Sign in to follow this
Followers
0
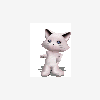
Simple Login In Visual Basic 6 user interaction example trough login programm
By
kitty, in General Discussion
By
kitty, in General Discussion
Terms of Use | Privacy Policy | Guidelines | We have placed cookies on your device to help make this website better. You can adjust your cookie settings, otherwise we'll assume you're okay to continue.