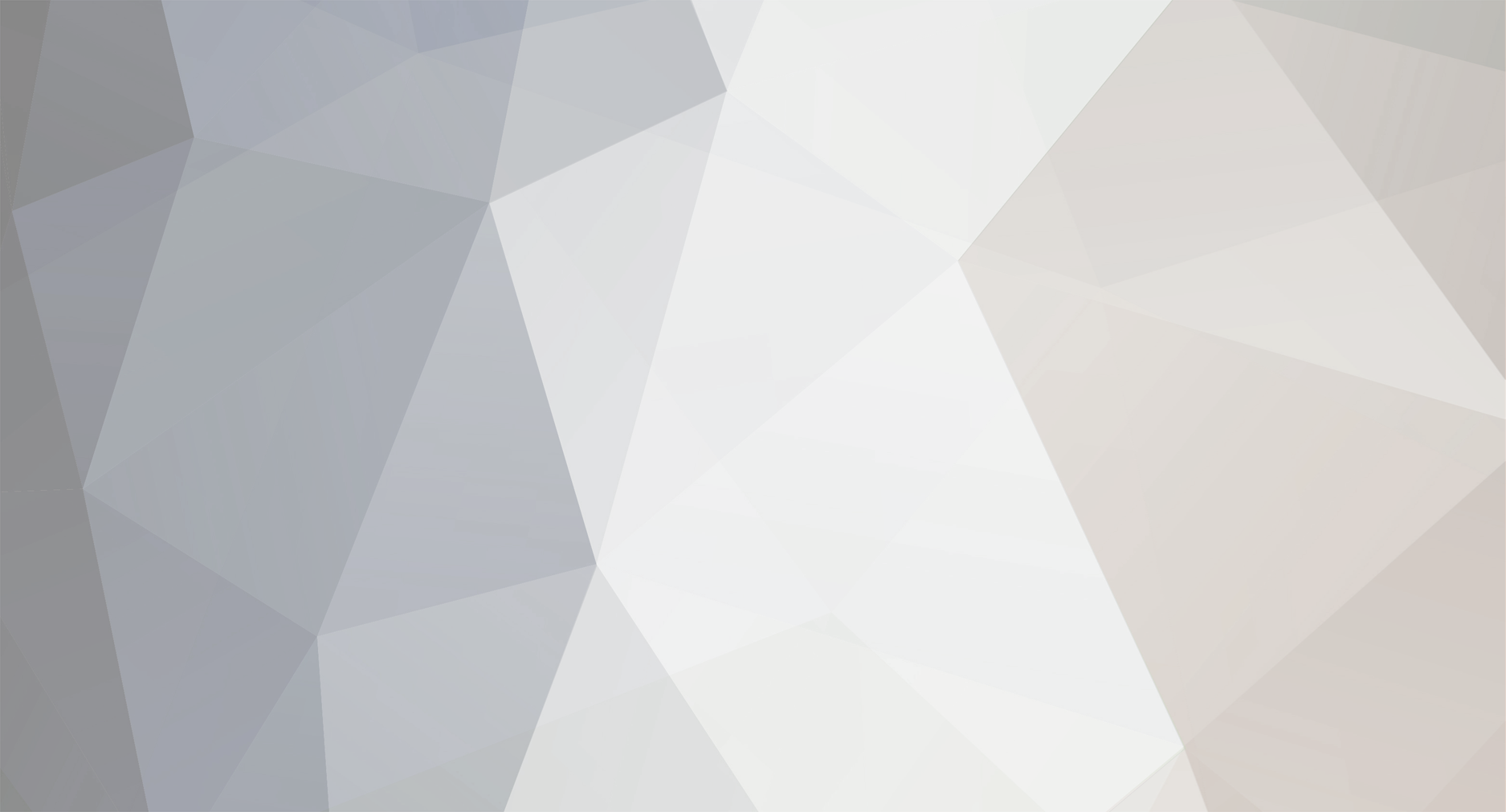
sachavdk
Members-
Content Count
66 -
Joined
-
Last visited
Everything posted by sachavdk
-
Many people want to make logins to their site. The easiest way to do this is using PHP. I will describe here all steps creating one. You can copy and paste the code and save it as the name of the file given above the code. Save everything in the same directory. NOTE: However the code can be copied its still no basic php anymore. If you have questions you can ask them but a little knowledge of php is useful. First of all we need to make a login form. This can be an htmlpage. We are going to ask the user for his username and his password. File is named: "login.html" <html><head><title>My website</title></head><body><form action="login.php" method="post"><!-- action will be the url where the form is posted to, it can be a page (http://yourdomain/login.php) or a page with variables(http://yourdomain/?page=login) for examle.The method is the way the form is submitted. Using GET the form will be submitted using the addressbar and all values will be visible. Since we're sending apassword this is defenetly not what we want. That's why we're going to use POST. Post sends the form in an "invisible" way. This means the input can not beviewed by the user. This way of sending can still be hacked but in your case it is probably not important enough to do so. --><h1>Login</h1>Username: <input type="textbox" name="username"><br>Password: <input type="password" name="password"><br><input type="submit" value="Login!" name="submit"><br>No an account yet? <a href="register.html">Register</a></form></body></html> That was pretty easy. Now I'm expecting you have your database set up (I use MySQL with these scripts). If not do this now.You will need a table named "tblusers" with fields: * ID, Integer, Null, auto_increment, primary key * Username, Text * Password, Text In the example I will use the following script included in every script so I will have to declare only once my database login settings. Change the settings below to yours. Page is named: "DatabaseConfig.php" <?php // every var gets a prefix "db_" do declare its about the database connection later$db_username="username"; //database username$db_password="password"; //database password$db_host="localhost"; //host of your database$db_database="dbmylogin"; //database name$db_table="tblusers"; // tablename$db_conn = mysql_connect($db_host,$db_username,$db_password) or die (mysql_error());$db_conn = @mysql_select_db($db_database,$db_conn) or die (mysql_error()); // using the "@" gives a secure connection?