Sign in to follow this
Followers
0
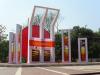
Conditional Statements Of Javascript Tutorial for beginers
By
Tourist, in Programming
By
Tourist, in Programming
Terms of Use | Privacy Policy | Guidelines | We have placed cookies on your device to help make this website better. You can adjust your cookie settings, otherwise we'll assume you're okay to continue.