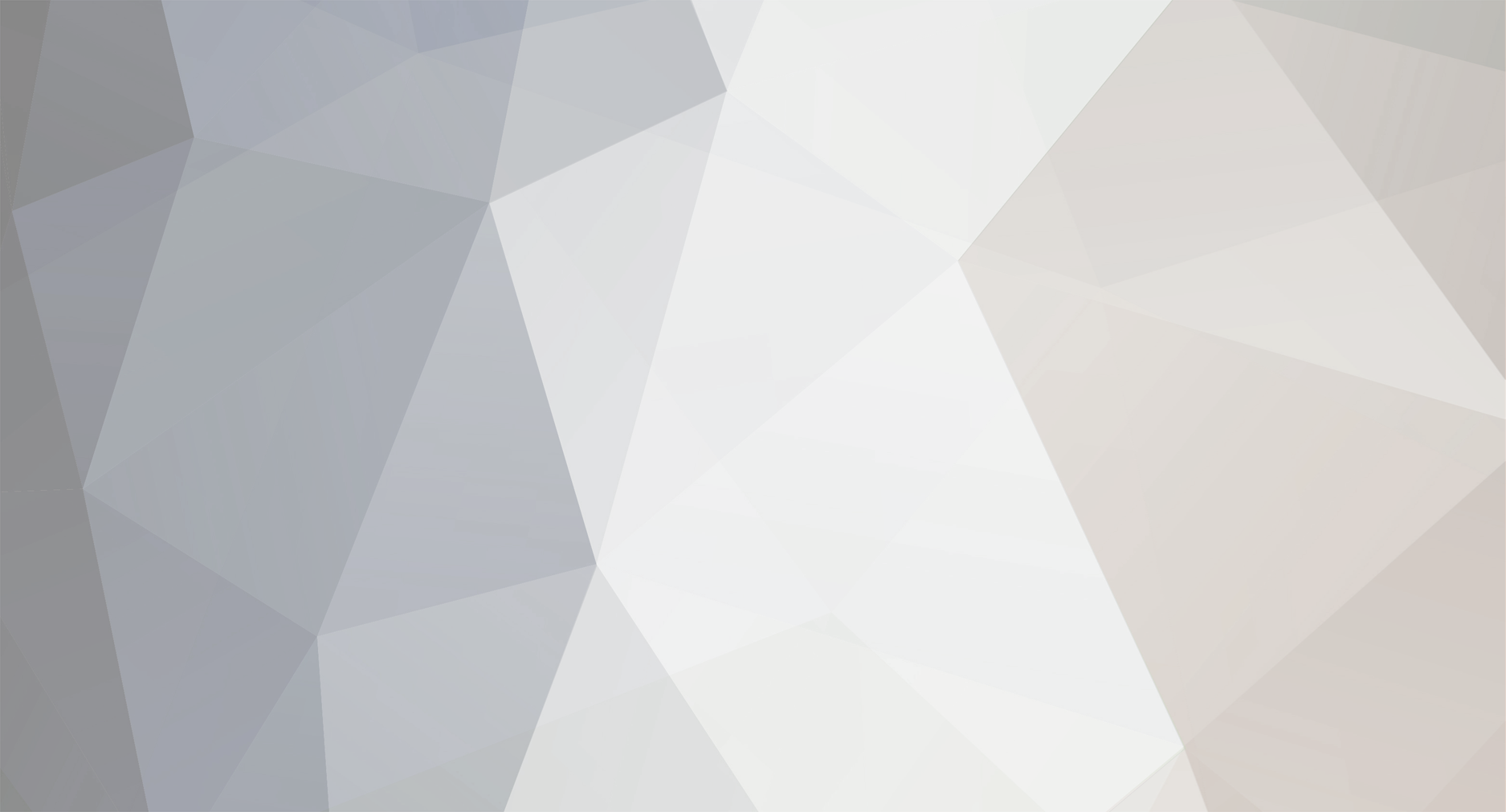
signatureimage
Members-
Content Count
49 -
Joined
-
Last visited
Everything posted by signatureimage
-
Dear kevlar557,My laptop is running Windows XP Pro Service Pack 2and Mozilla Firefox 1.0.3 without problems.Everyday, I have the virus-checker run its update process,and the same goes for the spyware detector.Connection to the internet is via a broadband router+switch+nat,with imbedded solid firewall (50$ these days).Are you certain that your machine is "clean"?
-
In this tutorial, we will explore the possibilities of generating a PDF file - on-the-fly - with PHP. The samples that are presented can be run on Xisto.com. Why would we want to generate a PDF on-the-fly ? Well, we might want to include in the PDF some data that must be entered by our surfer, by means of a html form. Or we might want to include in the PDF some data that comes from a database that is updated by another process. Or some other reason. You invent one. All reasons are legit! (1) The first thing to do, when we want to generate a PDF file with PHP, is to check that the PHP libraries, that support the creation of PDF files, are present and available to PHP. When you install a PHP distribution on your own home test-machine, you have to make shure that these PHP PDF libraries are available. On the Xisto.com server, they are available and active. Here is how to check this: - Create a new text file, let's name it phpinfo.php. - Insert the following code: <?phpinfo();?>- Upload it to your web site.- Browse to your phpinfo.php file on your web site. - Check the results. The phpinfo() function generates a html page with all the information about the PHP installation, including the information about the Apache web server, the version and settings of the PHP compiler, and the supplemental PHP libraries that are installed and activated. We have to check the pdf libraries. The results should look something like this: PDF Support: enabled PDFlib Gmbh Version: 5.0.3 Revision: $Revision: 1.112.2.11 $ (This is the result of Xisto.com - your own home test-machine may show a different result, depending on your PHP distribution.) Now that we have confirmation that the PDF libraries for PHP are OK, let's go on with our first PHP application: (2) A simple test. The creation of a PDF with PHP takes place a the web server, in memory. The first thing to do is to create a PHP PDF object, and the last thing to do is to destroy it. Here is that bit of code: <?php$mypdf = PDF_new();PDF_delete($mypdf);?>In between these two instructions, we will insert the rest of the PHP code. With this PHP PDF object, let's create a PDF file. In memory, not on the file-system of the web-server. The first thing to do is to open a PHP PDF file, and the last thing to do is to close it. Here is that bit of code: <?php$mypdf = PDF_new();PDF_open_file($mypdf, "");PDF_close($mypdf);PDF_delete($mypdf);?>In between these instructions, we will insert the rest of the PHP code. Once the PHP PDF file is open, we can start writing PDF pages to it. The first thing to do is to start a PHP PDF page, and the last thing to do is to end it. Here is that bit of code: <?php$mypdf = PDF_new();PDF_open_file($mypdf, "");PDF_begin_page($mypdf, 595, 842);PDF_end_page($mypdf);PDF_close($mypdf);PDF_delete($mypdf);?>In between these instructions, we will insert the rest of the PHP code. What do these two numbers (595 and 842) mean? They are the size of the page, measured in 1/72 inch. Our example specifies a width of 595 points = 595 x 1/72 inch = A4 paper Our example specifies a height of 842 points = 842 x 1/72 inch = A4 paper Your needs may vary... Once the PHP PDF page has been started, we can start writing dots to it. Let's write some text. First we have to choose a text font, and a text font size, in points. Here is that bit of code: <?php$mypdf = PDF_new();PDF_open_file($mypdf, "");PDF_begin_page($mypdf, 595, 842);$myfont = PDF_findfont($mypdf, "Times-Roman", "host", 0);PDF_setfont($mypdf, $myfont, 10);PDF_end_page($mypdf);PDF_close($mypdf);PDF_delete($mypdf);?>The PHP PDF function PDF_findfont() looks for the Times-Roman font,and sets the $myfont PHP variable. Then the PHP PDF function PDF_setfont() uses the PHP PDF object, and the $myfont PHP variable to select a font text size of 10 points. Our example can now start to show some text on the page. Here is that bit of code: <?php$mypdf = PDF_new();PDF_open_file($mypdf, "");PDF_begin_page($mypdf, 595, 842);$myfont = PDF_findfont($mypdf, "Times-Roman", "host", 0);PDF_setfont($mypdf, $myfont, 10);PDF_show_xy($mypdf, "Sample PDF, constructed by PHP in real-time.", 50, 750);PDF_show_xy($mypdf, "Made with the PDF libraries for PHP.", 50, 730);PDF_end_page($mypdf);PDF_close($mypdf);PDF_delete($mypdf);?>The PHP PDF function PDF_show_xy() prints the text at the specified positions. X=50 and Y=750 for the first text line, and X=50 and Y=730 for the second text line. Again, the values are in 1/72 inch. The origin (0,0) is in the BOTTOM-lefthand corner! So: Y=750 is ABOVE Y=730! Now the PHP PDF file - in memory is complete. How do we get it into the browser of our surfer? We will use the PHP PDF function PDF_get_buffer() to obtain a copy of the PHP PDF object in our own memory buffer, determine the length of it, create the http headers that are required for a PDF file, and let the web server send everything to the browser of the surfer: Here is that bit of code: <?php$mypdf = PDF_new();PDF_open_file($mypdf, "");PDF_begin_page($mypdf, 595, 842);$myfont = PDF_findfont($mypdf, "Times-Roman", "host", 0);PDF_setfont($mypdf, $myfont, 10);PDF_show_xy($mypdf, "Sample PDF, constructed by PHP in real-time.", 50, 750);PDF_show_xy($mypdf, "Made with the PDF libraries for PHP.", 50, 730);PDF_end_page($mypdf);PDF_close($mypdf);$mybuf = PDF_get_buffer($mypdf);$mylen = strlen($mybuf);header("Content-type: application/pdf");header("Content-Length: $mylen");header("Content-Disposition: inline; filename=gen01.pdf");print $mybuf;PDF_delete($mypdf);?>- Save this code as a new text file, let's name it gen01.php.- Upload it to your web site. - Browse to your gen01.php file on your web site. - Check the results. - Your first PDF, generated by PHP, will show on your machine ! Congratulations ! So, where do we go from here? Let's add some graphics to our PDF page. $myimage = PDF_open_image_file($mypdf, "jpeg", "training_bground.jpg");PDF_place_image($mypdf, $myimage, 50, 650, 0.6);The PHP PDF function PDF_open_image_file() looks for the specified image file on the web server,and sets the $myimage PHP variable. Then the PHP PDF function PDF_place_image() uses the PHP PDF object, and the $myimage PHP variable to place a copy of the image onto the PDF page. Here is the complete code: <?php$mypdf = PDF_new();PDF_open_file($mypdf, "");PDF_begin_page($mypdf, 595, 842);$myfont = PDF_findfont($mypdf, "Times-Roman", "host", 0);PDF_setfont($mypdf, $myfont, 10);PDF_show_xy($mypdf, "Sample PDF, constructed by PHP in real-time.", 50, 750);PDF_show_xy($mypdf, "Made with the PDF libraries for PHP.", 50, 730);PDF_show_xy($mypdf, "A JPEG image, on 60 % of its original size.", 50, 710);$myimage = PDF_open_image_file($mypdf, "jpeg", "training_bground.jpg");PDF_place_image($mypdf, $myimage, 50, 650, 0.6);PDF_end_page($mypdf);PDF_close($mypdf);$mybuf = PDF_get_buffer($mypdf);$mylen = strlen($mybuf);header("Content-type: application/pdf");header("Content-Length: $mylen");header("Content-Disposition: inline; filename=gen02.pdf");print $mybuf;PDF_delete($mypdf);?>- Save this code as a new text file, let's name it gen02.php.- Upload it to your web site. - Browse to your gen02.php file on your web site. - Check the results. Of course you will have to upload the jpeg image also !!!!! And we can add line-drawing functions too. The PHP PDF function PDF_setcolor() takes several parameters. PDF_setcolor($mypdf, "stroke", "rgb", 1, 0, 0);The first parameter is the PHP PDF object.The second parameter instructs that the color is used for drawing lines. The third parameter indicates that the color definition will be in RGB (Red-Green-Blue). The following parameters are the values for the Red, Green, and Blue components of the color. Our example specifies 100% Red (1) - 0% Green (0) - 0% Blue (0). Look, another PDF quirck: 1 means 100%. The actual drawing is a three-stage process: - Determine the first point. PDF_moveto() - Determine the second pont. PDF_lineto() - Draw. PDF_stroke() PDF_moveto($mypdf, 20, 735);PDF_lineto($mypdf, 575, 735);PDF_stroke($mypdf);Here is the complete code: <?php$mypdf = PDF_new();PDF_open_file($mypdf, "");PDF_begin_page($mypdf, 595, 842);$myfont = PDF_findfont($mypdf, "Times-Roman", "host", 0);PDF_setfont($mypdf, $myfont, 12);$myimage = PDF_open_image_file($mypdf, "jpeg", "training_bground.jpg");PDF_place_image($mypdf, $myimage, 30, 750, 0.6);// RGB colors: 1 = 100 % (255)PDF_setcolor($mypdf, "stroke", "rgb", 1, 0, 0);PDF_moveto($mypdf, 20, 735);PDF_lineto($mypdf, 575, 735);PDF_stroke($mypdf);PDF_moveto($mypdf, 20, 55);PDF_lineto($mypdf, 575, 55);PDF_stroke($mypdf);PDF_show_xy($mypdf, "A sample document.", 50, 40);PDF_end_page($mypdf);PDF_close($mypdf);$mybuf = PDF_get_buffer($mypdf);$mylen = strlen($mybuf);header("Content-type: application/pdf");header("Content-Length: $mylen");header("Content-Disposition: inline; filename=gen03.pdf");print $mybuf;PDF_delete($mypdf);?