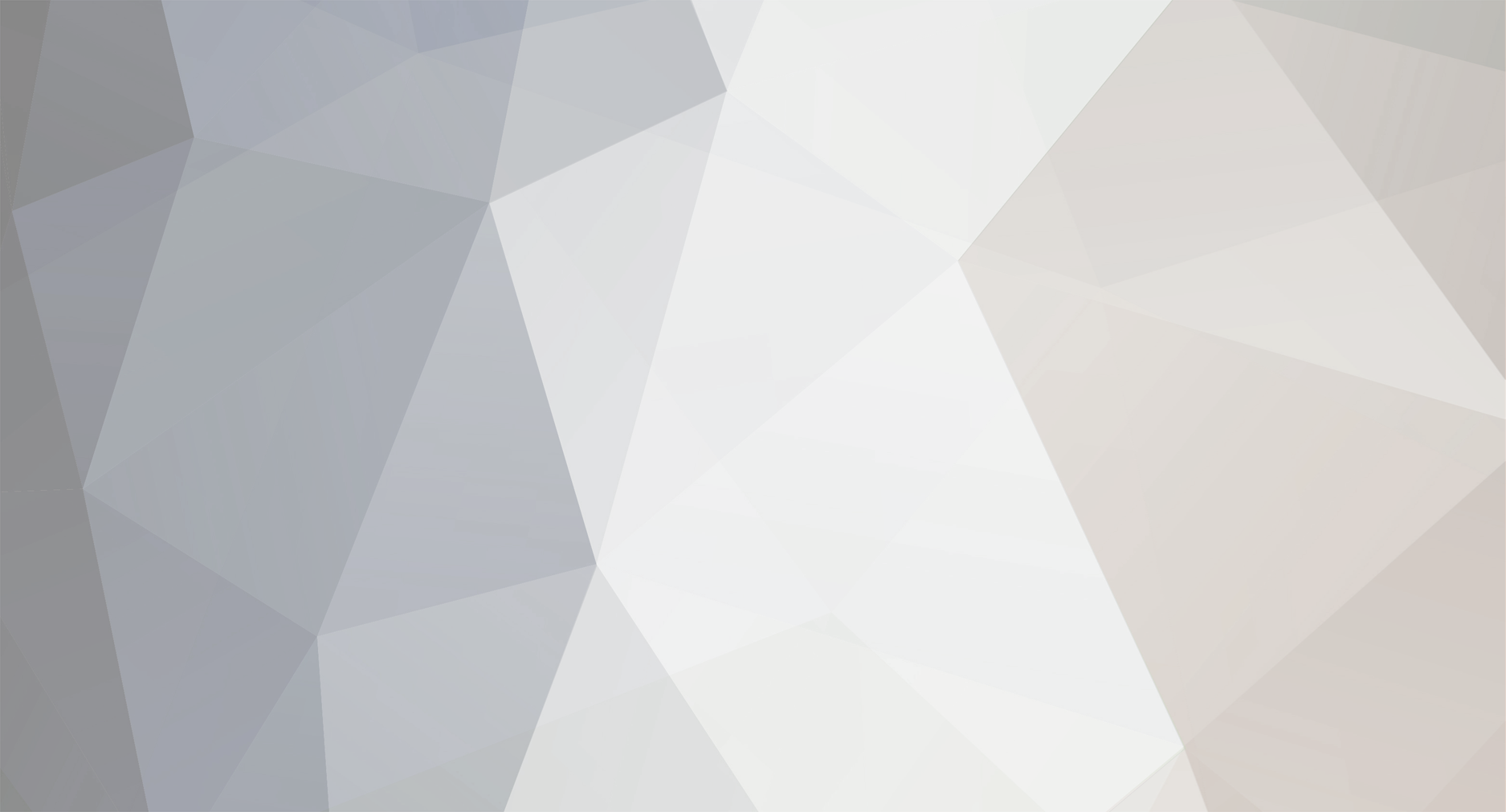
8ennett
Members-
Content Count
435 -
Joined
-
Last visited
Everything posted by 8ennett
-
I have made 13 posts so far at KS (including this one) but I still haven't started receiving myCENTs yet. After what I read it usually takes around 5 posts, and half the posts I have made have also been a decent size as well. Anyone got any ideas?
