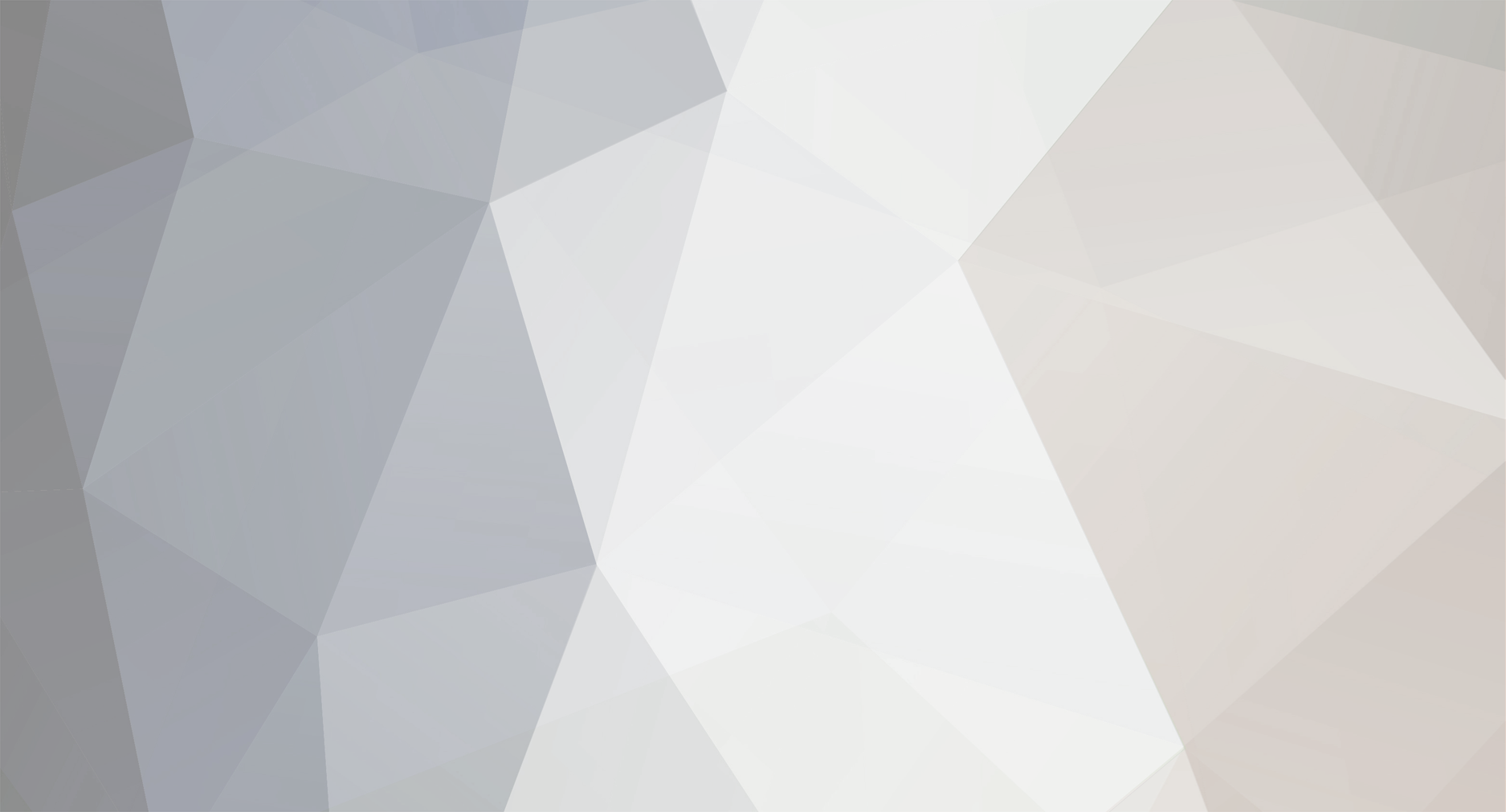
iFail
Members-
Content Count
8 -
Joined
-
Last visited
About iFail
-
Rank
Newbie
-
Here are my 2 cents... #include <iostream>using namespace std;int main() { double num[99], avg, range, median, mode; int i, j, n; char s; cout << "How many numbers would you like to enter? (max 99): "; cin >> n; for ( i = 0; i < n; i++ ) { cout << "Enter a number: "; cin >> num[i]; } cout << "\n\n"; /*** Here i added several lines to calc the mean**/ double prevNumber = num[0] - 1; int timesUsed, mostUsed = -1; for ( i = 0; i < n; i++ ) { if (prevNumber == num[i]) { timesUsed++; } else { prevNumber = num[i]; timesUsed = 1; } if (timesUsed > mostUsed) { mostUsed = timesUsed; mode = num[i]; }//end of meany stuff for ( j = i + 1; j < n; j++ ) { if ( num[i] > num[j] ) { swap(num[i], num[j]); //easier, ain't it?;-) //why not use STL sort/qsort? } } } avg = 0; for ( i = 0; i < n; i++ ) avg += num[i]; avg /= n; cout << "Would you like to display the numbers you inputted in order? (y/n) : "; cin >> s; if ( s == 'y' || s == 'Y' ) { //C languages are case-sensitive... for ( i = 0; i < n; i++ ) { if ( i % 3 == 0) { //means, "every third character..." cout << "\n"; } cout << num[i] << "\t\t"; //equally spaced-out numbers } cout << "\nRead from left to right; top to bottom..." << "\n"; } cout << "\n\n";/*** Range -- easier, min/max -- removed unnecessary code (see the outputs)**/ range = num[n-1] - num[0];/*** Median -- take the middle score or the avg of 2 middle scores**/ if (n % 2) { median = num[n/2]; } else { median = (num[n/2] + num[n/2-1]) / 2; } cout << "Minimum: " << num[0] << "\n"; //look at this cout << "Maximum: " << num[n-1] << "\n"; //and this cout << "Range: " << range << "\n"; cout << "Average: " << avg << "\n"; cout << "Median: " << median << "\n"; cout << "Mode: " << mode << "\n"; system("PAUSE"); return 0; }
