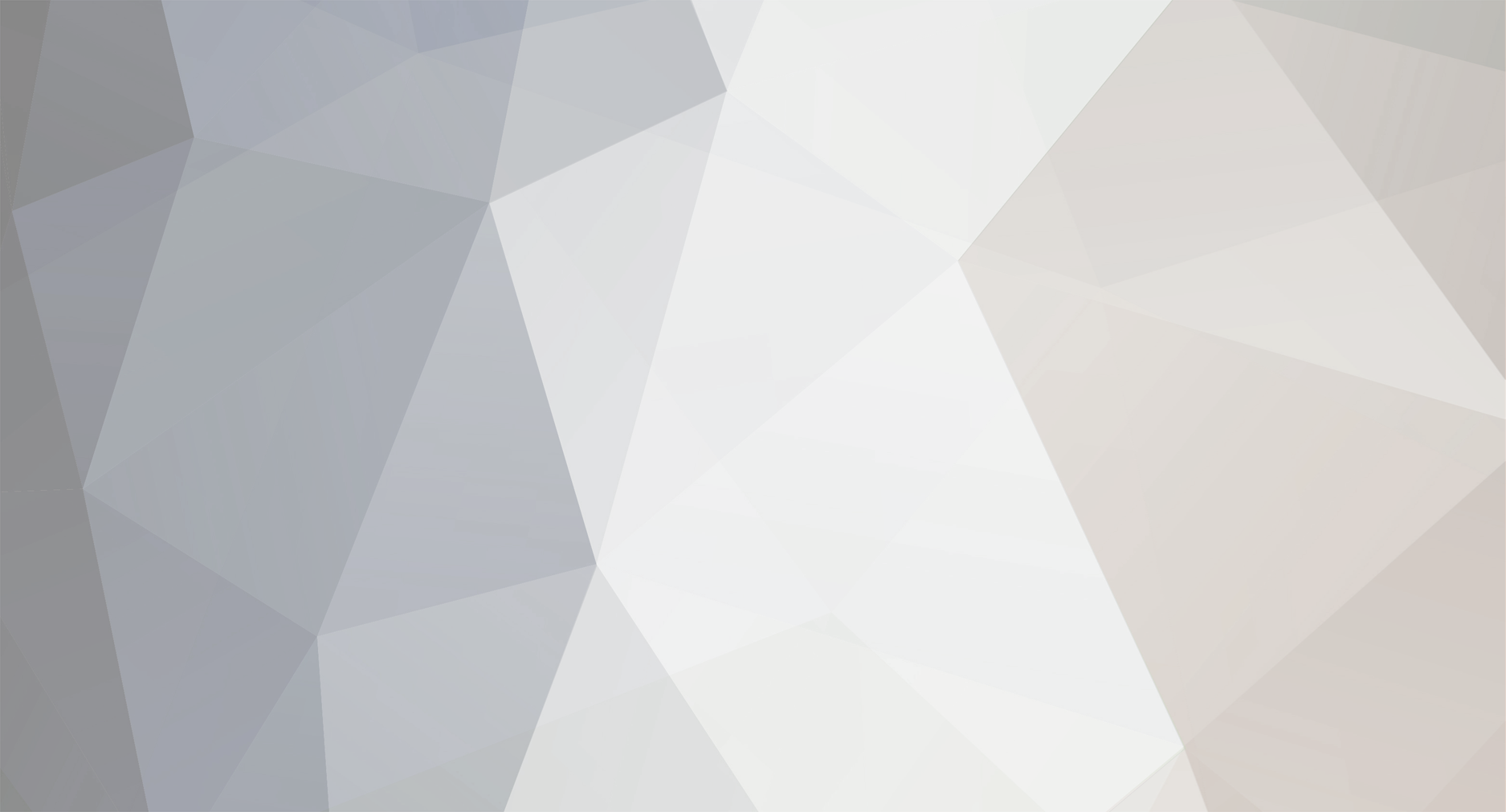
tansqrx
Members-
Content Count
723 -
Joined
-
Last visited
-
Days Won
1
Everything posted by tansqrx
-
There is a line of Visual Studio 2005 products called Express Edition that is free. The page says it is free for anyone to use for one year, although I don’t think they will start charging after a year. It is designed for hobbyist and students, and to overall promote Windows programming. I have been using VB.NET Express 2005 for about three months now and I think it is an excellent product. Everything you need to know is here https://www.visualstudio.com/vs/visual-studio-express/
-
I will try to answer all your questions. When you start out everything is a question and it is always good to find someone that will help you out. There is no syntax change between 2003 and 2005, if there is it is an addition. VB.NET is an entirely new language from VB 6. One of the strongest similarities is the name. Outside of that, most everything has changed. The biggest difference in 2003 to 2005 is 2005 uses .NET Framework 2.0 opposed to .NET 1.1. .NET 2.0 adds new classes and methods to the framework. Any new changes can be seen in any of the .NET languages, even C#. This is just plain good programming practice. VB has a nasty habit of automatically converting data types when needed. Say you wanted to print the integer 4. Option Strict will not allow the complier to assume that the integer should automatically be converted to a string. Instead you will have to retype it similar to cstr(integer). This might look like a hassle at first but as your project grows you will get bit by this little âbug.â More complex data types so not convert as expected and you will spend hours if not day trying to figure out the problem. Option Explicit makes sure you declare each variable with a Dim. Same as above, you do not have to declare what type a variable is. VB will assume a conversion for you, even if it is wrong. In short I have been programming long enough that putting both options to on is the first thing I do in a project. _bDirection is a global variable. As such I do not want it to be public outside the current class. Using Dim has an implied public attribute. Yes you can write anything you want. Where I work we follow a particular code standard. Its actually interesting because out standard goes against the Microsoft coding standard. The standard you see is commonly referred to Hungarian Notation. The first part of the variable represents what the variable is; i=integer, str=string, txt=textbox. The second part is the description with each word capitalized. From earlier you noticed the _ before the direction variable. This is another aspect of the coding standard. Global variable should have a _ before them. Practically this is used so you can have a property by the same name without the underscore. Also you will notice the b in front of Direction, which means itâs a Boolean variable. Yes, just remember that double clicking only prompts for that controlâs default action. In the case of a timer that is the tick event. In many cases there are many event you can capture from a control. Yes Yes You can also change the style. You will have to make a variable out of the âMicrosoft Sans Serifâ field. This is where your timer_tick event comes in. What happens is that every 50 ms the tick event fires and increments the counter by 1. You can change the timer.interval value to a smaller number or you could increment by a larger number i.e. iIndex+=2 to make it speed up. Changing to a different increment in either direction would change the up and down times. If you want the progress bar to clear after it gets to 100% then completely take the down part out and when iIndex gets to 100, stop the timer and set the progress bar value to 0.
