Sign in to follow this
Followers
0
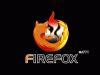
Java Lab 01 My first installment of Java tutorials
By
dyknight, in Programming
By
dyknight, in Programming
Terms of Use | Privacy Policy | Guidelines | We have placed cookies on your device to help make this website better. You can adjust your cookie settings, otherwise we'll assume you're okay to continue.