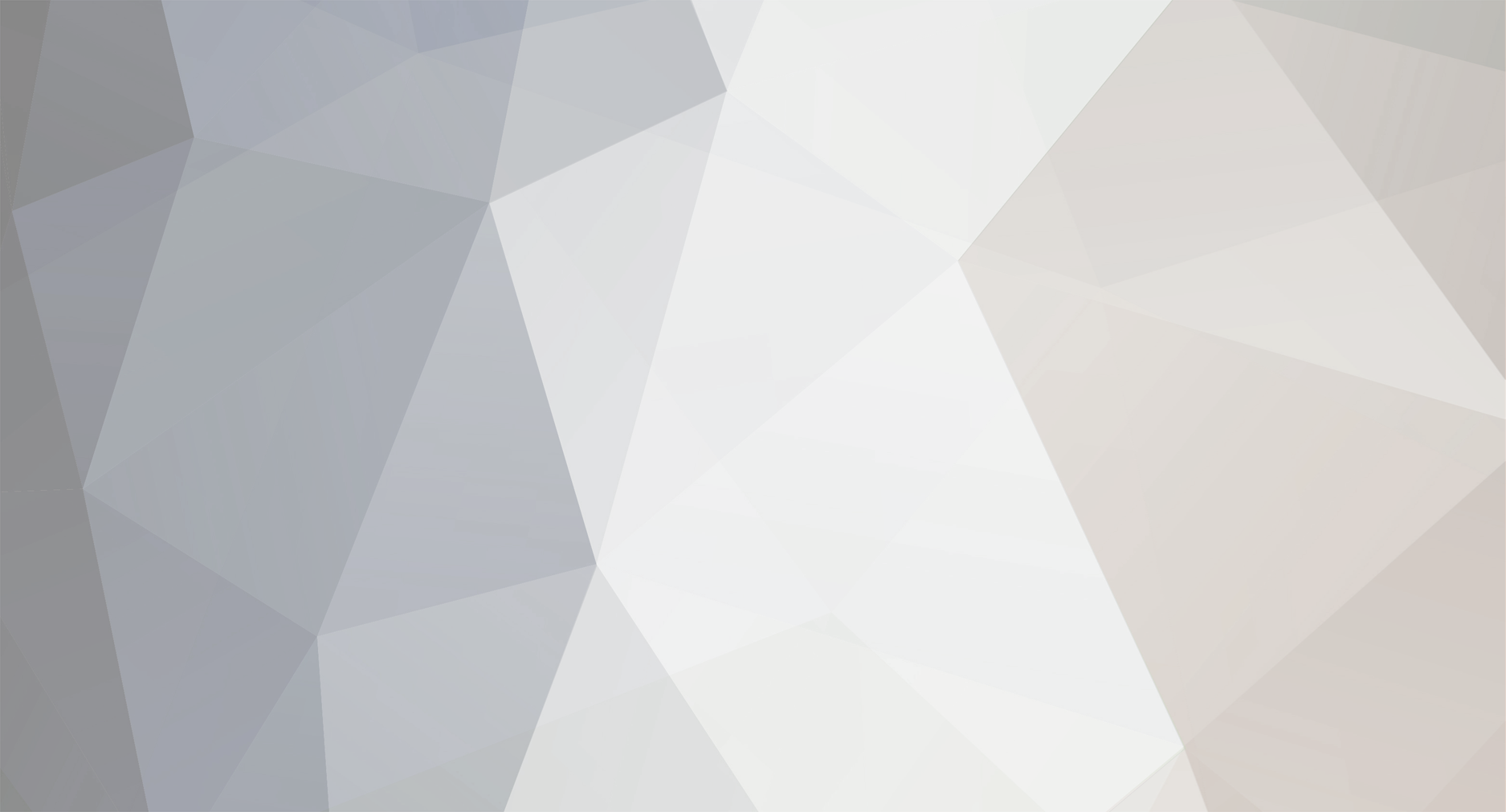
tansqrx
Members-
Content Count
723 -
Joined
-
Last visited
-
Days Won
1
Everything posted by tansqrx
-
Help On Inherit A Class In VB.NET ! Especially System.Net.Sockets.Socket
tansqrx replied to PureHeart's topic in Programming
Can you give me an example of what you are trying to do. When you are going from VB6 to .NET the rules have changes alittle bit. According what the event you are thrying to handle is, different ways will be used. Errors will be handled by a Try/Catch. Events that are created with add event will need a handler function. More information would much more helpful. -
I could not get the file to upload to the board so it can be found at http://forums.xisto.com/no_longer_exists/ Recompile and then run.
