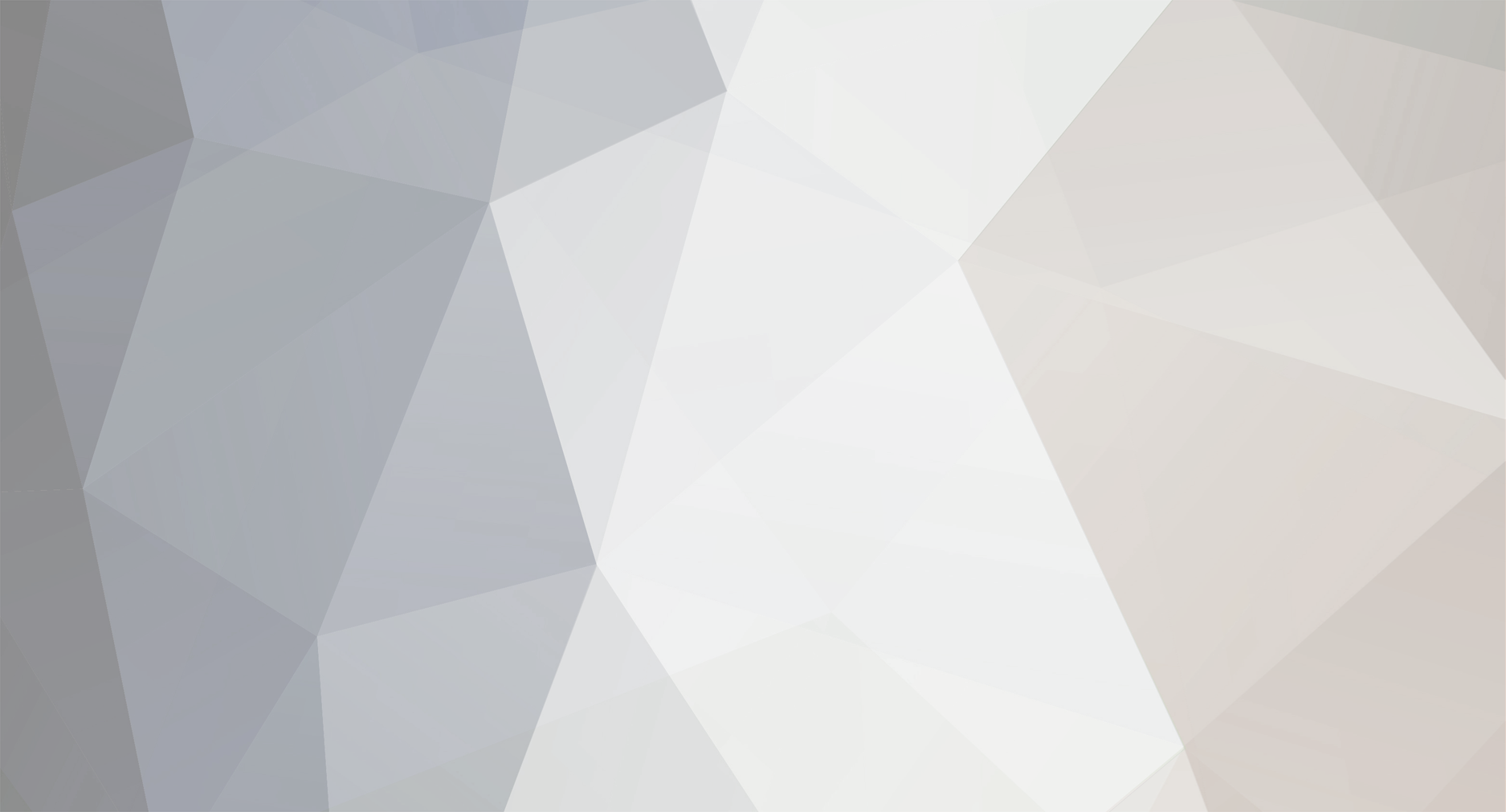
bluefish1405241537
Members-
Content Count
72 -
Joined
-
Last visited
Everything posted by bluefish1405241537
-
Is It Worth It To Get Gmail ? Is Gmail Good?
bluefish1405241537 replied to wazzupster0204's topic in Search Engines
GMail is pretty much the best provider around. The only part that isn't so good is switching, but for most people it shouldn't be too much of a problem. -
Ajax: Achieve Ajax Program In 5 Lines Of Code!