-
Ok, I have been through your initial sample code and made some changes/amendments. I've also never seen someone go through a sql result the way you did. Also while looping I noticed you keep assigning values to the same variable over and over, this will overwrite the information put in the variable when previously looped. Anyway here's my version of the code. <?php$username="mrdee";$password="**********";$database="mrdee_reloader";mysql_connect('localhost', $username, $password);mysql_select_db($database);$result = mysql_query("SELECT Name, Town, Email FROM rouw");if (mysql_num_rows($result) > 0){ echo '<table><tr><td>Name</td><td>Town</td><td>Email</td></tr>'; while($row = mysql_fetch_assoc($result)){ echo '<tr><td>'.$row['Name'].'</td><td>'.$row['Town'].'</td><td>'.$row['Email'].'</td></tr>'; } echo '</table>';}else { echo 'No results';}mysql_close();?> So the above code first opens the database like before, but I changed it around because for some reason you had a query run with no query data, then closed the database connection before running your real query. Next we run the mysql_query and put the result in to the $result variable, however I put the query data straight in to the mysql_query() function because I never saw the need to put it in a seperate variable if you aren't modifying it first. I also modified the query so it only selects Name, Town and Email instead of * which is all. This should reduce query time significantly. Next up we check to see if there are one or more rows returned from the query and if so we move on, if not then we echo No results. Now we have confirmed results, first we echo the start of the html output for our table. After that we run a while loop, but instead of looping through the amount of rows returned by the query instead we loop through the query result itself using mysql_fetch_assoc and assign the results of each row to the $row variable. So now we can access each returned column value through $row which is now an array of 3, $row['Name'], $row['Town'], $row['Email'] and obviously there would be more if you chose to select more from the database. So we insert these array items now in to a new table row (<tr></tr>) which constructs the table. At the end of the loop we close off the table and that's it. Now we are returning the results of our query in to a html table. If you don't want to echo the results then try using the following instead to place the table in to a variable instead. <?php$username="mrdee";$password="**********";$database="mrdee_reloader";mysql_connect('localhost', $username, $password);mysql_select_db($database);$result = mysql_query("SELECT Name, Town, Email FROM rouw");if (mysql_num_rows($result) > 0){ $resulttable = '<table><tr><td>Name</td><td>Town</td><td>Email</td></tr>'; while($row = mysql_fetch_assoc($result)){ $resulttable .= '<tr><td>'.$row['Name'].'</td><td>'.$row['Town'].'</td><td>'.$row['Email'].'</td></tr>'; } $resulttable .= '</table>';}else { $resulttable = 'No results';}mysql_close();?> If you use this code at the top of your php page then later on in the page if you want to display your new results table you would merely 'echo $resulttable;' and that's it. I hope this helps you!
-
The best php tutorial I have come across for beginners is located here https://devzone.zend.com/6/php-101-php-for-the-absolute-beginner/ It is in-depth and with witty comments and helpful examples, if you are starting out in php then it is definately where you want to go. After running through the tutorial then you will want to start looking up more advanced topics, but only after you have run through the entire 101 tutorial set.
-
There is another method to sending email from a local server without having to install an smtp server on your machine as well. If you have an account with an online smtp server (GMail for instance) and have a valid username and password for this server then you can download and install the PEAR Mail library for php.Once you have downloaded the PEAR Mail library you can then follow the instructions outlined on the PEAR website for sending mail through an smtp server, you can also use smtp servers with ssl encryption using PEAR. http://pear.php.net/package/Mail/redirected/ This will definately help if you are behind a blocked firewall or router without an open smtp port.