> Thats it for the database. You see this makes things a lot easier. People want to register on your site before they can login. Here's the registration form: Page is named: "register.html" <html><head><title>My website - Registration</title></head><body><form action="registration.php" method="post"><h1>Registration</h1>Desired username: <input type="textbox" name="username"><br>Desired password: <input type="password" name="password"><br><input type="submit" value="Register!" name="submit"><input type="button" value="Login" onClick="javascript:window.location='login.html'"></form></body></html> That's the registration form.Now we have the registration script wich is going to validate username if it exists and if not, register it. Page is named: "registration.php" <?phpsession_start();if (!isset($_POST["submit"])) // checks wheter the page is called using the registration form{// it is not called using the registration formheader("Location:register.html"); // the script sends the user to the registration page} else {// it is called using the registration formif ($_POST["username"] == NULL || $_POST["password"] == NULL) // check wheter both username and password are filled{ // one of the two is not filled echo "<font color=\"red\">Not all fields were filled. Please go <a href=\"javascript:history.back(1)\">back</a> and fill them</font>";} else { // they are both filled, check wheter the username exists in the database // remember we made "DatabaseConfig.php"? Here it will be included so connection is made with the database. include("DatabaseConfig.php"); // now its simple, otherwise we had to make connection everytime with much more code. $usernameexists = mysql_query("SELECT ID FROM tblusers WHERE username='".$_POST["username"]."'") or die (mysql_error()); if (mysql_num_rows($usernameexists) != 0) { // user exists echo "<font color=\"red\">User exists. Please go <a href=\"javascript:history.back(1)\">back</a> and chose another username.</font>"; } else { // user doesn't exists $add_user = mysql_query("INSERT INTO tblusers (username,password) VALUES ('".$_POST["username"]."','".$_POST["password"]."')"); if ($add_user) { // user is succesfully added echo "<font color=\"red\">You are succesfully registered. You can now <ahref=\"login.html\">login</a></font>"; } else { // error occurd echo "<font color=\"red\">An error occurd. Please go <a href=\"javascript:history.back(1)\">back</a> and try again.</font>"; } } // don't forget to close the database connection when you don't need it anymore mysql_close();}}?> End of registrationscript Loginscript Page is named: "login.php" <?phpsession_start();if (!isset($_POST["submit"])) // checks wheter the page is called using the login form{ // it is not called using the registration form header("Location:login.html"); // the script sends the user to the registration page} else { // login form is used, continue with login if ($_POST["username"] == NULL || $_POST["password"] == NULL) // check wheter both username and password are filled { // one of the two is not filled echo "<font color=\"red\">Not all fields were