bluefish1405241537 replied to demolaynyc's topic in Programming
This seems pretty good. For my AJAX, I created a similar structure. My only suggestion for improvement would be to add additional functions to handle return values, instead of using getElementsByTagName or childNodes. Just a suggestion, but there's no need to do it because what you have is simple and functional, instead of complex. -
In this tutorial I will attempt to introduce you to the basics of AJAX programming. AJAX is basically the name given to a type of programming combining server-side and client-side scripting to create a seamless web application that is both secure and functional. It has all the benefits of web sites and regular applications. Here are a few key features that attract so many people to this type of programming: -Instant feedback to user -No waiting for pages to load because loading is done in background -Easy communication with server to allow security and functionality at a good speed -With a standard web site you need to wait for the browser to not only download a page but also render it. With AJAX, that wait is gone: it is displayed almost as soon as it is downloaded AJAX (usually) uses to following technologies: -JavaScript, for server side scripting -HTML, CSS, DOM, etc. to render page for user -A server side scripting language (it is irrelevant which one) -XML for communication between server and client, usually used by server to client communication Since we are in the JavaScript section, I will assume that you are comfortable with JavaScript. I will first give you an introduction to XML. If you are already comfortable with XML, you may skip this section. XML XML is basically a way to describe objects. Objects are organized in a hierarchy. The opening part of a (sub)hierarchy is represented by <NAME>, where NAME is the type of object. The end of an object is represented by </NAME>. You should recognize this as being similar to HTML. It is. Since you should already know HTML, I will simply compare them. -XML is much more strict than HTML, and your pages must comply, otherwise it should generate errors -A page is started by <?xml version="1.0"?>-After the <?xml?> tag, the rest of the document must be enclosed within a single pair of tags-Every tag may have attributes. Attribute values must be enclosed within double quotes -Whitespace is preserved, but ignored by the parser except when data is extracted -Objects are referred to as child nodes of those directly above them -Each object also has a text node, which is the text within the opening and closing tags For more specific specifications, please refer to W3C - they have a good tutorial. Here is a sample XML document: <?xml version="1.0"?><Xisto><tutorial type="programming/javascript"><post><title>AJAX</title><description>Learn AJAX</description><author>bluefish</author><content>hi</content></post></tutorial></Xisto>As you can see, in this example I described this post. In general, the attributes are used to give detail on the actual data, and should not represent data. To get a more advanced knowledge, click on W3 Schools XML Tutorial. The Basics of Interaction Now we should know the basics of the technologies described above (with the possible exception of DOM and/or CSS). I will show you the JavaScript object that allows for AJAX to happen: The XMLHttpRequest Object Or, as Microsoft likes to call it, ActiveXObject("Microsoft.XMLHTTP") As a side note: I recommend Firefox browser with the Firebug extension. Firefox follows the W3C recommendations, and there is no debugger around that compares with Firebug for AJAX programming. Now, to use this object, we should use a code like the following: var t;if(window.XMLHttpRequest)t = new XMLHttpRequest();else if(window.ActiveXObject) //Internet Explorert = new ActiveXObject("Microsoft.XMLHTTP");elsealert("Your browser does not support XMLHttpRequests!");This creates t as an XMLHttpRequest if it exists, otherwise tries ActiveXObjects, and should it fail that, gives a message that it failed. Then we need to make it do something. First, we need to assign a handler function for when it loads. t.onload = myfunction;function myfunction() {alert("Hi! We got our request back!");} Simple enough? Good.The generally recommended method of passing data to the server is via GET data, which is basically embedded in the URL you send. For example, "astahost; contains three get values: post, new_post, and 93, indexed by act, do, and f, respectively. Now we're ready to pass data to the server. t.open("GET", page, true);t.send(null);Simple? Good. GET is the type of data we are passing. page is the variable containing our page url, possibly with GET data. The last argument indicates that we are using asynchronous mode, which allows the handler function to be called instead of looping until a response is received, which can cause many problems. The section function is unimportant but necessary. Now all we need to do is wait for the information to be received! Here is a function a created to encompass a request. function createRequest(page, handler) {if(window.XMLHttpRequest) {req = new XMLHttpRequest();} else if(window.ActiveXObject) {req = new ActiveXObject("Microsoft.XMLHTTP");} else {alert("Your browser does not support XML HTTP requests! Please switch