-
Yes, you haven't really given us a lot to go on here. We need to know the structure of your current code and database, then that would make it easier for us to help you modify the code to allow for user banning. If you want to create a list of banned email addresses then you could create a database table which contains each of these banned addresses then when a user tries to register with your site it will check the email address they entered against the list of blocked addresses. If it returns a value then the email address is banned and the user cannot register. eg. $checkban = mysql_query("SELECT id FROM banned WHERE address='".$email."'");if (mysql_num_rows($checkban) == 0){// Do registration stuff here}else {// Take user to register failed page} And also add a banned column to your userlist table in the database. So create a new field in your userlist called banned, make it an int(50) as well with a default value of 0. Next when your user either logs in or visits a logged in page on your site, check to see if the banned field is higher than the time() function. This way you can ban a users account for a set amount of time without having to manually unban them. So for example, during the login code... $checkban = mysql_query("SELECT banned FROM userlist WHERE userid='".$userid."' AND banned>".time());if (mysql_num_rows($checkban) == 0){// Do login here}else {// Go to login failed page} So it's a very simple concept, and writing the ability to ban user accounts in to your admin panel should be very simple. So the standard would be, check banned addresses during registration, check banned account during login, modify banned settings in admin panel. Then you will have a banning system in place for your site. It's highly adviseable that you also study how to prevent SQL injection from user inputted data, otherwise people will be able to manipulate your system to unban their accounts and maybe even delete your entire database. If you are unsure how to create the code yourself, don't. Put more time and study in to what it is you are doing before jumping in at the deep end and not only putting your website at risk, but your users information as well. There are many security issues you will need to study when php programming, as there is always the threat of some little hacker kid out there who has nothing but malicious intentions. Also marketing companies will stop at nothing to get a hold of personal information about people and use that information to their own ends.
-
Fun little toy lol nice work
-
Over at asta it all depends on what time you make your post, probably the same here as well. It generally tends to run at about 13 minutes past the hour (GMT) or around 43 minutes past the hour. Like was mentioned in the first post, the script that calculates the amount of myCents you get runs periodically and not constantly to prevent server load.
-
When it comes to the higher quality anti-virus programs, wether they be Norton, Kaspersky or AVG then there really isn't a 'best' one. It all depends really on personal choice and hardware. If you don't really want to spend the money on an anti-virus program but want a good all-round scanner for free then AVG is probably the best choice, then in the future if you want to upgrade your security package and pay for a premium scanner and maybe more, then since you have been using AVG free it would be a good idea to buy the premium version of AVG as you would be used to the interface already. If you want to go straight in to a premium package then you would probably go for something like Norton as it has the reputation and easy to use interface. Or maybe you had a months free trial on a new computer or laptop and you decide to extend the license. If you are running a computer with lower specs such as CPU speed or limited RAM then a lighter virus scanner would probably be in order. Norton uses far too much memory for slower computers and can end up chewing your entire operating speed. The same goes with kaspersky. I find that it's the interface more than the actual scanning that drives down computer speed, although the resident shield also is a significant drain. The choice is really down to you when it comes to choosing an anti-virus program, maybe give a few of the more popular ones a try using their free trials and see what you are most comfortable using, because in the end they mostly do the exact same thing anyway.
-
Sounds like a problem with your host, do they add a table prefix before the table name? eg. nyusername_shoutbox? If so, then when running the mysql query you will need to add the prefix to the beginning of the table name. You could also try changing while($r=mysql_fetch_array($result)){ to while($r=mysql_fetch_assoc($result)){ and see if that works. If that's not the case then make sure you are connected to the correct database through mysql_fetch_db() and this might also have a prefix on it (although that shouldn't matter) that needs including. This is a very old script, one of my first in fact and I haven't gone through and tested or debugged it. Am too busy with PGE nowadays as well to go through my old tutorials and double-check everything sorry.
-
Just a quick update for anyone who is interested. The engine is coming along fantastically, the module and addon installation is fully functional and impressive considering it's the first one i've written. Also, the community forums are up and running for help and support using PGE. I've been working hard on the next release and it should be capable of producing a fully working and secure game, which means you will be able to publish your new games with the next release then update the engine as new releases are made. I won't however be creating patches and updates for the engine until the 1.0 release (current is 0.8.2a which is still unstable) so might be an idea to hold off for a little while. Anyway, just thought I would add an update and let the asta community know I'm still here, just busy working on PGE. Take care all, 8ennett
-
Actually, I got sick of people all over the web looking for this type of thing, so I made one! You can make your own rpg game without any programming knowledge at all, and you can keep your games unique through modules and addon manager. Simply download the modules from the site (still under construction) then its simply a matter of clicking a button to install it. I haven't finished writing the engine yet but if you want to check it out then its at http://forums.xisto.com/no_longer_exists/
-
I don't think this can be very accurate as like was said before the women used seem to be more attractive than less (although beauty is in the eye of the beholder) but in order to get a true average you would have to merge the faces of every single woman in each country, and obviously that would be an impossible task.
