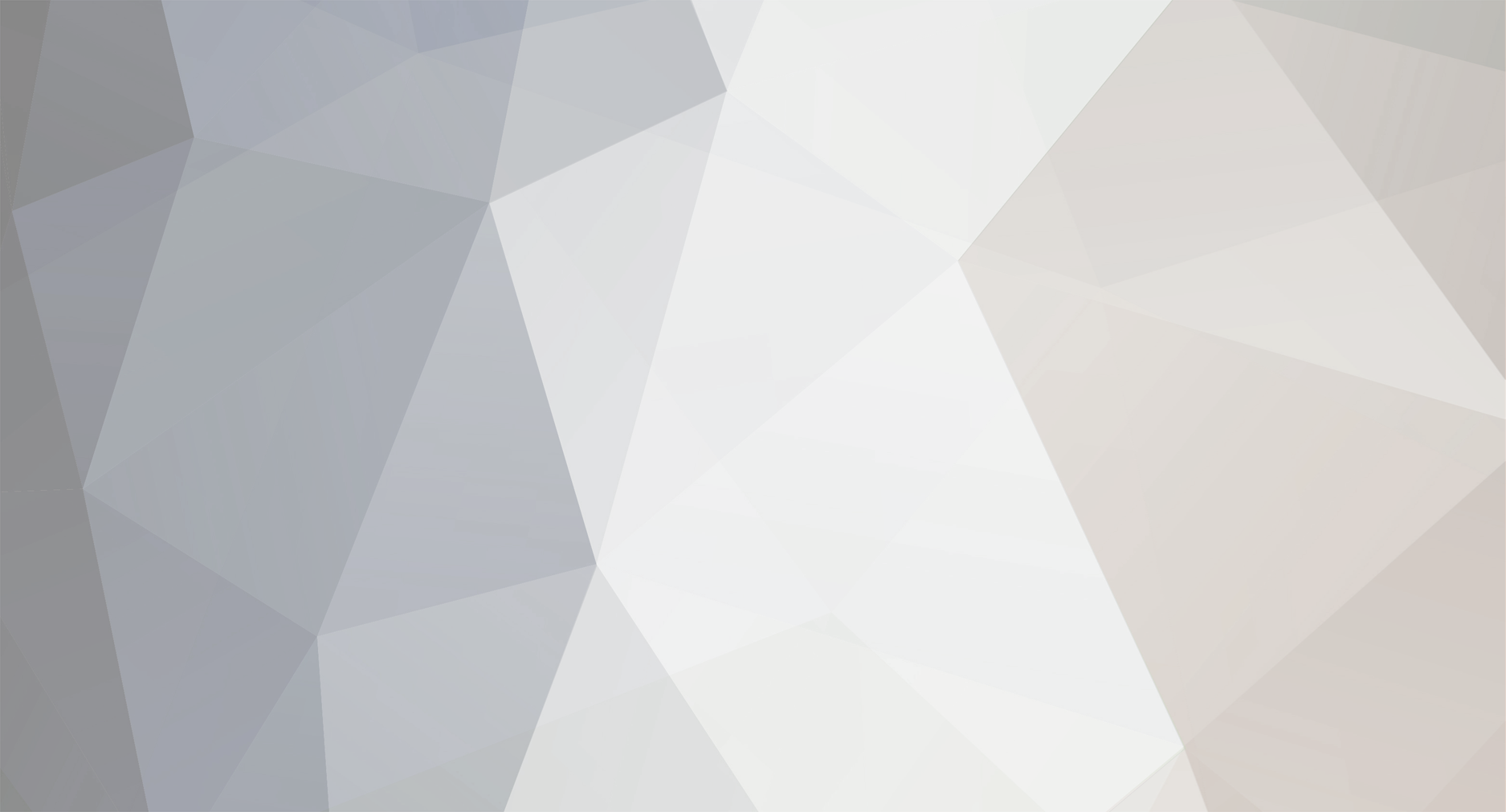
Codemaster Snake
Members-
Content Count
69 -
Joined
-
Last visited
Everything posted by Codemaster Snake
-
Download it from here! in html WELCOME TO AN EXCITING JOURNEY INTO PROGRAMMING WITH C++! This Tutorial is written for all those beautiful minds there who are interested in computer programming but don't know where to start. Well, You have just came the right place. This tutorial is for an absolute beginner. Since, I am writing this tutorial for a beginner I will only teach basics. HISTORY OF C++ The C++ language was developed at AT & T bell Laboratories in the early 1980's by Bjarne Stroustrup. It as originally known as 'C with classes' as two languages contributed to its design: C, which provided the class concept. C++ is an example of an object-oriented language. Other object-oriented languages include Java and Smalltalk. C++ language is a superset of C. Like the C language C++ is compact and can be use for system programming. It can use the existing C software libraries. (Libraries are collections of programs that you can reuse in your program.) C++ also has object-oriented programming (OOP) capabilities similar to an earlier language called Simula67. C++ is called a hybrid language because it can be used both as a procedural language like C or as an object-oriented language like Simula67. Other object-oriented languages include Smalltalk and Ada. C++ is a 'Programming Language' in which we will create 'Programs'. A program is a set of instructions that are written to carry out some meaningful task. In other words, you program a computer to do some work. This is done by writing a set of lines. This program is written in a specific language which a computer can understand. Since a computer is going to some work for us so, it is necessary for us to write this set of lines in a language which a computer can understand and interpret. This language is called 'Programming Language'. We will use C++ for this. You will need a C++ compiler to make your own program. I assume that you have 'Turboc3 IDE' and know how to use it. Before we start our programming let us try to have an idea about a c++ program. Let us take a real life example:- Suppose your mom asks you to bring 1 Kg of Apples from market. So, what would you do ? You will first take the money and a bag with you. Then you will then go to market and ask the greengrocer to give you a Kg of Apples. Then you will take them and get back to home and give Apples to your mom. As you can see, every thing is done in systematic way. So is done in c++. That's it. You are now ready to create your own programs. Now let us write our first c++ program : Program # 1 : -------------------------------------------------------------------------------- #include<iostream.h> void main() { cout << "Hello, This is my first c++ program."; } -------------------------------------------------------------------------------- This is a very very basic program. Save this code (those set of lines written is called a code) as filename.cpp. Compile and run it. When you run this program you will see following output : Now, Let me explain this code to you. #include <iostream.h> This is called preprocessor directive. This statement lets computer know that what functions you are going to use in your program (this is similar to including money and bag in your journey to market). iostream.h is a name of a header file which contains some functions in it which we used. There are lots of them. I have listed few of them below: string.h It contains functions used for string manipulation. conio.h It contains functions used for screen manipulation. stdio.h It contains some standard functions. fstream.h It contains functions used for file manipulation. dos.h It contains some dos related functions for e.g. #include <stdio.h> #include <dos.h> void main() is a function (it is called main function). Any word in c++ ending with '()' is called a functions. there are thousands of functions in C++ stored in their corresponding Header File. That is why we have so many Header Files. This is same as those books which are arranged subject wise in a library to avoid confusion. Even a computer can get confused;) { this is the starting point of a program (we also started our journey from our home). cout << "Hello, This is my first c++ program."; this statement tells computer to print 'Hello, This is my first c++ program.'