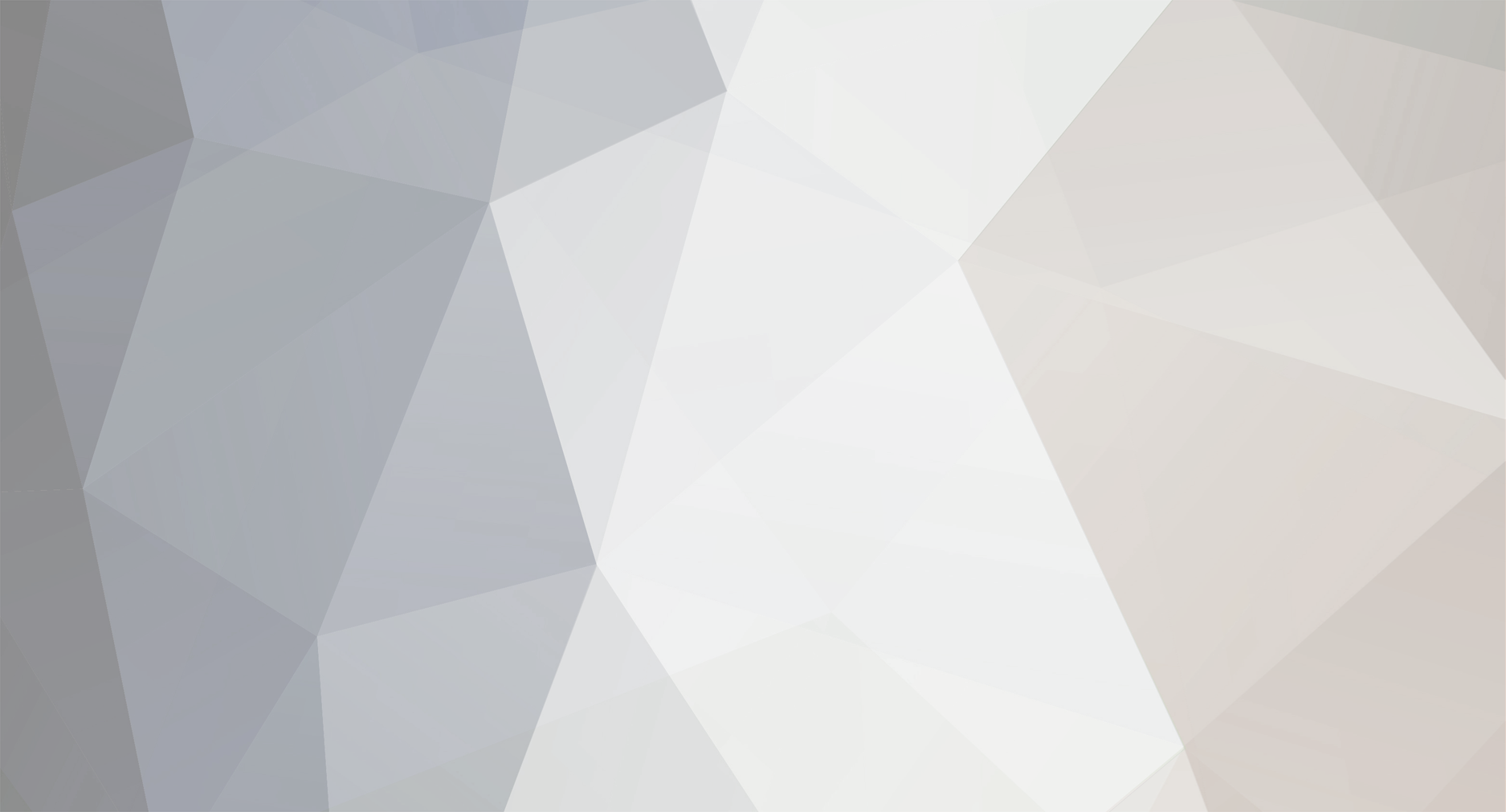
AdityaKar
Members-
Content Count
54 -
Joined
-
Last visited
About AdityaKar
-
Rank
Member [Level 1]
-
AdityaKar started following Article On Concurrency In Java, Increase Your Bandwidth By 20%, My Windows Shuts Down By Itself After Sometime and and 5 others
-
How can I increase my hard disk speed in Windows XP/2003? test and post comments.. Notice from OpaQue: Post found to be copied
-
Increase your Bandwidth by 20% Windows uses 20% of your bandwidth! Get it back A nice little tweak for XP. M*crosoft reserve 20% of your available bandwidth for their own purposes (suspect for updates and interrogating your machine etc..) Here's how to get it back: Click Start-->Run-->type "gpedit.msc" without the " This opens the group policy editor. Then go to: Local Computer Policy-->Computer Configuration-->Administrative Templates-->Network-->QOS Packet Scheduler-->Limit Reservable Bandwidth Double click on Limit Reservable bandwidth. It will say it is not configured, but the truth is under the 'Explain' tab : "By default, the Packet Scheduler limits the system to 20 percent of the bandwidth of a connection, but you can use this setting to override the default." So the trick is to ENABLE reservable bandwidth, then set it to ZERO. This will allow the system to reserve nothing, rather than the default 20%. works on XP Pro, and 2000 other OS not tested
-
Lucas Electronic Magnifier This half bottle shaped gadget is rather fun and unique. Its the Lucas Electronic Magnifier which magnifies text or images and shows it on your television. Put a newspaper or a book under it, connect it with the TV and see the magnified image on your television. Lucas features a 1/4″ CCD color camera and a control to handle magnification ratio. However, the ratio also varies with the size of television. The Lucas is made up of acrylic resin, aluminum and glass lens for camera. The price tag is $329 for the whole set which comes with the magnifier, AC adaptor and an instruction manual.