>- Save this code as a new text file, let's name it gen03.php.- Upload it to your web site. - Browse to your gen03.php file on your web site. - Check the results. Besides lines, we can also draw rectangles and circles. And fill them with a color. The sample PHP PDF function PDF_setcolor($mypdf, "fill", "rgb", 1, 1, 0); prepares the fill color as yellow (Red = 100% - Green = 100% - Blue = 0%) The sample PHP PDF function PDF_setcolor($mypdf, "stroke", "rgb", 0, 0, 0); prepares the draw color as black (Red = 0% - Green = 0% - Blue = 0%) The actual drawing is a two-stage process: - Determine the area. PDF_rect() or PDF_circle() - Draw. PDF_fill_stroke() Here is the complete code: <?php$mypdf = PDF_new();PDF_open_file($mypdf, "");PDF_begin_page($mypdf, 595, 842);$myfont = PDF_findfont($mypdf, "Times-Roman", "host", 0);PDF_setfont($mypdf, $myfont, 10);PDF_show_xy($mypdf, "Drawings in PDF.", 450, 765);// RGB colors: 1 = 100 % (255)PDF_setcolor($mypdf, "fill", "rgb", 1, 1, 0);PDF_setcolor($mypdf, "stroke", "rgb", 0, 0, 0);PDF_rect($mypdf, 50, 500, 200, 300);PDF_fill_stroke($mypdf);PDF_setcolor($mypdf, "fill", "rgb", 0, 1, 0);PDF_setcolor($mypdf, "stroke", "rgb", 0, 0, 1);PDF_circle($mypdf, 400, 600, 100);PDF_fill_stroke($mypdf);PDF_end_page($mypdf);PDF_close($mypdf);$mybuf = PDF_get_buffer($mypdf);$mylen = strlen($mybuf);header("Content-type: application/pdf");header("Content-Length: $mylen");header("Content-Disposition: inline; filename=gen04.pdf");print $mybuf;PDF_delete($mypdf);?>- Save this code as a new text file, let's name it gen04.php.- Upload it to your web site. - Browse to your gen04.php file on your web site. - Check the results. With the PHP instruction for () {} to do some repetitive things, we can easily create a page that contains a matrix of small squares: First the vertical lines, left to right: for ($x=0; $x<=595; $x+=20) { PDF_moveto($mypdf, $x, 0); PDF_lineto($mypdf, $x, 842); PDF_stroke($mypdf); }Then the horizontal lines, bottom to top: for ($y=0; $y<=842; $y+=20) { PDF_moveto($mypdf, 0, $y); PDF_lineto($mypdf, 595, $y); PDF_stroke($mypdf); } Moreover, let's make two pages, slightly different.Here is the complete code: <?php$mypdf = PDF_new();PDF_open_file($mypdf, "");PDF_set_parameter($mypdf, "openaction", "fitpage");PDF_begin_page($mypdf, 595, 842);PDF_setcolor($mypdf, "stroke", "rgb", 0.90, 0.90, 0.90);for ($x=0; $x<=595; $x+=20) { PDF_moveto($mypdf, $x, 0); PDF_lineto($mypdf, $x, 842); PDF_stroke($mypdf); }for ($y=0; $y<=842; $y+=20) { PDF_moveto($mypdf, 0, $y); PDF_lineto($mypdf, 595, $y); PDF_stroke($mypdf); }$myfont = PDF_findfont($mypdf, "Times-Roman", "host", 0);PDF_setfont($mypdf, $myfont, 10);PDF_show_xy($mypdf, "Drawingpaper.", 450, 765);PDF_end_page($mypdf);PDF_begin_page($mypdf, 595, 842);PDF_setcolor($mypdf, "stroke", "rgb", 0.725, 0.725, 0.725);for ($x=0; $x<=595; $x+=50) { PDF_moveto($mypdf, $x, 0); PDF_lineto($mypdf, $x, 842); PDF_stroke($mypdf); }for ($y=0; $y<=842; $y+=50) { PDF_moveto($mypdf, 0, $y); PDF_lineto($mypdf, 595, $y); PDF_stroke($mypdf); }PDF_end_page($mypdf);PDF_close($mypdf);$mybuf = PDF_get_buffer($mypdf);$mylen = strlen($mybuf);header("Content-type: application/pdf");header("Content-Length: $mylen");header("Content-Disposition: inline; filename=gen05.pdf");print $mybuf;PDF_delete($mypdf);?>- Save this code as a new text file, let's name it gen05.php.- Upload it to your web site. - Browse to your gen05.php file on your web site. - Check the results. Those of you who were very eager to learn, will have noticed one new PHP PDF function. Read the previous code again and try to find it. (It's in the begining of the code.) Or cheat and read the next paragraph. The PHP PDF function PDF_set_parameter($mypdf, "openaction", "fitpage"); allows you to have some influence over the behaviour of the Adobe Acrobat Reader, when the PDF is shown to your surfer. The setting of PDF parameters will be elaborated upon later in this post. But now is the time to add some PDF properties to our PDF file: The PHP PDF function PDF_set_info(); allows you to add meta information to the PDF file. Meta information is information about information. The information we will add will be visible with the full version of the Adobe Acrobat Reader, as document properties. Here is the complete code: <?php$mypdf = PDF_new();PDF_open_file($mypdf, "");PDF_set_info($mypdf, "Creator", "gen06.php");PDF_set_info($mypdf, "Author", "John");PDF_set_info($mypdf, "Title", "Prototype (PHP)");PDF_set_info($mypdf, "Subject", "Sample");PDF_set_info($mypdf, "Keywords", "PHP PDF PDFlib");PDF_begin_page($mypdf, 595, 842);$myfont = PDF_findfont($mypdf, "Times-Roman", "host", 0);PDF_setfont($mypdf, $myfont, 10);PDF_show_xy($mypdf, "Sample with the Document Properties. Can be observed in the full Acrobat Reader.", 50, 750);PDF_end_page($mypdf);PDF_close($mypdf);$mybuf = PDF_get_buffer($mypdf);$mylen = strlen($mybuf);header("Content-type: application/pdf");header("Content-Length: $mylen");header("Content-Disposition: inline; filename=gen06.pdf");print $mybuf;PDF_delete($mypdf);?>- Save this code as a new text file, let's name it gen06.php.- Upload it to your web site. - Browse to your gen06.php file on your web site. - Check the results. So, what have we learned so far? - We create and destroy a PHP PDF object. - We open and close a PHP PDF file - in memory. - We start and end a PDF page. - We select a text-font and a text-font-size. - We draw text at a specified place on the page. - We select a fill color and a stroke color. - We draw lines, filled rectangles and circles. - We have influence on the behaviour of the Adobe Acrobat Reader. - We add meta data to the PDF file. - We copy the PDF file into a PHP buffer and send this - via http - to the browser of the surfer. Neat. What now? Let's find out how many lines of text we can put on one page, shall we? First approach: we use the PHP PDF function PDF_show_xy(); to draw the text, and with the PHP instruction for () {} we determine the vertical position of the line. Here is the complete code: <?php$mypdf = PDF_new();PDF_open_file($mypdf, "");PDF_set_info($mypdf, "Creator", "gen07.php");PDF_set_info($mypdf, "Author", "John");PDF_set_info($mypdf, "Title", "Prototype (PHP)");PDF_set_info($mypdf, "Subject", "Sample");PDF_set_info($mypdf, "Keywords", "PHP PDF PDFlib");PDF_begin_page($mypdf, 595, 842);$myfont = PDF_findfont($mypdf, "Times-Roman", "host", 0);PDF_setfont($mypdf, $myfont, 10);for ($y=820; $y>=00; $y-=15) { PDF_show_xy($mypdf, $y, 50, $y); PDF_show_xy($mypdf, "ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789", 70, $y);}PDF_end_page($mypdf);PDF_close($mypdf);$mybuf = PDF_get_buffer($mypdf);$mylen = strlen($mybuf);header("Content-type: application/pdf");header("Content-Length: $mylen");header("Content-Disposition: inline; filename=gen07.pdf");print $mybuf;PDF_delete($mypdf);?>- Save this code as a new text file, let's name it gen07.php.- Upload it to your web site. - Browse to your gen07.php file on your web site. - Check the results. But why bother with calculating the vertical position ourselves? What if we change the text font size? Let's have the PHP PDF library do the hard work for us. Second approach: we use the PHP PDF function PDF_continue_text(); to draw the text, and we let the PHP PDF library calculate the exact vertical position of the line, according to the selected text font size... Here is the complete code: <?php$mypdf = PDF_new();PDF_open_file($mypdf, "");PDF_set_info($mypdf, "Creator", "gen08.php");PDF_set_info($mypdf, "Author", "John");PDF_set_info($mypdf, "Title", "Prototype (PHP)");PDF_set_info($mypdf, "Subject", "Sample");PDF_set_info($mypdf, "Keywords", "PHP PDF PDFlib");PDF_set_parameter($mypdf, "openaction", "fitpage");PDF_begin_page($mypdf, 595, 842);$myfont = PDF_findfont($mypdf, "Times-Roman", "host", 0);PDF_setfont($mypdf, $myfont, 10);PDF_show_xy($mypdf, "Times-Roman; 10 points. First line on: x = 30; y = 830", 30, 830);for ($y=0; $y<=80; $y++) { PDF_continue_text($mypdf, "80 Lines. ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789");} PDF_continue_text($mypdf, "Last line. (Line 82)");PDF_end_page($mypdf);PDF_close($mypdf);$mybuf = PDF_get_buffer($mypdf);$mylen = strlen($mybuf);header("Content-type: application/pdf");header("Content-Length: $mylen");header("Content-Disposition: inline; filename=gen08.pdf");print $mybuf;PDF_delete($mypdf);?>- Save this code as a new text file, let's name it gen08.php.- Upload it to your web site. - Browse to your gen08.php file on your web site. - Check the results. So, now we know that Times-Roman 10 point text fits 82 lines on a A4 page. How about Times Roman 12 point? Here is the complete code: <?php$mypdf = PDF_new();PDF_open_file($mypdf, "");PDF_set_info($mypdf, "Creator", "gen09.php");PDF_set_info($mypdf, "Author", "John");PDF_set_info($mypdf, "Title", "Prototype (PHP)");PDF_set_info($mypdf, "Subject", "Sample");PDF_set_info($mypdf, "Keywords", "PHP PDF PDFlib");PDF_set_parameter($mypdf, "openaction", "fitpage");PDF_begin_page($mypdf, 595, 842);$myfont = PDF_findfont($mypdf, "Times-Roman", "host", 0);PDF_setfont($mypdf, $myfont, 12);PDF_show_xy($mypdf, "Times-Roman; 12 points. First line on: x = 30; y = 830", 30, 830);for ($y=0; $y<=66; $y++) { PDF_continue_text($mypdf, "66 Lines. ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789");} PDF_continue_text($mypdf, "Last line. (Line 68)");PDF_end_page($mypdf);PDF_close($mypdf);$mybuf = PDF_get_buffer($mypdf);$mylen = strlen($mybuf);header("Content-type: application/pdf");header("Content-Length: $mylen");header("Content-Disposition: inline; filename=gen09.pdf");print $mybuf;PDF_delete($mypdf);?>- Save this code as a new text file, let's name it gen09.php.- Upload it to your web site. - Browse to your gen09.php file on your web site. - Check the results. Yes, now we know that Times Roman 12 point text fits only 68 lines on a A4 page. Let's compare three different text font sizes in one single PDF file, on three PDF pages. Here is the complete code: <?php$mypdf = PDF_new();PDF_open_file($mypdf, "");PDF_set_info($mypdf, "Creator", "gen10.php");PDF_set_info($mypdf, "Author", "John");PDF_set_info($mypdf, "Title", "Prototype (PHP)");PDF_set_info($mypdf, "Subject", "Sample");PDF_set_info($mypdf, "Keywords", "PHP PDF PDFlib");PDF_begin_page($mypdf, 595, 842);$myfont = PDF_findfont($mypdf, "Times-Roman", "host", 0);PDF_setfont($mypdf, $myfont, 10);PDF_setcolor($mypdf, "stroke", "rgb", 1, 0, 0);PDF_show_xy($mypdf, "Times-Roman; Page one", 50, 800);PDF_end_page($mypdf);PDF_begin_page($mypdf, 595, 842);$myfont = PDF_findfont($mypdf, "Times-Roman", "host", 0);PDF_setfont($mypdf, $myfont, 12);PDF_setcolor($mypdf, "stroke", "rgb", 1, 0, 0);PDF_show_xy($mypdf, "Times-Roman; Page two", 50, 800);PDF_end_page($mypdf);PDF_begin_page($mypdf, 595, 842);$myfont = PDF_findfont($mypdf, "Times-Roman", "host", 0);PDF_setfont($mypdf, $myfont, 14);PDF_setcolor($mypdf, "stroke", "rgb", 1, 0, 0);PDF_show_xy($mypdf, "Times-Roman; Page three", 50, 800);PDF_end_page($mypdf);PDF_close($mypdf);$mybuf = PDF_get_buffer($mypdf);$mylen = strlen($mybuf);header("Content-type: application/pdf");header("Content-Length: $mylen");header("Content-Disposition: inline; filename=gen10.pdf");print $mybuf;PDF_delete($mypdf);?>- Save this code as a new text file, let's name it gen10.php.- Upload it to your web site. - Browse to your gen10.php file on your web site. - Check the results. Enough simple text PDF pages. Ever wondered how they create these left-side "Bookmarks" and "Thumbnails" in PDF? Well we can create that with PHP too ! Let's create the "Thumbnails" first. The PHP PDF function PDF_add_thumbnail(); will create one "Thumnail" image for the PDF page that we are creating. So, this function must be called for every PDF page. When the Adobe Acrobat Reader presents the PDF file, your surfer will be able to click on one of the "Thumbnail" images, and the Adobe Acrobat Reader will then jump to the appropriate PDF page. Furthermore, we will use the PHP PDF function PDF_set_parameter($mypdf, "openmode", "thumbnails"); to instruct the Adobe Acrobat Reader to show the "Thumbnail" tab when the PDF file is opened. Here is the complete code: <?php$mypdf = PDF_new();PDF_open_file($mypdf, "");PDF_set_info($mypdf, "Creator", "gen11.php");PDF_set_info($mypdf, "Author", "John");PDF_set_info($mypdf, "Title", "Prototype (PHP)");PDF_set_info($mypdf, "Subject", "Sample");PDF_set_info($mypdf, "Keywords", "PHP PDF PDFlib");PDF_set_parameter($mypdf, "openmode", "thumbnails");PDF_set_parameter($mypdf, "openaction", "fitpage");$myfont = PDF_findfont($mypdf, "Times-Roman", "host", 0);PDF_begin_page($mypdf, 595, 842);PDF_setfont($mypdf, $myfont, 10);PDF_show_xy($mypdf, "Page one", 50, 800);$myimage = PDF_open_image_file($mypdf, "jpeg", "board1.jpg");PDF_place_image($mypdf, $myimage, 90, 750, 0.5);PDF_place_image($mypdf, $myimage, 90, 650, 0.5);PDF_place_image($mypdf, $myimage, 90, 550, 0.5);PDF_place_image($mypdf, $myimage, 90, 450, 0.5);PDF_place_image($mypdf, $myimage, 90, 350, 0.5);PDF_place_image($mypdf, $myimage, 90, 250, 0.5);PDF_place_image($mypdf, $myimage, 190, 750, 0.5);PDF_place_image($mypdf, $myimage, 190, 650, 0.5);PDF_place_image($mypdf, $myimage, 190, 550, 0.5);PDF_place_image($mypdf, $myimage, 190, 450, 0.5);PDF_place_image($mypdf, $myimage, 190, 350, 0.5);PDF_place_image($mypdf, $myimage, 190, 250, 0.5);PDF_place_image($mypdf, $myimage, 290, 750, 0.5);PDF_place_image($mypdf, $myimage, 290, 650, 0.5);PDF_place_image($mypdf, $myimage, 290, 550, 0.5);PDF_place_image($mypdf, $myimage, 290, 450, 0.5);PDF_place_image($mypdf, $myimage, 290, 350, 0.5);PDF_place_image($mypdf, $myimage, 290, 250, 0.5);PDF_place_image($mypdf, $myimage, 390, 750, 0.5);PDF_place_image($mypdf, $myimage, 390, 650, 0.5);PDF_place_image($mypdf, $myimage, 390, 550, 0.5);PDF_place_image($mypdf, $myimage, 390, 450, 0.5);PDF_place_image($mypdf, $myimage, 390, 350, 0.5);PDF_place_image($mypdf, $myimage, 390, 250, 0.5);PDF_add_thumbnail($mypdf,$myimage);PDF_end_page($mypdf);PDF_begin_page($mypdf, 595, 842);PDF_setfont($mypdf, $myfont, 10);PDF_show_xy($mypdf, "Page two", 50, 800);$myimage = PDF_open_image_file($mypdf, "jpeg", "board2.jpg");PDF_place_image($mypdf, $myimage, 90, 750, 0.5);PDF_place_image($mypdf, $myimage, 90, 650, 0.5);PDF_place_image($mypdf, $myimage, 90, 550, 0.5);PDF_place_image($mypdf, $myimage, 90, 450, 0.5);PDF_place_image($mypdf, $myimage, 90, 350, 0.5);PDF_place_image($mypdf, $myimage, 90, 250, 0.5);PDF_place_image($mypdf, $myimage, 190, 750, 0.5);PDF_place_image($mypdf, $myimage, 190, 650, 0.5);PDF_place_image($mypdf, $myimage, 190, 550, 0.5);PDF_place_image($mypdf, $myimage, 190, 450, 0.5);PDF_place_image($mypdf, $myimage, 190, 350, 0.5);PDF_place_image($mypdf, $myimage, 190, 250, 0.5);PDF_place_image($mypdf, $myimage, 290, 750, 0.5);PDF_place_image($mypdf, $myimage, 290, 650, 0.5);PDF_place_image($mypdf, $myimage, 290, 550, 0.5);PDF_place_image($mypdf, $myimage, 290, 450, 0.5);PDF_place_image($mypdf, $myimage, 290, 350, 0.5);PDF_place_image($mypdf, $myimage, 290, 250, 0.5);PDF_place_image($mypdf, $myimage, 390, 750, 0.5);PDF_place_image($mypdf, $myimage, 390, 650, 0.5);PDF_place_image($mypdf, $myimage, 390, 550, 0.5);PDF_place_image($mypdf, $myimage, 390, 450, 0.5);PDF_place_image($mypdf, $myimage, 390, 350, 0.5);PDF_place_image($mypdf, $myimage, 390, 250, 0.5);PDF_add_thumbnail($mypdf,$myimage);PDF_end_page($mypdf);PDF_begin_page($mypdf, 595, 842);PDF_setfont($mypdf, $myfont, 10);PDF_show_xy($mypdf, "Page three", 50, 800);$myimage = PDF_open_image_file($mypdf, "jpeg", "board3.jpg");PDF_place_image($mypdf, $myimage, 90, 750, 0.5);PDF_place_image($mypdf, $myimage, 90, 650, 0.5);PDF_place_image($mypdf, $myimage, 90, 550, 0.5);PDF_place_image($mypdf, $myimage, 90, 450, 0.5);PDF_place_image($mypdf, $myimage, 90, 350, 0.5);PDF_place_image($mypdf, $myimage, 90, 250, 0.5);PDF_place_image($mypdf, $myimage, 190, 750, 0.5);PDF_place_image($mypdf, $myimage, 190, 650, 0.5);PDF_place_image($mypdf, $myimage, 190, 550, 0.5);PDF_place_image($mypdf, $myimage, 190, 450, 0.5);PDF_place_image($mypdf, $myimage, 190, 350, 0.5);PDF_place_image($mypdf, $myimage, 190, 250, 0.5);PDF_place_image($mypdf, $myimage, 290, 750, 0.5);PDF_place_image($mypdf, $myimage, 290, 650, 0.5);PDF_place_image($mypdf, $myimage, 290, 550, 0.5);PDF_place_image($mypdf, $myimage, 290, 450, 0.5);PDF_place_image($mypdf, $myimage, 290, 350, 0.5);PDF_place_image($mypdf, $myimage, 