-
It varies now, a .com is usually around 10-12 but a couple of them I have applied for have been up to 17 so there seems to be other factors involved.
-
Personally I think the whole concept of SEO has become highly corrupt and dishonest in recent years. Originally it was a way to properly prepare your site for search engines to format and rank accordingly. If a different website had more helpful information regarding the users search than your own then you would obviously expect to be a lower rank than that site. Not only would you expect it though, you would also respect it. Today however SEO has become a breeding ground for corrupt programmers who take on clients promising them they will get them to the top of the search results by any means necessary short of breaking the law (and for some, breaking the law). They flood message boards and websites with unwanted spam, completely abusing the intended purpose of these places, and deface innocent peoples websites, all so they can create a load of backlinks that search engines will pick up on in the hopes of increasing search engine ranks. My forums have only been online for a few weeks and already I have been targetted by a so-called SEO Optimisation service. After sending several warnings to the operator I still haven't had a response. Also the user is using a WhoIs protection service so I can't find out anymore information about the company or where they operate from. The site is xrumerservices dot org so if anyone knows the person operating xrumer from this site, please let me know so I can find and hit the guy.
-
Ultimately I would have to say winrar is my favourite for the reasons stated above and of course the way it acts just like an explorer window. You can keep going back a directory by clicking the .. folder icon and go back right out of the archive so you don't have to keep closing the software then opening a new process to skip between archives. If it is ultimate compression you want though, the best I have ever seen was insane. Somebody had managed to compress a 700mb CD in to an archive that was...wait for it...24KB in size. This was achieved using the KGB Archiver (the official site has had its account suspended unofrtunately but is still available for download elsewhere). KGB uses the PAQ6 compression algorithm and is one of only a few pieces of software to succesfully achieve this. The only draw-back to such a massive compression ratio is the amount of CPU cycles and RAM required to run the compression. Although KGB runs off 256mb RAM minimum and a 1.5GHz processor, if you want maximum compression and don't have the specs it can take days or even weeks to compress a 1GB collection of files. The best part of the KGB archiver is it is completely free. The 2.0 version is supported by Windows, and the 1.0 version is supported by UNIX type operating systems (command-line based). If you have a lot of files that need storing indefinately, use KGB and you won't go wrong. Just don't expect a quick extraction
-
Thanks, that's all I needed to know. Looking forward to posting on ks, over at asta it's more topic-relevant replies only but over at ks things are a little more relaxed and social.
-
Hey guys, i'm usually on Xisto more than KS but was wondering, on KS is there a myCent system? Can't seem to find any reference on the forums but over at asta people talk about ks being a myCent forum as well.Thanks in advance
-
I have started creating a game engine designed to create an entire text based php rpg game without the need for any coding. It also has a module and add-on feature where you can download additional game content and then install/uninstall it easily as well. The project can be found at http://forums.xisto.com/no_longer_exists/ and also a sample of a game created using PGE (Praetorian Game Engine).Definately worth a look once v0.8 has been released anyway!