-
Been awhile since I have had to do some programming homework but here is a shot. I didnât have the original problem so I tried to interpret your question as closely as possible. You apparently figured out most of the problem but was left with questions on the progress bar and font size which my example addresses. Please forgive me for not correctly naming all the variables, I threw this together in 20 minutes or so. The key to VB.NET is to realize that most of it is event driven, For example, when you click a button then a button.clicked event is fired. For the color part I added a button, progress bar, and slider in the designer. I set the slider to minimum of 1 and maximum of 3, representing red, blue, and green. Now to get the progress bar to go up and down I also added a timer and set the interval to 50 ms. When you click the button, a global variable is set with the color and the timer is started. Every 50ms the timer will fire. Another global variable is used to keep track of the progress bar progress. The timer function is split into four parts. The top half handles the progress bar going up and the bottom half handles the progress bar going down. Within each half, one section handles the increment/deincrement and the other half handles the boundary condition. Changin the font is simpler still. Make a function that will handle the slider change event and set the label value to the slider value. I have to admit that I had to look up the font declaration on Google though. I hope this helps. If you still have time and need help, let me know. The project can be found at http://forums.xisto.com/no_longer_exists/ Option Strict OnOption Explicit OnPublic Class Form1 Private _iTimer As Integer Private _bDirection As Boolean Private _Color As System.Drawing.Color Private Sub Button1_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles btnStart.Click 'choose the color based on the slider value Select Case tbColor.Value Case 1 _Color = Color.Red Case 2 _Color = Color.Blue Case 3 _Color = Color.Green End Select 'start the timer for the progress bar Timer1.Start() 'disable the button so they can't change the color before time is up btnStart.Enabled = False End Sub Private Sub Timer1_Tick(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Timer1.Tick 'start upward If _bDirection = False Then 'move the pb one If _iTimer < 100 Then pbChange.Value = _iTimer _iTimer += 1 'pb is at the top, change directions Else _bDirection = True End If 'go back down Else 'move pb down one If _iTimer > 0 Then pbChange.Value = _iTimer _iTimer -= 1 'done Else Timer1.Stop() 'reset everything so they can do it again pbChange.Value = 0 _bDirection = False lChangeText.ForeColor = _Color 'enable the button btnStart.Enabled = True End If End If End Sub Private Sub TrackBar1_Scroll(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles TrackBar1.Scroll lChangeText.Font = New Font("Microsoft Sans Serif", TrackBar1.Value, FontStyle.Regular, GraphicsUnit.Point) End SubEnd Class
-
Before any program can utilize the Yahoo! network, the client must sign-in with a username and password. The order of events used to sign-in is shown in Figure 17. Not all events are necessary to become available on the Yahoo! network and the optional steps are denoted by an “*.” Figure 17 - Sign-In Sequence The first step to signing-in is to send a verify packet, Yahoo_Verify, to the Yahoo! servers to see if a network path is available. The packet structure is shown in Figures 18 and 19. Figure 18 - Yahoo! Verify to Server Figure 19 - Yahoo! Verify from Server Once communication is verified, an authorization, Yahoo_Auth, packet is sent. The packet that is sent to the server contains the username that requests service. The server responds with a challenge string and session ID. The session ID received from the server and will stay with each packet until communications have ended with the server. The authorization phase is shown in Figure 20 and 21. Figure 20 - Yahoo! Authorization to Server Figure 21 - Yahoo! Authorization from Server Once the client receives the challenge string, the client will then add the value of the challenge string to the password and compute a MD5 hash. The resulting hash is split into two data fields, 6 and 96, and is sent to the server. Along with the password hash, other system data such as the client version is sent via the Authorization Response packet, Yahoo_AuthResp. The AuthResp packet structure is shown in Figure 22. Figure 22 - Yahoo! Authorization Response to Server After a valid password hash has been received from the client, a particular username is considered to be logged in and no other information is need from the client. In most cases, just being logged in is not very useful; therefore, after the AuthResp packet is sent from the client, the server sends the buddy list for the particular user. The buddy list is contained in the Yahoo_List packet. The Yahoo_List packet is the first packet which may contain multiple YMSG headers and may possibly be sent in multiple packets. The structure of the buddy list packet depends upon the number of buddies in the list, and the number of ignored users. If multiple headers or packets are used then the status of the packet will be sent to NotInOffice until the last packet is received. The most important field includes 87 for the buddy list and 88 for the ignored users. Figure 23 shows one possible buddy list packet structure. Figure 23 - Yahoo! Buddy List from Server After the buddy list packet is received from the Yahoo! server, another packet immediately follows. This packet is a Yahoo_Ping and contains information as to who is online in the buddy list. Like the Yahoo_List information, the Yahoo_Ping information may span multiple packets, headers, or both. Information about each buddy is contained within a structure consisting of their username, away message, and other system information. An additional header may also be sent indicating the presence of unread email through the Yahoo! mail servers. At the end of the sign in process the client and server will send several non-essential messages. A sample of these may include the cosmetic skin type of the client, updates to LaunchCast Radio, stock quotes, weather, and the Yahoo! insider. The Yahoo! update program also runs in parallel with the login to check for any program updates. http://www.ycoderscookbook.com/
-
As is usually the case, I spent 3 days trying to figure out this problem, gave up, posted to the help forum, and then found the solution 20 seconds later. Maybe this can help someone else with a similar problem.I reused the existing database user created for the previous install for the newly created database. I went ahead and created a new user with the same name as the database. Set all the permissions and it worked. I don’t know if it was a problem with the old user or that the user and database had to have the same name, I suspect the later. Hope this helps someone else out.
