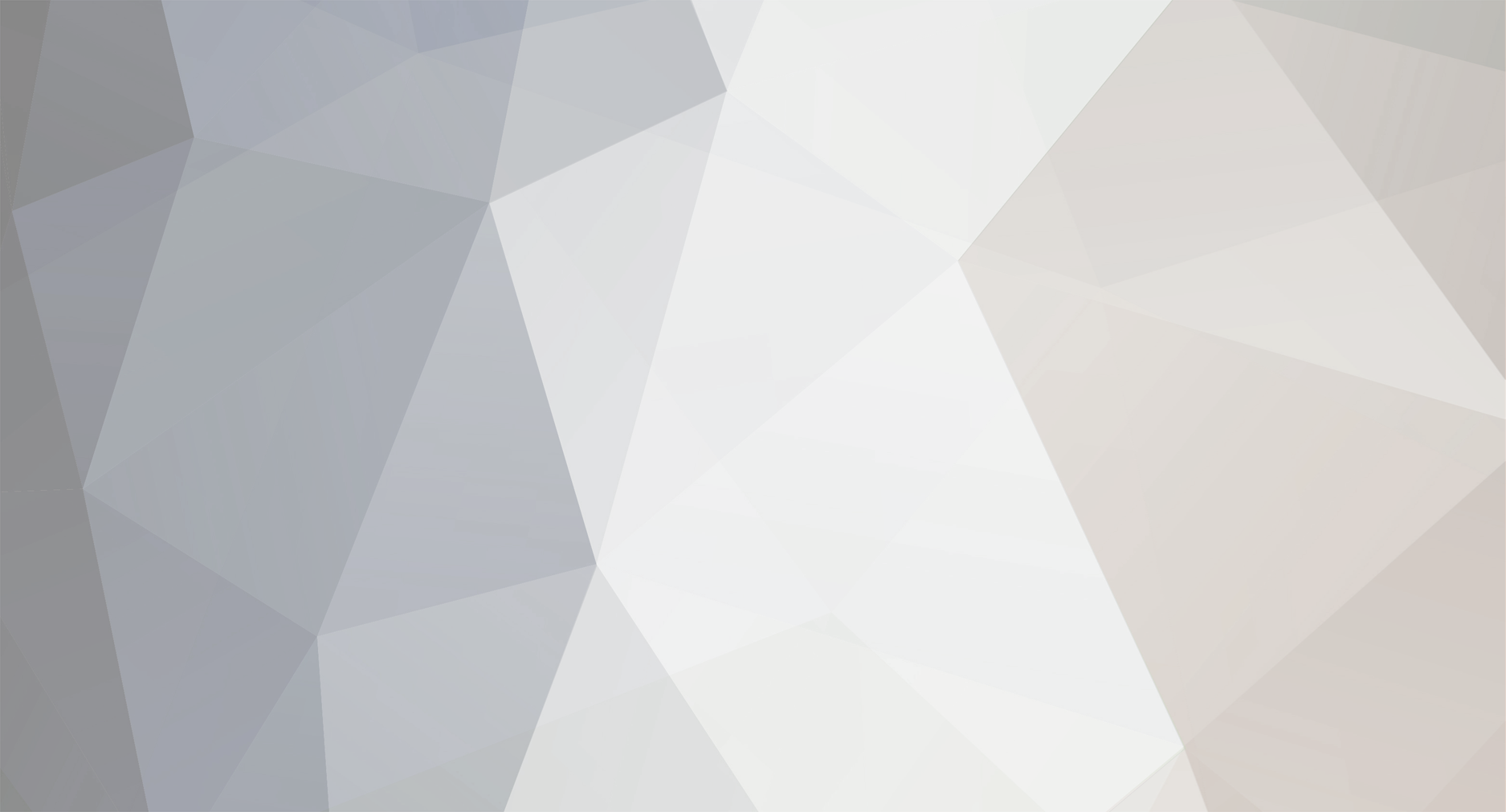
Frazzman
Members-
Content Count
17 -
Joined
-
Last visited
About Frazzman
-
Rank
Newbie [Level 1]
-
Welcome to my PHP tutorial on how to create a Login script using PHP that connects to a MySQL database and reads usernames and passwords before continuing. STEP 1 (ONE) - Getting prepared First off, you will need to install a program called XAMPP. You can download it from Here. Just follow their step-by-step tutorial on how to set it up. You will need this so you can code in PHP and test it by using phpmyadmin and MySQL. Now, the program I recommend for coding PHP is Notepad++. This is an epic program that can be used to code many different languages. You can download it from Here. STEP 2 (TWO) - Setting up the files First off, we need to create the files for this tutorial. Go ahead and open Notepad++ and create 2 new files. Save them both in the same file, one with the name "index.php" and "login.php" STEP 3 (THREE) - The Login Form Now we need to make the login form for our login script. Open up the index.php and enter this code. <form action='login.php' method='POST'> This will start off the form. action='' means what file this form will be relying on for input data, so we put login.php as our action. The method can either be POST or GET. POST means that a user will be inputting data to send a request, which in this case will be our action. Now put this underneath Username:<br /> <input type='text' name='username' /><br /> Password:<br /> <input type='password' name='password' /><br /> <input type='submit' value='Login' /> </form> Username: is just the text in front of the textbox The input is an option for some sort of input for the user. type='text' means is will be a normal textbox for a user to type something. name='username' is just the name of the textbox. This is crucial for this tutorial. <br /> is just a line break, meaning the next line of coding will be placed on a separate line. input type='password' means this will be a textbox for a password. When a user types something in, it will show up as Dots or *. The name is password. Once again, this is crucial. input type='submit' is the code for a button. value='Login' is the text that will be shown on the buttton. That is the index.php done, now to the next step. STEP 4 (FOUR) - Starting the Login Script and creating our database Open up the "login.php" First, we need to put the PHP tags for our PHP code to work. <?php ?> Our coding will go in between these tags. We are going to start off by defining 2 (two) variables. Our Username and password. Enter this code: $username = $_POST['username']; $password = $_POST['password']; $username and $password are the 2 (two) variables we are going to be defining. $_POST['username'] and $_POST['password'] is what our variable is. $_POST is the method we put in our login form, which was POST. The ['username'] and ['password'] is the name of those textboxes in our login form. The login will be reading off of those textboxes. Now after that, type this: if ($username&&$password) { } else { die("Error: Please enter a username AND password!"); } This means, is the 2 (two) textboxes have something in them, then it will continue on with the script between the { and }. If 1 (one) or both are empty, then the script will terminate itself, showing an error of "Error: Please enter a username AND password!". That is our else statement. Now we need to create an SQL database for the next step. If you did what I said before and installed XAMPP, go to your browser and in the address bar, type: LOCALHOST/ NOTE: Make sure your Apache and MySQL services are running! Enable