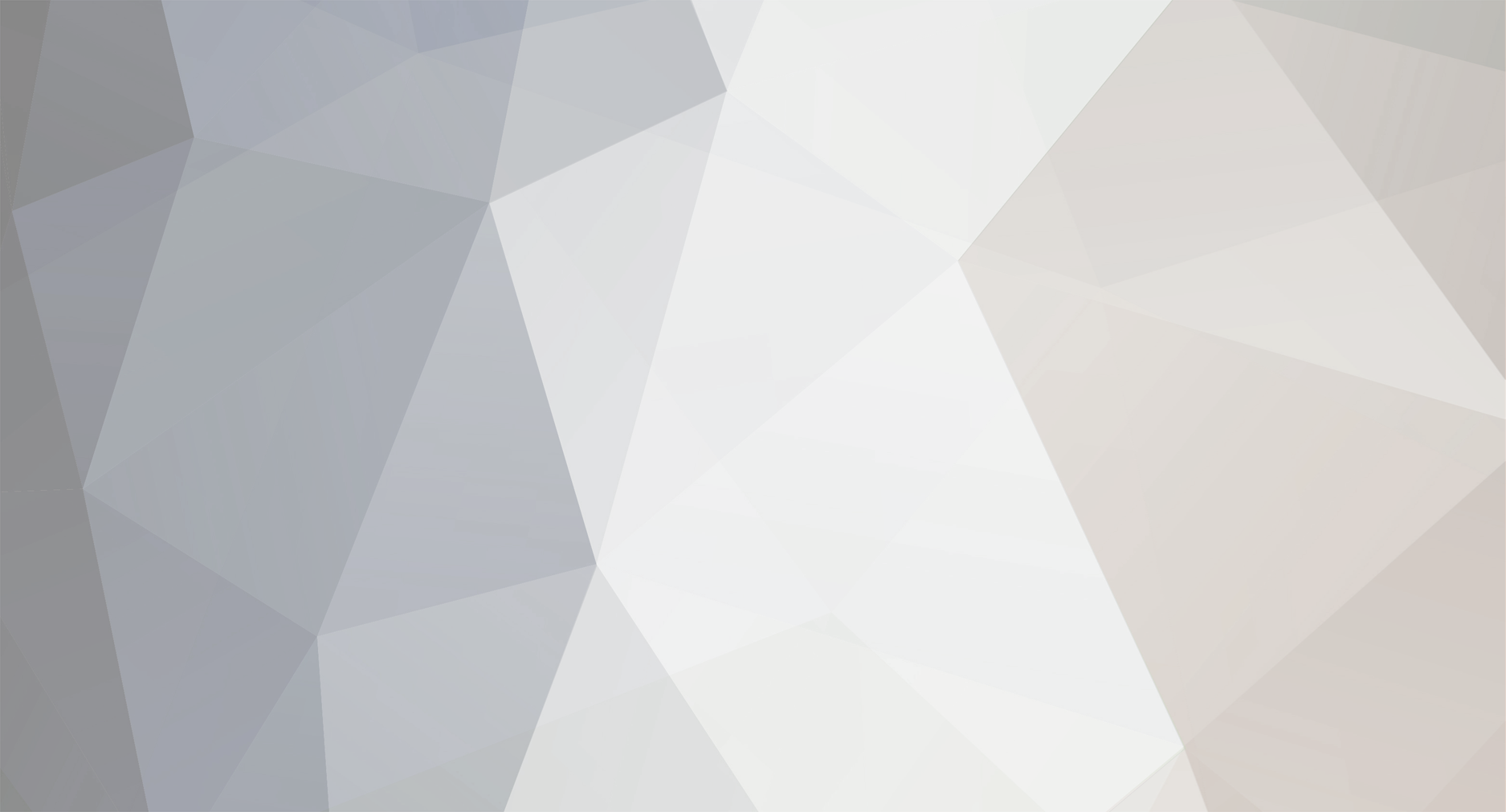
t3jem1405241533
Members-
Content Count
48 -
Joined
-
Last visited
Everything posted by t3jem1405241533
-
hehe, honestly I don't think it's even a question. I have used many free webhosts in the past and all of them would destroy my website with ads. Xisto has to be the best free web host on the web, if not the best web host period. I love how you can use just about any type of script and have databases and everything. I am very happy with Xisto and when ever I get to making websites for people it will be my first suggestion. P.S. Im not just saying this, I really mean it
-
It does depend on what you are using your computer for. If your going to be gaming, but not moving around at all you should go with a desktop, you can upgrade it easier and cheaper. Laptops are best if you have to move around alot. I use my laptop because I go back and forth between two houses about 3 times a week. Laptops are more expensive with less bells and wistles on it. If I only lived in one house I would definitly go for a desktop though, that way I could play Call of Duty 2
-
I suggest learning C++ without C because C++ is pretty much a different version of C. I learned C++ then went on to OpenGL, the book I used though was about 10 years old so I don't know of any recent books to use, but I use very little C++, just some basic C++ knowledge should suffice to be able to understand how my OpenGL tutorials work. You could probably find a few short tutorials on the internet that would help.
-
In this tutorial I am going to teach you how to texture map your polygons and implement basic keyboard control. There is alot of new material in this tutorial and I hope I have explained it enough. Before you get started you will want to name a bmp file "image.bmp" or "image" depending on your computer settings (if one doesn't work then use the other) and place it in the same folder as your project. Now to start we will be making a seperate header file where we will write our function to load a bitmap file. You should name it "bmpload.h". The code to write in it follows. first we want to link to our openGL libraries, then include our windows then openGL header files. #pragma comment(lib, "opengl32.lib")//Link to the OpenGL libraries#pragma comment(lib, "glu32.lib")#pragma comment(lib, "glaux.lib")#include <windows.h> // Header File For Windows#include <gl\gl.h> // Header File For The OpenGL32 Library#include <gl\glu.h> // Header File For The GLu32 Library#include <gl\glaux.h> // Header File For The Glaux Library this is our function that will load our bitmap images. The first parameter is storage for our texture array which will store our image. The second parameter is where you would tell the program where your file is located. and the third parameter is the column you want to store the