filled. Please go <a href=\"login.html\">back</a> and fill them</font>"; } else { // they are both filled, check wheter the username and password exists in the database, password must be for the same user // remember we made "DatabaseConfig.php"? Here it will be included so connection is made with the database. include("DatabaseConfig.php"); // now its simple, otherwise we had to make connection everytime with much more code. $usernameexists = mysql_query("SELECT ID FROM tblusers WHERE username='".$_POST["username"]."' && password='".$_POST["password"]."'") or die (mysql_error()); if (mysql_num_rows($usernameexists) != 0) { // user exists $_SESSION["mywebsite_userid"] = mysql_result($usernameexists,'',"ID"); ?> <font color="red">You are successfully logged in.</font><br> Go to the secret page: <a href="secret.php">Secret page</a> <?php } else { // user doesn't exists echo "<font color=\"red\">Wrong combination username/password. <a href=\"login.html\">Try login again</a> or <a href=\"register.html\">register</a></font>"; } // don't forget to close the database connection when you don't need it anymore mysql_close();}}?>that's the loginscript Now the last script we need is the one who takes care on the secured pages to see if you are logged in. And this is the script to check if you're logged in: It's named "checklogged.php" <?phpsession_start();if (!isset($_SESSION["mywebsite_userid"])){ // visitor is not logged in echo "You need to be logged in to see this page. <a href=\"login.html\">Login</a> or <a href=\"register.html\">register an account</a></font>"; die();}echo "<a href=\"logout.php\">Logout</a><br>";?> You use following piece of code in every page you want to secure a page. Just set it in the very top of your script. EVERY PAGE YOU WANT TO SECURE MUST BE A PHP SCRIPT!!! <?phpinclude("checklogged.php");?> And ofcourse we need a logout script:Page is named: "logout.php" <?phpsession_destroy();echo "You are logged out. <a href=\"login.html\">Login</a>."echo $_SESSION["mywebsite_userid"];?> Now that's all. Since I don't know what problems you might have just put them here. PS: for people who don't know php, you can't test it on you pc unless you have a server running on it. But you can test it on Xisto. But nevertheless you will have to create you database. You can do that accessing your control panel and go to "MySQL Databases" and create one there. I'm not used to it on Xisto but I think there are people who will help you with that using this topic. The structure and the name of the table you find some higher on this page. Of course then you have to edit the DatabaseConfig.php script with your settings. PPS: important is that posting this in this topic the structure of the code when you past it isn't correct anymore. If you want to use the script simply download the attachment. It's my working script.