to a different browser before using this website.");return false;}req.onload = handler;req.open("GET", page, true);req.send(null);return req;} As you can see, it uses all the parts we introduced. We would use it like so: myRequest = createRequest("hello.php", responseHandler);function responseHandler() {//Do stuff with myRequest} The only thing we have left to do is receive the actual data. There are two relevant properties that we can use in our function: responseText, and responseXML. The text is simply the text of the response. The XML is an object representing the structure of the document, which contains several properties and methods. You can look them up, or you can use the handy function I created for your use (tried and tested): function getValue(request, term) {/*term needs to be parsed, appears in form:a/b/cwhere b is child of a, c child of beach node can be "#1", where "1" is the index of the child nodeor "name#1" where "name" is the name of the type of node and "1" is the index of the nodes of that typeor just "name" which defaults to index 0 for that type*/try {var nodes = term.split("/");var current = request.responseXML;for(i=0;i<nodes.length;++i) {if(nodes[i].indexOf("#")==0) { //Type 1current = current.childNodes[nodes[i].replace("#","")];} else if(nodes[i].indexOf("#")>0) { //Type 2var s = nodes[i].split("#");current = current.getElementsByTagName(s[0])[parseInt(s[1])];}else { //Type 3current = current.getElementsByTagName(nodes[i])[0];}}return current.nodeValue;} catch(e) {throw new exception("Invalid XML document/query!");}}It takes two arguments: the first is the request, and the second is a string that will be parsed to get data from the document. To use it, use a "/" as a divider, and have the node names. Alternately, you can use #0, where 0 is the index of the child node, or node#0, which takes the 0th element of node at that part of the hierarchy. For example, to get the value of the title of my message in the XML document above, I could use "Xisto/tutorial#0/post/title/#0". We need to add #0 at the end to get the text value. Next, we need to use a server-side language to create the XML document. I won't walk you through that, but if you have any trouble, feel free to reply and I will assist you in any way I can.
-
Telescopes May Be Our New Artist Paintbrushes
bluefish1405241537 replied to mtnbluet's topic in Websites and Web Designing
That is really cool . Orca, the "fake" effect is probably caused by what vitorious described.It looks so... Electrical. Explosion. Fire. I can't even describe it... Completely awesome. -
Yeah, it really depends on the source, and the author. My local newspaper is liberal (so I'm told). The whole issue of biased media is one of much debate. If we spent the time trying to figure out how to make a completely unbiased newscast, by the time we had prioritized the news it would be past the deadline. And truly there is no agreement on which stories should be given priority. For example, a story of a local shooting could be front page news; it all depends on what the media thinks the public wants, because the media exists to tell the public either a ) what the public wants to hear, b ) what the government wants them to hear, or c ) what the newscaster/media station wants you to hear. It's not so much the actual importance of the news item as the potential impact it could have on the station's consumers. A nuclear bomb could go off in Asia and if the papers didn't want to say it, it wouldn't be said. (of course, they would want to say it because it makes a nice headline, but you get the idea)
-
Xbox Scratches Your Games another action by Microsoft
bluefish1405241537 replied to Netwalker's topic in Software
All the more reason to buy a Wii instead. But I imagine that for an Xbox user, scratched disks would really suck. I find that Microsoft as a company is pretty bad on the customer service end, and refuses to acknowledge that any problem could exist in their software or hardware. -
Programming In Vb .net, Vista Compatible ?
bluefish1405241537 replied to dhanesh1405241511's topic in Programming
I believe all applications created with the .NET framework 2.0 should be compatible with Vista. .NET 3.0 is apparently (from the MSDN site) the same as 2.0, except with added features. Unless your product uses specific XP things, I believe it should be compatible (don't quote me on this, though). I wouldn't imagine that Windows would have changed their *cough*bad*cough* code that you need to interact with it. -
Can't Import Backup Sql File To Localhost
bluefish1405241537 replied to WeaponX's topic in Programming
If WAMP has phpMyAdmin, I would suggest to just use that utility. You would avoid any possible syntax problems, not to mention it is just easier. -
Wow! This is so awesome!After playing around for about half an hour, I'm now waiting for my ocean to fill up. Then I can infest it with plant, then I can burn it all! Mwahahaha!
-
Isn't this more or less what the Fasterfox add-on does? Having a look at the preference window, it seems like it is basically a GUI for editing these values, along with several preset options. Pipelining, cache, connections... It looks the same. I think that if you want to do this, you might as well use the add-on because it's just a little easier.