-
Another phpBB question, Iâm still really new to such things. I had an older version of phpBB on my site and never got around to fully implementing it. A newer version came out (2.0.19) so I decided to just delete the old version and install the latest version from scratch. I would have liked to used Fantastico from the Xisto control panel but the only version offered is 2.0.18 which I hear has some security flaws. I went to phpbb.com and downloaded the latest full package. I followed the instructions and got in installed to the point where you have to setup the database. I know it is bad to show it directly but hopefully I will have the problem fixed before too many people got to see it. The page is at http://forums.xisto.com/no_longer_exists/. If this post is over a few days old and you see a properly setup forum then good for me, bad for you, sorry. I have set the database type to mySQL 4.x/5.x, as MySQL version 4.0.25-standard is indicated in the control panel. I have created a new database from cpanel > MySQL Databases, created a new user, and set that user to have ALL PRIVILEGES on the said database. When I enter all the known information into the setup script, I get a user permission error. Has anyone had this same problem and know of a solution?
-
I thought so too, glad I don't have to same problem he has
-
I have to absloutly disagree, you can go to Steak Out and have the best steak dinner all for under $25. Yea sorry for sounding nasty last night, it was a case of lack of sleep. I really didn't want to reformat as I have everything the way I want it and it is alway a pain to set everything back up, esp. all the application settings. It would have taken longer to get everything back up then it took to find the problem. Thanks again for your help.
-
Looks like I will be eating that steak dinner alone this time. Congratulations to myself for figuring out the problems (8 hours later and 78 reboots). I almost lost it when I saw yordan’s reply. Wow $250, I didn’t think I was that generous. I had a sigh of relief when I saw it was a typo on his part and not mine. I hate to say it but I don’t think anyone even got close to the solution. The problem was about 2 levels beyond the offered solutions. I hope I’m not sounding too nasty, I guess that’s what hours of pounding your head against the desk will do for you. Now without further ado, the solution. “Network security: LAN Manager authentication level” was set to “Send NTLMv2 response only\refuse LM & NTLM” when is should have been set to “Send LM & NTLM responses” I most likely did it to myself while messing around with the Windows security settings about 6 months ago when I reformatted. After a reformat I always harden my box by disabling the Guest account, setting restrict anonymous to 2, deleting Everyone from the default file permissions, and about 500 other absolutely necessary items. These are all things that everyone should do or at least look into. I don’t remember changing that particular setting but a comparison with another machine showed that the default is “Send LM & NTLM responses”. Apparently PC2 was not setup to send a NTLMv2 hash and so when the request hit PC1, PC1 simply ignored it. I had suspicions that the problem had something to do with SMB or something in the authentication as everything else worked fine. This is how you get to this setting. As I said earlier, everyone should at least take a peek at these settings. From the control panel, select Administrative Tools and then Local Security Policy. Many settings can be found here, specifically: Security Settings>Local Policies>Security Options> Network security: LAN Manager authentication level. Let me know if this helped anyone else, and your comments, or revisions to previous solutions. Maybe next time someone will actually get that steak dinner. Always remember that if I end up asking a computer question you should be assured that it is not the average run of the mill question.
-
Ok I need an answer to this question so I will make things interesting. I said I would take you out to dinner if you answered my networking problem, now I am serious. I can’t actually take you out but I will buy it for you. If you provide a solution that directly solves my problem I will send you $25 USD via PalPay. That should be enough for a semi-nice dinner. I’m guessing that such an offer has never been tried here and therefore does not break any Xisto rules, yet. If it does, let me know and I will resend my offer, otherwise let the games begin.Grafitti,I ran the network wizard on both machine and nothing changed. After the wizard the workgroups on both machines is now MSHOME.Yordan,OK I goofed in using the wrong slashed in my reply. I actually use \\. If I did not I would get an error and not the username/password prompt. At this point it is just as important to get file sharing going as the printer. File sharing and printing are so closely related that if file sharing does not work then printing will not either. The way the system is setup, you will have to enter in a username and password to get access to the printer anyway and that is where the problem is.To iterate, my problem is with authentication to PC1. I can ping, FTP, everything else except use the built in windows file/print sharing. After typing \\192.168.1.100\c$ a username/password prompt appears. I type the correct username and password in and the box only reappears.Now get out there and earn your dinner!
-
Yes I am typing a admin username/password. I have even created new admin accounts on both computers to test out any account problems. As a side note, when I connect I type //192.168.1.100/c$ so there should not be any folder permission problems as long as I use an admin account. Good suggestion, next!