-
So I thought I would put up a list of the tools available to help you in designing your websites. This ranges from firefox addons to downloadable software. When designing your website it is essential that you have the right tools at your disposal. You will generally need tools for debugging, cross-browser compatibility, page comparisons and so on. Just jumping in and throwing yourself at it can lead to some unexpected results in the long run. ############################# Web Developer Toolbar (Firefox Add-on) ############################# This is a brilliant toolbar for firefox. It contains all the tools you will need for debugging web pages quickly. The key features of this add-on are: CSS and Javascript checker with green ticks or red crosses if there are any errors and the source and line number of the error. Forms formatting including live element formatting, reveal password fields and removing max length. Full page information including listing all elements, javascript (and sources) and meta info. Page validator and so much more This is probably an essential tool for any web designer and highly recommended. ############################# User Agent Switcher (Firefox Add-on) ############################# Switches your browsers user agent to mimick other browsers. Useful for visiting websites that only allow particular browsers, or for debugging browser-specific coding in your own web design. I also wrote a tutorial on how you can use this add-on to create a competely hidden website within another website, Creating a hidden website ############################# Fox Splitter (Firefox Add-on) ############################# Remove the tab system in firefox and displays all of your open tabs in the same firefox window. Very handy for checking design consistency of all your sites pages and for doing two things at the same time. ############################# iMacros (Firefox Add-on) ############################# Really useful tool for recording reptitive tasks in forefox. Record your macro and then simply click play every time you want to run the macro. ############################# Firebug (Firefox Add-on) ############################# Firebug is handy and very popular. It allows you to view code live in the page, edit live in the page, modify and view pages css, also has a great set of debugging tools. ############################# IE Tab 2 (Firefox Add-on) ############################# This add-on is for firefox 3.6+, for versions lower than this you will need IE Tab. This add-on allows you to convert one of your current Firefox tabs to an internet explorer tab (requires IE to be installed). This is good for cross-browser compatibility testing without opening multiple browsers. ############################# FireFTP (Firefox Add-on) ############################# An FTP client that is built in to FF and provides a great interface and is fast and easy to use. Makes uploading and updating stuff to your servers very simple and very fast. ############################# GIMP (Software) ############################# Excellent piece of free software used for advanced image editing, touching, design. Supports multiple operating systems. ############################# Aptana (Software) ############################# Free, open-source, cross-platform, JavaScript-focused development environment for building Ajax applications. Features code assist on JavaScript, HTML, and CSS languages, FTP/SFTP support and a JavaScript debugger to troubleshoot your code. ############################# WebXACT (Website) ############################# WebXACT is a free online service that lets you test single pages of web content for quality, accessibility, and privacy issues. ############################# JsUnit (Software) ############################# Unit Testing framework for client-side (in-browser) JavaScript. Also included is a platform for automating the execution of tests on multiple browsers and mutiple machines running different OSs. ############################# Xenu (Software) ############################# Checks Web sites for broken links. Link verification is done on "normal" links, images, style sheets, frames, local image maps, plug-ins, backgrounds, scripts and java applets. It displays a constantly updated list of URLs which you can sort by different criteria. A report can be produced at any time. ############################# VisiCheck (Software/Website) ############################# Vischeck is a way of showing you what things look like to a person who is color blind. You can try Vischeck online by either running Vischeck on your own image files or running Vischeck on a web page. You can also download programs to let you run it on your own computer. ############################################### So that's the list for now, have a look through and see if anything there interests you. Everything is free btw. If you have any other free tools that you think can assist other in web development then add them to this post.
-
Gamma Server Down Anyone else having trouble?
8ennett replied to 8ennett's topic in Web Hosting Support
I reported this problem over 6 months ago sorry, and it didn't turn out to be the problem I thought it was. All my accounts are on alpha server now so I don't know about gamma sorry. If your site is down then I suggest logging in to Xisto - Support and filing a support ticket, also let us know the outcome as well. -
Ask.Com Is Better Than Google In My Opinion
8ennett replied to PublicThinker's topic in Search Engines
Google is most definately the better search engine for people who know their stuff and what they want. Its advanced features and custom code make it great. The translation part aside, if I want to search a single website for specific text but the site doesn't have its own search then I would simply type "site:thesite.com keywords" and it would return any content its webcrawlers have uncovered on the site with the keywords. Also the AI involved with its search results is amazing. Another good code for it is the inurl: command. You can lookup web pages where the url contains the string instead of the site. Very handy if you can only remember part of a websites url. The image search is really handy as well, especially how you can specify dimensions as well. My opinion of ask.com was somewhat dissapointing. I found it a very basic website designed for plain and common questions, it wasn't capable of properly returning results for my searches. -
If you want to use a pre-built one like phpBB or something similar then that's what they are there for. If you want to make your own then we can point you in the right direction and give you hints and tips and help you with coding problems, I'd be more than happy to write a tutorial covering the basics on making forums. If you want someone to build the whole thing on their own and just give it to you then PM me and I will tell you what my hourly rates are.