-
Option Strict OnOption Explicit On 'this is where you add reference to command classesImports System.NetImports System.IOImports System.TextPublic Class modSocket Public Function request(ByVal strLocation As String) As String 'create all the variable needed Dim httpRequest As HttpWebRequest Dim httpResponse As HttpWebResponse Dim responseStream As Stream Dim responseEncoding As Encoding Dim responseStreamReader As StreamReader Dim strResponse As String 'for operations that may fail or can throw an exception you should have 'a try/catch Try 'This is a little gotcha, it seems to me that you should just be 'able to just dim a HttpWebRequest request type but this is how MS 'wanted to do it. httpRequest = CType(WebRequest.Create(strLocation), HttpWebRequest) 'if for some reason you wanted to set the timeout, in ms httpRequest.Timeout = 20000 'this is what is passed as the request headers. Not needed but I wanted 'my app to look like it came from IE 6 httpRequest.Accept = "image/gif, image/x-xbitmap, image/jpeg, image/pjpeg, application/x-shockwave-flash, application/vnd.ms-excel, application/vnd.ms-powerpoint, application/msword, */*" httpRequest.UserAgent = "Mozilla/4.0 (compatible; MSIE 6.0; Windows NT 5.1; SV1; .NET CLR 1.1.4322)" 'Create the object to hold the response httpResponse = CType(httpRequest.GetResponse(), HttpWebResponse) 'The response needs to be converted to a stream responseStream = httpResponse.GetResponseStream() 'Telling what type of stream encoding responseEncoding = System.Text.Encoding.GetEncoding("utf-8") 'Now make into a StreamReader for added functionality responseStreamReader = New StreamReader(responseStream, responseEncoding) 'check to see if anything was returned If Not responseStreamReader Is Nothing Then 'transfer the data into the StreamReader strResponse = responseStreamReader.ReadToEnd End If 'handle possible exceptions Catch ex As WebException 'this is for the special exception such as a timeout MessageBox.Show(ex.Status.ToString) Catch ex As Exception 'any other exceptions MessageBox.Show(ex.ToString) End Try 'send the response string back to the main form Return strResponse End FunctionEnd Class
-
'Socket Programming Example in VB.NET 2003'written by tansqrx'1/11/05'This is a basic tutorial for connecting to a webpage the easy way in .NET. I have'also added many comments for one post in particular, as he is a beginner to .NET'As a general rule you should always put strict and explicit on'This will save you many headaches down the road especially in large projectsOption Strict OnOption Explicit On Imports System.IO'name of the main class, in this case I have renamed it. You can change what class'runs first by going to Project>Properties>Common Properties>General and selecting'FrmMain in the Startup Object.Public Class FrmMain Inherits System.Windows.Forms.Form#Region " Windows Form Designer generated code " Public Sub New() MyBase.New() 'This call is required by the Windows Form Designer. InitializeComponent() 'Add any initialization after the InitializeComponent() call End Sub 'Form overrides dispose to clean up the component list. Protected Overloads Overrides Sub Dispose(ByVal disposing As Boolean) If disposing Then If Not (components Is Nothing) Then components.Dispose() End If End If MyBase.Dispose(disposing) End Sub 'Required by the Windows Form Designer Private components As System.ComponentModel.IContainer 'NOTE: The following procedure is required by the Windows Form Designer 'It can be modified using the Windows Form Designer. 'Do not modify it using the code editor. Friend WithEvents btnRun As System.Windows.Forms.Button Friend WithEvents txtRaw As System.Windows.Forms.TextBox Friend WithEvents Label1 As System.Windows.Forms.Label Friend WithEvents AxWebBrowser1 As AxSHDocVw.AxWebBrowser Friend WithEvents Label2 As System.Windows.Forms.Label <System.Diagnostics.DebuggerStepThrough()> Private Sub InitializeComponent() Dim resources As System.Resources.ResourceManager = New System.Resources.ResourceManager(GetType(FrmMain)) Me.btnRun = New System.Windows.Forms.Button Me.txtRaw = New System.Windows.Forms.TextBox Me.Label1 = New System.Windows.Forms.Label Me.AxWebBrowser1 = New AxSHDocVw.AxWebBrowser Me.Label2 = New System.Windows.Forms.Label