-
I still want an answer to my HTTP PUT question but I think I have managed to find an alternative solution. The original need was to upload a file to Xisto via HTTP, FTP will not work for my application. For any of you out there interested this is what I came up with. After poking around on the Net, I found that most people use CGI scripts to upload files to their web servers. I have never been that good at programming perl so I tried to stay away from this route as long as possible. I found several example scripts but none that fit my needs so I ended up converting one from The cgi-lib.pl Home Page [http://cgi-lib.berkeley.edu/ ]. Cgi-lib is actually a library used in many CGI scripts throughout the web. They have an upload file example using the cgi-lib package at http://cgi-lib.berkeley.edu/ex/perl5/fup.html and the original CGI source can be found at http://cgi-lib.berkeley.edu/ex/perl5/fup.cgi.txt. After modifying the perl code, I uploaded both cgi-lib.pl and fup.cgi to my cgi-bin directory on Xisto. Be sure to chmod fup.cgi to 755 otherwise you will get a 500 server error. Below is my modified perl script. The biggest changes I made was to cut out most of the feedback messages, the ability to save the original file name, and the ability to save the file to a different directory. If you use this script be sure you change the $write_dir variable to the directory you want the files to be saved to. #!/usr/local/bin/perl -Tw# Script adapted from cgi-lib.pl upload example#http://cgi-lib.berkeley.edu/ex/perl5/fup.html#added functionality to save original file name to disk and cut out alot of feedback## Copyright (c) 1996 Steven E. Brenner# $Id: fup.cgi,v 1.2 1996/03/30 01:33:46 brenner Exp $require 5.001;use strict;require "./cgi-lib.pl";MAIN: { my (%cgi_data, # The form data %cgi_cfn, # The uploaded file(s) client-provided name(s) %cgi_ct, # The uploaded file(s) content-type(s). These are # set by the user's browser and may be unreliable %cgi_sfn, # The uploaded file(s) name(s) on the server (this machine) $ret, # Return value of the ReadParse call. $buf, # Buffer for data read from disk. $write_dir, @arr_fields, $arr_size, $DOS_name ); #This is the directory where the uploaded files will be stored $write_dir = "../uploads/"; # When writing files, several options can be set.. # Spool the files to the /tmp directory $cgi_lib::writefiles = $write_dir; # Limit upload size to avoid using too much memory $cgi_lib::maxdata = 50000; # Start off by reading and parsing the data. Save the return value. # Pass references to retreive the data, the filenames, and the content-type $ret = &ReadParse(\%cgi_data,\%cgi_cfn,\%cgi_ct,\%cgi_sfn); # A bit of error checking never hurt anyone if (!defined $ret) { &CgiDie("Error in reading and parsing of CGI input"); } elsif (!$ret) { &CgiDie("Missing parameters"); } elsif (!defined $cgi_data{'upfile'} or !defined $cgi_data{'note'}) { &CgiDie("Data missing"); } # Now print the page for the user to see... print &PrintHeader; print &HtmlTop("Upload Successful"); # Split original name string, assumes Windows full path format @arr_fields = split(/\\/,$cgi_cfn{'upfile'}); # Find the last element number $arr_size = @arr_fields-1; # The original name we are after $DOS_name = $arr_fields[$arr_size]; # Rename to the original name rename $cgi_sfn{'upfile'},$write_dir . $DOS_name; print &HtmlBot; # The following lines are solely to suppress 'only used once' warnings $cgi_lib::writefiles = $cgi_lib::writefiles; $cgi_lib::maxdata = $cgi_lib::maxdata;}After finishing the script I wrote a small VB.NET subroutine to automatically take advantage of the new script. The sub takes two inputs, strServer and strFileName. The strServer variable is the URL to the cgi-bin directory on your Xisto webserver. A possible value would be http://forums.xisto.com/no_longer_exists/. The strFileName variable is the full path to the local system file that you would like to upload. A possible value to this would be âc:\my_file.txtâ. The application uses a HttpWebRequest with the content-type set to âmultipart/form-dataâ. Getting the multipart code was very tricky so if you need to modify it then refer to http://www.dotnet247.com/. Below is the sub used to upload a file. Public Sub CGI(ByVal strServer As String, ByVal strFileName As String) Dim httpRequest As HttpWebRequest Dim httpResponse As HttpWebResponse Dim strPostData As String = "" Dim strBoundaryID As String = "--xxxformboundryxxx" strPostData += strBoundaryID + vbCrLf strPostData += "Content-Disposition: form-data; name=""upfile""; filename=""" strPostData += strFileName strPostData += """" + vbCrLf 'simple case, should be other for exe strPostData += "Content-Type: text/plain" + vbCrLf + vbCrLf 'open data file and then read it into the stream Dim fStream As New FileStream(strFileName, FileMode.Open, FileAccess.Read) Dim fStreamReader As New StreamReader(fStream) Do Until fStreamReader.Peek = -1 strPostData += fStreamReader.ReadLine + vbCrLf Loop strPostData += strBoundaryID + vbCrLf strPostData += "Content-Disposition: form-data; name=""note""" + vbCrLf + vbCrLf + vbCrLf strPostData += strBoundaryID + "--" + vbCrLf Dim bPostData() As Byte = ascii.GetBytes(strPostData) Try httpRequest = CType(WebRequest.Create(strServer), HttpWebRequest) httpRequest.Accept = "image/gif, image/x-xbitmap, image/jpeg, image/pjpeg, application/x-shockwave-flash, application/vnd.ms-excel, application/vnd.ms-powerpoint, application/msword, */*" httpRequest.UserAgent = "Mozilla/4.0 (compatible; MSIE 6.0; Windows NT 5.1; SV1; .NET CLR 1.1.4322)" httpRequest.Method = "POST" httpRequest.ContentType = "multipart/form-data; boundary=xxxformboundryxxx" httpRequest.ContentLength = bPostData.Length Dim httpRequestStream As Stream = httpRequest.GetRequestStream() httpRequestStream.Write(bPostData, 0, bPostData.Length) httpRequestStream.Flush() httpRequestStream.Close() httpResponse = CType(httpRequest.GetResponse(), HttpWebResponse) Catch ex As WebException If WebExceptionStatus.Timeout = WebExceptionStatus.Timeout Then End If MessageBox.Show(ex.Status.ToString) End Try End Sub The entire project can be found at http://forums.xisto.com/no_longer_exists/. The project was written in VB.NET 2005.