-
Article on Concurrency in JAVA I've been writing this one for the last few days and I know this has turned out to be a long article but go through it you might find something in it for you. Concurrency A microprocessor can only execute 1 instruction at any point of time. Therefore to increase the productivity the concept of concurrency or parallelism is used. This is similar like you watch TV and also eat, sleep and also breathe etc. Therefore by using concurrency we can increase the throughput of the processor. But the way a processor handles concurrency is different than our body handles concurrency. The body has many different neurons to pass signals to each other and therefore support parallelism but since a processor donât have those and it can execute only 1 instruction at any point of time therefore parallelism is handled by software and sometimes hardware ( we will talk about it shortly ). Concurrency through software A software handled concurrency requires careful coding for the different resources and their availability. This can be done through a programming language that has a rich set of APIs for this purpose, however most concurrency APIs do need support from the target Operating System so careful choosing of a programming language should be done. The general APIs used are POSIX Thread Libraries (C/C++) and the JAVA Threading API. POSIX libraries are hard to use while java is easier to use however I donât think thereâs any portability issue. Concurrency through hardware Actually this is only an illusion. The one with software is also an illusion but for a single processor, for multiple processor systems concurrency actually can take place. If anyone has idea about pipelining they would know about this. This kind of concurrency technique is implemented with use of hardware and software (compiler) in which the logic for implementing it is hardwired (canât be changed) into the processor itself. However this is another way in which the throughput of the processor can be increased but it is very much complicated and optimizations from compiler are often necessary in association with careful coding by the programmer himself to remove hazards and stalling as much as possible. Furthermore all the instructions canât be pipelined (I think!) . So this is very restrictive but can be quite fast. In this tutorial we will only consider software techniques for handling concurrency. Basic Thread Model For multiprogramming Operating Systems it is possible to run multiple programs concurrently but at any point of time the processor is working on only one of them. This time is so small that to us it looks like things are happening concurrently. The simplest way to do this job is to switch between the programs. There are different policies by which this switch between the programs can be performed and the OS handles. This switch between the programs requires quite a few amount of resources so full executable program are also called heavy weight processes. But the switch between the programs is not the basic switching mechanism , for example we have all played games, worked on programs with some animation or banner moving around it. These tasks are embedded within the program itself to be executed concurrently with other active components of the program like waiting for an input with the banner also flashing. These tasks are called Thread. So a thread is a basic unit of code that can be switched among other threads of a program which is in execution. Threads are also called light weight processes. Since few resources are required for switching between the threads. The resources that Iâm talking about are registers, data etc (you can find it in any OS book). Javaâs Support for Concurrency Java provides the Threading API for managing concurrent code. The class used for this purpose is java.lang.Thread. This class has all the functionality needed to implement threads and thus therefore acts as a base class for all the concurrent task handling classes. The Thread class has a method run () (public void run ()). All the concurrent code is written inside the run () method. The following example shows how public class MyThreads extends Thread{ int threadCount=10; int threadNumber;public MyThreads (int threadNumber) { this.threadNumber=threadNumber;System.out.println (âThread number â+ threadNumber+âis now createdâ); this.start (); } public void run () { while (true){System.out.println (this);threadCount--;if (threadCount==0) break; } } public String toString () // This is required for use in run () { return âThread Numberâs â+ threadNumber +âcount value= â+ threadCount; } public static void main (String [] args) { for (int i=0; i<10; i++) new MyThreads (); }} The above programâs description is given as below. We have created a class named MyThreads which is used to make 10 threads of concurrent execution. The threads are created inside of the main method. Just extending from the Thread class doesnât mean that threading will take place, to make sure the start () method of the Thread class is called. This method initiates the thread and in turn calls the run () method. Usually run () is coded with an infinite loop and then break out of it when a given condition occurs. In our case we break out of the run () when a threadâs counter goes to 0.When run () is over the thread is also complete. It is important to note that unless start () method is called you canât initiate or start a thread. Also calling run () directly from the thread object wonât help so start () has to be called if threading is to take place. NOTE: Many believe that sleep () method is required for threading. This is not true as can be seen from the above program. sleep () is useful when we need to temporarily halt a thread for some reason however its not necessary to use sleep () while writing threaded code. Also since threads are handled by the OS in a different way than variables and other objects they are NOT garbage collected automatically i.e. there is a reference to these objects somewhere (like in above example new MyThreads (i) these threads wonât be garbage collected even if they donât have any reference variable attached to them). To make sure that they are garbage collected we need to assign null to the reference which also kills the corresponding thread. Controlling Concurrency Concurrency can be controlled either by using yield () or by using the sleep () methods. sleep () just suspends the thread for a specified duration DURING WHICH IT STILL HOLDS THE CPU if it was in execution, while yield () relinquishes the cpu to some other thread waiting in the queue. Therefore sleep () causes waste of time and therefore should be used with precautions as it can slow down the processing of the program. The following examples show their use. Example 1 modified for sleep ()public class MyThreads extends Thread{ int threadCount=10; int threadNumber;public MyThreads (int threadNumber) { this.threadNumber=threadNumber;System.out.println (âThread number â+ threadNumber+âis now createdâ); this.start (); } public void run () { while (true){System.out.println (this);threadCount--;try{ sleep (10); // This method throws an //exception which has to be //handled}catch (InterruptedException){ System.out.println (âThread â+threadNumber+âis Interruptedâ);}if (threadCount==0) break; } } public String toString () { return âThread Numberâs â+ threadNumber +âcount value= â+ threadCount; } public static void main (String [] args) { for (int i=0; i<10; i++) new MyThreads (); }}Example 1 modified for yield ()public class MyThreads extends Thread{ int threadCount=10; int threadNumber;public MyThreads (int threadNumber) { this.threadNumber=threadNumber;System.out.println (âThread number â+ threadNumber+âis now createdâ); this.start (); } public void run () { while (true){System.out.println (this);threadCount--;if (threadCount==0) break;yield (); // This will halt temporarily this thread//and allows some other