290, 250, 0.5);PDF_place_image($mypdf, $myimage, 390, 750, 0.5);PDF_place_image($mypdf, $myimage, 390, 650, 0.5);PDF_place_image($mypdf, $myimage, 390, 550, 0.5);PDF_place_image($mypdf, $myimage, 390, 450, 0.5);PDF_place_image($mypdf, $myimage, 390, 350, 0.5);PDF_place_image($mypdf, $myimage, 390, 250, 0.5);PDF_add_thumbnail($mypdf,$myimage);PDF_end_page($mypdf);PDF_begin_page($mypdf, 595, 842);PDF_setfont($mypdf, $myfont, 10);PDF_show_xy($mypdf, "Page four", 50, 800);$myimage = PDF_open_image_file($mypdf, "jpeg", "board4.jpg");PDF_place_image($mypdf, $myimage, 90, 750, 0.5);PDF_place_image($mypdf, $myimage, 90, 650, 0.5);PDF_place_image($mypdf, $myimage, 90, 550, 0.5);PDF_place_image($mypdf, $myimage, 90, 450, 0.5);PDF_place_image($mypdf, $myimage, 90, 350, 0.5);PDF_place_image($mypdf, $myimage, 90, 250, 0.5);PDF_place_image($mypdf, $myimage, 190, 750, 0.5);PDF_place_image($mypdf, $myimage, 190, 650, 0.5);PDF_place_image($mypdf, $myimage, 190, 550, 0.5);PDF_place_image($mypdf, $myimage, 190, 450, 0.5);PDF_place_image($mypdf, $myimage, 190, 350, 0.5);PDF_place_image($mypdf, $myimage, 190, 250, 0.5);PDF_place_image($mypdf, $myimage, 290, 750, 0.5);PDF_place_image($mypdf, $myimage, 290, 650, 0.5);PDF_place_image($mypdf, $myimage, 290, 550, 0.5);PDF_place_image($mypdf, $myimage, 290, 450, 0.5);PDF_place_image($mypdf, $myimage, 290, 350, 0.5);PDF_place_image($mypdf, $myimage, 290, 250, 0.5);PDF_place_image($mypdf, $myimage, 390, 750, 0.5);PDF_place_image($mypdf, $myimage, 390, 650, 0.5);PDF_place_image($mypdf, $myimage, 390, 550, 0.5);PDF_place_image($mypdf, $myimage, 390, 450, 0.5);PDF_place_image($mypdf, $myimage, 390, 350, 0.5);PDF_place_image($mypdf, $myimage, 390, 250, 0.5);PDF_add_thumbnail($mypdf,$myimage);PDF_end_page($mypdf);PDF_begin_page($mypdf, 595, 842);PDF_setfont($mypdf, $myfont, 10);PDF_show_xy($mypdf, "Page five", 50, 800);$myimage = PDF_open_image_file($mypdf, "jpeg", "board5.jpg");PDF_place_image($mypdf, $myimage, 90, 750, 0.5);PDF_place_image($mypdf, $myimage, 90, 650, 0.5);PDF_place_image($mypdf, $myimage, 90, 550, 0.5);PDF_place_image($mypdf, $myimage, 90, 450, 0.5);PDF_place_image($mypdf, $myimage, 90, 350, 0.5);PDF_place_image($mypdf, $myimage, 90, 250, 0.5);PDF_place_image($mypdf, $myimage, 190, 750, 0.5);PDF_place_image($mypdf, $myimage, 190, 650, 0.5);PDF_place_image($mypdf, $myimage, 190, 550, 0.5);PDF_place_image($mypdf, $myimage, 190, 450, 0.5);PDF_place_image($mypdf, $myimage, 190, 350, 0.5);PDF_place_image($mypdf, $myimage, 190, 250, 0.5);PDF_place_image($mypdf, $myimage, 290, 750, 0.5);PDF_place_image($mypdf, $myimage, 290, 650, 0.5);PDF_place_image($mypdf, $myimage, 290, 550, 0.5);PDF_place_image($mypdf, $myimage, 290, 450, 0.5);PDF_place_image($mypdf, $myimage, 290, 350, 0.5);PDF_place_image($mypdf, $myimage, 290, 250, 0.5);PDF_place_image($mypdf, $myimage, 390, 750, 0.5);PDF_place_image($mypdf, $myimage, 390, 650, 0.5);PDF_place_image($mypdf, $myimage, 390, 550, 0.5);PDF_place_image($mypdf, $myimage, 390, 450, 0.5);PDF_place_image($mypdf, $myimage, 390, 350, 0.5);PDF_place_image($mypdf, $myimage, 390, 250, 0.5);PDF_add_thumbnail($mypdf,$myimage);PDF_end_page($mypdf);PDF_begin_page($mypdf, 595, 842);PDF_setfont($mypdf, $myfont, 10);PDF_show_xy($mypdf, "Page six", 50, 800);$myimage = PDF_open_image_file($mypdf, "jpeg", "board6.jpg");PDF_place_image($mypdf, $myimage, 90, 750, 0.5);PDF_place_image($mypdf, $myimage, 90, 650, 0.5);PDF_place_image($mypdf, $myimage, 90, 550, 0.5);PDF_place_image($mypdf, $myimage, 90, 450, 0.5);PDF_place_image($mypdf, $myimage, 90, 350, 0.5);PDF_place_image($mypdf, $myimage, 90, 250, 0.5);PDF_place_image($mypdf, $myimage, 190, 750, 0.5);PDF_place_image($mypdf, $myimage, 190, 650, 0.5);PDF_place_image($mypdf, $myimage, 190, 550, 0.5);PDF_place_image($mypdf, $myimage, 190, 450, 0.5);PDF_place_image($mypdf, $myimage, 190, 350, 0.5);PDF_place_image($mypdf, $myimage, 190, 250, 0.5);PDF_place_image($mypdf, $myimage, 290, 750, 0.5);PDF_place_image($mypdf, $myimage, 290, 650, 0.5);PDF_place_image($mypdf, $myimage, 290, 550, 0.5);PDF_place_image($mypdf, $myimage, 290, 450, 0.5);PDF_place_image($mypdf, $myimage, 290, 350, 0.5);PDF_place_image($mypdf, $myimage, 290, 250, 0.5);PDF_place_image($mypdf, $myimage, 390, 750, 0.5);PDF_place_image($mypdf, $myimage, 390, 650, 0.5);PDF_place_image($mypdf, $myimage, 390, 550, 0.5);PDF_place_image($mypdf, $myimage, 390, 450, 0.5);PDF_place_image($mypdf, $myimage, 390, 350, 0.5);PDF_place_image($mypdf, $myimage, 390, 250, 0.5);PDF_add_thumbnail($mypdf,$myimage);PDF_end_page($mypdf);PDF_begin_page($mypdf, 595, 842);PDF_setfont($mypdf, $myfont, 10);PDF_show_xy($mypdf, "Page seven", 50, 800);$myimage = PDF_open_image_file($mypdf, "jpeg", "board7.jpg");PDF_place_image($mypdf, $myimage, 90, 750, 0.5);PDF_place_image($mypdf, $myimage, 90, 650, 0.5);PDF_place_image($mypdf, $myimage, 90, 550, 0.5);PDF_place_image($mypdf, $myimage, 90, 450, 0.5);PDF_place_image($mypdf, $myimage, 90, 350, 0.5);PDF_place_image($mypdf, $myimage, 90, 250, 0.5);PDF_place_image($mypdf, $myimage, 190, 750, 0.5);PDF_place_image($mypdf, $myimage, 190, 650, 0.5);PDF_place_image($mypdf, $myimage, 190, 550, 0.5);PDF_place_image($mypdf, $myimage, 190, 450, 0.5);PDF_place_image($mypdf, $myimage, 190, 350, 0.5);PDF_place_image($mypdf, $myimage, 190, 250, 0.5);PDF_place_image($mypdf, $myimage, 290, 750, 0.5);PDF_place_image($mypdf, $myimage, 290, 650, 0.5);PDF_place_image($mypdf, $myimage, 290, 550, 0.5);PDF_place_image($mypdf, $myimage, 290, 450, 0.5);PDF_place_image($mypdf, $myimage, 290, 350, 0.5);PDF_place_image($mypdf, $myimage, 290, 250, 0.5);PDF_place_image($mypdf, $myimage, 390, 750, 0.5);PDF_place_image($mypdf, $myimage, 390, 650, 0.5);PDF_place_image($mypdf, $myimage, 390, 550, 0.5);PDF_place_image($mypdf, $myimage, 390, 450, 0.5);PDF_place_image($mypdf, $myimage, 390, 350, 0.5);PDF_place_image($mypdf, $myimage, 390, 250, 0.5);PDF_add_thumbnail($mypdf,$myimage);PDF_end_page($mypdf);PDF_close($mypdf);$mybuf = PDF_get_buffer($mypdf);$mylen = strlen($mybuf);header("Content-type: application/pdf");header("Content-Length: $mylen");header("Content-Disposition: inline; filename=gen11.pdf");print $mybuf;PDF_delete($mypdf);?>- Save this code as a new text file, let's name it gen11.php.- Upload it to your web site. - Browse to your gen11.php file on your web site. - Check the results. Of course you will have to upload the jpeg images also !!!!! And now is the time to create PDF bookmarks. The PHP PDF function PDF_add_bookmark(); will create one "Bookmark" for the PDF page that we are creating. So, this function must be called for every PDF page. When the Adobe Acrobat Reader presents the PDF file, your surfer will be able to click on one of the "Bookmark" lines, and the Adobe Acrobat Reader will then jump to the appropriate PDF page. Furthermore, we will use the PHP PDF function PDF_set_parameter($mypdf, "openmode", "bookmarks"); to instruct the Adobe Acrobat Reader to show the "Bookmarks" tab when the PDF file is opened. What is so special about the PDF bookmarks implementation, is the fact that the PDF bookmarks are "hierarchical". This means that the PDF bookmarks view is like a tree view, with different levels. Furthermore, some of these levels are visible, while other levels are invisible, or "collapsed". The PHP PDF function PDF_add_bookmark(); takes the required parameters to obtain these results. - The first parameter is the PHP PDF object. - The second parameter is the text of the bookmark. - The third parameter is the level (0 for the highest level) - The fourth parameter is 1 for children-are-visible, and 0 for children-are-invisible (collapsed). Sample: $mytopparent = PDF_add_bookmark($mypdf, "First page", 0, 1);$mychild = PDF_add_bookmark($mypdf, "Second page", $mytopparent, 1);$mytopparent = PDF_add_bookmark($mypdf, "Third page", 0, 1);$mychild = PDF_add_bookmark($mypdf, "Fourth page", $mytopparent, 0);$mygrandchild = PDF_add_bookmark($mypdf, "Fifth page", $mychild, 1);$mygrandchild = PDF_add_bookmark($mypdf, "Sixth page", $mychild, 1);$mytopparent = PDF_add_bookmark($mypdf, "Seventh page", 0, 1); Here is the complete code: <?php$mypdf = PDF_new();PDF_open_file($mypdf, "");PDF_set_info($mypdf, "Creator", "gen12.php");PDF_set_info($mypdf, "Author", "John");PDF_set_info($mypdf, "Title", "Prototype (PHP)");PDF_set_info($mypdf, "Subject", "Sample");PDF_set_info($mypdf, "Keywords", "PHP PDF PDFlib");PDF_set_parameter($mypdf, "openmode", "bookmarks");$myfont = PDF_findfont($mypdf, "Times-Roman", "host", 0);PDF_begin_page($mypdf, 595, 842);PDF_setfont($mypdf, $myfont, 10);PDF_show_xy($mypdf, "Page one", 