image to in your array. bool loadbmp(UINT textureArray[], LPSTR strFileName, int ID)//(NEW) the name of our function{ first we check if a filename was entered, if it wasnt then we exit the program. if there was a filename then we load the image into a new variable for us to manipulate into a format that we want. if(!strFileName) return false;//If no file name was given then return a false value AUX_RGBImageRec *pBitMap = auxDIBImageLoad(strFileName); //Load the file into a new variable where we can then manipulate it into our texture array we check to see if data was loaded, if not we exit, otherwise we generate our texture in our texture array. if(pBitMap == NULL) exit(0);// If no data was loaded then exit the program. glGenTextures(1, &textureArray[ID]);// Generate one texture into our texture array in the slot defined glBindTexture binds our image to textured objects, more about this later. glTexParameteri() defines how the texture is filtered, the first parameter tells the program it is a 2D texture, next we define linear filtering when the texture is near the screen and same for when it is far. We will go farther into filtering in later tutorials. glTexImage2D() finalizes our image as a texture in our array. First we tell the program our image is 2D, then we set the detail, which is usually set to 0, then how many components (red, green, blue), after that we have the program find the width and height, next we set the size of the border, then what type of image it is (in this case RGB), then the type of data it will be read in (unsigned bytes), and finally where to find the data. after we finish creating the image we have to clear our variable to help prevent CPU clogging. glBindTexture(GL_TEXTURE_2D, textureArray[ID]);//This binds the texture to a texture target glTexParameteri(GL_TEXTURE_2D,GL_TEXTURE_MIN_FILTER,GL_LINEAR);//set our filter glTexParameteri(GL_TEXTURE_2D,GL_TEXTURE_MAG_FILTER,GL_LINEAR); //set our filter glTexImage2D(GL_TEXTURE_2D, 0, 3, pBitMap->sizeX, pBitMap->sizeY, 0, GL_RGB, GL_UNSIGNED_BYTE, pBitMap->data);//create our image if (pBitMap) //If pBitMap still has a value then we want to clear it. { if (pBitMap->data) { free(pBitMap->data); } free(pBitMap); } return true;} This code first checks to make sure you entered a file location, it then creates a variable that stores the data from the file and then checks to make sure the file was loaded. Next it generates the texture and sets up filters for it. The only two values for the filters are GL_NEAREST and GL_LINEAR linear makes better looking textures, but nearest uses less CPU, keep this in mind when creating more textured polygons. The next bit of code is your actual program that will load our new header file then the texture using the function we just created then it will draw the texture onto a square that we can