thread to use //CPU. } } public String toString () { return âThread Numberâs â+ threadNumber +âcount value= â+ threadCount; } public static void main (String [] args) { for (int i=0; i<10; i++) new MyThreads (); }} Daemon Threads These are the threads which have no life of their own. That is they can only remain active until a program is finished or they finish before the main program. These threads are usually those who do their job in background of the main program and their existence is forbidden when the main program terminates. Thus a program is over when there are no more NON-Daemon threads pending. The daemonhood of a thread can be set by using the methods setDaemon () (public void setDaemon (boolean)) and can be tested using isDaemon () (public boolean isDaemon ()).Example is shown below class DaemonThread extends Thread{ static int daemonCreated; public DaemonThread () { System.out.println (âDaemons Created till now=â+ (++daemonCreated)); this.setDaemon (true); this.start (); } public void run () { while (true) { System.out.println (âDaemons are left =â+daemonCreated); } }}public class ThreadEX2 { public static void main (String [] args) throws Exception { for (int i=0; i<100; i++) { new DaemonThread (); sleep (100); //let JVM handle exception } }} Setting Priorities among Threads This is generally required when we have some task that requires more attention by the CPU because of its importance in the application although it must still run in parallel with all other threads. This can be done using the setPriority () method of the Thread class. The JAVA rates priorities on a scale of 0-10 with 0 having the least priority and 10 the max priority. These are also defined as static final constants in Thread class. These are MAX_PRIORITY, MIN_PRIORITY and NORM_PRIORITY. Any other priority can be calculated by using these three defined constants. The syntax of setPriority is <thread object>. setPriority (<int priority>). Example: Try an example by creating different threads with different priorities and see how CPU handles the threads then. Another way to Threaded Code We can also write threaded code by not extending our class from the Thread class but by implementing the Runnable interface. This is required when our class has already extended some other class say JFrame for example and now we wish to add some threaded code to this class. Since JAVA doesnât support multiple base classes therefore an interface comes in handy. Even class Thread implements the Runnable interface. To write threaded code this way we need to create a thread object with the Runnable object as its parameter and then call the start () method of Thread class. Example is shown below: public class RunnableCodeExample implements Runnable{//This class is now a Runnable object also since it //implements the Runnable interface. Remember the //inheritance rule of up casting.Thread myThread; public RunnableCodeExample () {myThread=new Thread (this); // a new Thread//object is created with the Runnable object as itâs //argument. Since the object of this class is Runnable //therefore we give the argument as this in the // constructor of Thread. myThread. start (); } public void run () { int i=0; while (i! = 200) { System.out.println (âI =â+i); ++i; } } public static void main (String [] args) { new RunnableCodeExample (); }} In the above program first the RunnableCodeExample is made to be an object of type Runnable by implementing Runnable interface. Now Thread class requires a Runnable object. A Runnable object is nothing but a class which implements the Runnable interface and thus has the run () method defined for the Thread class. Thereâs just a single method in this interface so we need to define this only. PS: As an example you can try to make a frame (import javax.swing.*) with some controls and add to the same class a non-changeable display which shows the time / generates some random numbers through a separate Thread. You can also try to make this thread a daemon. Also itâs a good practice to extend from the Thread class if you can and implement the Runnable interface in case you canât. However if you intend to write some general purpose thread then you should use Runnable and let the end user programmer do the threading and start the thread. That is you just provide a Runnable object to the programmer. Sharing Resources By running the code above and writing some code on your own up to this point of time. However as you can see the threads run wildly unless they are control. But why should we control threads? Well its not needed always for example when to tasks run mutually exclusive of each other thereâs no need for controlling threads, like a thread displaying banner and a thread displaying time may not require controlling and thus can be let run wild without controlling. However if suppose they both try to access same resource and change itâs state than a conflict may arise. For example consider two/ more threads trying to write to same file, without some control this will be disastrous. Mutual Exclusiveness of Threads For threads to be controlled or access the resources available in synchronized manner the concept of mutual exclusiveness is used. This principle states that a thread has exclusive access to the resource and any other thread trying to access the same resource is denied and forced to wait. This concept is implemented by use of monitors. A monitor is a data structure that holds the accessing information of the resource and thus therefore can be reused. The mutual exclusive property is enforced at the monitor boundary since thereâs only 1 monitor for each available resource and the thread which has the monitor locked can have exclusive access rights to that resource. All other threads trying to access that resource are forced to wait until the monitor is freed by the thread that owned it and acquired by some other waiting thread. This way of handling threads is also more feasible since it allows a thread to use a resource as much as it wants to till it holds the monitor. Once the monitor of a resource is freed one of the threads in the waiting queue is allowed to capture / acquire the lock on the monitor and use it. PS: Threads are synchronized on the same OBJECT / RESOURCE. Remember this! The synchronized keyword is used to synchronize the code and the methods wait (), notify () and notifyAll () are used to control and pass messages to the synchronized code. An example of this is shown below. We are generating even and odd numbers in separate threads, but the order of generation should be 1 even followed by 1 odd or vice versa. Therefore controlling of threads is required. class EvenOddGenerator // This is the resource type.{private int oddCount; private int evenCount; private int startCount; private boolean semaphore; public EvenOddGenerator (int count,int startCount) { oddCount=count; evenCount=count; this.startCount=startCount; } /*This code is synchronized and therefore requires a monitor to access it*/ public synchronized void oddGenerator () { for (int i=startCount; i<oddCount+startCount; i++) { if (semaphore==true) { try { wait (); } catch (Exception e) { } } if (i%2!=0) { System.out.println("From Odd Generator ODD number="+i); semaphore=true; notifyAll (); } } System.out.println ("ODD Generator is Over!!"); semaphore=true; notifyAll (); }/*This code is synchronized therefore requires a monitor to access it*/ public synchronized void evenGenerator () { for(int i=startCount;i<evenCount+startCount;i++) { if (semaphore==false) { try { wait (); } catch(Exception e) { } } if(i%2==0) { System.out.println("From Even Generator EVEN number="+i); semaphore=false; notifyAll(); } } System.out.println("EVEN Generator is Over!!"); semaphore=false; notifyAll(); }}/*This is some thread which uses the resource. Remember our resource was EvenOddGenerator*/class EvenGenerator extends Thread{ EvenOddGenerator eoG; // This is the resource to use public EvenGenerator(EvenOddGenerator eoG) { this.eoG=eoG; this.start(); } public void run() { eoG.evenGenerator(); } }/*This is some thread which uses the resource. Remember our resource was EvenOddGenerator*/class OddGenerator extends Thread{ EvenOddGenerator eoG; //This is the resource to use public OddGenerator(EvenOddGenerator eoG) { this.eoG=eoG; this.start(); } public void run() { eoG.oddGenerator(); }}public class SynchronizedExample{ public static void main(String [] args) { EvenOddGenerator eoG= new EvenOddGenerator(11,100); // create a single resource /*The new threads will now access the same single resource in a synchronized manner.