50, 800);$mytopparent = PDF_add_bookmark($mypdf, "First page", 0, 1);PDF_end_page($mypdf);PDF_begin_page($mypdf, 595, 842);PDF_setfont($mypdf, $myfont, 10);PDF_show_xy($mypdf, "Page two", 50, 800);$mychild = PDF_add_bookmark($mypdf, "Second page", $mytopparent, 1);PDF_end_page($mypdf);PDF_begin_page($mypdf, 595, 842);PDF_setfont($mypdf, $myfont, 10);PDF_show_xy($mypdf, "Page three", 50, 800);$mytopparent = PDF_add_bookmark($mypdf, "Third page", 0, 1);PDF_end_page($mypdf);PDF_begin_page($mypdf, 595, 842);PDF_setfont($mypdf, $myfont, 10);PDF_show_xy($mypdf, "Page four", 50, 800);$mychild = PDF_add_bookmark($mypdf, "Fourth page", $mytopparent, 0);PDF_end_page($mypdf);PDF_begin_page($mypdf, 595, 842);PDF_setfont($mypdf, $myfont, 10);PDF_show_xy($mypdf, "Page five", 50, 800);$mygrandchild = PDF_add_bookmark($mypdf, "Fifth page", $mychild, 1);PDF_end_page($mypdf);PDF_begin_page($mypdf, 595, 842);PDF_setfont($mypdf, $myfont, 10);PDF_show_xy($mypdf, "Page six", 50, 800);$mygrandchild = PDF_add_bookmark($mypdf, "Sixth page", $mychild, 1);PDF_end_page($mypdf);PDF_begin_page($mypdf, 595, 842);PDF_setfont($mypdf, $myfont, 10);PDF_show_xy($mypdf, "Page seven", 50, 800);$mytopparent = PDF_add_bookmark($mypdf, "Seventh page", 0, 1);PDF_end_page($mypdf);PDF_close($mypdf);$mybuf = PDF_get_buffer($mypdf);$mylen = strlen($mybuf);header("Content-type: application/pdf");header("Content-Length: $mylen");header("Content-Disposition: inline; filename=gen12.pdf");print $mybuf;PDF_delete($mypdf);?>- Save this code as a new text file, let's name it gen12.php.- Upload it to your web site. - Browse to your gen12.php file on your web site. - Check the results. How about some Powerpoint Presentation Effects ? The Adobe Acrobat Reader is capable of showing some nifty page-transition effects. We will use PHP to add the instructions required for these effects into our PDF file. The PHP PDF function PDF_set_parameter($mypdf, "transition", $effect); will be used. Here is the complete code: <?php$mypdf = PDF_new();PDF_open_file($mypdf, "");PDF_set_info($mypdf, "Creator", "gen13.php");PDF_set_info($mypdf, "Author", "John");PDF_set_info($mypdf, "Title", "Prototype (PHP)");PDF_set_info($mypdf, "Subject", "Sample");PDF_set_info($mypdf, "Keywords", "PHP PDF PDFlib");$myfont = PDF_findfont($mypdf, "Times-Roman", "host", 0);PDF_begin_page($mypdf, 595, 842);PDF_setfont($mypdf, $myfont, 10);PDF_show_xy($mypdf, "Demonstration of PDF effects. Please Paginate.", 50, 800);PDF_set_parameter($mypdf, "openaction", "fitpage");PDF_end_page($mypdf);PDF_begin_page($mypdf, 595, 842);PDF_setfont($mypdf, $myfont, 10);PDF_show_xy($mypdf, "Page one", 50, 800);for ($y=0; $y<=66; $y++) { PDF_continue_text($mypdf, "66 lines. ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789");}PDF_set_parameter($mypdf, "transition", "split");PDF_end_page($mypdf);PDF_begin_page($mypdf, 595, 842);PDF_setfont($mypdf, $myfont, 10);PDF_show_xy($mypdf, "Page two", 50, 800);PDF_end_page($mypdf);PDF_begin_page($mypdf, 595, 842);PDF_setfont($mypdf, $myfont, 10);PDF_show_xy($mypdf, "Page three", 50, 800);for ($y=0; $y<=66; $y++) { PDF_continue_text($mypdf, "66 lines. ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789");}PDF_set_parameter($mypdf, "transition", "blinds");PDF_end_page($mypdf);PDF_begin_page($mypdf, 595, 842);PDF_setfont($mypdf, $myfont, 10);PDF_show_xy($mypdf, "Page four", 50, 800);PDF_end_page($mypdf);PDF_begin_page($mypdf, 595, 842);PDF_setfont($mypdf, $myfont, 10);PDF_show_xy($mypdf, "Page five", 50, 800);for ($y=0; $y<=66; $y++) { PDF_continue_text($mypdf, "66 lines. ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789");}PDF_set_parameter($mypdf, "transition", "box");PDF_end_page($mypdf);PDF_begin_page($mypdf, 595, 842);PDF_setfont($mypdf, $myfont, 10);PDF_show_xy($mypdf, "Page six", 50, 800);PDF_end_page($mypdf);PDF_begin_page($mypdf, 595, 842);PDF_setfont($mypdf, $myfont, 10);PDF_show_xy($mypdf, "Page seven", 50, 800);for ($y=0; $y<=66; $y++) { PDF_continue_text($mypdf, "66 lines. ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789");}PDF_set_parameter($mypdf, "transition", "wipe");PDF_end_page($mypdf);PDF_begin_page($mypdf, 595, 842);PDF_setfont($mypdf, $myfont, 10);PDF_show_xy($mypdf, "Page eight", 50, 800);PDF_end_page($mypdf);PDF_begin_page($mypdf, 595, 842);PDF_setfont($mypdf, $myfont, 10);PDF_show_xy($mypdf, "Page nine", 50, 800);for ($y=0; $y<=66; $y++) { PDF_continue_text($mypdf, "66 lines. ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789");}PDF_set_parameter($mypdf, "transition", "dissolve");PDF_set_value($mypdf, "duration", 3);PDF_end_page($mypdf);PDF_begin_page($mypdf, 595, 842);PDF_setfont($mypdf, $myfont, 10);PDF_show_xy($mypdf, "Page ten", 50, 800);PDF_end_page($mypdf);PDF_begin_page($mypdf, 595, 842);PDF_setfont($mypdf, $myfont, 10);PDF_show_xy($mypdf, "Page eleven", 50, 800);for ($y=0; $y<=66; $y++) { PDF_continue_text($mypdf, "66 lines. ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789");}PDF_set_parameter($mypdf, "transition", "glitter");PDF_set_value($mypdf, "duration", 3);PDF_end_page($mypdf);PDF_begin_page($mypdf, 595, 842);PDF_setfont($mypdf, $myfont, 10);PDF_show_xy($mypdf, "Page twelve", 50, 800);PDF_end_page($mypdf);PDF_begin_page($mypdf, 595, 842);PDF_setfont($mypdf, $myfont, 10);PDF_show_xy($mypdf, "Page thirteen", 50, 800);for ($y=0; $y<=66; $y++) { PDF_continue_text($mypdf, "66 lines. ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789");}PDF_set_parameter($mypdf, "transition", "replace");PDF_end_page($mypdf);PDF_close($mypdf);$mybuf = PDF_get_buffer($mypdf);$mylen = strlen($mybuf);header("Content-type: application/pdf");header("Content-Length: $mylen");header("Content-Disposition: inline; filename=gen13.pdf");print $mybuf;PDF_delete($mypdf);?>- Save this code as a new text file, let's name it gen13.php.- Upload it to your web site. - Browse to your gen13.php file on your web site. - Check the results. And one more particularity of the PDF file, is the possibility to add "notes". The PHP PDF function PDF_add_note(); will be used. When the Adobe Acrobat Reader presents the PDF file to your surfer, he/she will have the possibility to open the note by double-clicking on the little note icon, placed somewhere in the text of the PDF page. Here is the complete code: <?php$mypdf = PDF_new();PDF_open_file($mypdf, "");PDF_set_info($mypdf, "Creator", "gen14.php");PDF_set_info($mypdf, "Author", "John");PDF_set_info($mypdf, "Title", "Prototype (PHP)");PDF_set_info($mypdf, "Subject", "Sample");PDF_set_info($mypdf, "Keywords", "PHP PDF PDFlib");$myfont = PDF_findfont($mypdf, "Times-Roman", "host", 0);PDF_begin_page($mypdf, 595, 842);PDF_setfont($mypdf, $myfont, 10);PDF_show_xy($mypdf, "When we look more closely at this way of working,", 50, 750);PDF_add_note($mypdf, 245, 660, 550, 770, "Yes, what is going to happen here? We are not used to work that way, and now this statement stares at us ! ", "What do you mean, WORK ?", "note", 0);PDF_show_xy($mypdf, "we can clearly see the more open side of this project.", 50, 730);PDF_end_page($mypdf);PDF_close($mypdf);$mybuf = PDF_get_buffer($mypdf);$mylen = s
-
Intro Effect And Exit Effect In Html
signatureimage replied to iGuest's topic in Websites and Web Designing
Dear ejasoft, (1) First, there are double-quotes missing in the sample code Your code: <META http-equiv="Page-Enter" CONTENT="RevealTrans(Duration=4,Transition=1)>the exit effect <META http-equiv="Page-Exit" CONTENT="RevealTrans(Duration=2.500,Transition=6)> Correct code: <META http-equiv="Page-Enter" CONTENT="RevealTrans(Duration=4,Transition=1)">the exit effect <META http-equiv="Page-Exit" CONTENT="RevealTrans(Duration=2.500,Transition=6)"> (2) Second, this only works in the Microsoft Internet Explorer browser... -
Very nice, overture. .jump { font-family: Verdana, Geneva, Arial, Helvetica, sans-serif; background-color: #dddddd; color: #505050; font-size: 10px; width: 130px;} What I have experienced with different browsers, on different OS'es, is the way they handle the font-size. Therefore, I now use the "point" instead of the "pixel" notation. May I propose font-size:10pt;This works more uniformly on different browsers. While we are on the subject of user-experience, perhaps we should also adapt the "width" specification. Especially when our surfer decides to change the text size with the "view" menu of his/her browser. Let's make shure that the dropdown menu always shows at least 13 characters. So instead of width:130px; which is good when the font is font-size:10px; - let's specify width:13em; which is 13 characters, regardless of font size... Happy CSS'ing !