CType(Me.AxWebBrowser1, System.ComponentModel.ISupportInitialize).BeginInit() Me.SuspendLayout() ' 'btnRun ' Me.btnRun.Location = New System.Drawing.Point(24, 16) Me.btnRun.Name = "btnRun" Me.btnRun.TabIndex = 0 Me.btnRun.Text = "Run" ' 'txtRaw ' Me.txtRaw.Location = New System.Drawing.Point(24, 72) Me.txtRaw.Multiline = True Me.txtRaw.Name = "txtRaw" Me.txtRaw.ScrollBars = System.Windows.Forms.ScrollBars.Both Me.txtRaw.Size = New System.Drawing.Size(544, 120) Me.txtRaw.TabIndex = 1 Me.txtRaw.Text = "" ' 'Label1 ' Me.Label1.Location = New System.Drawing.Point(24, 48) Me.Label1.Name = "Label1" Me.Label1.TabIndex = 2 Me.Label1.Text = "Raw Output" ' 'AxWebBrowser1 ' Me.AxWebBrowser1.Enabled = True Me.AxWebBrowser1.Location = New System.Drawing.Point(24, 224) Me.AxWebBrowser1.OcxState = CType(resources.GetObject("AxWebBrowser1.OcxState"), System.Windows.Forms.AxHost.State) Me.AxWebBrowser1.Size = New System.Drawing.Size(544, 128) Me.AxWebBrowser1.TabIndex = 3 ' 'Label2 ' Me.Label2.Location = New System.Drawing.Point(24, 200) Me.Label2.Name = "Label2" Me.Label2.TabIndex = 4 Me.Label2.Text = "Web Browser" ' 'FrmMain ' Me.AutoScaleBaseSize = New System.Drawing.Size(5, 13) Me.ClientSize = New System.Drawing.Size(592, 374) Me.Controls.Add(Me.Label2) Me.Controls.Add(Me.AxWebBrowser1) Me.Controls.Add(Me.Label1) Me.Controls.Add(Me.txtRaw) Me.Controls.Add(Me.btnRun) Me.Name = "FrmMain" Me.Text = "Form1" CType(Me.AxWebBrowser1, System.ComponentModel.ISupportInitialize).EndInit() Me.ResumeLayout(False) End Sub#End Region 'This is the place to put items that need to be started when the app loads Private Sub FrmMain_Load(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles MyBase.Load End Sub 'This function runs when you press the Run button on the form Private Sub btnRun_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles btnRun.Click 'from here I created a new class: right click on project name in 'solution explorer>Add>Add New Item and name it modSocket.vb 'create an instance of modSocket just like any other variable 'make sure you have new keyword Dim socket As New modSocket Dim strLocation As String = "https://www.google.de/?gfe_rd=cr&ei=7AkjVIatDsKH8QfNkoC4DQ&gws_rd=ssl; Dim strResponse As String 'run the request sub,pass the strLocation variable, and get the response strResponse = socket.request(strLocation) 'write strResponse into the raw output text box txtRaw.Text = strResponse 'now I want to diplay the requested string graphically. I can use the COM 'object AxWebBrowser which can be added via the designer. This is purely an 'extension of functionality and can be obmitted. 'First the response string must be saved to a local file and then read into 'the AxWebBrowser 'create a FileStream with create attributes Dim fWriter As New FileStream("temp.html", FileMode.Create) 'create a StreamWriter to help us out Dim strWriter As New StreamWriter(fWriter) 'Transfer all data from the string into the StreamWriter Dim iIndex As Integer For iIndex = 0 To strResponse.Length - 1 strWriter.Write(strResponse.Chars(iIndex)) Next 'make sure everything is out of the stream and then close strWriter.Flush() strWriter.Close() 'navigate to the local file. The name is already provied in the FileStream AxWebBrowser1.Navigate(fWriter.Name.ToString) 'notice that no images are seen, that's because they are retrieved in a 'seperate HTTP request. End SubEnd Class
-
Help On Inherit A Class In VB.NET ! Especially System.Net.Sockets.Socket
tansqrx replied to PureHeart's topic in Programming
Keep the questions coming. This Thread has been dead way too long and now I get to gab about something I want to. I have written a tutorial especially for you at http://forums.xisto.com/topic/88313-topic/?findpost=1064335400. The short answer to your question is to use the Imports statement. I.e Imports System.IO right before the class name Option Strict On Option Explicit On Imports System.Net Public Class FrmMain Inherits System.Windows.Forms.Form ....... -
This little tutorial will help anyone jump into the world of sockets in .NET. Under .NET you will use the system.net namespace.This tutorial simply goes to the Google homepage, retrieves the HTML and displays it in both a raw output and graphical form. This project was written in VB.NET 2003 and can be downloaded below.