them in your XAMPP control panel. When you are at the XAMPP main screen, select phpMyAdmin In the text area where it says Create new database, type whatever name you want your database and click Create. Don't worry about the other stuff. It will take you to a new screen, saying it created the database. In the text field where it says Create new table..., Name the table accounts and put the number of fields as 3 (three). In the first field, put the name as id and the type as INT. Go down to where it says index and choose PRIMARY. Also tick the box for AUTO_INCREMENT. This means the id will start at 0 or 1 and continue everytime a new user is made. In the second field, name it username and the type and VARCHAR, set the max length to 25. For the third field, name it password and the type as VARCHAR. Again, set the max length to 25. Now click Save down the bottom. Now that we got the database out the way, we need to make a username and password for it. Go back to the database you made and at the top of, select the tab that says Privileges. Select New User and choose a username and password for the database. Make sure you have ALL privileges. Ok, that is phpMyAdmin out the way. Still a lot more to go (: STEP 5 (FIVE) - The rest of the Login Script. In between the { and } type: $connect = mysql_connect("Host","DB Username","DB Password") or die("Couldn't connect"); mysql_select_db("Name of the database here") or die("Couldn't find database"); In this, we are defining the variable $connect. This will execute a MySQL command to connect to the databse, mysql_connect. In the brackets, change the Host to your MySQL host. In this case, it would be localhost. DB Username and DB password is the user you created for the privileges to the database. If it cannot connect, it will terminate the script with the message "Couldn't connect". If all is good, it will execute a MySQL command to select a database, mysql_select_db. In the brackets, type the name of the database you created. Make sure you keep the ""! If it can't find the database, it will terminate. After this we are going to perform a MySQL query. Type this after the above code. $query = mysql_query("SELECT * FROM accounts WHERE username='$username'"); $query is the variable we are defining. mysql_query is the command to execute a query. In the brackets it says "SELECT * FROM accounts WHERE username='$username'", this means it will search every row in the table accounts for a username $username which was the variable we defined at the top of the script for our username text box. After that, type: $numrows = mysql_num_rows($query); if ($numrows!=0) { } else { die("That user does not exist!"); } We are defining the variable $numrows to scourer through the table, mysql_num_rows, for the username you entered for the $query variable. If it finds a username that is the same as the one you entered, it will perform the next step. If it does not find it, it will terminate the script with a message That user does not exist!. Now, in between the { and }, enter: while ($row = mysql_fetch_assoc($query)) { $dbusername = $row['username']; $dbpassword = $row['password']; } This one is pretty much defining a variable associated with one of the columns from your database. In this case, we are defining $dbusername and the row username in your table and $dbpassword as the row password in your table. After that, type: if ($username==$dbusername&&$password==$dbpassword) { header( 'Location: Your location here'); $_SESSION['username']=$username; } else die("Incorrect Password!"); This will check if the username textbox, $username, matches one of the usernames