Sign in to follow this
Followers
0
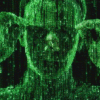
Sql Injection, How To Do It, And How To Prevent It NOT a hacking tutorial, learn how to secure PHP scripts
By
shadowx, in General Discussion
By
shadowx, in General Discussion
Terms of Use | Privacy Policy | Guidelines | We have placed cookies on your device to help make this website better. You can adjust your cookie settings, otherwise we'll assume you're okay to continue.