-
OK I will agree with that, just bad coding style. I have just found out that bad coding style can sink a ship quicker than almost anythign else.
-
ohhh, ohh, ohh, pick me, pick me (jumps up and down with hand raised) I actually had to look this one up because at first I thought they were all completely valid and done the same thing. After typing the variable name in Visual Studio I quickly had to turn to the help. I started in .NET and as such I am mostly ignorant of VB6 which this crap almost certainly came from. My example is for .NET. In short, ResourceName valid variable name although it will not compile because no type is given (string, integer, etc.) ResourceName$ Dim ResourceName as String ResourceName& Dim ResourceName as Long Special characters are short hand for assigning a type to a variable name. I have seen this used in older VB6 code but I would never use such bad techniques myself. Other types include: % - Integer @ - Decimal ! â Single # - Double The MSDN Document 2.2.1 Type CharactersSee Also Identifiers A type character following a non-escaped identifier denotes the type of the identifier. The type character is not considered part of the identifier. If a declaration includes a type character, the type character must agree with the type specified in the declaration itself; otherwise a compile-time error occurs. If the declaration omits the type, the type character is implicitly substituted as the type of the declaration. No white space may come between an identifier and its type character. There are no type characters for Byte or Short. Appending a type character to an identifier that conceptually does not have a type (for example, a namespace name) will cause a compile-time error. The following example shows the use of type characters: ' The following line will cause an error: standard modules have no type.Module Module1#End ModuleModule Module2 ' This function takes a Long parameter and returns a String. Function StringFunc$(ByVal LongParam&) ' The following line causes an error because the type' character conflicts with the declared type of' StringFunc and LongParam. StringFunc# = CStr(LongParam@) ' The following line is valid. StringFunc$ = CStr(LongParam&) End FunctionEnd Module TypeCharacter ::= IntegerTypeCharacter | LongTypeCharacter | DecimalTypeCharacter | SingleTypeCharacter | DoubleTypeCharacter | StringTypeCharacterIntegerTypeCharacter ::= %LongTypeCharacter ::= &DecimalTypeCharacter ::= @SingleTypeCharacter ::= !DoubleTypeCharacter ::= #StringTypeCharacter ::= $ Notice from jipman: code-tags for code next time
-
PureHeart, Do you have any specific needs reguarding sockets? I have written quite a few programs using the system.net functions and maybe I could give you a start. Do you need two programs to communicate or something different? BTW does anone know if 2005 added a timeout to the HttpWebRequest initial request? I know there is a timeout that you can specify for already opened connections (httpRequest.Timeout) but not for the initial request. I look and pulled many a hair out till I found that it just simply could not be done in 2003. It always defaults to some predetermined value (21 seconds I believe). While I am on the subject, here is some code that just about all beginners need when messing around with system.net. This code connects to a specified website and returns the HTML. Try httpRequest = CType(WebRequest.Create(âhttp://google.com;, _HttpWebRequest) âset theconnection timeout to 10 seconds httpRequest.Timeout = 10000 âchange some of the headers so it makes the program look âlike it cam from IE 6.0 httpRequest.Accept = "image/gif, image/x-xbitmap, image/jpeg, _image/pjpeg, application/x-shockwave-flash, application/vnd.ms _excel, application/vnd.ms-powerpoint, application/msword, */*" httpRequest.UserAgent = "Mozilla/4.0 (compatible; MSIE 6.0; Windows _NT 5.1; SV1; .NET CLR 1.1.4322)" httpResponse = CType(httpRequest.GetResponse(), HttpWebResponse) responseStream = httpResponse.GetResponseStream() responseEncoding = System.Text.Encoding.GetEncoding("utf-8") responseStreamReader = New StreamReader(responseStream, _responseEncoding) âThis is the HTML string âShould put more error handling here, you will need it dim strResponse as string = responseStreamReader.ReadToEndCatch ex As WebException MessageBox.Show(ex.Status.ToString)Catch ex As System.InvalidOperationException MessageBox.Show(ex.Status.ToString)End Try
-