from the table, $dbusername. It will also make sure on top of that the password textbox, $password, is exactly the same as one of the passwords in the table, $dbpassword. If this is true, then it will redirect you to your desired webpage. Change your location here to your desired address, It will also make a session. This means we need to go back to the top of the script and after the <?php we need to put: session_start(); I will explain this a little further down. If this is not true then it will terminate the script. STEP 6 (SIX) - The session Where we put $_SESSION['username']=$username, this will make a session for that username. What this means is: -If you make a webpage where you want a user to login, then you would put if ($_SESSION['username']){ } else { } In between the { and } you would put the code that the user must be logged in to see, and at the top of the webpage, you must put session_start(); STEP 7 (SEVEN) - Testing the Script Now, in you phpMyAdmin, make an account in the table accounts to test your script. If all went well, you will be fine (: You have no idea how long this took me to type up lol. Thanks would be greatly appreciated.
-
Best Thing For Making Website Templates! website templates
Frazzman replied to addict's topic in Websites and Web Designing
Thanks alot but I think I would rather use my hands and bare code it but this tool would be pretty good If I wanna make up a template quickly but they come out looking a little shabby but I guess it depends on your knowledge of these stuff thanks anyway will attemtP more -
I know HTML to a High level but this great to recap and jog my memory since I have not done HTML for ages it's a core programming skill and I love it just so cool
-
Vbulletin hide ressuruction hack is awesome you can hide from many things like posts usergroups if they have thanked etc which makes this very helpful for prevention of Lee hers
-
I love vbulletin best piece of forum software going and I'm extremely skilled in using it, the price is 100% worth it as you can make that back via donations etc anyway. Also there are bulled versions for those who cannot afford it, I know a site with nulled bulletin and it releases loads of mods to therefore a great substitue and is identical to bulletin as it is vbulletin hope I helpedd
-
Looks amazing i really want this such a pitty the site is down i would die for this, looks awesome, would be very helpful and get me closer to the dream of making a game similar to movoda.net good luck with whatever your making and i hope you fufil my request lol
-
Thank you for the list this will come in helpful for the future, i use Notepad++ Or dreamweaver
-
I vote bring back the site it was one of the most helpful things ever i would really appreciate the files and all that, thanks.
-
Whats happend to the site bring it back i love your tuts and your stuff keep us updated
-
Kinda skipped a few and saw some forums these look awesome! Well made! Thanks i love your work! Keep this up any more updates coming soon?
-
Preparing To Make A Online Text-based Game...
Frazzman replied to Curt200518's topic in Computer Gaming
Good luck, my i ask what kind is it going to be, a gangster type or a skills type like woodcutting fishing etc? -
Hi, Thanks for this tutorial, i see this is turning into a gangster Text based game i would serisouly appreciate help in being able to transfer this into a game similar to http://movoda.net/ http://www.syrnia.com/ thanks!
-
Just the information i was looking for thanks alot i will follow this like theres no tommorow hopefully i can work with these engines well and make a cool looking mmorpg thanks.