*/ new OddGenerator(eoG); //argument is same resource new EvenGenerator(eoG);//argument is same resource }} This example demonstrates the use of wait, notify and notifyAll methods. These methods can ONLY BE CALLED from a synchronized block of code otherwise you will get an exception. The purpose of these methods is as described below: Wait (): This method just makes a thread halt with CPU also being taken away from the thread from which this is called. Therefore any other thread(s) waiting in queue may get the CPU for execution. A thread which is waiting must be notified by some other thread in execution. This makes the waiting thread a part of the ready queue (waiting to get its hand on the CPU). A thread in which wait () is called is also called a blocked thread and only some other thread in execution may wake it up, however a thread can block itself while itâs running. Notify (): This method makes the thread that called the wait () method recently a part of the ready queue. That is it only wakes up only 1 thread that was blocked. NotifyAll (): This method wakes up all the threads which were blocked and makes them part of the ready queue. PS: THE SYNCHRONIZED METHODS CAN ONLY BE CALLED BY A THREAD WHICH CURRENTLY HOLDS THE MONITOR,ALL OTHER THREADS ARE FORCED TO WAIT EVEN IF THEY WISH TO CALL A DIFFERENT METHOD FROM THE ONE USED BY THE THREAD IN EXECUTION. For ex: in above example if EvenGenerator is the Thread in execution and holds the monitor only it can access both the synchronized methods the other thread canât, even if they call different methods like above. However non synchronized methods can be called since they donât require a monitor.So therefore when you need to do synmchronization just make a class(define a RESOURCE ) and put all those methods that are synchronized. Make an object of this class (Actually make a resource now ) and share this object among threads to achieve synchronization. Sometimes itâs needed that some part of the method is available to all the threads in execution however some part is available to only that thread which currently holds the monitor for the resource. Therefore concept of synchronized blocks is used. A synchronized block is same as a synchronized method except that some part of that method may be accessed by any thread. Since a resource is needed therefore syntax of synchronized block is Method declaration (without synchronization keyword) //âŚâŚcode synchronized (<synchronized resource>) { âŚâŚâŚâŚ synchronized code } Some Questions that you may want to try outâŚâŚ 1.) Create 500 threads that do nothing just write an infinite loop in the run method. Code for the finalize method and show how many threads have been garbage collected. Donât assign reference to any so that garbage collector is called. You may also try to call the garbage collector explicitly what happens then? 2.) Write a program to add odd numbers and even numbers in separate threads. The threads should be supplied the count of numbers to be added and a start count. 3.) Write a program to add numbers divisible by either 4 or 6 in separate threads. The threads should be supplied the same info as in the above program. Note this is different from above since a number can be divisible by 4 and 6 at the same time. 4.) Try the producer consumer problem. Is there any other way to do this problem? If there is why not use it in all synchronization problems? (Hint look in I/O streams to find the answer). 5.) Implement a multithreaded Server/Socket application which can handle multiple connections simultaneously. Server/Socket Programming Java provides a way to handle interconnections between computers through the use of its network API. The classes described here are part of the java.net package. Java.net.ServerSocket this class defines a server. This class has all the functionality needed to implement a network connection and create a server side implementation. The constructor of this class takes a port as parameter. This port is then used to establish connections with the clients. Server is created as /*this throws an exception which must be dealt with in case a ServerSocket at the port specified couldnât be made. Also make sure the port number is above 1000 since below that the OS uses for different purposes so Java might not be able to create a ServerSocket there*/ ServerSocket server= new ServerSocket (int port); Once a server is created successfully you need to make the server listen for connections at the port. This is done by the accept () method of ServerSocket class. The accept () method is blocking therefore it will wait for the connection to occur. On successful connection it returns the client as a Socket object. Socket client= server. accept (); Once a connection is established the messaging can be done by acquiring the input/ output streams of the client and the server components. This can be done using the getInputStream () and getOutputStream() of the Socket class. In order to establish a multithreaded server you must listen for connections more than once and as soon as the connection is made a separate thread must be made to handle the messaging. Therefore something like this should be done in the server side coding. While (true) { Socket client= server. accept () ; new ClientThread(client); } This ClientThread is what you need to code. We have passed the Socket object in this because the thread must have some way of getting the I/O streams. You can also pass I/O streams as parameters but a Socket object has a bit more info like the InetAddress etc, look up the JDK Docs. A client side coding just requires to make a connection to the server which is running. This can be done using the Socket class like this. /*This also throws an exception if the Server is not present/connection isn't made for any reasons*/ Socket client=new Socket(âURLâ, int port); The URL is a string which tells the location of the server and the port is where the server is listening for the connections. This is same as the port given in the ServerSocket while creating server. For testing on the same PC you can use localhost or a fully qualified InetAddress. Once this is done you can pass messages to server and server can message to you. But you must understand the I/O streams for implementing this thing. For any methodâs description look up JDK docs. The commonly used functions are as follows InetAddress.getByName (âString urlâ); ObjectOutputStream.writeObject(Object); Object ObjectInputStream.readObject(); Similarly for other I/O streams and Readers. Readers are useful only when strings are sent. This means pure Strings and not anything wrapped inside your object. Otherwise readObject () and writeObject () must be used. Also in case you are using your own objects for message passing make sure they are serialized so that you can read them exactly on the other end. The class Socket is not Serializable so canât put it inside your class that you wish to make serializable. It wonât work. PS: To find out wether a class is serializable use the serialver tool provided by JDK. On the command type serialver <fully qualified class name> if the class is serializable it will show a serializable number for that class otherwise a string that the class is not serializable. Example use serialver java.net.Socket and use serialver java.util.Vector.