As with any basic instant messaging service, Yahoo! Messenger offers several basic functions. In general, an instant messenger offers conversations between two users in real time. As a rule, both users will see the conversation line by line as it is typed. Although not required, instant messengers usually offer the ability to show away messages, reside in the system tray until needed, and offer a user buddy list [http://forums.xisto.com/no_longer_exists/ In addition to these basic services, the latest version of Yahoo! Messenger also offers more advanced features. Although not unique, these services make the instant messaging experience more enriching. The features described here pertain to version 7.0 of Yahoo! Messenger [https://messenger.yahoo.com/ Figure 1 - Yahoo! Messenger Main Window The official Yahoo! Messenger site breaks the features of Messenger into four main groups Entertainment Photo Sharing Messenger makes it possible to drag and drop photos onto the IM window and share them easily. LAUNCHcast Radio LAUNCHcast is a relatively new service that allows users to listen to music while signed into Messenger. LAUNCHcast sorts music by genre or theme and even allows the user to create their own station. The current music track is displaying in the away message which allows other users to also listen to that station. Yahoo! Games Yahoo! provides a variety of games for users to utilize. Games are divided into single player and multiplayer categories. IMVironments IMVironments are themes that can be used to customize a particular IM window. Both sender and receiver will see the IMVironment. IMVironments frequently have extra functionality built in or are sponsored by a third-party. Figure 2 - LAUNCHcast Radio [tutorials/images/features3_games.gif] Figure 3 - Yahoo! Games [tutorials/images/features5_IMviroments%20file.gif] Figure 4 - Heart IMviroment with File Transfer [tutorials/images/features6_audiables.gif] Figure 5 Audibles Productivity Find, Add, Share Friends As with most IM clients, Messenger allows users to find others with similar interests. This can be taken a step further by sharing entire lists with other users. The latest version allows data mining of new contacts by importing addresses from several email programs. Yahoo! Search Utilize the Yahoo! search engine from within any IM window. File Transfer File transfers are an essential feature among any sufficiently advanced IM client. The latest version of Messenger has improved its file transfer ability in the fact that it actually works now. Address Book Similar to the address book in Microsoft Outlook, the Messenger address book allows additional information to be stored about a buddy. Stealth Settings Sometimes it is desirable to not allow certain or all of your buddies to see when you are online. This gives a user the chance to hide himself from other users when needed. This setting can be applied in general, to a group, or to a particular buddy. [tutorials/images/features7_emoticons.gif] Figure 6 Emoticons [tutorials/images/features8_avatars.gif] Figure 7 Avatars Personalization Audibles Audibles are cute little flash animations used to express or heighten an IM message. Emoticons These are the standard smiley faces seen in just about every IM application. Yahoo! Avatars Avatars allow a user to create a graphic doll that represents their personality. Avatars can be customized to ethnicity, clothing, mood, and several other variables. A different type of feature, also called an avatar is also seen in Yahoo! chat rooms. These avatars are Unicode characters placed in front of a users chat response. Chat avatars are not recognized by all Yahoo! chat clients. Display Images An image can be placed by a user s name. Skins Skins are used to customize Messenger and all of its windows. Messenger skins are akin to themes in Microsoft Windows. [tutorials/images/features11_webcam.gif] Figure 10 Webcam [tutorials/images/features12_conference.gif] Figure 11 Conference [tutorials/images/features13_mobile.gif] Figure 12 - Yahoo! Mobile Communication Voice Messenger gives the user the chance to verbally communicate with other users. Messenger 7.0 incorporates the use of free PC-to-PC calls via Net2Phone. A user can leave a voice message, see call history, and change ringtones. Take Yahoo! with You One of the more unique features of Yahoo! Messenger is the ability to load it onto a cell phone and receive IMs away from the computer. Yahoo! offers many of its other services via mobile phone such as search and ringtones de.mobile.yahoo.com http://forums.xisto.com/ ]. From a security point of view, this expansion into the mobile arena may produce many fruitful results in years to come. Webcam Another feature ubiquitous to many IM clients is the webcam. Messenger has a few unique features not found in other IM clients such as Super Webcam and allowing multiple users to see the cam simultaneously. Conference Messenger allows multiple users to talk together in a setting similar to a private chat room.