-
Dear soleimanian, Your problem description was interpreted by me, and I came up with the following proposal: <html><head><title>News Page</title></head><body><form><select onchange="location.href=this.option[this.selectedIndex].value" size="1"><option value="#">latest news:</option><option value="page20050503.html">May 3, 2005</option><option value="page20050502.html">May 2, 2005</option><option value="page20050501.html">May 1, 2005</option><option value="page20050430.html">April 30 2005</option><option value="page20050429.html">April 29 2005</option><option value="page20050428.html">April 28 2005</option><option value="page20050427.html">April 27 2005</option><option value="page20050426.html">April 26 2005</option><option value="page20050425.html">April 25 2005</option><option value="page20050424.html">April 24 2005</option><option value="page20050423.html">April 23 2005</option><option value="page20050422.html">April 22 2005</option><option value="page20050421.html">April 21 2005</option><option value="page20050420.html">April 20 2005</option><option value="page20050419.html">April 19 2005</option><option value="page20050418.html">April 18 2005</option><option value="page20050417.html">April 17 2005</option><option value="page20050416.html">April 16 2005</option><option value="page20050415.html">April 15 2005</option><option value="page20050414.html">April 14 2005</option><option value="page20050413.html">April 13 2005</option><option value="page20050412.html">April 12 2005</option><option value="page20050411.html">April 11 2005</option><option value="page20050410.html">April 10 2005</option><option value="page20050409.html">April 9 2005</option><option value="page20050408.html">April 8 2005</option><option value="page20050407.html">April 7 2005</option><option value="page20050406.html">April 6 2005</option><option value="page20050405.html">April 5 2005</option><option value="page20050404.html">April 4 2005</option><option value="page20050403.html">April 3 2005</option><option value="page20050402.html">April 2 2005</option><option value="page20050401.html">April 1 2005</option></select></form></body></html> Of course, when you use PHP, you can dynamically make the page, perhaps something like this proposal: <html><head><title>News Page</title></head><body><form><select onchange="location.href=this.option[this.selectedIndex].value" size="1"><option value="#">latest news:</option><option value="page.php?date=20050503">May 3, 2005</option><option value="page.php?date=20050502">May 2, 2005</option><option value="page.php?date=20050501">May 1, 2005</option><option value="page.php?date=20050430">April 30 2005</option><option value="page.php?date=20050429">April 29 2005</option><option value="page.php?date=20050428">April 28 2005</option><option value="page.php?date=20050427">April 27 2005</option><option value="page.php?date=20050426">April 26 2005</option><option value="page.php?date=20050425">April 25 2005</option><option value="page.php?date=20050424">April 24 2005</option><option value="page.php?date=20050423">April 23 2005</option><option value="page.php?date=20050422">April 22 2005</option><option value="page.php?date=20050421">April 21 2005</option><option value="page.php?date=20050420">April 20 2005</option><option value="page.php?date=20050419">April 19 2005</option><option value="page.php?date=20050418">April 18 2005</option><option value="page.php?date=20050417">April 17 2005</option><option value="page.php?date=20050416">April 16 2005</option><option value="page.php?date=20050415">April 15 2005</option><option value="page.php?date=20050414">April 14 2005</option><option value="page.php?date=20050413">April 13 2005</option><option value="page.php?date=20050412">April 12 2005</option><option value="page.php?date=20050411">April 11 2005</option><option value="page.php?date=20050410">April 10 2005</option><option value="page.php?date=20050409">April 9 2005</option><option value="page.php?date=20050408">April 8 2005</option><option value="page.php?date=20050407">April 7 2005</option><option value="page.php?date=20050406">April 6 2005</option><option value="page.php?date=20050405">April 5 2005</option><option value="page.php?date=20050404">April 4 2005</option><option value="page.php?date=20050403">April 3 2005</option><option value="page.php?date=20050402">April 2 2005</option><option value="page.php?date=20050401">April 1 2005</option></select></form></body></html>
-
Dear ebbinger_413,You talked about "wine".This is the Linux application that is neededto run programs written for Microsoft Windows.What this "wine" does, is offer the API's(Application-Program-Interface) for Windows32.So, when the original program calls a functionthat resides inside one of the MS Windows DLL's(Dynamic-Load-Libraries) the "wine" equivalentof these DLL's takes over, and translates theoriginal code to the one that the Linux environmentoffer.Concerning games however, the majority of theAPI's that are used by games written for theMicrosoft Windows platform, use the DirectX API's,which were written by Microsoft specifically forthe games industry. The "wine" application is notwritten to translate the DirectX API's. You will need"TransGaming WineX" for games.This is not free, however...
-
Dear overture, You asked for the code: //---------------------------------------------------------------------------------------------------------------------------------------------------// Called by F_Create_Folder()function F_Make_Folder($P_foldername) { if (file_exists('../'.$P_foldername)) { return "OK"; } else { if ($rc = mkdir('../'.$P_foldername)) { return "OK"; } else { return "KO"; } } }//--------------------------------------------------------------------------------------------------------------------------------------------------- Note: the if ($rc = mkdir('../'.$P_foldername)) is not a comparison, it is an assignment, whose boolean value is tested. Therefore, only one equal sign is used... Dear overture, Your suggestion to modify the permissions to 777 will temporarily be tried.
-
My PHP script, running on Xisto.com,calls the PHP function mkdir() to create a new directory.This works on my home development-server on Microsoft Windows with Apache.This works on my home test-server on Linux Fedora with Apache.This does NOT work on Xisto.com: "Permission denied".According to the CPanel File Manager, the properties ofthe parent directory are: permissions 755:User: Read-Write-ExecuteGroup: Read-ExecuteWorld: Read-ExecuteAny idea, administrators?
-
Yes, you are right.Using HTML TABLEs for lay-out is now considered bad style.Yes, you are right.Getting the same results using CSS is much more of a hassle.At least when confronted with it for the first time.But, as always, as more and more people do it, as more and more programs generate it,the use of HTML TABLEs will drop for lay-out andwill continue to be used for what they were invented for:the presentation of tabular data.In my opinion, CSS is the way to go.Personally, I still have troubles with them,so any help that I can find is welcome...
-
Oldest Intel PC: IBM XT 8086 - 512 Kilo ram - 30 Mega harddisk Runs the first version of Microsoft Windows (v1.02) - very slowly by actual standards! Oldest 8-bit machine: Apple ][ - 48 Kilo ram - 100 Kilo floppies Runs the first version of Microsoft Flight Simulator (v1) - scenery drawn with lines - no full rendering on a 1 MHz processor! Runs the very first spreadsheet application: VisiCalc Download the PC version from the author's website
-
On a suggestion from M^E, I went to the site of Andreas Norman at subzane.com in Sweden. A number of PHP applications can be downloaded from that site. One of the applications was of interest to M^E, so I selected the SZProjectManager. Simply unzipping the SZProjectMngr.zip into its own folder on the Apache web-structure was not sufficient to run the application. A second visit to subzane.com was needed to obtain the packages: - MySQLHandler - BlobHandler - ImageHandler These packages are the "helper" packages, that offer a number of additional PHP functions, needed by the SZProjectManager application. Here are the steps needed to prepare the four packages: (1) First, from the package MySQLHandler, modifications are to be done in the PHP file: MySQLHandler.class.php This file contains the code that prepares a number of PHP variables, with the values that are specific for a given installation. We are talking here about the 4 values that need to be set in every application that makes use of a mySQL database: $SERVER = "__";$USERNAME = "__";$PASSWORD = "__";$DATABASE = "__"; So, I changed the 4 values to the ones that are specific for my home test-server. (2) Next, I noticed that the packages BlobHandler and ImageHandler both came with a PHP file: header.inc.php Because the content was different, but the usage of the files were similar, I opted to merge the content of both files, and store the combined result on the Apache web-structure. <?require('MySQLHandler.class.php');require('BlobHandler.class.php');require('ImageHandler.class.php');$sql = new MySQLHandler();$sql->Init();$BlobHandler = new BlobHandler($sql);$ImageHandler = new ImageHandler($sql);//----------------------------------------------// 2005/04/24 MERGED:// BlobHandler/header.inc.php// ImageHandler/header.inc.php//----------------------------------------------?> (3) Now it was time to investigate the options of my home test-server PHP installation. Some versions of PHP - even some sub-versions - differ in the handling of PHP statements and functions. For example, the SZProjectMngr applications makes use of the PHP function file_get_contents() which is a PHP 4.3.0 function. So, it was time to update my home test-server PHP compiler. (4) Another option that is specific to a given PHP installation is the register globals setting. For those not familiar with PHP register globals, here is a short intriduction: When the PHP option register globals has the value ON, all the HTML FORM variables that arrive from the surfer's browser - via HTTP - in the PHP engine, will automatically become PHP variables, immediately available for your PHP code. Easy, but also dangerous. So, the latest default setting is: register globals OFF What does this mean? Well, your PHP now needs to specifically request the content of the HTML FORM variables by using the $_GET['varname'] or the $_POST['varname'] constructions. A more general modification of older PHP code can be obtained with the quicker extract($_GET) or extract($_POST) function. This is what I inserted into each PHP program from subzane. (5) So, after the inspection of the downloaded PHP code, it was time to look at the mySQL database definitions. The SQL to create the tables that are required by the SZProjectMngr application, and the tables that are required by the 3 helper packages were provided in the download. So, up with the browser, and quickly surfing to the home test-server myPHPadmin pages. First we had to create a new database - whose name is to be reflected in the $DATABASE = "__"; statement of the PHP file: MySQLHandler.class.php - and I selected SZProjectMngr Then with the myPHPadmin the BlobHandler.sql , the ImageHandler.sql , and the SZProjectMngr.sql were run, which resulted in the creation of the empty mySQL tables needed by the application. The BlobHandler Table: CREATE TABLE blobs ( id bigint(14) unsigned NOT NULL auto_increment, file_name varchar(50) NOT NULL default '', data longblob NOT NULL, file_size varchar(10) NOT NULL default '', mimetype varchar(30) NOT NULL default '0', extension varchar(4) NOT NULL default '', checksum varchar(10) NOT NULL default '', PRIMARY KEY (id), KEY id (id)) TYPE=MyISAM; The ImageHandler Tables: CREATE TABLE db_images ( id bigint(14) unsigned DEFAULT '0' NOT NULL auto_increment, image longblob NOT NULL, file_size varchar(10) NOT NULL, width int(4) unsigned DEFAULT '0' NOT NULL, height int(4) unsigned DEFAULT '0' NOT NULL, extension varchar(4) NOT NULL, PRIMARY KEY (id), KEY id (id)) TYPE=MyISAM;CREATE TABLE db_thumbs ( id bigint(14) unsigned NOT NULL auto_increment, thumb longblob NOT NULL, file_size varchar(10) NOT NULL default '', width int(4) unsigned NOT NULL default '0', height int(4) unsigned NOT NULL default '0', PRIMARY KEY (id), KEY id (id)) TYPE=MyISAM;CREATE TABLE db_image_mapping ( id bigint(14) unsigned NOT NULL auto_increment, image_id bigint(14) unsigned NOT NULL default '0', thumb_id bigint(14) unsigned NOT NULL default '0', PRIMARY KEY (id), KEY image_id (image_id,thumb_id)) TYPE=MyISAM; The SZProjectMngr tables: ## Table structure for table `project_categories`#CREATE TABLE project_categories ( id int(11) unsigned NOT NULL auto_increment, category varchar(30) NOT NULL default '', description text NOT NULL, PRIMARY KEY (id)) TYPE=MyISAM;# --------------------------------------------------------## Table structure for table `project_dependencies`#CREATE TABLE project_dependencies ( project_id int(11) unsigned NOT NULL default '0', dependent_on_id int(11) unsigned NOT NULL default '0', KEY project_id (project_id,dependent_on_id)) TYPE=MyISAM;# --------------------------------------------------------## Table structure for table `project_history`#CREATE TABLE project_history ( id int(11) unsigned NOT NULL auto_increment, project_id int(11) unsigned NOT NULL default '0', version varchar(5) NOT NULL default '', body text NOT NULL, PRIMARY KEY (id), KEY project_id (project_id)) TYPE=MyISAM;# --------------------------------------------------------## Table structure for table `project_screenshots`#CREATE TABLE project_screenshots ( id int(11) unsigned NOT NULL auto_increment, image_id int(11) unsigned NOT NULL default '0', project_id int(11) unsigned NOT NULL default '0', name varchar(50) NOT NULL default '', description text NOT NULL, PRIMARY KEY (id), KEY image_id (image_id,project_id)) TYPE=MyISAM;# --------------------------------------------------------## Table structure for table `projects`#CREATE TABLE projects ( id int(11) unsigned NOT NULL auto_increment, date bigint(14) unsigned NOT NULL default '0', last_update bigint(14) unsigned NOT NULL default '0', source_blob_id int(11) unsigned NOT NULL default '0', example_blob_id int(11) unsigned NOT NULL default '0', binary_blob_id int(11) unsigned NOT NULL default '0', category_id int(11) unsigned NOT NULL default '0', name varchar(30) NOT NULL default '', short_description varchar(255) NOT NULL default '', description text NOT NULL, price varchar(50) default NULL, platforms varchar(20) NOT NULL default '', version varchar(5) NOT NULL default '', forum_topic_link varchar(255) NOT NULL default '', demo_link varchar(200) NOT NULL default '', downloads int(10) unsigned NOT NULL default '0', PRIMARY KEY (id), KEY source_blob_id (source_blob_id,example_blob_id), KEY binary_blob_id (binary_blob_id), KEY category_id (category_id)) TYPE=MyISAM; SZProjectMngr: (6) So far, so good. Time to start the SZProjectMngr application. First, an explanation. What is this SZ Project Manager? What is the target audiance? Well, there are 2 types of users for the SZ Project Manager application: First there are the people who offer the results of a project. Their web application starts with project.list.php Then there are the people who consume the results of a project. Their web application starts with page.php The first thing that has to be done by the offerers - or project owners - is the declaration of a number of project categories like Gaming, Tools, Utilities, Imaging, Office, Compression, and so. Then it is time to add the projects, - place them into one of the Categories, - specify the OS Platform it is written for, - give the Version of the project, - and upload the 3 possible project files: --- a binary file --- a source file --- a sample file As a supplement, screen-shots and history information can be added. As could be deducted from the database tables created, these project files will be stored inside the mySQL database as BLOBs (Binary Large OBjects) Evaluation: My evaluation of the SZProjectMngr package: This package is aimed at presenting to the consumers of your projects the files that can be downloaded from your web site. It is therefore not a Project Management application that can be used during the life-cycle of your project, where the persons that participate in a project collaborate together on the same repository of files that comprise a project. And this is generally more than 1 executable, 1 source and 1 sample. Besides the sources of the programs that will eventually result in a finished project, other files are to be stored, with their versions and modifications. Like project plans, project time-sheets, project meetings-notes, project test-data, project resource-estimations, and more. An application that can manage this kind of information is what M^E was looking for, I thought. SZProjectMngr is not that.