-
How Can I Create A Gui Using C++ I need easy steps
iFail replied to negativezero's topic in Programming
I cannot post any of the books that I used, because, unfortunately, they are not in English. Sorry. And even if they were, I'd have a hard time trying to find them anyway (backups...) So I'll just post my code here, for creating a basic window. #include <windows.h>#include <windowsx.h>LRESULT CALLBACK WindowProc(HWND hWnd, UINT message, WPARAM wParam, LPARAM lParam);int WINAPI WinMain(HINSTANCE hInstance, HINSTANCE hPrevInstance, LPSTR lpCmdLine, int nCmdShow) { HWND hWnd; WNDCLASSEX wc = (WNDCLASSEX) { sizeof(WNDCLASSEX), CS_HREDRAW | CS_VREDRAW, //size, style (WNDPROC)WindowProc, 0, 0, hInstance, NULL, //WndProc, {cls,wnd}Extra (WTF?) LoadCursor(NULL, IDC_ARROW), (HBRUSH)COLOR_WINDOW, //cursor, brush NULL, "Window Class 123", NULL}; //menu, name, def icon RegisterClassEx(&wc); hWnd = CreateWindowEx(0, "Window Class 123", "Stupid window requires so much code...", WS_OVERLAPPEDWINDOW, 0, 0, 640, 480, NULL, NULL, hInstance, NULL); ShowWindow(hWnd, nCmdShow); MSG msg; while(GetMessage(&msg, NULL, 0, 0)) { TranslateMessage(&msg); DispatchMessage(&msg); } return msg.wParam;}LRESULT CALLBACK WindowProc(HWND hWnd, UINT message, WPARAM wParam, LPARAM lParam) { switch(message) { case WM_DESTROY: { PostQuitMessage(0); return 0; } break; } return DefWindowProc (hWnd, message, wParam, lParam);} The code is mine, but you can use it freely (coz it's so common it would be stupid to copyright it) -
Just a general question...Why does it have to be MSDOS, and not the cmd.exe? The commands are pretty much the same, but do not require any additional hardware to buy, or anything to install...This is going to be a bad question, but: why not learn GNU/Linux?