-
Yahoo! started its life as âJerry and Davidâs Guide to the World Wide Webâ in January of 1994. Its creators David Filo and Jerry Yang, started Yahoo! as a way to track their personal interests. As word spread of this new effective search engine, resources were soon strained. Moving from Stanford University to Netscape facilities, and finally to its own headquarters, Yahoo! has become one of the largest Internet names in history. At the end of the day Yahoo! is still a business and like all businesses, Yahoo! has to make a profit and adhere to a business model. Yahoo! follows an advertisement based business model. Similar to television and radio, Yahoo! provides a free service to its customers. In exchange, for this service, Yahoo! customers and users agree to listen, watch, or view advertisements created by Yahoo! sponsors. With this type of business model, the more services provided, the more ads that can be sold, and in theory make more money made for Yahoo! Striving to make more money (actually in recent years just to break even), Yahoo! has added a abundance of services to its network. Yahoo! Groups is a new spin on Usenet. Providing groups of particular interest, users can leave messages and photos in a group that are viewed as HTML in a regular web browser. With the acquisition of GeoCitites, Yahoo! users can now host their own personal web pages on Yahoo! Yahoo! Mail is still one of the largest and most used free mail services. Yahoo! has even gone mobile by providing many of its services to cellular phone users. These are just a highlight of the many services offered by Yahoo! Lastly and most important to this paper is Yahoo! Messenger. Yahoo! Messenger provides a widely popular instant messenger service to Yahoo! customers. http://www.ycoderscookbook.com/
-
Ever since I read this article I have been looking for the right Coke machine. Too bad I haven't found one. Great article.
-
A few obversations:Is this a UK only site/service?The second is never underestimate the stupidity/ignorance/nieveness of computer users. After following computer security for a few year it still absloutly suprises me how unprotected many Internet users are. Only by sheer luck do many users dodge the bullet of complete system comprimise. The information may be basic to us and can be found virturally anywhere on the web, but to some users this information needs to be blasted to them. All Internet users need a level of education when it comes to computer security. The more places the better. Perhaps this one page can save a few people for certain Internet doom.In short, I like it. This should be plastered in more places.
-
I would like to know how may of you use Gaia Online at http://www.gaiaonline.com/. I came across Gaia by accident when a friend told me about it. I don't really have much interest in such things as I don't have enough time to do the things I want to do as is. My interest was sparked when I signed up for an account and noticed that you get gold every time you move to another page or even refresh the same page. Now we are talking about a site that might interest me.Of course I had to try it out and the result is a nice little program that I am about to finish up in the next few days. With a little creativity and programming I was able to get a little over 2.2 million gold in under two weeks.I just want to know how many people here have ever used Gaia and what are your opinions. Also would anyone be interested in making alittle gold on the side?I thought that this thread might get at least a small reaction, guess not
-
I am talking about the HTTP header meathod PUT. http://www.w3.org/Protocols/rfc2616/rfc2616-sec9.html section 9.6. I need to quickly store a log file generated by a remote machine without the use of FTP.
-
Does Xisto allow http PUT requests. I am writting a program and I am looking for a quick simple way to transfer a file to Xisto. I would like to use PUT but I'm not sure if Xisto is allowing it. The directory that I am working with has world write access.If Xisto does allow PUT then how would one enable it?
-
Thank you, I will put the request in soon. Of cousre SSH is fine, just was wondering about a telnet type of access.
-
Is There Anyway To Get Telnet Access?Here is another crazy request. I would like to run the Entropy Search index builder every couple of days or so. Is there any way to schedule this as a CRON job?
-
Has anyone updated to phpBB 2.0.18 yet? If so I would like to know the simplest way to do so. I found the update only files on the phpBB site but I not really sure if I have telnet access.
-
Ms Sec. Advisory: Flash Player 7 Vulnerability
tansqrx replied to jedipi's topic in Security issues & Exploits
I too was kinda wonder what was going on when Microsoft released an advisory for a non MS prodect. I wonder if MS will allow other vendors to do the same or if this was a one time special deal. Another thought, will MS eventually push other vendor's patches via Windows Update? -
Part 2 - History The need for humans to communicate faster and more efficiently has been one of the driving forces behind the Internet. Not since the invention of the telephone has communications between humans been more readily available. The communication power of the Internet began to take shape in its infancy with one of the first Internet applications, email. While the Internet was still ARPANET and with only four links, the first email message was sent by Ray Tomlinson in 1971. The first message consisted of the text âTesting 1-2-3â and did not contain any of the features associated with modern email. Even with its humble beginnings, within twelve months of the first message most of the features associated with modern email had been incorporated [http://www.let.leidenuniv.nl/history/ivh/chap3.htm]. One problem with email is that it only permits one to one communications. If a person needs to get