-
Help: Trying To Create Web-based Compiler W/ Php
signatureimage replied to miCRoSCoPiC^eaRthLinG's topic in Programming
Dear M^E,First a suggestion for the HTML form:The <TEXTAREA> tag can be modifiedby adding a WRAP= attribute.WRAP= specifies how to handle word-wrapping inside the text area.WRAP="OFF" --- word wrapping is disabled (the default)WRAP="PHYSICAL" --- the text is displayed and submitted word-wrapped.WRAP="VIRTUAL" --- the test is displayed word-wrapped, but is submitted as typed. -
Best Content Manager Which is the best?
signatureimage replied to spistols678's topic in Web Hosting Support
Dear madcrow,Yes, you are right: Mambo is big.Perhaps that is the reason why many consider it to be the best.... -
Best Content Manager Which is the best?
signatureimage replied to spistols678's topic in Web Hosting Support
Take a look at "MAMBO"... Very impressive! Mambo's Home -
And now for something completely cool ! When I read your post correctly, nachgeist03, you have a text nav bar. Most - if not all - web-sites have some form of navigation. But when I make a print of a web-page, what use have I of the navigation information on my printed copy? None! So, why don't we - as web-designers - remove the navigation from the printed version of our web-pages? Can that be accomplished? Yes of course, and here is how: (1) Elaborating on my previous post, we will modify the HTML that calls our common CSS file: original= <HEAD><LINK href="mycss.css" type="text/css" rel="stylesheet"></HEAD>new= <HEAD><LINK media="screen" href="mycss.css" type="text/css" rel="stylesheet"></HEAD> Did you see the new tag? This indicates that the contents of the mycss.css must be applied by the browser, only when the browser is rendering the HTML for a screen. (2) Now we will create a new text file, name it something like "myprintcss.css" and place it in some convenient place on our web-structure. Then we place some new CSS definitions inside this file: myNav {display:none;} (3) Again we modify the HTML that calls our common CSS file: original= <HEAD><LINK media="screen" href="mycss.css" type="text/css" rel="stylesheet"></HEAD>new= <HEAD><LINK media="screen" href="mycss.css" type="text/css" rel="stylesheet"><LINK media="print" href="myprintcss.css" type="text/css" rel="stylesheet"></HEAD> Did you see the new "print" tag? This indicates that the contents of the myprintcss.css must be applied by the browser, only when the browser is rendering the HTML for a printer. What will be the effect? Well, the "display:none;" definition will inhibit the rendering of our navigation on the printed copy of our web-pages. And what happens when our navigation not only contains <A> </A> tags, like in our example, but also some static text? Well we surround that static text with <SPAN> </SPAN> tags that contain a call to our myNav CLASS, and the printed copy will not show that static text. <SPAN CLASS="myNav">Navigation menu:</SPAN><BR /><A CLASS="myNav" HREF="some-link-to-some-URL1">text1-to-be-highlighted</A><BR /><A CLASS="myNav" HREF="some-link-to-some-URL2">text2-to-be-highlighted</A><BR /><A CLASS="myNav" HREF="some-link-to-some-URL3">text3-to-be-highlighted</A> Cool or what?
-
Want To Connect Computers.. For a LAN party
signatureimage replied to nakulgupta's topic in Computer Networks
Dear nakulgupta,My personal experience is: YESWhen I hear you talk about a LAN party,I suppose that it is for gaming...Well, all games written for the Windows32 platforms use the Microsoft DirectX libraries to support multi-play.And DirectX - since Windows 95 - provides for multi-play in different ways, including LAN.(Also for 2 players with a null-modem cable on the COM serial ports)Greetings,John. -
Dear nachtgeist03, In order to limit the CSS effects to the HTML <A> </A> tags of the text navigation bar only, we have to combine the style-definitions with a CLASS. Let's elaborate the example: (1) First, in the CSS definitions we will choose a name for our class, most obvious would be: myNav <HEAD><STYLE>a.myNav {color:#009;background-color:#cfc;text-decoration:none;}a.myNav:hover {color:#009;background-color:#fcc;text-decoration:none;}</STYLE></HEAD> (2) Then, in the HTML, we will choose the same class name in the text nav bar <A> </A>, and not in the other <A> </A>. <A CLASS="myNav" HREF="some-link-to-some-URL1">text1-to-be-highlighted</A><BR /><A CLASS="myNav" HREF="some-link-to-some-URL2">text2-to-be-highlighted</A><BR /><A CLASS="myNav" HREF="some-link-to-some-URL3">text3-to-be-highlighted</A> The effects that were described in my previous post will now be applied only to the <A> </A> of the text nav bar. Concerning your new question, about using the same CSS in all your pages, without having to specify the <STYLE> </STYLE> in each and every page, that is easy too. (1) First we make a new text file, name it something like "mycss.css" and place it in some convenient place on our web-structure. Then we place the CSS definitions inside this file: a.myNav {color:#009;background-color:#cfc;text-decoration:none;}a.myNav:hover {color:#009;background-color:#fcc;text-decoration:none;} Only the definitions, no <STYLE> </STYLE> tags ! (2) Then, in every HTML file we place a reference to this file: <HEAD><LINK href="mycss.css" type="text/css" rel="stylesheet"></HEAD>
-
Dear nachtgeist03, Because you talked about "text link", I suppose that you mean a HTML <A > </A> tag. In that case, it is easy, even without the help of JavaScript. I propose to use CSS. Here is an example: (1) First we will declare a style-sheet definition. This can be done in a separate file, or it can be done in-line, in the HTML. Let's do it in-line. In the <HEAD> </HEAD> section we write something like the following: <HEAD><STYLE>a{color:#009;background-color:#cfc;text-decoration:none;}a:hover{color:#009;background-color:#fcc;text-decoration:none;}</STYLE></HEAD>(2) Then we can write the <A> </A> tag. <A HREF="some-link-to-some-URL">text-to-be-highlighted</A>What is the effect? The "color:#009;" definition will show the "text-to-be-highlighted" in dark-blue. The "background-color:#cfc;" definition will show the "text-to-be-highlighted" on a pale green background. The "text-decoration:none;" definition will remove the underlining from the "text-to-be-highlighted". When the surfers moves the mouse over the "text-to-be-highlighted", then the CSS definition "a:hover" is activated by the browser, and the net effect is that the "background-color:#fcc" definition will show the "text-to-be-highlighted" on a pale red background. I hope this answers your question. If I misread your question, and you did mean "some text links but not all text links on the page", then the use of CSS can be activated with the use of CLASS attributes. If I misread your question, and you did not mean "text link" to be a HTML <A> </A> tag, then the use of CSS can be activated on other HTML elements with the use of JavaScript. Greetings, John
-
Dear chiiyo, Thank you for pointing us to the Whatpulse site. I have picked up an idea for my hit-chart graph: (point 6 in my previous post) Originally I wanted to show a statistical graph of all the hits on the user's signature image, based on the Country-code of the surfers. But now I will try to show also the Country-flags on the chart, if I can find all of them. But I suppose that will be almost 250 flag images. P.S. Sorry that you do not (yet) understand PHP. Every computer language is easy, with the reference books by your side... and some friendly forum members on the 'net...
-
Dear chiiyo,After reading your post, I went to investigate J-sig.Indeed, the creation of the J-sig image is probably donein the same way as my prototype.But where J-sig differs is in the data that J-sig displays:First, the J-sig server script captures informationabout the surfers's browser, just like my prototype does.Second, the J-sig server script captures informationabout the J-sig subscriber's own machine, and in orderto do so, the J-sig subscriber needs to install the J-sigsoftware on his/her PC.This is in fact some sort of privacy intrusion, don't you think?What I want to do now:I need a PHP/mySQL hosting service, because my idea isto use a mySQL database.First, I will store in the mySQL database the texts thatare to be displayed inside the signature image.This will have to be done for each user.Second, I will have to write a PHP dialog that will allownew users to subscribe to the signature image service,and that will allow existing users to modify their texts.Third, I will store in the mySQL database the hits on thesignature images, when a surfers displays the image.Fourth, I will have to write a PHP script to draw an imagewith the statistics of the hits on each user's signature image.This chart image will function in the same manner as thesignature image itself, now that I know how to do that.Fifth, I will store in the mySQL database the TCP/IP addressesof all the Internet Service Providers in the world, togetherwith their Country-codes. The raw data - in CSV format - is freely available on the Internet.I have already programmed the SQL to load a mySQLdatabase with this information on my home test-server.Sixth, I will modify the PHP that draws the signature image,and the PHP that draws the hit-chart, and include the Country-codeof the surfers that view the signature images.Just like J-sig does.Now you see why I need a PHP/mySQL hosting service....
