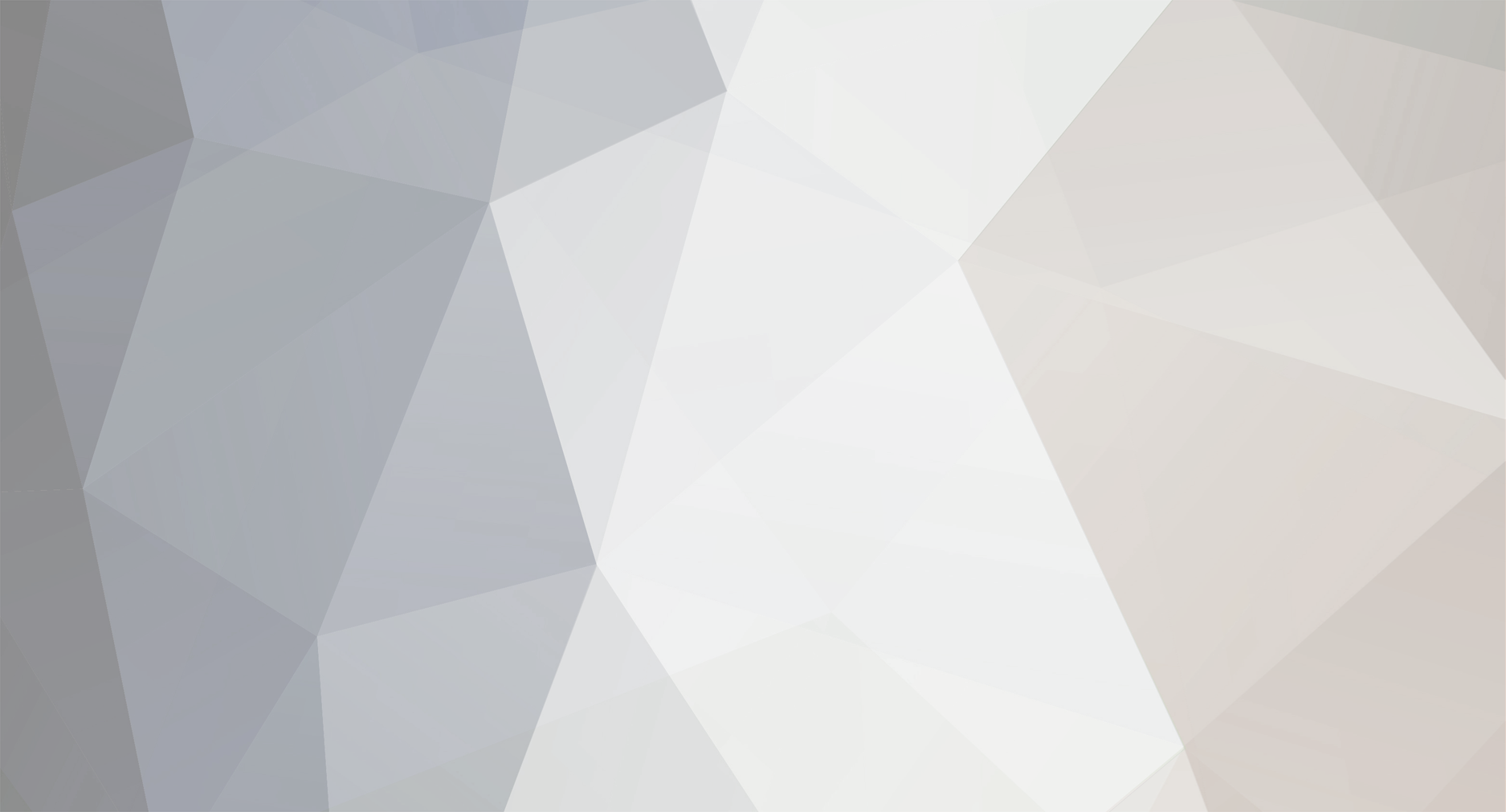
mitchellmckain
Members-
Content Count
403 -
Joined
-
Last visited
Everything posted by mitchellmckain
-
Avast! Free anti-virus software
mitchellmckain replied to friso's topic in Security issues & Exploits
Well I had some trouble with a conflict with ZoneAlarm. Avast detects ZoneAlarm and knows about the conflict after restarting your system for the first time. The problem is that it ask you if you want some sort of invisble proxy for web shield disabled (or something like that). Definitely say Yes. Otherwise it will not allow any cookies at all and web surfing is nil. I couldn't find any way to fix this except by uninstalling Avast and reinstalling (saying no to scheduled scan this time) so that I could give the proper answer of "Yes" to the question about disabling the invisible proxy... whatever. Now surfing seems to work fine. -
Avast! Free anti-virus software
mitchellmckain replied to friso's topic in Security issues & Exploits
Well since I was looking for a free replacement for Norton AV since I removed it a week ago, I followed your recomendation and installed avast today. Wow. I am impressed. The initial scan took about an hour, but the number of trojans that it found, that nothing else has found so far blew me away. I am not too crazy about the two programs running in backgroud, although the sound effects are hillarious. I am willing at least to see how it goes. The real test will be to see if interferes with other demanding programs running at the same time. I am not too willing to endure gameplay slowdown for any programs sake. After paying the Norton AV subscription for the last two years, a free program is hard to beat. Regardless, it is clear that Norton AV just plain sucks. -
(Personal note: I am a physics teacher and a hobby programmer. Since I doubt anyone here is interested in a physics tutorial I figured it would be better to write a tutorial on something I learned while programming.) What I will cover here may be considered something of a lost art since keyboard control has been largely replaced by the use of the mouse. A lot of users are bewildered by the keyboard and the effort of deciding which one to push. Therefore, point and click has come to be considered a superior method of program control. Most programmers only deal with keyboard input after some windows procedure like getch is through with it and all you see is an ascii code. They might not realize that this ascii code has very little to do with the keyboard in front of them. In a Win32 program you handle messages that originate from the keyboard, and all the information from the original keyboard event can be recovered. Programming in Win32 is a messy subject and a lot of it is best side-stepped by modifying a previous program and learning as you go along. In any case, there are a lot of great Win32 tutorials out there already. Since this material is based on Win32 I will point out a few here, but all are found easily with Google. http://www.functionx.com/win32/http://www.winprog.org/tutorial/ members.net-tech.com.au/alaneb/win32.html http://forums.xisto.com/no_longer_exists/ http://www.catch22.net/tuts/ The basic win32 program calls a proceedure to set up the window then goes into an endless loop which checks for messages (caused by user input) and updates the display. The messages are handled by a callback proceedure (sometimes called WndProc), written by you, but which is called by the operating system. This WndProc gets four parameters but only three important parameters: a message identifier and two words of data often refered to as wParam and lParam though we could call them data1 and data2. Your WndProc usually consist of nested switch statements in order to respond to the various messages according to the identifier and data sent to it. There are constants defined in windows.h like WM_CREATE and WM_COMMAND for different message identifiers that aid you in constructing this switch statement. For example, WM_CREATE message is sent when the window is created so you can use procedures like AppendMenu and SetMenu to create a window menu bar. The WM_COMMAND message is sent when a window menu item has been selected and the data1 pararameter tells you which one is selected. When you handle the message you return a 0 back the caller, otherwise pass the parameters on to the default windows