. cout << is defined in iostream.h header file. That's why we have included iostream.h. You should enclose your message between " " otherwise it will give error (more on this later). Notice that semicolon at the end of that statement. It is called string terminator. Each statement must end with it. } this is the ending point of a program (we also ended our journey at our home). Each and every c++ program starts at main() and ends at main(). Next comes comments. // or /* */ anything written after this is ignored by compiler. for e.g.. // this is a single line comment. /* this is also a comment */ // this may be used if you want to give a single line comment /* this may be used if you want many lines to be commented*/ Some of you would be thinking that if compiler ignores comments then why use it? Well, these can be used in many ways. You can use them at the top of your program for future reference to know what your program is about just reading it which is better than getting to conclusion after reading 7869 lines of code;). We can modify our code as : Program # 2 : -------------------------------------------------------------------------------- #include <iostream.h> /* this is called preprocessor directive. it tell compiler what functions we are going to use in our program*/ void main() /*this is a function main(). Each and every program must have this.*/ { /*this is the starting point of our program.*/ cout<<"Hello, This is my first c++ program."; /*here we write our instructions which will be followed by computer.*/ } /*this is the ending point of our program.*/ -------------------------------------------------------------------------------- Output of program # 2 will be: Difficult to understand, don't worry it will take time to get familiar with that. For now just learn it by heart. Now let us do something else. Program # 3 : -------------------------------------------------------------------------------- #include<iostream.h> void main() { cout << "My Name is Neeraj."; } -------------------------------------------------------------------------------- Output of program # 3 will be : When computer executes our program it searches for main() function then reads first line of main() and executes it. Then it reads second line and executes it, then it executes third and so on. In our program we have only one line in main() so computer will read it and executes it then it encounters } and program in then ended. Let us elaborate our code : Program # 4 : -------------------------------------------------------------------------------- #include<iostream.h> void main() { cout << "******************"; cout << "\n"; cout << "My Name is Neeraj."; cout << "\n"; cout << ******************"; } -------------------------------------------------------------------------------- Output of Program #4 will be : Nice graphics;) Let us take a look at the code of above program. You would be able to see some four new statements. Well, don't panic that's nothing. Let us understand what's going on : cout << "******************"; This prints ****************** cout << "\n"; This is called new line character. It starts a new line cout << "My Name is Neeraj."; This prints My Name is Neeraj. cout << "\n"; It also starts a new line cout << ******************"; This prints ****************** If the above code is : -------------------------------------------------------------------------------- #include<iostream.h> void main() { cout << "******************"; cout << "My Name is Neeraj."; cout << ******************"; } -------------------------------------------------------------------------------- Then the output will be : See that difference. Try Printing Something else. Remember more you practice more you learn. -------------------------------------------------------------------------------- Practice Questions #1 Write a program to print your name on the screen. #2 Write a program to print following : ************ ****** ** #3 Write a program to print following : * * * ******** -------------------------------------------------------------------------------- Home