-
Very Easy But Very Dangerous Virus
AdityaKar replied to me-here1405241520's topic in Security issues & Exploits
Ok first of all this is not a virus even in the lamest terms. This is just a DOS batch file. You can also save the file as .bat file and the functioning will be the same. This file won't erase anything without confirmation from the user. You've tried to delete just a single file thats why it didn't ask for confirmation from you. Try deleting a folder with this file and you will get the following message in a DOS window: erase c:\<directory name>c:\<directory name>\*, Are you sure (Y/N)?Unless you press 'y' nothing will happen. A virus, by definition, is not just supposed to damage a computer, it is also supposed to spread on its own. And this file can't even damage a computer without affirmation from the user. I wonder who would be dumb enough to affirm the destruction of his own computer. Atleast use "echo off" before the erase statement to hide the "erase c:\windows" command being displayed. And by the way, I think you haven't ever used Linux. Otherwise you would know that Linux doesn't support DOS commands and neither does it use the Windows/DOS filesystem. -
I did read about this phone a couple of months back on allaboutsymbian.com . The phone has excellent features for corporate users and a brilliant display. But the keypad doesn't look as comfortable as that of Communicator 9500. Moreover, the phone is massive in size! And it doesn't have touchscreen either, which is essential for a PDA. I would prefer the SonyEricsson P910i instead. Its a true PDA cum mobile phone. I have never liked the Blackberry and neither does this phone appeal to me. The lack of camera is also a drawback. But then I'm a student. The demands of corprorate users from a phone are much different. Here's how the phone looks like: For a detailed preview of the phone go to: http://forums.xisto.com/no_longer_exists/
-
Video Editing Tutorial (using VirtualDub) Before I start with this tutorial please download VirtualDub from VirtualDub.org. Many of us want to save just a small portion of a video, discard unwanted scenes from a video or add a logo of your choice to the video. Its very easy in virtual dub. First open the video in virtual dub. Virtual dub opens all kinds of video files with exception of the wmv and asf format as these are patented by microsoft. But you can find a version which opens asf too on doom9.org . Opened Video The bottom buttons are the controls for the video playback and editing. There are play, stop, forward, rewind, jump to last and first index, jump to previous and next key frames, scan for scene change and frame markers.First of all a little knowledge on how video is compressed in the mpeg format. The video when uncompressed, stores each and every frame in the plain rgb(Red Green Blue) format. But there are many redundant frames in any sort of video which are viewable by us. The mpeg format truncates those duplicate frames and stores only the information on the pixels that changed from the previous frame to the next frame. The first frame is known as the key frame. But the scenes are all not redundant but change after some time totally. So when the scene changes, a new key frame is added to maintain the compressed size and quality of the video file. Now the frame markers are used to select the no of frames which are to be deleted. Select the frames using the frame markers and the progress bar above the buttons. The mark in, mark out and the selected frames can be seen in the picture. Press delete to delete the frames. You can follow the same procedure to delete all the unwanted frames. This comes in very handy when you need only a song from the entire movie. Still the video needs saving. To save the video, there are two ways. First a direct stream copy and next the full processing in the video modes option. The former just copies the frames as it is to the new file excluding the ones you deleted but the latter requires for the compression format before save. Direct stream copy is not compatible with some formats and hence virtual dub might give you an error. In that case choose full processing mode and In this mode you can save it to any format you like. Choose the compression format before you save. Save Window In this window you can also add the saving process to a batch job and can save multiple videos with the virtual dub's job control. Also you can break the video so that it fits on a cd and so on. Virtual dub has a many plug ins which you can use to enhance your videos. They are available under filters. These filters can change your movie to black and white or can put a logo on the video which can be your own photo. Under video filters click on add and a window with all the inbuilt and added filter plug ins will be displayed. Video Filters When you choose a filter its configuration window will open after which the filter will be added to the list of added filters. The logo filter lets you display your own photo on top of the video. It asks for the image file for the image to displayed and the transparency and the screen justification can also be set from there. But the images are accepted only in the form of bmp and tga. If you don't have a picture in that format then you can use any existing image editing software to convert it into that format. Also don't forget to resize the image before you insert it, else the image may cover the screen with its size. Click on OK and save the video to get the job done. Filter Logo You can experiment with the other filters and have fun. There is one more cool thing that you can edit in a video. You can change the audio of the video file so that it plays your desired audio when you play the video. You can also choose to keep more than one sound track which is only supported in some formats. Windows media player can be used to choose between sound tracks when you view the video. To do this open up the stream list under audio menu and manipulate the sound tracks as you want. To disable the previous track click on disable and the audio will be deleted from the file. Use move up, move down to change the order of the tracks when you save multiple tracks. You can also extract the audio in the wav format by selecting the sound track and clicking demux. It will ask for a place to save the track and de multiplex the audio from the video to that location. Always remember to save the video after you have edited it as by not doing so you will lose all the editing you have done.
-
Yes pixel2life.com is the biggest tutorial site I have ever seen. It has more than 6,500 photoshop tutorials and for other graphics tools as well. I would like to add few more sites to the list: http://www.good-tutorials.com/ - They've quite a lot of photoshop tutorials divided into different categories. Though the tutorials are not actually hosted at their site. They are linked to different sites and forums. http://forums.xisto.com/no_longer_exists/ - Good number of tutorials and the tutorials in the Effects and Photography section are really useful. They also have lots of video tutorials. http://photoshopcafe.com/ - Large number of clearly explained tutorials divided into 6 categories.
-
I Give Up - How Do You Work This cPanel Thing?
AdityaKar replied to lonebyrd's topic in Websites and Web Designing
Well now I know what the problem is, you deleted the default index.html file and didn't replace it with your own file either thats why you are getting the '403 Forbidden' error. And the file that you put in the FTV Main folder is not named index.html so it won't show up just by accessing ftv.astahost.com/FTVmain/To do so, rename it to index.html. And moreover, put it in the public_html folder instead of FTVmain folder if you want to access just by typing ftv.astahost.com. And one more thing I realized, you have named the folder FTV Main and not FTVMain (there's a space in between). So to access the file where it is right now, you have to type http://forums.xisto.com/no_longer_exists/ You have also made an 'index.html' folder, it is not needed. I said you need an index.html file. Just rename FTVPG1.html to index.html and put it in public_html and everything should show up fine. -
I Give Up - How Do You Work This cPanel Thing?