-
Run A Webserver From Usb-stick On Windows Guide
bluefish1405241537 replied to bakr_2k5's topic in Computer Networks
This is awesome! It's just what I've been looking for. I work on a variety of different computers, and it's very annoying to have to set up servers on each one and make sure that I'm using the newest version of the server. It's great that you know these programs. I used WAMP5 before, and it's all right, but it went kind of weird on Windows 2000 (I'm not sure if it was just the version of Windows, but there was a bug). XAMPP seems to be better, with more features (not that I really need it - I'm a down-to-the-basics kind of guy, but it's handy all the same). And WOS is a real discovery. Kudos to you for bringing this to my (and hopefully other people's) attention! -
Thanks MC,I solved the first problem by using DOM. I don't know what the original problem was, but it might have something to do with innerHTML not activating the script object. Anyways, it worked out without any real problems. As for the second problem, it was my fault for appending the lines of HTML separately, when I should have put them in a string and then put the whole string on. InnerHTML automatically closes unclosed tags when it's changed, so it could't tell that I intended to close it later.
-
Hello, I have started to try to create a JavaScript application (or rather, AJAX, but the JavaScript is the part I'm having trouble with). I have come across a roadblock, though. I try to load an external JavaScript file by editing the innerHTML of a div tag to contain <script src="URL" type="text/javascript"> (with a real URL). The problem is that it is not loading. I have used FireBug to check the dynamic HTML status, I get the following (with code removed): <html><head/><body><div id="status"/><div id="main"/><script type="text/javascript"></script><div id="scripts"><!--START DYNAMIC JAVASCRIPT--><script src="source/main_login.js" type="text/javascript"></script><!--END DYNAMIC JAVASCRIPT--></div><script src="source/main.js" type="text/javascript"></script></body></html> I have done extensive testing and am sure that main_login is not loading. When I insert the relevant code into the actual file, it works fine. Is there anything I am doing wrong and/or is there a solution? Note: This could be related to the fact that FireBug does not update the dynamically added code when I change it, but that may just be a bug. I am also having a problem with inserting HTML into a <div> tag - my tags seem to close themselves, as in the following code. It seems quite possible that the two problems are related. <div id="main"><div id="main/login"/><form onsubmit="java script:login(this);"/><table/>User Name:<input type="text" name="usr_name"/>Password:<input type="password" name="usr_pass"/><input type="submit" value="Log In"/></div>(the table, and form should encompass the whole)
-
Problems With phpMyAdmin (Unable To Edit The Fields)
bluefish1405241537 replied to niran's topic in Web Hosting Support
It could be the internet browser. It might do something weird to the post values which could be the problem.I have had a look at the offending source code, and it confuses me. It checks to see if a certain variable exists - if not, changes another variable to null. But then that variable is used in a foreach statement?!?!?!? That seems to be the problem, and if so, is probably with phpMyAdmin.EDIT: The second error message (headers) is just a side-effect of the first error. When the error message is echoed by PHP, it automatically sends the headers. Then when a script tries to change the headers, they were already sent so the second error message appears. It is just the first one that is the problem.I think Xisto should upgrade phpMyAdmin. They're only on 2.9.0.2, when the newest is 2.9.2. -
I've had a look at attachments, and it seems horribly complicated. The best way might be to store it on the server. Keep in mind that you'll need some sort of limitation, or they'll use up all your space. I don't know if you already have that in your site, but if you don't you should add it. I'd use some sort of code like the following (inserted beside the mail function in your script: $filesplit = explode("\\", $file); //Split file to find extension$f = fopen("uploaded_$from_".time()."_".$filesplit[count($filesplit)-1], "xb") or die("Could not upload file."); //Create file with name "uploaded_person_time_filename.ext" and end if failedfwrite($f, implode("", file($file))); //Write file as string to new filefclose($f);//Close fileI have not tested the code, so if it doesn't work you can try to figure out the problem or you can tell me what's wrong. Good luck.