Chapter # 2 Chapter # 3 Chapter # 4 -------------------------------------------------------------------------------- Have Suggestions? Then, Email Me If you like this tutorial then please vote me at Planet-Source-Code -------------------------------------------------------------------------------- I created this tutorial back in 2003... just want to share those momments.. Notice from BuffaloHELP: We appreciate your tutorial, however, excessive copy of published material is not part of quality contribution to our forum. Refrain from copying so much but express with your own, genuine words when making your post in our forum. Thank you. Edited the post as said!
-
Open Source Vs. Closed Source
Codemaster Snake replied to Codemaster Snake's topic in General Discussion
I would like to answer the third question!You are somewhat right that the millions of projects that start out at sourceforge.net don't go anywhere beyond the first step. But everything is also not accepted by the world! You can't just make everybody love your project. It's upto them whether they like it or not. This is true for closed source also.... there are so many dead commercial products which nobody bothers to even think of. Look around Linux changed the world alot I mus' say. It was started as a research project (UNIX) and Mr. Linus launched it for public. Now almost every web server runs on Linux. -
Smokers Or Non-smokers: Who?s More At Risk?
Codemaster Snake replied to Saint_Michael's topic in General Discussion
I must say that I am also a heavy smoker. But I always some there where nobody's around! I also used to hate smoking earlier.... But the irony is that I am also a heavy smoker now! -
Open Source vs. Closed SourceOpen Source: What is it?Well it's applies to the software that is freely avaliable to everybody. But, that doesn't mean that you can everytime modify the source code and give the new compiled software the name you wish.There are many Licenses that apply to the open source. You may get GPL, LGPL etc. open source means the software product you are using is available with/without (yeah! that's true some can also charge you!) cost and the source code if you want. This means that you can ask the developer for the source code of the software product. Then according to the licenses you can/cannot modify the provided source code.Some open source software products: * MySQL * XOOPS * agrosoft mail serverClosed Source: What is it?The Closed source is bassically applied to those software that is always available at a price as in case of Windows Operating System or to the shareware products like game demos.Generally the developer creates his own terms and conditions for the software and how it can be used. This license in many cases is knows a EULA (End User License Agreement). You can ask for the source code or cannot modify the work of the developer. If you do that you'll go to jail!Which is Better?This depends. Sometimes open source is better and sometimes closed source is better. Generally people go for closed source because in that case the developer provides you the support and gurantee to the software. which is not provided in the case of open source.Which is more secure?I would say that open source is more secure! Yeah I know this is an open invitation to a hot debate.My point in the favour of open source: That an open source software product is always available with it's source code. And there are millions of progrmmers around the world! if some one finds a bug then he/she can modify the work and release it again. This is why open source software updates sp frequently![[Original work]]
-
You can recover data from your screwed up disks by using the following simple method!1) Get something like nail-polish remover from your mum or a deo from your dad :P2) get some cotton and wet it with whatever you got from above.3) rub the transparent plastic side of the disc in a circular motion from the center to outward direction.4) rub it getnly but yet strongly. I mean don't make more sctraches.5) put it in the disk reader and VOILA! it runs.This works for me maybe it can help you also.... I recovered WinXP SP2 CD like this.The bottom line of the process is to remove the sctraches by rubbing.