-
How I programmed my dynamic Forum Signature Image with PHP: (See the Signature Image at the bottom of this post) Part [1] Prepare the texts: Job [1.1] Find the TCP/IP address of the surfer: [tab][/tab]$SurferAddress = $_SERVER['REMOTE_ADDR']; Job [1.2] Find the name of the Internet Provider of the surfer: Job [1.2] Step [1.2.1] Find the name of the machine of the surfer: [tab][/tab]$SurferHostName = gethostbyaddr($_SERVER['REMOTE_ADDR']); Job [1.2] Step [1.2.2] Fetch the two last qualifiers (three if United Kingdom) [tab][/tab]$x1 = strrpos($SurferHostName,'.'); // Find the LAST . in the host-name:[tab][/tab]$Last = substr($SurferHostName,1+$x1); // Take the LAST word:[tab][/tab]$Rest = substr($SurferHostName,0,$x1); // Take previous part:[tab][/tab]$x2 = strrpos($Rest,'.'); [tab][/tab] // Find the NEXT-TO-LAST . in the host-name:[tab][/tab]$NextToLast = substr($Rest,1+$x2); // Take the NEXT-TO-LAST word:[tab][/tab]$Rest = substr($Rest,0,$x2); [tab][/tab] // Take previous part:[tab][/tab]$x3 = strrpos($Rest,'.'); [tab][/tab] // Take the NEXT-TO-NEXT-TO-LAST . in the host-name:[tab][/tab]$NextToNextToLast = substr($Rest,1+$x3);[tab][/tab]// Take the NEXT-TO-NEXT-TO-LAST word:[tab][/tab]$Rest = substr($Rest,0,$x3); [tab][/tab] // Take previous part:[tab][/tab]if ($Last == 'uk') { [tab][/tab] $SurferProvider = "$NextToNextToLast.$NextToLast.$Last"; }[tab][/tab]else { [tab][/tab] $SurferProvider = "$NextToLast.$Last"; } Job [1.3] Find the name of the browser: [tab][/tab]$BrowserInfo = $_SERVER['HTTP_USER_AGENT'];[tab][/tab]if (ereg("MSIE", $BrowserInfo)) { [tab][/tab] $SurferBrowser = "Internet Explorer"; }[tab][/tab]elseif (ereg("Firefox", $BrowserInfo)) { [tab][/tab] $SurferBrowser = "Firefox"; }[tab][/tab]elseif (ereg("Lynx", $BrowserInfo)) { [tab][/tab] $SurferBrowser = "Lynx"; }[tab][/tab]elseif (ereg("Opera", $BrowserInfo)) { [tab][/tab] $SurferBrowser = "Opera"; }[tab][/tab]elseif (ereg("WebTV", $BrowserInfo)) { [tab][/tab] $SurferBrowser = "WebTV"; }[tab][/tab]elseif (ereg("Konqueror", $BrowserInfo)) { [tab][/tab] $SurferBrowser = "Konqueror"; }[tab][/tab]elseif (ereg("Epiphany", $BrowserInfo)) { [tab][/tab] $SurferBrowser = "Epiphany"; }[tab][/tab]elseif (ereg("Gecko", $BrowserInfo)) { [tab][/tab] $SurferBrowser = "Mozilla"; }[tab][/tab]elseif ( (eregi("bot", $BrowserInfo)) || (ereg("Google", $BrowserInfo)) || (ereg("Slurp", $BrowserInfo)) || (ereg("Scooter", $BrowserInfo)) || (eregi("Spider", $BrowserInfo)) || (eregi("Infoseek", $BrowserInfo)) ) { [tab][/tab] $SurferBrowser = "Bot"; }[tab][/tab]elseif ( (ereg("Nav", $BrowserInfo)) || (ereg("Gold", $BrowserInfo)) || (ereg("Mozilla", $BrowserInfo)) || (ereg("Netscape", $BrowserInfo)) ) { [tab][/tab] $SurferBrowser = "Netscape"; }[tab][/tab]else { [tab][/tab] $SurferBrowser = "a browser"; } Job [1.4] Find the name of the operating systeem: [tab][/tab]if (ereg("Win98", $BrowserInfo)) { [tab][/tab] $SurferOs = "Windows 98"; }[tab][/tab]elseif (ereg("Windows 98", $BrowserInfo)) { [tab][/tab] $SurferOs = "Windows 98"; }[tab][/tab]elseif (ereg("Windows NT 5.1", $BrowserInfo)) { [tab][/tab] $SurferOs = "Windows XP"; }[tab][/tab]elseif (ereg("Windows NT 5.0", $BrowserInfo)) { [tab][/tab] $SurferOs = "Windows 2000"; }[tab][/tab]elseif (ereg("Windows NT", $BrowserInfo)) { [tab][/tab] $SurferOs = "Windows NT"; }[tab][/tab]elseif (ereg("Win", $BrowserInfo)) { [tab][/tab] $SurferOs = "Windows"; }[tab][/tab]elseif ( (ereg("Mac", $BrowserInfo)) || (ereg("PPC", $BrowserInfo)) ) { [tab][/tab] $SurferOs = "Macintosh"; }[tab][/tab]elseif (ereg("Linux", $BrowserInfo)) { [tab][/tab] $SurferOs = "Linux"; }[tab][/tab]elseif (ereg("FreeBSD", $BrowserInfo)) { [tab][/tab] $SurferOs = "FreeBSD"; }[tab][/tab]elseif (ereg("SunOS", $BrowserInfo)) { [tab][/tab] $SurferOs = "SunOS"; }[tab][/tab]elseif (ereg("IRIX", $BrowserInfo)) { [tab][/tab] $SurferOs = "IRIX"; }[tab][/tab]elseif (ereg("BeOS", $BrowserInfo)) { [tab][/tab] $SurferOs = "BeOS"; }[tab][/tab]elseif (ereg("OS/2", $BrowserInfo)) { [tab][/tab] $SurferOs = "OS/2"; }[tab][/tab]elseif (ereg("AIX", $BrowserInfo)) { [tab][/tab] $SurferOs = "AIX"; }[tab][/tab]else { [tab][/tab] $SurferOs = "a cool machine"; } Job [1.5] Find a cool message; always different: TODO: in MySQL [tab][/tab]$Text_Element[1] = "NO Software Patents !";[tab][/tab]$Text_Element[2] = "More games !";[tab][/tab]$Text_Element[3] = "Perl rulez !";[tab][/tab]$Text_Element[4] = "Rexx rulez !";[tab][/tab]$Text_Element[5] = "ADA rulez !";[tab][/tab]$Text_Element[6] = "Python rulez !";[tab][/tab]$Text_Element[7] = "C++ rulez !";[tab][/tab]$Text_Element[8] = "Basic rulez !";[tab][/tab]$Text_Element[9] = "Java rulez !";[tab][/tab]$Text_Element[10] = "JavaScript rulez !";[tab][/tab]$Text_Element[11] = "ALGOL rulez !";[tab][/tab]$Text_Element[12] = "COBOL rulez !";[tab][/tab]$Text_Element[13] = "Delphi rulez !";[tab][/tab]$Text_Element[14] = "Pascal rulez !";[tab][/tab]$Text_Element[15] = "FORTRAN rulez !";[tab][/tab]$Text_Element[16] = "Ruby rulez !";[tab][/tab]$Text_Element[17] = "Eiffel rulez !";[tab][/tab]$Text_Element[18] = "PHP rulez !";[tab][/tab]$Text_Element[19] = "mySQL rulez !";[tab][/tab]$Text_Element[20] = "SmallTalk rulez !";[tab][/tab]$This_Second = date('s'); // "00" --- "59"[tab][/tab]$Text_Index = 1 + (int)($This_Second/3);[tab][/tab]$Random_Text = $Text_Element[$Text_Index]; Part [2] Create the Signature Image: Job [2.1] Take a copy of the Template PNG Image that will serve as the background: Job [2.1] Step [2.1.1] Determine the sizes: [tab][/tab]$New_Width = 308;[tab][/tab]$New_Height = 123; Job [2.1] Step [2.1.2] Identify the Template PNG Image: [tab][/tab]$Source_Image_Name = "template.png"; Job [2.1] Step [2.1.3] Create a copy the Template PNG Image in a memory structure: [tab][/tab]$New_Image = ImageCreateFromPNG($Source_Image_Name) or die("Problem In Opening The Source Template Image"); Job [2.2] Write the 3 lines of text, and the 2 lines of signature, inside the new PNG image: Job [2.2] Step [2.2.1] Determine the geometry --- in order to position correctly: [tab][/tab]$Label_Width = 304;[tab][/tab]$Label_Height = 55;[tab][/tab]$Label_1_X_Offset = 2;[tab][/tab]$Label_1_Y_Offset = 10;[tab][/tab]$Label_2_X_Offset = 2;[tab][/tab]$Label_2_Y_Offset = 26;[tab][/tab]$Label_3_X_Offset = 2;[tab][/tab]$Label_3_Y_Offset = 42;[tab][/tab]$Sign_1_X_Offset = 2;[tab][/tab]$Sign_1_Y_Offset = 82;[tab][/tab]$Sign_2_X_Offset = 2;[tab][/tab]$Sign_2_Y_Offset = 98; Job [2.2] Step [2.2.2] Determine the colors to be used: [tab][/tab]$Default_Color = ImageColorAllocate($New_Image, 0xFE, 0xFE, 0xFE);[tab][/tab]$Label_Color = ImageColorAllocate($New_Image, 0, 0, 0); // must already be in the palette ![tab][/tab]$Sign_Color = ImageColorAllocate($New_Image, 151, 75, 51); // must already be in the palette ! Job [2.2] Step [2.2.3] Determine the texts for the 3 text lines, and the 2 lines of signature: [tab][/tab]$Label_Text_1 = "You are $SurferAddress at $SurferProvider";[tab][/tab]$Label_Text_2 = "You use $SurferBrowser on $SurferOs";[tab][/tab]$Label_Text_3 = $Random_Text;[tab][/tab]$Label_Font = 2; // 1 = mono (too small) --- 2 = mono-in-thin --- 3 = 2-in-bold (too large)[tab][/tab]$Sign_Text_1 = "Greetings,";[tab][/tab]$Sign_Text_2 = "John";[tab][/tab]$Sign_Font = 3; // 3 = mono-in-bold --- 4 = large-mono-in-thin (too large) --- 5 = 4-in-bold (too large) Job [2.2] Step [2.2.4] Determine the sizes of the text lines, depending on font and content: [tab][/tab]$Label_Text_1_Width = ImageFontWidth($Label_Font) * strlen($Label_Text_1);[tab][/tab]$Label_Text_2_Width = ImageFontWidth($Label_Font) * strlen($Label_Text_2);[tab][/tab]$Label_Text_3_Width = ImageFontWidth($Label_Font) * strlen($Label_Text_3); Job [2.2] Step [2.2.5] Determine the place of the text lines: 3 centered, and 2 left aligned: [tab][/tab]$Label_Text_1_X = $Label_1_X_Offset + ( ($Label_Width - $Label_Text_1_Width) / 2 );[tab][/tab]$Label_Text_1_Y = $Label_1_Y_Offset;[tab][/tab]$Label_Text_2_X = $Label_2_X_Offset + ( ($Label_Width - $Label_Text_2_Width) / 2 );[tab][/tab]$Label_Text_2_Y = $Label_2_Y_Offset;[tab][/tab]$Label_Text_3_X = $Label_3_X_Offset + ( ($Label_Width - $Label_Text_3_Width) / 2 );[tab][/tab]$Label_Text_3_Y = $Label_3_Y_Offset;[tab][/tab]$Sign_Text_1_X = $Sign_1_X_Offset;[tab][/tab]$Sign_Text_1_Y = $Sign_1_Y_Offset;[tab][/tab]$Sign_Text_2_X = $Sign_2_X_Offset;[tab][/tab]$Sign_Text_2_Y = $Sign_2_Y_Offset; Job [2.2] Step [2.2.6] Draw the text lines: [tab][/tab]ImageString($New_Image, $Label_Font, $Label_Text_1_X, $Label_Text_1_Y, $Label_Text_1, $Label_Color);[tab][/tab]ImageString($New_Image, $Label_Font, $Label_Text_2_X, $Label_Text_2_Y, $Label_Text_2, $Label_Color);[tab][/tab]ImageString($New_Image, $Label_Font, $Label_Text_3_X, $Label_Text_3_Y, $Label_Text_3, $Label_Color);[tab][/tab]ImageString($New_Image, $Sign_Font, $Sign_Text_1_X, $Sign_Text_1_Y, $Sign_Text_1, $Sign_Color);[tab][/tab]ImageString($New_Image, $Sign_Font, $Sign_Text_2_X, $Sign_Text_2_Y, $Sign_Text_2, $Sign_Color); Job [2.3] Write the new PNG image via http to the browser of the surfer: [tab][/tab]header("Content-type: image/png");[tab][/tab]ImagePNG($New_Image); I hope that the comments explain what is going on to produce the following result: (refresh to see the changes...)
-
With interest I have read these Topics. What I want to do with PHP is the following: Signatue-image This is the Forum Signature of my cousin, who lives in Europe. The image is generated in real-time. (Refresh the page, and see for yourself) My cousin refuses to tell me how he did this. But I want to do the same thing, also with PHP. Any ideas? Greetings, John. Notice from microscopic^earthling: This is NOT a tutorial and in future you should be more careful about where you're posting your threads. Moved to Programming > Scripting.