AdityaKar replied to lonebyrd's topic in Websites and Web Designing
Hi lonebyrd! Please tell me the following first: 1)Do you want your page to appear when someone accesses "ftv.astahost.com" or do you want it to appear when accessing "ftv.astahost.com/FTVmain/" ? 2) Did you name your HTML file index.html or did you name it something else? 3) Did you remove the default index.html file from public_html folder? I'm asking this coz the default Xisto page should appear when accessing ftv.astahost.com unless it has been overwritten. But that is not the case here. This might be the reason for the '403 Forbidden' error. Since you put your file under FTVmain folder, you can access it by going to ftv.astahost.com/FTVmain/ and not ftv.astahost.com. If it is named index.htm or index.html then it will be picked up automatically, otherwise you'll have to specify the name of the file such as ftv.astahost.com/FTVmain/filename.html -
Ever Wondered About QWERTY Keyboard Layout? layout
AdityaKar replied to amitbhandari's topic in Software
No, I don't think that is quite the reason. If at all the reason was to slow down the typing speed then the design layout would have never been carried forward to modern day keyboards. And even with the QWERTY(pronounced as kwer-tee) layout people can type at extremely fast speeds. I did a little search and I found out that QWERTY layout was introduced in 1872 on a typewriter by its inventor Christopher Shole. Thought the reason why this layout was selected is not exactly known, it is believed that in this layout the common letter pairs were put on opposite sides of the keyboard thus, making typing easier. Some other layouts have also been presented such as Dvorak, which claim to be superior than QWERTY. One interesting thing to note about QWERTY is that all the letters of the TYPEWRITER is on the top row of this design. -
Well you can get the whole list of services provided by google by going to https://www.google.co.in/intl/en/about/products/ or by clicking on 'more' on Google's main page. Also try out Google Labs to get a preview of Google services and products that are still in development stage. Google Labs
-
Google used to have the https://www.google.com/gwt/n link on its WAP homepage as Google Proxy. But its quite a pain to see the websites on your PC without any formatting. I think a better idea would be view the page in Google Cache (if the page you want to view is not updated regularly).If your ISP doesn't block the free proxy servers then you can simply use https://anonym.to/ for proxy. Its the most popular web-proxy around and if your ISP has blocked out free proxys as well(as is the case with organicbmx) then chances are you won't be able to access this one either.
-
Writing Interrupt Service Routines(ISR s) It is assumed in this tutorial that you have knowledge of the C language. Although something about pointers is mentioned but this is NOT a C language tutorial. So here we go. The Basics Many functions of the computer are performed by use of interrupts. These include the typing, printer, task switching etc to name some of them. Now how does these things happen? We are here to answer some of these questions. Pointers in C language: Pointers in C language are an amazing thing to work with and very dangerous also if you don’t know what you are doing with them. Now since you know C I assume that you must also have the basic knowledge of pointers. So we move on to the better stuff. As you know pointers can be made to point to just about anything but we are interested here in pointers to functions, why is that you will know shortly. Pointer to a function can be declared as shown below <return_type_of_function> (*ptr)(<arguments_of _function>) PS: The parenthesis around the ptr are necessary. Why this is necessary you will know when we describe the rule of reading what pointer declarations mean. Let’s see an example of this. Consider that we have a function named myfunc with prototype as ( void myfunc() ), now to declare a pointer to this function from the above rule will be like this void (*ptr)(). This declares ptr to be a type of pointer which points to a function that takes no arguments and doesn’t return anything. Note that the function this pointer points to hasn’t been told yet so this pointer is dangerous to use. Initializing the pointer is very easy just do ptr=myfunc; and we have the pointer ptr pointing to the function myfunc since the name of the function appears to give the address of the function where it is stored just like arrays where name gives the address of the array from where it starts. Similarly if we had a function with prototype as (int myfunc2(int,int)) the pointer to this function will be declared as int (*ptr)(int,int). Actually this pointer can point to any function with a prototype as that of myfunc2. I think you got the idea. Rule to read pointers declarations Well this rule is not some kind of a standard but it almost never fails however you are very smart people I think you will find something where this rule doesn’t apply. THE RULE: For any type of pointer declaration start from the name of the pointer variable then go to right and add up to the declaration meaning after that go to the left and add to whatever you just found about the declaration of pointer. You will be stopped whenever you find parenthesis or some terminate symbol like ‘=’. Let’s take a few examples to make this more clear 1.) int *ptr; The meaning of this declaration according to above rule. We start from name ptr then we go to right we find a ‘=’ so we move on to left and find a ‘*’ so the declaration upto this point is “ptr is a pointer…….” now since we didn’t find any terminators we continue moving left and find an ‘int’ so the declaration means “ptr is a pointer to an integer”. 2.) int (*ptr)(); Applying the above said rule we start from ptr then go to right and find a parenthesis. So we move to left and find a ‘*’ therefore till now “ptr is a pointer”. Now since we find a “(“ we stop moving left and move right and find “()”. Therefore the declaration till now becomes “ptr is a pointer to a function which has no arguments” we are stopped by ; so we move left again there we find an int so the declaration is “ptr is a pointer to a function that takes no arguments and return an integer value”. 3.) int *ptr[5]: starting at ptr move right we find “[5]” this means till now “ptr is an array of 5” we are stopped by “;” so we move left and find a “*” therefore decleration till now means “ptr is an array of 5 pointers” we continue moving left since there’s nothing to stop we find “int” so the full declaration becomes “ptr as an array of 5 pointers to integers”.I think you got what I’m saying. So how about you trying some examples … A) int* (*ptr)()b[b][/b]) int* (*ptr)(int*,char)c)int *[](*ptr)(int *[],char *)[/color]Back to writing ISR s With the pointer terminology clear now take a look what these pointers mean in the code. Well there are 2 kind of pointers a ) which we know b ) which our teachers never taught us. Actually there are 3 types. 