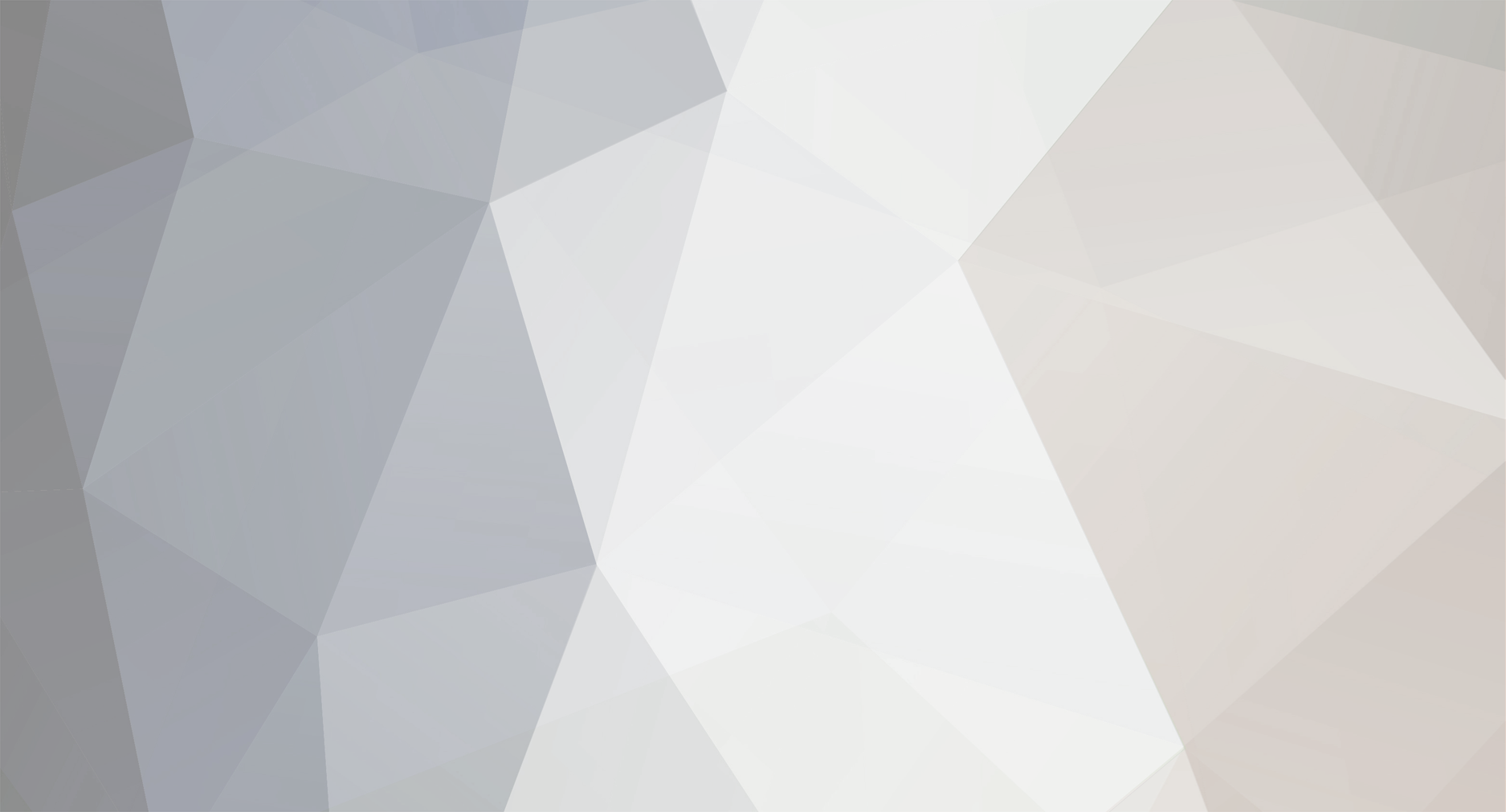
larryf
Members-
Content Count
3 -
Joined
-
Last visited
About larryf
-
Rank
Newbie
-
-
I did this for a Texas Hold'em game once... I found it pretty simple to represent the cards using a single integer. (You're only talking about 52 cards.. Come on..). So, for 4 suits, you need two bits. Then, value the cards from 1 to 14 using 4 bits. Now, you are only using 6 bits, even in byte you have left over data that you COULD use to hold simple flags like "Face Card" etc. Store your numbers in your array, and get the values like this: #define SUIT_SPADES 0x0 #define SUIT_CLUBS 0x1 #define SUIT_HEARTS 0x2 #define SUIT_DIAMOND 0x3 Then, as each byte represents a card, you could even define the mask values as such. #define SUIT_MASK 0x30 #define CARD_MASK 0xF Then, just AND these with your card values. So, the Ace Of Spades would equal 1, the ace of clubs would equal 17, the ace of hearts would equal 33 and the Ace of Diamonds would be 49. So, what would the Queen of Hearts be? 44... (Starting at bit 6, the value is 2 for Hearts, and 12 for the Queen. (A,2,3,4,5,6,7,8,9,10,J,Q,K). Larry
-
C++ Roman Numeral Conversion Code Help If-else Problem
larryf replied to Larry Rosario's topic in Programming
This code doesen't do Roman Numbers, but it does like Avalon talked about. It does it as 101="One Hundred and One", or if you pass the possessive to TRUE, will return "One Hundred First". Maybe this will give you some ideas. char *tens[] = {"", "", "twenty", "thirty", "forty", "fifty", "sixty", "seventy", "eighty", "ninety"};char *ones[] = {"zero", "one", "two", "three", "four", "five", "six", "seven", "eight", "nine", "ten", "eleven", "twelve", "thirteen", "fourteen", "fifteen", "sixteen", "seventeen", "eighteen", "nineteen"};char *possessiveones[] = { "", "first", "second", "third", "fourth", "fifth", "", "", "", "", "", "", "twelfth" };char *possessivetens[] = { "", "", "twentieth", "thirtieth", "fortieth", "fiftieth", "sixtieth", "seventieth", "eightieth", "ninetieth" };CString NumberToText(long num, CString Retval, bool bPossessive){ long rem; if(num < 0) { Retval += "negative "; num = - num; } if(num >= 1000000000) { rem = num % 1000000000; Retval += NumberToText(num / 1000000000); if(!rem && bPossessive) { Retval += "billionth"; } else { Retval += "billion "; } if(rem) { Retval = NumberToText(rem, Retval, bPossessive); } } if(num >= 1000000) { rem = num % 1000000; Retval += NumberToText(num / 1000000); if(!rem && bPossessive) { Retval += "millionth"; } else { Retval += "million "; } if(rem) { Retval = NumberToText(rem, Retval, bPossessive); } } else if(num >= 1000) { rem = num % 1000; Retval += NumberToText(num / 1000); if(!rem && bPossessive) { Retval += "thousandth"; } else { Retval += "thousand "; } if(rem) { Retval = NumberToText(rem, Retval, bPossessive); } } else if(num >= 100) { rem = num % 100; Retval += NumberToText(num / 100); if(!rem && bPossessive) { Retval += "hundredth"; } else { Retval += "hundred "; } if(rem) { Retval = NumberToText(rem, Retval, bPossessive); } } else if(num >= 20) { rem = num % 10; CString ten; if(!rem && bPossessive) { ten.Format("%s ", possessivetens[num/10]); } else { ten.Format("%s ", tens[num/10]); } Retval += ten; if(rem) { Retval = NumberToText(rem, Retval, bPossessive); } } else { CString res; if(bPossessive && num < 6) { res.Format("%s", possessiveones[num]); } else if((bPossessive) && (num > 5 && num < 20 && num != 12)) { res.Format("%sth", ones[num]); } else if(bPossessive && num == 12) { res.Format("%s", possessiveones[num]); } else { res.Format("%s ", ones[num]); } Retval += res; } return Retval;} -
Here is an algorithm I wrote using the Rabin/Miller test to test for prime numbers. I even commented it years ago. This is the best way to test for primes, and it will give you all the primes up to MAXINT. n - 1 = 2km for some k > 0 and odd integer m. a = 1 < a < n - 1 We say that n passes the Rabin-Miller test for the base a if either the first term in this sequence is 1, or 1 occurs later in this sequence and is immediately preceded by -1. If the test fails, we know that n is composite, and describe the base a as a witness to the compositeness of n. The crucial extra information we can derive is that if (odd) n is composite, then at least 3/4 of the numbers a with 1 < a < n - 1 are witness to this fact. First calculate m, in C++ we do this by simply shifting the bits right k times, until m is odd and less than n. Next, we calculate a, such that a is less than n - 1, and a > 1. Then, we calculate a ^ m mod n. If this result is 1, then we need to generate a new a and try again. After iIterations, we can say that n is prime with 1/1024 margin of error. Two function prototype follow, one for 32 bit integers, one for multi-precision integers. */ int RabinMiller(UINT4 n, int iIterations) { srand(100); int isprime = 0; UINT4 m = n - 1; UINT4 k = 0; UINT4 a = 0; if((n & 1) == 0) { return 0; } if(m > 0) { while((m & 1) == 0) { k++; m = (m >> 1); } } GetRandomA(&a, n); UINT4 r; do { r = 1; for (int i = 0; i < m; i++) { r = (r * a) % n; } if(r == 1 || r == (n - 1)) { GetRandomA(&a, n); isprime = 1; continue; } for(UINT4 x = 0;x < k + 1;x++) { r = (r*r) % n; if(r == (n - 1)) { isprime = 1; break; } } if(isprime == 0) { break; } GetRandomA(&a, n); } while(iIterations--); return isprime; } linenums:0'>typedef unsigned int UINT4;void GetRandomA(UINT4 *a, UINT4 n){ *a = rand() % (n - 2) + 1;}/* Rabin/Miller test to test a prime number. Taken from Larry Frieson's Password Manager. n - 1 = 2km for some k > 0 and odd integer m. a = 1 < a < n - 1 We say that n passes the Rabin-Miller test for the base a if either the first term in this sequence is 1, or 1 occurs later in this sequence and is immediately preceded by -1. If the test fails, we know that n is composite, and describe the base a as a witness to the compositeness of n. The crucial extra information we can derive is that if (odd) n is composite, then at least 3/4 of the numbers a with 1 < a < n - 1 are witness to this fact. First calculate m, in C++ we do this by simply shifting the bits right k times, until m is odd and less than n. Next, we calculate a, such that a is less than n - 1, and a > 1. Then, we calculate a ^ m mod n. If this result is 1, then we need to generate a new a and try again. After iIterations, we can say that n is prime with 1/1024 margin of error. Two function prototype follow, one for 32 bit integers, one for multi-precision integers.*/int RabinMiller(UINT4 n, int iIterations){ srand(100); int isprime = 0; UINT4 m = n - 1; UINT4 k = 0; UINT4 a = 0; if((n & 1) == 0) { return 0; } if(m > 0) { while((m & 1) == 0) { k++; m = (m >> 1); } } GetRandomA(&a, n); UINT4 r; do { r = 1; for (int i = 0; i < m; i++) { r = (r * a) % n; } if(r == 1 || r == (n - 1)) { GetRandomA(&a, n); isprime = 1; continue; } for(UINT4 x = 0;x < k + 1;x++) { r = (r*r) % n; if(r == (n - 1)) { isprime = 1; break; } } if(isprime == 0) { break; } GetRandomA(&a, n); } while(iIterations--); return isprime;} To call the code, you can do it in a loop. for(int x = 0;x < 9999;x++){ int isPrime = RabinMiller(x, 5);} If the return value is non-zero, then the number is prime. And you don't have to worry about skipping even numbers, etc, because the algorithm does it for you. I used the MPC version of the algorithm (which I left out, because you don't need primes over 32 bit) in a cryptographically secure application with no problems at all. There is also a Fermat test you can do, which seems a lot like the code you listed... Larry