his idea out to a large number of recipients then he has to use email lists. Even then, it is not guaranteed that everyone that wants access to the information can get it. This led to the next great communication medium for the Internet, Usenet. Usenet is a type of email list that is stored locally on servers. This allows users to access any group they want, read all the messages, all without having to be included in a mass email list. The first signs of Usenet appeared in the summer of 1979 when Tom Truscott and Tim Ellis presented the idea for Usenet at a meeting of Academic UNIX User Group. Within a short time period, the idea caught on and Usenet became one of the major foundations for the Internet. Email and Usenet were the early predecessors for computer communication but a large hole still existed for real time communication or chat. IRC was one of the first to fill this void. Building on BBS style chat applications, Jarkko âWiZâ Oikarinem created Internet Relay Chat or IRC in the summer of 1988 [https://daniel.haxx.se/irchistory.html] Based on a centralized server system, IRC users pick a username and password and then login to an IRC server. The IRC architecture provides real time chat in chat rooms as its primary feature. Additional features include PM or Private Message to a user, DCC file transfer to a user, and other UNIX style commands. IRC now consists of several major networks such as Undernet, Dalnet, and EFnet, all of which support tens of thousands of channels. Starting in the mid 1990âs, several technologies started, mixed, joined, broke up, and fused to create the modern instant messaging experience. ICQ was the first instant messaging application on the market. ICQ which stands for âI seek youâ was developed by Israeli company Mirobilis and released in November 1996. Shortly afterwards, America Online introduced another messaging client called AIM or American Online Instant Messenger [http://forums.xisto.com/no_longer_exists/] AOL had a similar messenger service for members only, which one AOL user could IM or instant message another AOL user. This service soon became so popular among AOL users that AOL expanded the service to non-AOL users. With such a popular technology evolving and a lot of money at stake, law suits began to emerge. A battle between AOL and ICQ broke out over the right to instant message. In December 2002, AOL Time Warner announced that ICQ had been issued a United States patent for instant messaging. In turn, the term âinstant messengerâ is a service mark for AOL Time Warner and can not be used by any other company or program [http://forums.xisto.com/no_longer_exists/] This all became a moot point because AOL Time Warner acquired ICQ in the summer of 1998 [https://icq.com/windows/de] Along the way many other instant messenger type services have popped up. A sampling of a few more common messengers is Yahoo! Messenger, Microsoftâs .NET Messenger service and several open source attempts at making a universal messenger system [http://forums.xisto.com/no_longer_exists/ ] http://www.ycoderscookbook.com/
-
One of the security passions that I have maintained over the past few years is the one with Yahoo! Messenger. In recent months Yahoo! Messenger has seen a decline in users due to some new policies. Although not as strong as perhaps a year ago, it is still very important to keep a watch on Yahoo! Messenger from a security point of view. Messenger, just like may of the programs we use, open a door out to the Internet. With each new door comes a unique set of security concerns. Perhaps the biggest reason to keep an eye on Yahoo! Messenger is because the user base is so large. What if you can exploit Messenger, how many million users would be affected? The upcoming tutorial has been a work of mine for several years. It covers the basics behind the Yahoo! Messenger protocol. The protocol is closed source so you will for the most part not find the details on the web and certainly not from Yahoo! If you ever wanted to know what happens when you login to a Yahoo! server, the password hashes used, or how an IM works then this is the place for you. The tutorial is long, well over 60 pages in Word. I will try and keep it all in the same thread to prevent spamming by myself. I will break the tutorial into several parts that will cover several months. After the years of hard work I donât think I will only get five days worth of credit out of this one. If the moderators feel that it should be put into larger sections so be it. Also if the moderators wish for me to do anything different please feel free to let me know. PART Ia - Introduction This section of my Yahoo! tutorial introduces you to the reason why I am studying Yahoo! and why I know/donât know what I do. Hopefully this will give you a small insight into my world of Yahoo! My love/hate affair with Yahoo! Messenger started only less than two years ago. By that standard I shouldnât know that much about Yahoo! Messenger, as many friends I know have been using Yahoo! Messenger for many times that many years and still donât know the first thing about Yahoo! Messenger. For some odd reason, Yahoo! Messenger took hold over me and I had to know more about it. I have used online IM services since the time AIM opened up to non-members which was who knows how many years ago. After a few short weeks of playing around with AIM the fancy of instant messaging soon lost its luster. The fact was that everyone on my buddy list was a close personal friend and if I wanted to talk to them then I would just call them on the phone. A second reason AIM soon lost its intrigue was that only a select few of my friends owned a computer and none of us even dreamed of DSL or cable connections. Now fast forward a few years. I have since expanded my interest in computers and have even made a living at knowing the ins and outs of computers. I have learned several computer languages and work with computers constantly. Three almost separate events brought Yahoo! Messenger into my life. The first was a friend that told me to contact him on Yahoo! Messenger to arrange a game of Starcraft one night. This of course caused me to download Messenger and start playing around with it. The second event involved a run-in with a booter in one of Yahoo!