-
Heh, I think the best antivirus is the user itself :)I use both Windows XP and different Linux flavours, quad-booted from one machine -- mad, isn't it? :)Anyway, for linux I installed ClamAV to use from time to time -- e.g. cleaning the USB drives before usage. For Windows, I prefer avast. Does not use up much memory, detects potentially dangerous stuff that others have failed to detect (or ignored?).So far, I had only one Windows reinstall due to viruses -- I was stupid enough to turn the "On-access protection" off. XDAnd I would encourage everyone to use the antivirus protection software, to help prevent the spread of viruses. Even in my school, the network is infected, and the tech admin does not bother to update the definitions. Some of them are 6 months old, and I was trying to avoid using those computers ever since it killed a gig worth of my own programs (backups are a must, too)
-
It'd probably be even worse than Vista... Those Micro$oft guys never learn. I use WinXp SP3 for gaming/study and the likes, however for programming and trying out stuff I use Gentoo Linux with VirtualBox onboard. Though, I still prefer Wine over VBox... Yes, I know that Gentoo is for developers only, or for servers, so that they have better security. But systems bundled (is this the right word? plz correct me) with Gnome, KDE or xfce pull in a LOT of dependencies that cannot be solved by unchecking the needed components. OpenSuSE is prob the best one in this sense (I used SuSE 10.something, not the Open one yet), as the YaST manager checks for dependencies before the install. Hell... that was a relatively short post. Hi all!)) [that was my 3rd post]
-
Man, I'm gonna sound a lil biased, but here is the truth:C++ is the language that gives you the best understanding of how your program runs. If you do not understand that process, you are totally screwed [/colloquial language] On a more serious note... I'm 15, too. "Fluent" in Pascal (Delphi), ActionScript(Flash), C/C++. Already had about 5-6 years of serious programming experience. What is different between those languages? Only the conventions -- the rules that spell out HOW you are supposed to write stuff. Even C/C++, java and Flash use similar syntax structure. Dev-C++ is the best IDE (C++ being the language), IMHO. However, I am moving to Code::Blocks for better flexibility/convinience now. So far, I suggest using DirectX with C++ to create games. Wayne, you can try directxtutorial.com for some basic overview of HOW a simple game looks like. I know, it IS scary, but quite simple. It's like learning a new language (speaking, not computers ) -- you get better at it if you practice. So, I'd suggest to you to get a copy of GameMaker and experiment with that. Or a Macomedia Flash Player. Or a similar product. For the least typing, I'd suggest Delphi with DelphiX component. Easiest professional(?) language/tool to master.
-
I suggest you look at some OpenGL books -- I believe they are relatively easier than the DirectX ones. Try "Premier Press - Beginning OpenGL Game Programming - 2004". Sorry, but I cannot post it here, though it is freely available as a torrent You can try DirectXTutorial.com as well -- it's a good source of info, though not a complete one. It's good for getting to know DX. I'm using it right now for my school game project For some easy drag-and-drop programming, you can try Delphi, with a component called "DelphiX". Haven't used it much. It is also important to know which platforms you use -- I assume Windows. That certainly means DirectX. You should also learn multithreading, if you haven't already. And I believe, C/C++ has the least bloated executable, and it is the fastest one. (i don't count assembler though ) Most importantly, do NOT start making games unless you have grasped most of the concepts of programming in your language. Try some challenges, too -- like TopCoder, or IOI tasks.