-
This tutorial will show you how to create a simple user validation script with PHP. We will need two files: "protect.php" and "login.php". The protect file is not meant to be viewed by itself. In order to protect a page, you need to include that file by using PHP code like the following: include("protect.php"); Keep in mind that this needs to be in between your <?php and ?> tags.This bit of code uses the include function. It is a handy function that reads all the information contained in one file and temporarily adds it to another. For example, this can be used to create an easily modifiable template. You donât really need to know exactly how it works to use it, though. The login page is where users will enter their username and password in order to log in to your website. Weâll start by working on the login.php file. <form action=login.php method=post>Username: <input type="text" name="username"><br>Password: <input type="password" name="password"><br><input type="submit" value="Login"></form>That is a bit of HTML that will create a login form, with two fields: username and password. When your users click the submit button, the page will reload (because we specified login.php as the action for the form â the action is the place the information contained will be sent to). When the page reloads, however, we want to see the post data â the information the user has sent, so that we can check if it is valid. To do that, we can use a bit of PHP code at the beginning of the page like the following: <?phpif(isset($_POST["username"])&&isset($_POST["password"])) {echo "Thank you for trying to login.";}?>If you put that code at the top of your login.php page, youâll notice that when you press submit it will show the text. The "if" statement that I used may look new to you. The isset function checks if the given variable exists. The $_POST array indexes all the information that has been posted to the page. So when we use $_POST["username"], we are getting the posted value of the input indexed as "username" (as determined by the name parameter of our "input" fields that I showed you earlier). When combined with isset, we can check whether the user has posted a value to the page. Now, we need to check if the user has entered correct information. To do so, we can use PHP code like the following (in place of the echo command in the above code). $user = $_POST["username"];$pass = $_POST["password"];$validated = false;//Begin validation codeif($user=="User1"&&$pass=="password1") $validated = true;if($user=="User2"&&$pass=="password2") $validated = true;//End validation code//Begin login codeif($validated)echo "Logged in as $user.";elseecho "Invalid username/password combination.";//End login codeThis is a rather simple way to check. If we have more users, we could use something like the following in place of the validation code above: $passwords = array("User1"=>"password1", "User2"=>"password2");if(isset($passwords[$user])) if($passwords[$user]==$pass) $validated = true;That code puts the passwords into an associative array, then checks to see if the password for the user is correct. Which method you choose does not matter. Now, of course, we need to actually do something when we log in. To do this, we will use cookies. Cookies are pieces of data that websites can store on usersâ computers. We will need to store login information. Each website has its own cookie, so we donât need to worry about having the same names as other websites. To set a cookie, we use the setcookie function. One important note about the setcookie function: you must use it before any statements that print data, e.g. echo. //Begin login codeif($validated) {setcookie("username", $user); //Sets a cookie storing the usernamesetcookie("password", MD5($pass)); //Sets a cookie storing the encrypted value of the passwordecho "Logged in as $user.";} else {echo "Invalid username/password combination.";}//End login codeNow, one thing you may be confused about is the MD5 function. The MD5 function encrypts data. This is a simple security measure, and is by no means foolproof, but it helps protect you. Iâll show you later how to use the MD5 function to check if the password is correct. Weâre done with the login.php page. It should now correctly log you in. Here is the full code: <?phpif(isset($_POST["username"])&&isset($_POST["password"])) {$user = $_POST["username"];$pass = $_POST["password"];$validated = false;//Begin validation codeif($user=="User1"&&$pass=="password1") $validated = true;if($user=="User2"&&$pass=="password2") $validated = true;//End validation code//Begin login codeif($validated) {setcookie("username", $user); //Sets a cookie storing the usernamesetcookie("password", MD5($pass)); //Sets a cookie storing the encrypted value of the passwordecho "Logged in as $user.";} else {echo "Invalid username/password combination.";}//End login code}?><form action=login.php method=post>Username: <input type="text" name="username"><br>Password: <input type="password" name="password"><br><input type="submit" value="Login"></form> Now, we need to edit the protect.php page.Weâll use a similar method for the login.php page to check if the user is logged in correctly. <?php$validated = false;//Use $_COOKIE to get the cookie data â same usage as $_POSTif(isset($_COOKIE["username"])&&isset($_COOKIE["password"])) {$user = $_COOKIE["username"];$pass = $_COOKIE["password"];//Begin validation codeif($user=="User1"&&$pass==MD5("password1")) $validated = true;if($user=="User2"&&$pass==MD5("password2")) $validated = true;//End validation code}if($validated) {//Ok; donât need to do anything} else {//Make user go to login pageheader("Location: login.php");exit;}?>The above code should look very familiar to you. It is basically the same as the login script, except for a few key differeneces:First, $validated has moved outside of the block of code. This is because as opposed to only doing something when they post, we need to protect our page all the time. Second, we use $_COOKIE instead of $_POST. This is because we want to get the cookie data. Nothing has been posted to the page, so $_POST is useless. Third, we use MD5 to encrypt our set password before comparing it to the stored password. This is because the stored password is already encrypted and by encrypting the other before comparing we make sure the comparison is fair. We can't decrypt the stored password because MD5 is one-way encryption. But don't worry about encryption â just make sure when you are comparing two values either both or neither of them should be encrypted for it to work properly. Fourth, the actions have changed. We no longer do anything when we have been validated, but if we havenât been validated, we use the header function. This is a complex function. All you need to know for now is that header("Location: page"); redirects the user to the given page. We want our users to be redirected to the login page if they are not allowed to access the page. Then, we need to exit the script because we are done with the page. Great! Now we have a working user validation script. Remember to include protect.php whenever you want to protect a page. This is only a simple script, though. There are many ways to improve it, such as: -use a MySQL database for users -automatically redirect back to the page the user came from when they log in -have an access level specifier that allows certain users access to certain pages -allow easy creation of users If you have any questions or comments, or if you notice a problem with my tutorial or code, please reply. Feel free to ask me for details if you want to extend your code using one of my suggestions.