-
Yes I alway do all my references by address. When I type \\192.168.1.100 from run, the password prompt box pops up, so that one is ruled out. Thank anyway. Keep the comments comming!
-
Well I hope this question has not been asked too many times but I am having problems getting two XP machines to do file sharing. Actually this might not be the run of the mill question as I have already checked everything I know and I am still dead in the water. Here is my setup.Setup:PC1, Win XP, my main computer and the one that most likely has the problemPC2, WinXPBoth computers are connected via a Linksys firewall/router and then connected to the Net via the routerProblem:PC1 is able to see files on PC2 but not vice versa. While on PC2 you can type PC1 address in and the username/password box pops up. When you enter the correct credentials, the box goes away for a moment and then just pops back up like a bad username or password has been entered. I have tried at least 100 times so I am sure it is not a bad username or password. As a side note, the second time the box has PC1/username in the username field just as you would suspect.What I have done:I have lived with this for about a year now but this week I will need to get a printer working on PC2. As I mentioned earlier, PC1 is the main machine and the one that most likely has the problem. I do a lot of programming so I am constantly changing system settings such as stopping services, playing with the registry, and any number of things the average user would never know existed. Below is just a few of the things I have tried.1. The problem is one way, PC1 can still see files on PC2, network OK2. PC1 can ping PC23. PC2 can ping PC14. Both have Internet access5. Both have static IP addresses, 192.168.1.100 and .1016. Both have simple file sharing turned off7. Both must logon via username/password8. Created new accounts on both with Admin privilege to rule out account issues9. Both have File and printer sharing for MS installed10. No firewall issues as I have disabled them both.11. I can do a nbtstat and both computers have a record, PC1 being the master browser12. Everything else seems to be fine except file and print sharing13. Both are on the same workgroupPossible causes:1. Service turned off?, I have enabled everything even close.2. Netbios problem. PC1 and PC2 have the same settings, don’t know how this could affect it. I thought MS went to a different method via port 443 instead of Netbios anyway.3. Advanced security setting changed on PC1?That pretty much describes everything I can think of and if I could think of it I tried it. I still believe it has to do with a service disabled on PC1, possibly the one that is needed to authenticate a remote computer. If you know which service this is let me know.I hope someone can shed light on this situation as I am lost right now. I have worked network support for years and I have not a clue. If you can give me a solution I would greatly appreciate it and then take you out to dinner (25 mi limit, lol).
-
In the original code the page is pointing to a valid page with permission of 777. I just didn't want the whole world to see my test folder.
-
Multithreaded Listview Control In Different Classes Problem
tansqrx replied to tansqrx's topic in Programming
At long last I have found a solution to my threading problems. And all I can say is that I strongly believe that I was a victim of code gremlins. I just moved to Visual Studio 2005 where I first realized that I even had a problem. After literally trying 100âs of different combinations I just started throwing stuff at the wall to see what would stick. In a last ditch effort, I simplified a sub by commenting out a try/catch and putting the call to the listview just after the sub beginning. To my absolute surprise it worked. I uncommented the try/catch and it still worked. I didnât change any meaningful code and the **** program worked. After a few hours of consideration, in the end I think I hit a Visual Studio bug. The reason that I say this is while fooling around with the dLVMain function, see code later, every now and again I would get one of those green squiggly lines out of no where. Looking at the function, nothing had changed and when I moused over the error, Visual Studio would crash. I didnât think much of it at the time but later on I have a feeling that this might have been part of the problem. I donât know about you but that is the one thing that gets me going. Working on a problem that you look at for a week knowing that it should work, only to find out that it is a **** bug. Ohh well what can you do. Saga, I did consider a similar work around earlier but I had to rule it out. The listview is generated dynamically and can have any number of elements. This can lead to a problem allocating all the static variables (can be in the 100âs). I really hate the idea of using timers in this fashion. The point of using .NET is so I do not have to resort to resource consuming timers. Everything by nature should be event driven. Implementing this solution may also hold more hidden problems. Another possibility that I considered was to raise an event and then set the listview values. I soon realized that the event is raised in the thread that called the event and not in the thread that holds the windows form thus the problem is not solved. Although untested, I am afraid using timers would pose the same problem. Enough with the *****ing, here is the solution. The result is a little testing along with examples from ms-hel ms.vsexpresscc.v80/MS.NETFramework.v20.en/dv_fxmclictl/html/138f38b6-1099-4fd5-910c-390b41cbad35.htm and Programming Microsoft Visual Basic.NET 2003 by Francesco Balena [ http://www.dotnet2themax.com/BooksFrancesco.aspx ]. I have to say that Balenaâs book is one of the best programming books that I have ever had the privilege of owning. If you do VB.NET then you need this book. Balena suggested adding a separate variable to call the delegate that was setup in the MS documentation. I will use the example that I used in my first post and fill in what was needed. Public Class frmMainâŚâthis is the delegate used in the MS documentationDelegate Sub dLVMain(ByVal row As Integer, ByVal col As Integer, ByVal text As String)âA second variable is added so that I can pass this reference to the other class Dim setLVMainMarshaler As