-
Do I Need To Be Active In The Forums? [resolved]
Codemaster Snake replied to Codemaster Snake's topic in Web Hosting Support
Thanks Very Much.But don't you think that rules are pretty strict here! -
Do I Need To Be Active In The Forums? [resolved]
Codemaster Snake replied to Codemaster Snake's topic in Web Hosting Support
Is there any minimum number of credits that I would have to maintain to be alive? -
Do I Need To Be Active In The Forums? [resolved]
Codemaster Snake replied to Codemaster Snake's topic in Web Hosting Support
Ho much do I need to post to have my website running -
I use Ubuntu LInux mainly.... I don''t use Windows much. I have everything that's needed, in UBUNTU.
-
Internet Explorer 7 The Greates Browser ive ever seen
Codemaster Snake replied to OmArEmAd's topic in Software
IE7 is good but not as firefox...Firefox has won many awards and is considered to be the safest browser till date...This is because FF is opensource and IE7 is not. When you have a open source soft. every can edit it's source code. and on finding a bug millions of developers across the world update it. FF gets updaetd very frequently.. -
What Country To Do You Live In, And Rate It.
Codemaster Snake replied to Fu Still Better's topic in General Discussion
IndiaI'll rate it 7.5/10Good Things: Fastest growing economy. Becoming one of most powerful country of the world. Has world's third largest Army. Great Culture. Has evry kind of terrain: Sea, Pleatue, Mountains (Great Himalayas), Valleys, Plains.Bad Thing: Curruption. I think it's the only thing that is responsible for evry other bad thing. -
How Turbo Charger Works Turbocharger
Codemaster Snake replied to musicfreak's topic in General Discussion
SOURCE: http://www.guyshandbook.com/cars/how-does-rbocharger-work Notice from Plenoptic: Giving a link to the source is nice but copying the information word for word is wrong. You must put into quote tags ANYTHING copied from another source or ANYTHING not in your own words. -
Do You Have Stairs In Your House? I am protected.
Codemaster Snake replied to Velveteen's topic in General Discussion
This is a silly question to be answered :PBut I love to answer them...I have a Duplex with two stairs... One leading to upper floor and one is used as a fire exit! -
PHP files are special script files that can only run on those web servers that have PHP installed on them.First look for a web server that have php support nstalled on it. then simply upload and view it in the browser...If you want to develop in PHP and want to be able to see PHP in your browser then You will have to get three things: * A Web Server Software ( IIS or Apache ) Apache is good. * PHP Scripting Support.You can dowload them free of cost from the net and install it on your computer.... You can then be able to use your computer as a web server and view the PHP files on it...
-
Hi all!I am Codemaster Snake!Well I am Comp. Engg. Student. I am looking for a free web host to host my site!My Site will be focussed on OS Development! I have huge amount of content with me... that can really help you people... I'll share all of it!
-
The Best Browser In Your Opinion for WIN32/64 systems
Codemaster Snake replied to jacob's topic in Software
I use Internet Explorer 7 on windows Vista!Don't worry dudes I have the Original Copy! I bought it from Micosoft!I also use Firefox 2.0.0.5 sometimes... I used to use it before IE 7 was there... But I now use IE 7 -
C is structured , C++ is Object Oriented.You can see C as a Subset of C++. C++ hosts every feature of C with some added freatures like:* Classes* OOP Features* Better Error Handling* More Data securityetc...