message handler, DefWindowProc. For example, LRESULT CALLBACK WndProc(HWND hWnd, UINT uMsg, WPARAM data1, LPARAM data2) { switch(uMsg) {[tab][/tab]case WM_CREATE: <code to create menus> return 0;[tab][/tab]case WM_COMMAND: switch(data1) { case ID_HELP_ABOUT: <code to display "About this File" message> return 0; <other cases to handle menu selections> }[tab][/tab]} // Pass all unhandled messages to DefWindowProc return DefWindowProc(hWnd,uMsg,wParam,lParam); } For this tutorial, the message identifiers we are interested in are: WM_KEYUP, WM_SYSKEYUP, WM_KEYDOWN, and WM_SYSKEYDOWN. These are sent whenever a keyboard key is pushed or released. All the information, about what key was pressed and how, is contained in data1 and data2. Data1 contains something called the virtual keycode. This is a code halfway translated to ascii that is sufficient for the keyboard control of many applications. For example all the letter keys have a virtual key code that is just the ascii code for the capital letters and the number keys above the letters have a virtual key code that is ascii codes for these digits. But, the numeric keypad numbers, for example, have different virtual key codes 96-105 to distinguish them from the other keys. However, the virtual keycode alone does not uniquely identify every key on todays enhanced keyboards, therefore data2 is useful in recovering this information. Data2 is a composite of different numbers and bits. The lower 16 bits (0 thru 15) is an auto repeat count. When a key is held down, depending on the windows settings, it is interpreted as repeated a number of times corresponding to how long the key was held down. If the key is held down long enough, more KEYDOWN messages are sent with repeat counts for the additional time they key is held down. Bit 30 is set in this case to indicate that these are additional KEYDOWN messages and that the key was already down. Bits 16 through 23 contain a more primitive keyboard code called the scan code. The scan code is closely related to the actual position of the keyboard buttons on the keyboard (so it is keyboard dependent), but it doesn't distinguish the keypad and many other keys on todays keyboards. On my keyboard for example, the numeric keys 1 through 9 and 0 have scan codes 2 through 11. The numbers on the key pad, and any other arrow keys, insert, delete, home, end, pgup, and pgdn all have the same scan codes 2 through 11. Bit 24 is set for the extended keys on todays extended keyboards. These include the right hand alt and ctrl keys, the arrow keys and the insert, delete, home, end, pgup, and pgdn keys, as well as the enter, / and numlock keys on the keypad. This helps distinguish a lot of keys that have the same scan codes or virtual key codes. Bit 29 is set if the alt key is being held down. Bit 31 is 1 for key up messages and 0 for key down messages. Bits 25 to 28 are not used. |_key_|already|alt key_|_not used_|extend_|_scan code_|_repeat count_| |_up__|_down_|_down_|(reserved) |_key__|__________ |____________| |__31_|__30__|__29___|_25-28___|__24__|__16-23____|___0-15_____| So for example, the repeat count is given by (data2 & 65535) and the scan code is is given by ((data>>16) & 255). Ok, now for programming. You usually want to keep track of what keys are up and what keys are down, especially the shift and ctrl keys. As for the alt key you can either keep track of its status yourself or use bit 29 in data2. I use both. The first for programming parallelism and the second to double check it. A 255 length boolean array using the virtual key code as the index is a standard way of keeping track of which keys are up and which are down. Clearing this array is all you usually want to do for WM_KEYUP, WM_SYSKEYUP. For WM_KEYDOWN and WM_SYSKEYDOWN, you need to test for the shift button (data1 == 16), the ctrl button (data1 == 17) and the alt button (data1 == 18) to update your boolean array and usually nothing else. For example, LRESULT CALLBACK WndProc(HWND hWnd, UINT uMsg, WPARAM data1, LPARAM data2) { switch(uMsg) {[tab][/tab]case WM_CREATE: <code to create menus> return 0;[tab][/tab]case WM_COMMAND: <code to handle menu selections> return 0;[tab][/tab]case WM_SYSKEYUP:[tab][/tab]case WM_KEYUP: keys[data1]=false; return 0;[tab][/tab]case WM_SYSKEYDOWN:[tab][/tab]case WM_KEYDOWN: if(data1>16 && data1<18){keys[data1]=true;return 0;} switch(data1){ <code to handle other keypresses> }[tab][/tab][tab][/tab]} // Pass all unhandled messages to DefWindowProc return DefWindowProc(hWnd,uMsg,wParam,lParam); } Although it makes sense to keep track of whether the shift, ctrl and alt keys are up or down, you cannot trust your determination in a winodowed program. The reason is that your program will only receive a key up message if your program is the currently selected window (in focus as they say). Therefore it is a good idea to verify the status of these buttons every few seconds or so with something like GetKeyState, for example. You pass the virtual key code to GetKeyState and it returns a nonzero integer if the key is down, unless it is a toggle key like caplock (in which case it returns 1 if the key is toggled but not down).
-
Software Copy Protection looking for ideas
mitchellmckain replied to mitchellmckain's topic in Programming
How does serial sign up work? Sound similar to what I am doing. I generate a number based on their computer info which they have to send me with their check then I can send them a number based on the one they sent be that will unlock registered features. Since the number I give them will not work on other computers copying will only result in unregistered copies on other computers. Does serial signup mean that you issue copies with their own unique serial number? How is copying discouraged? -
The windows updates seem to work great when you first install or you reinstall windows XP from scratch. But progressive updates are pain because they pile up like the neverending growth of law, beaurocracy, landfills and medical charges on hospital bills. I use a removable hard drive system for my system drive so I can switch operating systems. It also allows me to compare different installs of XP, and the differences in what windows updates are added by the automatic download system is amazing. I guess that a major flaw in the system is that it only installs new updates when it really needs to replace bad and obsolete updates as well.
-
I had an older version of Norton and didn't want to pay because I wasn't impressed and the older was causing problems so removed it. Spybot S&D is great and add to that "Hijack This". Built in firewall is great if you want something trouble free, invisible and useless. Replace that with zonealarm. Regedit.exe is an absolute must. Get used to using this. You can back up portions you are not sure about, before removing them. msconfig.exe one is the best early warning systems, since the startup list is usually the first thing that malware and worms try to modify. If I am worried I check this right away and feel a lot better when it is clean. Brains+Google = Absolute Power.
-
I just put a computer info based algorithm in my simulator today. I am finally moving toward making a version of my relativistic physics of spacefilight simulator available on the net with some features being registration activated. Looking for a web site is part of it.My first idea was to somehow get the physical location of the file on hard disk (haven't found a way of doing that yet either) as a basis for unique registration but then a disk defragmentation would invalidate the registration so that will not work, unless there is some way of making the file un-movable like some system file are. Although that sounds a bit unfriendly.Any thoughts would be welcome.
-
I have been hit several times with worms, adware and spyware. The most difficult job is cleaning up afterward and for that I have downloaded many: Spybot Remover, Adaware, hijack this, registry mechanic, and tds-3.For a long time I only had Norton antivirus, then I finally installed free Zonealarm, and I was impress by software that actually did something. All Norton ever did was make notices that I infected with something (which interfered with countermeasures). After my most recent invasion there was damage I never could track down until my Norton subscription ran out and I uninstalled the thing. My residual problems vanished. I have not yet replaced Norton AV, and I am only considering something free since I feel somewhat safe behind ZoneAlarm. I don't dare connect without it! I should say that I am also extremely cautious about web surfing, visiting most only when on high security setting of internet Options. I wont touch MS Outlook, Outlook express, or instant messengers either.