1) near 2) far 3) huge Near Pointers: we all know these so there’s nothing to talk about them really. These are the ones that our teachers dared to tell us (thanks 4 that). These pointers are 2 bytes long always i.e. any pointer that you declared normally they are 2 bytes long. This permits only 2^16 values which implies that you can access only the code which is in your data segment (since 1 segment is 64KB = 2^16). Any attempt to access data will result in segment protection error from the OS. Far and Huge Pointers: We take both these two since they are common and differ only when some arithmetic is performed using these pointers. Yes I think you have guessed these type of pointers our teachers never dared to tell us so something must be special about them! Far and Huge pointers are both 4 bytes long. This means they can they can handle about 2^32= 2GBof memory locations!! Now since we don’t have that much amount of memory when we are real mode (when computer starts up) we are safe since only 1MB is available (only 20 address lines available instead of 32 present address lines). We can access the whole of memory when we are in protected mode. This mode gives the whole 2GB memory as a flat i.e. no segments are available when in protected mode. But since we are in real mode our max memory limit is 1MB and our memory is fragmented into different segments of 64Kb each in size. Ok. So now you will ask we have 20 address lines and since each segment is 64KB= 2^16 how do we put the correct address. Well this is already done for you in the processor. To access any memory location in the 1MB limit we first find the starting address of the segment then to this address (base address of segment) an offset of 16 bits is added. The way these addresses are added is a bit tricky. First the segment’s address is left shifted by 4 bits (padded with 4 zeros in the end) and then the offset is added to it. Now this is a full 20 bit address and processor use this address to locate a memory location in the 1MB limit. The shifting need not be done by us since processor does that for us we just need to supply the segment’s base address and an offset within that segment. All segments starts at some multiple of 16 also called a paragraph boundary. This is because for each 16 bit segment address it is first left shifted by 4 bits ( 2^4=16 bytes). One more thing where these pointers differ from each other is in the way they are stored. Far pointers are not normalized while huge pointers are normalized. Normalization means that they have most part of the address in the segment. This is not a problem if you will perform logical / arithmetic operations on such pointers but if you do then using huge will benefit you since the results will be same as expected while with far pointers you may get strange results. Huge pointer arithmetic is therefore more complex which requires macros and thus slows down the performance. Declaring far /huge pointers The Turbo C provides us the keywords far and huge to declare such pointers. Just add these keywords and declare these pointers like you normally do. Example int far *ptr; (this ptr is a far pointer to an int) int huge *ptr(this ptr is huge pointer to an int) Why do we need these pointers while writing ISR s? Well as I said before with normal / near pointer declaration we can’t access the code outside of our data segment. Since ISR s require us to go beyond our data segment we must use these pointers. Only far can be used huge may not be required since there’s not much in the data segment which we need to access. Using huge pointers we can access more than 1MBs of data in the data segment while 1MB when we use far. The Booting process of PC When we push the start button of the PC many things are done. First a POST is done which checks the computer for its resources after the check is performed the BIOS (Basic Input Output System) is given the control. The earlier versions of BIOS used to come with BASIC loaded on them so in case an OS is not found the BASIC used to run on the computer now this doesn’t happen and that’s why we see Disk Error message when no OS is found. The purpose of the BIOS is to make computer ready to support the OS so that is can start up. Its purpose is to load the interrupt routines and start video output in text mode. The interrupt routines used by BIOS are very basic and they don’t interpret the data its up to the OS getting loading to make use of that data. The interrupt service is loaded through an IVT (Interrupt Vector Table) this is done so that if any other routine is required for some interrupt instead of the one provided by BIOS we just need to change the values present in the vector table. This makes interrupt handling robust. Interrupt Handling Process Whenever an interrupt occurs the processor stops whatever it was doing and handles the interrupt. Each interrupt (256 in total supported by Intel’s processors) has an entry in the IVT which is made by BIOS. Each entry is 4 bytes long. Therefore whenever an interrupt occurs its number is multiplied by 4 to access the ISR location. These locations are located in the low memory area of the memory, thus to have access to code outside of our segment we need to use far /huge pointers. An ISR is just a module which replaces the entry currently in the IVT to itself. Since these modules already present in the memory don’t have any names therefore the only way to access them is by use of pointers to functions. TC provides the keyword interrupt for writing ISR s. This keyword may be different on different compilers and the code that we will compile is for REAL MODE only (16 bit code). For 32 bit code we have to switch into protected mode which I don’t know myself yet. Also the IVT thing works only in REAL MODE in protected mode this changes to IDT (Interrupt Descriptor Table) this is way 2 advanced to handle here. Things that we need TC 16 bit compiler (that IDE one) Functions setvect() and getvect() A big heart to handle the errors that XP will show you when you run these programs! The functions setvect() and getvect() are both defined in dos.h. They provide a neat way to set the IVT for an interrupt to our ISR. A small program will demonstrate this. [color=green]#include<dos.h>#include<conio.h>char far *scr=(char far*) 0xb8000000l; // Address of VDU for //monochrome displays use 0xb0000000lvoid interrupt our(); // define an ISR ourvoid interrupt (*prev)(); //define a pointer to the location in IVTvoid main(){ prev=getvect(9); // Save previous value in IVT in the pointer setvect(9,our); // Set IVT to our ISR getch(); // u know that!