-
I did it and it actually worked.It takes now +/- 3 secs Very cool
-
Setting The Height Of A Tablerow Its variable so I want to use
sachavdk replied to sachavdk's topic in Programming
Extra note:This only serves to make sure the "Content listing Cell" reaches the bottom of the screen on every resolution. Since when I set the resolution to 1280*1024 it is shown correct. But when I set it ie. to 1024*768 the fixed height is to great and so you can scroll an empty page wich is sertainly not the intention. -
Setting The Height Of A Tablerow Its variable so I want to use
sachavdk replied to sachavdk's topic in Programming
I'm affraid you did . The minimal height of the "Content listing Cell" is the height of the window that shows the page (not the entire browserwindow ofcourse) minus the Y position off the "Content listing Cell". So I actually need the "Content listing Cell"'s Y position (I guess you can call it from a Javascript function using an ID for the cell) and the height of the screen (not the page) and then set the height of the "Content listing Cell" using its ID i guess again to the subtraction of screenheight and "Content listing Cell"'s Y position. So short said in pseudocode: Set the height of the "Content listing Cell" to screenheight (maybe document.height or so?) - "Content listing Cell".Ypos (maybe topOffset or so?). I'm not that familiar with JavaScript you see. I know the syntax but not that kind of functions. Hope someone can say me how to get those 2 vars and then apply the height to the cell. -
I have a html page with a table. The next "image" shows the layout: -------------------------------------------------------------- HEAD CELL -------------------------------------------------------------- CONTENT | Main content cell LISTING | CELL... | --------- Now the "Content listing cell" should always reach the bottom (its a grey cell and the main content is white, so their should be two columns). If the main content cell's content is long enough so you can scroll down, their is no problem. But when the "Main content cell"'s content doesn't reaches the bottom of the page, the table doesn't reaches the bottom of the page. How can I solve this? I think the only way is JavaScript but how... PS: I hope my explanation is clear
-
Here's also a good program for creating icons form bitmaps, gifs,... and it even supports psd. You can also create avi files from animated gifs and series of icons (ie 8bit, 24bit, 32bit(24bit/8bit alphatransparancy), 48*48,32*32,24*24,16*16)... http://www.gamani.com/ Unfortunately its not free but you can use a non-limited edition for 30 days. And it's also not to expensive to buy ($39.95) (when you've seen its possibilities you know why ) PS: there are also older versions available for download. Check that you download version 4.0.2 (4.0)
-
Yes indeed I've found a tutorial on the website of microsoft. Now I can also create my own in perfect quality.So problem is solved Previous reply was a little wrong. I don't want to let it be like it was a reply on what biscuitrat said (so only the first line was mended to be quoted). Sorry.
-
-
The only thing I did was adding an image to the page <td><img src="Icons_ICO/ii_DeskTop16.ico" border="0" dir="rtl"> Home</td>This worked fine with the downloaded icons but I wanted to use the better quality of the windows icons.Strange is that their is an image on the page (I can select it) but it's empty. Also the favicon is empty when I use such a windowsicon <link rel="shortcut icon" href="./ii_Users.ico" type="image/x-icon">[code=auto:0]
-
Hi, I'm making a website (I'm in de layout design process) and I want to use ico icons in my page. I donwloaded some "xp-icons" and all worked fine. But because windows' own icons have a better quality I used ResourceHacker to take windowsicons from Shell32.dll (in system32 dir).In thumbnailview they are shown and Windows Picture Viewer shows them also, but when I used them in my webpage, they weren't shown. They also don't work as favicons.It is probably the format of the icon but is there some way (batch, tool,...) to convert the icons to a web-usable ICON, not a gif or png.Not gif because it doesn't support alphatransparancy and not png because IE doesn't support png's alphatransparancy and I don't want to integrate a script which makes png usable in IE.So?
-
1. I've forgotten to tell it, sorry. 2. Wherever you want in your webroot. 3. It doesn't matter if you use Inetpub or some other. Imagine your wwwroot is "C:\MyDocuments\WWW\WEBSERVER\" and you have 5 webprojects in your webroot ie pro1, pro2, pro3, pro4 and pro5, each is located in "C:\MyDocuments\WWW\WEBSERVER\" so you have "C:\MyDocuments\WWW\WEBSERVER\pro1\" "C:\MyDocuments\WWW\WEBSERVER\pro2\" "C:\MyDocuments\WWW\WEBSERVER\pro3\" "C:\MyDocuments\WWW\WEBSERVER\pro4\" "C:\MyDocuments\WWW\WEBSERVER\pro5\" and maybe some configdir or a dir you dont want to have listed "C:\MyDocuments\WWW\WEBSERVER\whatever\" if you place the script in "C:\MyDocuments\WWW\WEBSERVER\" and you replace line 8 (if ($file != 'IIS_Services' && $file != 'RECYCLER' && $file != 'Config' && $file != 'System Volume Information')) by if ($file != 'whatever') the script will give you a list from all projects (pro1 to pro5). If you click one, the script will open the project (directory) in a new window. You can set this script whereever you like (ie you named this script list.php and set it in your rootdir) and go to http://forums.xisto.com/no_longer_exists/ TO EVERYONE WHO READS THIS: if ($file != 'IIS_Services' && $file != 'RECYCLER' && $file != 'Config' && $file != 'System Volume Information') is just a line of code so dirs I don't want to be listed, are not listed.If there are no dirs you don't want to be listed change the line to if ($file != '') this way all dirs are listed. I hope it's clear now to you.