-
Help With Ram not all memory being used
bluefish1405241537 replied to bluefish1405241537's topic in Hardware Workshop
The thing with the 192mb, is that it is two RAM cards - 64mb (don't ask me how old the computer is) and 128mb. I figured out the problem by experimenting. It turns out that the 64 conked out when I was fiddling with it (I'm not surprised), and the 256 was incorrectly labeled and packaged, when it was in fact 128 (as seen when it was isolated). The problem when all three were in together was that the two 128s were combining to make the 256, but since I expected the 64 to be contributing too I did not think of the possibility. Thanks for all your help. -
Hello. Recently, I upgraded the memory on my computer. Previously it had 192mb, and I added 256mb. I installed the RAM properly: it works fine, but it seems that it is now only recognizing the 256mb RAM chip, and ignoring the 192mb (two chips). E.g. when I go to the system menu (Win XP), it only says 256mb of RAM, but when summed it should be 448mb. Is this normal? Is there a way to fix it? Thanks in advance.
-
Yes you can, quite easily. I don't know the specific program, but it should act similar to what I know. For the server, just put in your address (i.e. yourname.astahost.com, or a TLD if you have one), and make sure the port # is 3306. Then login using the users you create on CPanel - make sure to prefix as in yourname_username (it says more about that on CPanel). Then just use your MySQL.
-
I have been looking around for a bit, and have not been able to find a solution to my problem. I was hoping someone here might know an algorithm for checking if two objects are overlapping.For example, I have two objects, each with an image. The objects have an x and y, but they have more than just a width and height - they have a shape. I'm not talking about rectangular collision, but actual (reasonably) precise measurements that don't take a huge amount of load or run-time processing.The best possible solution I could come up with would be to draw a blank object (off-screen, mind you), draw both objects, count up the number of background pixels remaining, and compare that with the total number of background pixels minus the sum of the number of pixels in the two objects.That should give you an idea of what I am looking for. This method would be faster than some other methods I could think of, but I'm sure there must be a better way. I have seen many programs with something like this, and I would like to know how it could be done in a practical way.
-
Or you could download and install PhpMyAdmin yourself... Not an ideal solution, but it would certainly work well enough. This is actually one of the few things that could be improved about Xisto, but like mark said, you can just use a separate client. Make sure to use port 3306 in the server name if you do that.
-
You can create a simple "language" pretty easily, you just need a parser, which usually doesn't take long - for example, I created a simple format to show links on web pages by parsing a text file, it would show links in the current directory and parent directories only. That took me less than an hour to get right. If you want a "real" language, I have no experience in that - like previously said, it takes a lot of work to create a good language from complete scratch. But translation is a much easier solution, but not always what you need - in that case, the only thing you could really do is simplify the language.
-
Solar System To Have 12 Planets
bluefish1405241537 replied to xboxrulz1405241485's topic in Science and Technology
Personally, I really don't see the reason for all the fuss about Pluto, etc. The way we define "Planet" is human - if we call the Sun a planet, that doesn't change anything. It's really all in perspective, and adding/removing planets really won't affect anything except teaching, and to be honest, (I think) most people wouldn't really care how many planets they are told there are. The main thing we want is consistency.Pluto is a planet? Yes. Wait - no. Maybe. Ask someone else.Rather than having these debates, people should focus on more important things and keep our terms consistent.