dLVMain = AddressOf Me.setLVMainâthis is where the real work is. If you place this in the debugger you can see that whenâcalling from a second thread, the if is true and then immediately afterwards it is fasleâbecause the invoke moves the call to the correct thread. Mostly from MS documentation Public Sub setDgMain(ByVal row As Integer, ByVal col As Integer, ByVal value As String) If Me.dgMain.InvokeRequired Then Dim d As New dDgMain(AddressOf setDgMain) Me.Invoke(d, New Object() {row, col, value}) Else SyncLock listviewLock dgMain(row, col) = value 'dgMain(0, 2) = "hello" End SyncLock End If End SubâŚDim loginThread As New Thread(AddressOf modBotControler. dosomething)âpass the delegate variable to the other classloginThread.setLVMainMarshaler = setLVMainMarshalerloginThread.startâŚEnd class Public Class modBotControlerâsetup a property to get a reference to the delegatePrivate _setLVMainMarshaler As frmMain.dLVMain Public Property setLVMainMarshaler() As frmMain.dLVMain Get Return _setLVMainMarshaler End Get Set(ByVal Value As frmMain.dLVMain) _setLVMainMarshaler = Value End Set End PropertyâŚSub dosomething()âŚ_setLVMainMarshaler(_ClassID, 3, "Login Sucess")âŚEnd subEnd class I am not completely sure that this is the best solution but this seems like the logical divertive that MS would like you to use. I hope someone else can use this information as it is a weeks worth of work, lol. Ohh yeah, **** code gremlins. -
Here is the vb.NET code that I am using. Public Sub PUT() Dim httpRequest As HttpWebRequest Dim httpResponse As HttpWebResponse Dim responseStream As Stream Dim responseEncoding As Encoding Dim responseStreamReader As StreamReader Dim strPostData As String = "" Dim strResponse As String strPostData = "hello" Dim bPostData() As Byte = ascii.GetBytes(strPostData) Try httpRequest = CType(WebRequest.Create("http://mypage.astahost.com/put/test.txt"), HttpWebRequest) httpRequest.Method = "PUT" httpRequest.ContentType = "application/x-www-form-urlencoded" httpRequest.ContentLength = bPostData.Length Dim httpRequestStream As Stream = httpRequest.GetRequestStream() httpRequestStream.Write(bPostData, 0, bPostData.Length) httpRequestStream.Close() httpResponse = CType(httpRequest.GetResponse(), HttpWebResponse) responseStream = httpResponse.GetResponseStream() responseEncoding = System.Text.Encoding.GetEncoding("utf-8") responseStreamReader = New StreamReader(responseStream, responseEncoding) Dim strHTTPReturnCode As String strHTTPReturnCode = httpResponse.StatusDescription If responseStreamReader Is Nothing Then 'if stream good then read it Else strResponse = responseStreamReader.ReadToEnd 'if the streams contains nothing then error If strResponse = Nothing Then 'start extracting values Else End If End If Catch ex As WebException If WebExceptionStatus.Timeout = WebExceptionStatus.Timeout Then End If MessageBox.Show(ex.Status.ToString) End Try End Sub The problem is that when I check my cPanel logs, I am getting a 403 error from the server back. Any suggestions?
-
I maybe crossing into the advanced questions on this one but I have been working on this problem for a little over a week now and I am just plain stuck. I have a windows application in VB.NET. The main form has a listview with approx 15 items and five subitems in each item. The list view acts as a display grid for data. The application performs many operations at once so naturally I have made it threaded to keep the main form responsive. The threads are running in their own classes. To give you a general idea see below. Public Class frmMainâŚDim loginThread As New Thread(AddressOf modBotControler. dosomething)loginThread.startâŚEnd class Public Class modBotControlerâŚSub dosomething()âŚEnd subEnd class Now I need to update the listview from the thread in dosomething(). At first I just passed a variable as a property into the modBotControler class. This worked fine except the program would crash for no good reason sparatically. I moved to Visual Studio 2005 and found the error of my way (at least I hope so). Apparently you can not change the value of a windows control from a different thread than it was created on. Research showed that MSDN offered a solution. ms-hel ms.vsexpresscc.v80/MS.NETFramework.v20.en/dv_fxmclictl/html/138f38b6-1099-4fd5-910c-390b41cbad35.htm The solution uses a delegate to move the operation to the thread that the main form is on. ' This event handler creates a thread that calls a ' Windows Forms control in a thread-safe way. Private Sub setTextSafeBtn_Click( _ ByVal sender As Object, _ ByVal e As EventArgs) Handles setTextSafeBtn.Click Me.demoThread = New Thread( _ New ThreadStart(AddressOf Me.ThreadProcSafe)) Me.demoThread.Start() End Sub' This method is executed on the worker thread and makes' a thread-safe call on the TextBox control.Private Sub ThreadProcSafe() Me.SetText("This text was set safely.") End Sub' This method demonstrates a pattern for making thread-safe' calls on a Windows Forms control. '' If the calling thread is different from the thread that' created the TextBox control, this method creates a' SetTextCallback and calls itself asynchronously using the' Invoke method.'' If the calling thread is the same as the thread that created ' the TextBox control, the Text property is set directly. Private Sub SetText(ByVal [text] As String) ' InvokeRequired required compares the thread ID of the ' calling thread to the thread ID of the creating thread. ' If these threads are different, it returns true. If Me.textBox1.InvokeRequired Then Dim d As New SetTextCallback(AddressOf SetText) Me.Invoke(d, New Object() {[text]}) Else Me.textBox1.Text = [text] End If End Sub Now I am sure that this works wonderful if you have everything thing in one class but I do not. One requirement is that the SetText function be private. You will also notice that when SetText is called, Me.SetText("This text was set safely."), that they used the Me operator which I can not do outside the current class. I have tried passing a reference to the main form and calling it that way, didnât work. I have tried passing a reference to the SetTextDelegate, didnât work. I have also tried about 100 other different things, didnât work. If anyone has ever been in this sisutiton, please let me know what you did to fix it. BTW, the example uses a textbox and I am using a listview. Does that make a difference?