-
Normally it could serve for Apache Servers. Run the script and see which directories are listed you don't want to if ($file != 'IIS_Services' && $file != 'RECYCLER' && $file != 'Config' && $file != 'System Volume Information') IIS_Services, Config are directories I created to install PHP and MySQL, RECYCLER and System Volume Information are part of IIS and can be deleted with apache. IE. you have three directories. Two are project and one is some configuration dir. 1. MyConfiguration 2. Project1 3. Project2 Now you don't want dir MyConfiguration listed, then you simply have to replace if ($file != 'IIS_Services' && $file != 'RECYCLER' && $file != 'Config' && $file != 'System Volume Information') by if ($file != 'MyConfiguration'). And for the links, normal it should display a link on every project this code takes care of that: echo '<tr><td>'; echo '<a href="'.$file.'/" target="_blank"> [ '.$file.' ]</a> '; echo '</td></tr>';if it shouldn't work, try this: echo '<tr><td>'; echo '<a href="./'.$file.'/" target="_blank"> [ '.$file.' ]</a> '; echo '</td></tr>';
-
Photoshop - Removing Red Eyes How to remove red eyes
sachavdk replied to sachavdk's topic in General Discussion
I'm sorry I read your reply wrong. I said I didn't find any tut but I meant on this forum . -
Photoshop - Removing Red Eyes How to remove red eyes
sachavdk replied to sachavdk's topic in General Discussion
Hmm, yes, though I wrote this tutorial myself and it's based on how I have found to remove red eyes in photoshop. It's something I accidentally found when I was trying to color a black/white picture for school. What do you mean with you can't even tell if a brush has been used? -
I recentely installed IIS with PHP and MySQL on my pc (previous I used UniServer, but that doesn't matter here). But I had always to go to LOCALHOST/websiteiwanted/ or I had to create a shortcut on my desktop for every site so I decided to create an "overviewpage". It shows all the websites in your wwwroot with a link to them. If you have folders you don't want to be included, you extense the && check (but I'll explain this lateron) Here's the total code: <ul><table cellpadding="3" cellspacing="3" border="0" width="95%"><?$dir=opendir('.');$isdirtrue = false;while ($file = readdir($dir)){ if($file != '..' && $file !='.' && $file !=''){ if (is_dir($file)){ if ($file != 'IIS_Services' && $file != 'RECYCLER' && $file != 'Config' && $file != 'System Volume Information') { $isdirtrue = true; echo '<tr><td>'; echo '<a href="'.$file.'/" target="_blank"> [ '.$file.' ]</a> '; echo '</td></tr>'; } } }}if ($isdirtrue == false) {echo "<div align='center'>- No projects -</div>";}closedir($dir);clearstatcache();?></table></ul> Now I'll explain every step $dir=opendir('.'); opens the current folder. You could use .. to specify the folder one level up or ./folder/folder to open other folders. $isdirtrue = false; is going to check if the opened file is a directory lateron in the script, this just initializes it while ($file = readdir($dir)){ is going to read every "file" in the opened directory. Also . .. are files in the opened folder. This can be convenient sometimes but now they don't matter thats why I'm gonna use following code. To see that the opened file not . or .. is: if($file != '..' && $file !='.' && $file !=''){ if (is_dir($file)){ checks wheter the file is a directory. if it is one, we go to the next step if ($file != 'IIS_Services' && $file != 'RECYCLER' && $file != 'Config' && $file != 'System Volume Information'){ here you can specify which folders don't participate in your projects list $isdirtrue = true; this sets the isdir to true because we have at least one project in the list. more explanation withing some lines echo '<tr><td>'; echo '<a href="'.$file.'/" target="_blank"> [ '.$file.' ]</a> '; echo '</td></tr>'; these are gonna write a link to the projectfolder if ($isdirtrue == false) { echo "<div align='center'>- No projects -</div>"; } now remember that we just set $isdirtrue to true because we had at least one project? at the beginning of the script we initialized it false. if there wouldn't have been a projectdirectory it would still be false and then it would give a message that there are no projects on your server. now very important are closedir($dir); and clearstatcache(); because if you don't use these, your cache memory will be left full of needless information. closedir() closes de directory you opened in the beginning of the script and clearstatcache() deletes all "temporary files" from the memory. Now that's it. I hope lots of people will follow my example and be more neater with their local webserver.