âs chat rooms. After being booted several times I became irritated to say the least. This behavior really didnât surprise me or cause much alarm as I have used IRC for several years and was already familiar with some of the nastiness that other users can throw my way. As a side note, I was actually fairly amazed at how benign Yahoo! attacks were. On IRC where a userâs IP address is freely available, much nastier attacks take place, usually within ten seconds of signing into a server. Finally the third factor in my Yahoo! odyssey involved my passion for computer security. Over the years I have always been fascinated with computer security. This aspect of my life really took off when I started taking a series of computer security courses. In the fall of 2004 I enrolled in a class entitled âInformation Assurance âI.â Information Assurance has to be one of my all time favorite classes that I have ever taken. The class was pretty much a how-to hack course; the basis of course was that you had to know how the enemy works. This combined with two of the best teachers that I have ever had, created an educational experience that will truly affect the rest of my life. One of the requirements for this class was to do a research project on any security aspect that I chose. The combination of playing around with Yahoo! Messenger for the previous few months, being booted, and having to write a paper about a security issue was the perfect spark to start my voyage into Yahoo! Messenger. Much the information contained within this tutorial is my findings from my security class. In the âInformation Assurance âIâ class I wrote a paper entitled âYahoo Booters.â I then took the second part of the class (âInformation Assurance âIIâ) in the spring of 2005 and continued my Yahoo! research with a second paper not surprisingly titled âYahoo! Booters Part II.â The first paper was a survey of Yahoo! Messenger, instant messaging history, a brief look at the Yahoo! Messenger protocol, and some of the common booters. The second paper went much deeper, investigating if remote arbitrary code execution was possible through existing booter methods. The second paper required me to make my own Yahoo! Messenger client and integrated booter. After creating a client, I spent countless hours debugging Yahoo! Messenger with OlyDbg after a boot packet was sent to it. All of this resulted in a mapping of most of the common Yahoo! protocol packets and much valuable information. One last question remains. Why am I going through all the trouble of publishing the information that I worked so hard to obtain? The first answer is I just suppose that I am a nice guy. The fact is that as hard as I tried I could not find any good information about the Yahoo! Messenger protocol on the web. The closest I came was a site that had a few packet captures from the login for version 11 [http://forums.xisto.com/no_longer_exists/ ] (the current version is 12.) Besides that I had little to nothing to work with. Of course Yahoo! itself will not publish any protocol information because it is a closed standard and Yahoo! uses Messenger to make money. I had to resort to firing up Ethereal and packet sniffing each packet as it traveled on the wire. If I can help anyone else out in the same situation, I will gladly give what I have learned. The second reason that I am going through all this trouble is I still need information myself. As with any good research, the more rocks you turn over, the more questions you are left with. I have so many Yahoo! questions that I could keep a team of programmers busy for two years to find the solutions. The whole point of this website is to create an open exchange of ideas. I canât do it alone. Maybe if I provide enough information, someone with more knowledge than I will feel sorry for me and answer some of my questions. Enough about me, lets get into some internet and instant messaging history. http://www.ycoderscookbook.com/
-
Unfortuntally I am viewing it in IE, I have even cleared the cache several times.
-
I have been playing around with phpbb2 2.0.17 trying to get a new style going. I have followed the directions at http://www.phpbbhacks.com/ Creating a Template With subSilver [ http://www.phpbbhacks.com/forums/viewtopic.php?t=13169 ] and made a new style. Everything looks fine with the new style, it has been added and I can edit it from the Styles Administration page. I can even make changes under Edit Theme and the changes show up in the forum. The problem comes when I try to customize the forum past the few things shown in Edit Theme. For starters I would like to change the scroll bar colors. I have changed <new theme>.css located in the template/<new theme> directory. The obvious answer would be to change the following. body { background-color: #0e7a8e; scrollbar-face-color: #23898e; scrollbar-highlight-color: #ffffff; scrollbar-shadow-color: #000000; scrollbar-3dlight-color: #63798D; scrollbar-arrow-color: #ffffff; scrollbar-track-color: #085766; scrollbar-darkshadow-color: #000000; color: #FFFFFF:} I have changed this and uploaded to the proper directory with no results. Do I have to do anything else or should this work?
-
I have an older post that I would like to edit (http://forums.xisto.com/topic/84321-topic/?findpost=1064306363). I need to move the location of the images and I don’t want to destroy the tutorial. Its likely been posted 10 bazillion times and the answer is right under my nose but maybe someone could help me out on this one.