}void interrupt our(){ int i=0; for (i=0;i<4000;i++) { if(i%2==0) { if(i>127) scr[i]=i-127; else scr[i]=i; } else scr[i]=i%16; //(I think only 16 colors are present in text mode) } (*prev)(); // necessary unless u know everything this interrupt does.}[/color]The above program creates an ISR for the keyboard interrupt( number 9). When we are inside an ISR we can’t generate another interrupt since we can handle only 1 interrupt at 1 time. Therefore we have written directly to the VDU in the our ISR. Since there are 80 cols and 25 rows => (80 X 25 =2000) memory locations. Each row/col entry comprises of a 2 byte value( that's why looping 4000 times). The even byte (1st byte) gives the value present at that location while the odd byte (2nd byte) gives its color and other attributes such as blinking, intensity etc. For using the internal clock use interrupt number 8. Watch what happens then! We have called (*prev)() from inside the our ISR this is necessary since at this point if time we don’t know what actually takes place on pressing the key. Therefore its best to call the previously stored ISR after we are done for smooth operation. To compile as a CPP file use 3 periods(...) in the argument section of the functions declarations. However this is only useful when we are not interested in the values present on the stack. When an interrupt occurs the processor responsibility is to save its state so that it can resume from that point when handling of interrupt is over. By default the processor just pushes 3 values on the current stack. These are the flags, IP(Instruction Pointer) and the CS(Code Segment) register. However to successfully execute the program we need that all registers state be saved. This is done by the compiler for us. Use this command tcc –s <filename>. This will generate an ASM file with the same name as that of the filename given. Now if you take a look at the statement proc our far in this asm code you will see that all general purpose registers are pushed before any instruction in the ISR is executed. However there’s no mention of the CS,IP and flags but they are present on stack and their values can be changed. These changed values if any will be popped back into the registers when the ISR is over. PS: Intel specifies that you can’t change the value in the IP register directly however through stack this can be done easily. If this gives you some bright ideas please meet me to discuss that I have thought on it but some problems are there! Using Stack inside of ISR. To use stack we need to pass some argument in the ISR so that these values are available in that argument and we can use them. The best way to do that is to declare a structure in the order in which the values appear in the ASM code while pushing. Also remember that to use completely Stack values the IP,CS and flag registers have also to be present in the structure. The structure is shown below struct INTERRUPT { unsigned bp,di,si,ds,es,dx,cx,bx,ax,ip,cs,fl; }; Now using this structure we can get values present in all the registers . I have developed a program to just that when the user presses the keyboard (i.e. we use interrupt number 9) to display the values. [color=green]#include<dos.h>#include<stdio.h>#include<conio.h>struct INTERRUPT { unsigned bp,di,si,ds,es,dx,cx,bx,ax,ip,cs,fl; };struct INTERRUPT r1;void interrupt (*prev)();void interrupt myfunc(struct INTERRUPT r){ r1=r; /*int i=0; cout<<"HELLO WORLD"; i=i*5; cout<<"iis="<<i; */ //cout<<"\nHELLO WORLD ONCE AGAIN"; (*prev)();}void main(){ /*cout<<"\nAddress of myfunc="<< (void*)(myfunc)<<"\n"; cout<<"\nAddress of myfunc2="<< (void*)(myfunc2)<<"\n"; */ int i=20; clrscr(); prev=getvect(9); setvect(9,myfunc); //myfunc(); while(i>0) { printf("VALUES OF R1 ARE\n"); printf("\nbp=%x,di=%x,si=%x,ds=%x,es=%x,dx=%x,cx=%x,bx=%x,ax,ip=%x,cs=%x,fl=%x", r1.bp,r1.di,r1.si,r1.ds,r1.es,r1.dx,r1.cx,r1.bx,r1.ax,r1.ip,r1.cs,r1.fl); getch(); i--; }}[/color] Another Program but it doesn’t work that well but shows the values of all register is shown below. Just press a key to get out of it If you can pls correct the malfunction that arises while printing values. Also if you see any negative values in the registers its just that the address is being interpreted or it has exceeded the maximum limit of the data type. No NEGATIVE addresses are there!!! [color=green]#include<dos.h> #include<conio.h> #include<stdio.h> char far *scr=(char far*)0xB8000000l; struct INTERRUPT { unsigned bp,di,si,ds,es,dx,cx,bx,ax,ip,cs,fl; }; struct INTERRUPT r1; char contents[49][80]; int counter=0,busy=0;// unsigned ip,fl,c;void interrupt (*prev)();void printToMemory(char *msg,int row,int col){ int i=0,j=0,status=0,k=0; for(k=0;k<strlen(msg);k++) { if(row>49) { row=49; //col=0; for(i=0;i<49;i++) { for(j=0;j<80;j++) contents[i][j]=scr[160*(i+1)+2*j]; } status=1; } if(col>79) { if(row<49) row++; col=0; } if(status==1) { for(i=0;i<49;i++) { for(j=0;j<80;j++) scr[160*i+2*j]=contents[i][j]; } }// else col++; scr[160*row+2*col]=msg[k]; col++; }}void printToMemory2(char *msg,int *row,int col){ int i=0; if(*row>49) *row=8; for(i=0;i<strlen(msg);i++) { scr[160*(*row)+2*col]=msg[i]; col++; }} void interrupt our(struct INTERRUPT r) { static int i=8; char str[20],str2[20]; if(counter==18 && busy==0) { busy=1; counter=0; itoa(r.ip,str,16); itoa(r.cs,str2,16);//WHY NEGATIVE VALUES IN CS?? DECLARED IT AS UNSIGNED!! strcat(str2,":"); strcat(str2,str); r1=r; //r1.ip=atoi(str); //Should hold value OF IP that's in str printToMemory(str2,i,8); //printToMemory2(str2,&i,8); i++; busy=0; } else { counter++; if(counter>18) counter=0; } (*prev)(); } void main() { int i=0; prev=getvect(8); setvect(8,our); clrscr(); for(i=0;i<30000;i++); printf("THE OUTPUT YOU SEE IS IN THE FORM CS:IP(or CODE_SEGMENT:PROGRAM COUNTER)"); printf("\n TO CHANGE THE OUTPUT FROM DECIMAL TYPE 16 in PLACE OF 10 in itoa()"); printf("\n Don't USE printf inside the ISR our.SINCE printf also uses an INTERRUPT"); //cout<<"AX="<<r1.ax<<"\nBX="<<r1.bx<<"\nCX="<<r1.cx<<"\nDX="<<r1.dx; printf("\nCS=%x \nDS=%d \nIP=%x",r1.cs,r1.ds,r1.ip); getch(); }[/color] That’s all for now try some programs by getting info on various interrupts. Note that all are not defined by the BIOS and are user defined interrupts like interrupt 0x21 this is defined by DOS not the bios. However you can use it and any other up until 255 (0-255 total interrupts).Please give some remarks for this tutorial. So that I know people are reading the stuff I write. Happy Coding.