-
Since I haven't found any tutorials on how to remove red eyes, I'm gonna explain it in a very short way. (Removing them is far easier than you probably think). What we first need is a photo of someone with red eyes like the picture below What we are going to do next is adding an "Adjustment Layer". Goto Layer => New Adjustment Layer => Channel Mixer When you get a dialog "New layer" press OK. Now in the next dialog copy these settings: Press OK. The image should look weird, but don't mind that. Now press D to reset your colors. (Your foregroundcolor should be white and your backcolor black) Then press LeftControl+Backspace, and the image will seem to look like before when you loaded it (with red eyes). If not check that you did everything correct. Now select the brush tool with these settings: make the size of the brush the same size as the one of the orphan. Now hold your brush exactly above the orphan and click one or several times. The red eye should become black like this one: Simple eh?
-
Rollout Menu In Javascript How making rollout menu using JavaScript
sachavdk replied to sachavdk's topic in Programming
Hmmm its great . I'm gonna try it to implement in my menus. Thanks -
Rollout Menu In Javascript How making rollout menu using JavaScript
sachavdk replied to sachavdk's topic in Programming
I'm sorry I'm double posting, but I've forgotten to mention the menu can use some html and images etc. -
Hi, As I suppose everybody knows the JavaScript menus when you roll over a button, a graphical submenu is rolled out (such as the menus in windows). Now I want to try making one myself since it is a project for school and I can't come up with a ripped version of some menu. The idea is when a user goes with the cursor over a tablecell, a javascriptmenu is shown. ie: <table><tr><td onMouseOver="javascript:show_menu('whatever')">Whatever</td></tr></table> I can work with JavaScript and I have some experience using little functions, but this is probably gonna be a huge arithmetical and graphical function. Is there someone who can help me starting to make such a menu?
-
Music Player Photoshop Create A MusicPlayer in photoshop
sachavdk replied to sachavdk's topic in General Discussion
Like I said it wasn't the meaning of making a player, but maybe I'll try if I find some time, so don't count on it. -
Cool Impressing my friends with "serversided html" though its just phpparsed html
-
Isn't it easier to do it in a way like this: <?$var1 = "foo";$var2 = "bar";$foobar = "the_thing_you_want";eval("\$finalvar = \$".$var1.$var2.";");echo $finalvar;?> The code above will write the_thing_you_want on the screen
-
Website Help Need php programmers to help me.
sachavdk replied to Ao)K-General's topic in Programming
First thing: DON'T ever ask paid help if the thing you want is not commercial and you're request is paid by a company. There are enough people willing to help you freely as you have seen. But explain your problem or otherwise it will be hard for others to help you. I'll see what I can do maybe together with the others. -
Maybe this is what you want: <html><body><select name="datum"><?$date = getdate();for ($i=$date[0];$i<=($date[0]+31536000);$i+=86400){ echo "<option value=\"".date("Y/d/m H:i:s",$i)."\">".date("Y/d/m H:i:s",$i)."</option>";}?></select></body></html> the list orders the dates from the day the list is called (ie when you're testing the script) until today next year. if you don't want today next year to be listed use in the code instead of 31536000 this number: 31449600. The date is written in the format YYYY/DD/MM HH:MM:SS (year/day/month hour:minute:second). You can change it if you want. If you want to use other look here http://be.php.net/manual/en/function.date.php. You only have to change the formatting "Y/d/m H:i:s" to ie "d/m/Y" but remember with this script you have to do it twice. Once for the value and once for the one that is written in de dropdownlist. If anything doesn't work like you want please ask