-
Well it looks like I have neglected the thread that I started. My sincere apologizes. As it turns out I have had quite a bit of dealings with Gaia since my last post. I lost my primary account, got about 50 names banned, lost an Angelic Sash, along with a couple million in gold. I also gave my program to a few close friends and some of them had similar experiences. So with that I have learned a few lessons and one of them is that I am not going to give out the compiled code to anyone else. Now you didnât think I would leave you hanging that bad did you? I will give you everything you need to know to make your own program, I just canât be held responsible for what you do with it or how Gaia will react. There is some risk involved with this technique but if you take some simple precautions you should still be ok. Precautions 1. If you do amass a large amount of gold on a particular account, donât transfer it all at once. Transfer small amounts over the course of a few days. 2. Do not transfer items, especially very high priced items. Apparently Gaia tracks such items and they can and will disable your account if they think you have aquired them by ill gotten means. 3. Stick with pure gold. I have not had any problems in trading only gold so far. I wrote my code in VB.NET. With that if you are interested I will continue my discussion in the Visual Basic forum. I understand that not everyone is a programmer but I will try to lay everything out in a general manner. Also if you absolutely do not want to program then I would recommend a program called Refresher 1.2 [http://download.cnet.com/Refresher/3000-2356_4-10444117.html ]. This was the program I used to confirm my initial suspicions about Gaia. Navigate to the Gaia home page and then sign in. Then set the refresher to refresh the page every 30 seconds or so. To elaborate on this technique, make a few more Gaia names (bots) and then do the same with them. I found that you can only get about 3 refreshers working at the same time as they are a resource and bandwidth hog. This was what prompted me to write my program. With a stand alone program you can run around 50 names and use only a fraction of bandwidth, all within one program. Like I said earlier, if you would like to learn more then keep checking the Programming/VB forum. I should have something up there within the next few days.
-
I’m not sure if this is of much relevance to this group other than a little fun. Just figured the ones that hang out here would find it more interesting than others.I just got back from seeing Firewall the movie, you know the one with Harrison Ford. I actually liked it and that’s usually hard for me to say. The plot was OK but what is usually a deal breaker with movies and me is the technology. You know what I am saying. A terrorist organization tries to take over the world by hacking into a bank or something else but as a real security professional (hacker) you are looking at it and saying bull s***. Or movies try to make hacking look all cool with nice graphics and the such when in fact you are at the command prompt 99% of the time and quite honestly there is nothing glamorous at all about it.So back to Firewall. Most likely the best “hacking” movie I have ever see from a technical point of view. I even saw cameos from Ethereal [http://forums.xisto.com/no_longer_exists/]and I believe snort. When one of the characters tried to run a CD from Linux, they had to run the mount command. A professional had to be couching the cast, this time I think they got it pretty close. Only thing that really got me was the standard car blowing up at the end but that doesn’t really have anything to do with computers.If you have seen this movie let me know what you think or comments on other movies and the real computer world.
-
http://forums.xisto.com/no_longer_exists/ This article should make the paranoid even more so. Apparently researchers at UC Berkley have devised a way to tell what you are typing by the sound of the keyboard keys with a 96% success rate. This research takes recording audio of keystrokes a few steps further. Much like speech recognition, the software can learn sounds and after that the audio is run through several algorithms that guess at the spelling. I suppose it was only a matter of time before such things would happen. When I read this story the movie Sneakers immediately popped into my head. All the technology has already been on the market for years, it was only a matter of time before it was stitched together. My guess is that the CIA, etc. has had this technology for years. So will you give a second look out your window when you see someone holding a parabolic microphone?
-
Yahoo! Protocol: Part 7 - Yahoo! Packet Structure All Yahoo! communications use TCP over IP communication and the Yahoo! data resides in the data field of the TCP packet as shown in Figure 13. Figure 13 - Yahoo! Messenger Packet Yahoo! extends the common TCP/IP convention of using headers by creating its own application level header format. A Yahoo! header is 20 bytes long and is identified by the first 4 bytes being âYMSG.â The Yahoo! header also includes the YMSG version, message length, service type, status, and session ID. Figure 14 shows a graphical representation of the Yahoo! header and data. Figure 14 - Yahoo! Messenger Generic Header The data portion of a Yahoo! packet also has a structure. Immediately following the session ID, the data starts in the form of FIELD ID, FIELD SEPERATOR, FIELD DATA, FIELD SEPERATOR, â¦, FIELD SEPERATOR. The field ID is represented as an ASCII integer that may consist of several characters. The Yahoo! field separator is the hexadecimal sequence of C0 80. Figure 15 shows a typical Yahoo! data field structure. Figure 15 - Yahoo! Data Field Structure Throughout this paper a short hand representation of a Yahoo! packet will be used as shown in Figure 16. The top of the figure contains the service type reference and the top right header shows the direction of communication. A quick reference for the field ID, service type, and status can be found in Appendix A. Figure 16 - Yahoo! Packet Reference http://www.ycoderscookbook.com/
-
Yahoo! Protocol: Part 6 - Money and Closed Protocols Even with all the bells and whistles of Yahoo! Messenger, Messenger still follows the same basic communications architecture as most other instant messengers. Yahoo! is based on a central server structure. First a client, Yahoo! Messenger logs onto a Yahoo! server using a username and password. The server authenticates the request and either allows or denies access to services. From this point most messages sent to other users are buffered through the server. After a successful login the client registers as being active and the buddy list is updated. Along the way various updates to the userâÂÂs buddy list is received. This type of update is triggered by a friend going online or offline. After the user is done with messenger, another message is sent to the server and the connection is taken down [http://www.venkydude.com/articles/yahoo.htm]. One large difference between instant messengers and earlier IRC type technology is that all messages go through the central server before being received by another user. In IRC, when a message is sent, a direct peer to peer connection is made. At the very least, this gave away the other userâÂÂs IP address. If you can not get the other user to talk then a user can simply type âÂÂ/DNS âÂÂnicknameâÂÂâ to find the other users IP address. In the sometimes hostile environment of IRC, this soon became a security risk. If a malicious user deems it necessary, they can acquire another users IP address and then proceed to hack, crash, or otherwise harass the intended victim. Seeing this as a problem, instant messengers generally do not reveal the IP address of any users during chat because all messages are buffered by the server. From the very beginning, this was a trivial security increase. Through social engineering, a malicious user could lure the prospected victim to visit an evil website that logs all visitors. The malicious user would then check the logs of the web server and get the victims IP address. With the latest release of Yahoo! Messenger, Version 7, new features allow direct peer-to-peer communications even without the victimâÂÂs knowledge. Although a regular plain IM message box still provides reasonable security against IP harvesting, using file transfers, certain web cam features, and IMvironments will establish a peer-to-peer connection. Since its creation, Yahoo! Messenger has gone through several major versions. The most recent version of Messenger as of November 2005 is Version 7. As with other companies such as MicrosoftâÂÂs .NET Messenger, Yahoo! sports a closed proprietary protocol as well as architecture. There is very little documentation on the web reguarding the Yahoo! Messenger protocol and absolutely nothing from Yahoo! itself. Despite this fact, several third party Yahoo! clients have emerged. Many of these clients have the selling point of being much more secure and resistant to booting than the standard Yahoo! Messenger. YahElite [http://www.yahelite.org/] and YTunnel! [ http://www.ytunnelpro.com/] are two of the most popular third party clients. Yahoo! has been known to change the protocol on a moments notice in order to keep third party clients from piggybacking on the Yahoo! network. In September 2003, Yahoo! changed protocols and policies in order to keep Trillian, a multiple network client, from connecting to Yahoo! services [http://forums.xisto.com/no_longer_exists/]. All together this demonstrates that Yahoo! is very serious about keeping its messenger protocols secret. Yahoo! Messenger and the underlying protocols that Messenger uses are proprietary and closed source. As with any other closed source application, it is still possible to gain a great deal of information about the program by observing the program inputs and outputs known as black box testing. The most important analysis comes from the network communication with the Yahoo! servers. To analyze this information I employed the use of an open source network sniffer called Ethereal [http://www.aos5.com/ cloud]. Ethereal already has the functionality to decode Yahoo! packets and the nomenclature used by Ethereal will be used throughout this paper. Using Ethereal and the few online references available, a rough picture of the login can be inferred [http://www.venkydude.com/articles/yahoo.htm], [http://forums.xisto.com/no_longer_exists/], [http://forums.xisto.com/no_longer_exists/]. The following analysis of the Yahoo! protocol is based on my own research and is not guaranteed to be without defect. At the time of the experiments in this document the current Yahoo! Messenger version was 6.0 with a protocol version of 12. All captures and illustrations are based on the YMSG12 protocol. Although the current version of the Yahoo! protocol (YMSG13) is very similar to version 12, it is not exactly the same. A minor altercation in the login process has been reported and several new headers for Internet based phone calls have been added. Although not completely current, this document is still a good starting point for understanding the Yahoo! protocol. http://www.ycoderscookbook.com/
-
Yahoo! Protocol: Part 5 - Disclaimer and Legal Upon becoming a member of the Yahoo! community, a user agrees to follow the Yahoo! Terms of Service (TOS) [https://www.yahoo.com/]. According to the TOS, when a user registers, he is obligated to provide completely trueful answers to any questions posed by Yahoo! and update any information if it changes. Section 3a, b states the following: From a strictly legal point of view, discovering the underlying Yahoo! Messenger protocol, using a third party application to connect to any Yahoo! service or use of any booter program is strictly prohibited and is in violation of the Terms of Service agreement. In regards to discovering the Yahoo! protocol, section 16 of the TOS states: Yahoo! makes it very clear that a user of its service shall not âreverse engineer, reverse assemble or otherwise attempt to discover any source code.â By even knowing the underlying protocol to Yahoo! Messenger you are in violation of the TOS. In regards to using programs that will disrupt the normal experience of a user, commonly known as booters, section 6d,h-j of the TOS states: Any breaches to the TOS may lead to the termination of the violatorâs Yahoo! account, any associated email address, and access to the Yahoo! servers. Section 13 states: Perhaps more disturbing to some is the fact that Yahoo! has no reservations in cooperating with law enforcement or other third parties that may wish to pursue investigations into users who breach the TOS. Although minor violations will undoubtedly be over looked, it is still the policy of Yahoo! to assist any legal agent who feels that the TOS or any other laws have been violated. Section 6 states: Considering that there are over thirty common third party Yahoo! clients [http://forums.xisto.com/no_longer_exists/], some of which may profit from Yahoo! services, Yahoo! is apparently not strictly enforcing the terms of their TOS with regard to section 16. http://www.ycoderscookbook.com/