rotate right and left using the 'r' and 'l' keys respectivly. This time we include our new header file and set our texture array along with our rotation value. Our texture array is of type unsigned integer. #include<glut.h>#include"bmpload.h"//(NEW) Load the new header file we createdGLuint textures[1];//(NEW) Storage for one texture. float rotation = 0;//(NEW) our variable for rotation in our init function we call our function to load our image for the program. void init(){ glClearColor(0,0,0,0); gluOrtho2D(-5,5,-5,5); loadbmp(textures, "image.bmp",0);// (NEW) Load our image using the function we created earlier} In our display function we must enable 2D texturing with glEnable(GL_TEXTURE_2D); and then bind the texture to texture objects with glBindTexture(). Texture objects are created when you use the function glTexCoord2f(); which set which point of the texture to draw on an object. We use it to draw our texture onto a square. void display(){ glClear(GL_COLOR_BUFFER_BIT); glEnable(GL_TEXTURE_2D);//(NEW) Enable 2D texturing glBindTexture(GL_TEXTURE_2D, textures[0]);// (NEW) used to select the texture you wanted to use glPushMatrix(); glRotatef(rotation, 0,0,1);//Rotate the square on the Z axis according to the rotation variable glBegin(GL_QUADS);// Just create a simple square glTexCoord2f(0,1);//(NEW) This is the coordinate position on the texture we want to draw at this vertex (This is the upper left corner) glVertex2f(-4,4);//This is the upper left corner of the square glTexCoord2f(1,1);//This is the upper right corner of the bitmap glVertex2f(4,4); glTexCoord2f(1,0);//This is the lower right corner of the bitmap glVertex2f(4,-4); glTexCoord2f(0,0);//The lower left corner glVertex2f(-4,-4); glEnd(); glPopMatrix(); glDisable(GL_TEXTURE_2D);//(NEW) Disable 2D texturing glFlush(); glutPostRedisplay();} our next function is to deal with keyboard events. The first parameter is used to retrieve which key was pressed, the next to are the positions of the mouse pointer, but we will learn how to use the mouse in later tutorials. We use a switch statement to reduce the amount of code needed for potentially hundreds of possible keys to be pressed. //(NEW) our keyboard functionvoid keyboard(unsigned char key, int x, int y){ switch(key)//(NEW) use a switch statement { case 'L':case'l'://(NEW) when L is pressed add to our rotation variable rotation += 20; break; case 'R':case'r'://Rotates the square to the right rotation -= 20; break; default: break;//Don't do anything if any other keys are pressed }} we add glKeyboardFunc() to our main function to tell our program where to find our keyboard function. void main(int argc, char ** argv){ glutInit(&argc, argv); glutInitWindowSize(800,600); glutInitWindowPosition(10,50); glutInitDisplayMode(GLUT_SINGLE|GLUT_RGB); glutCreateWindow("Lesson 5"); init(); glutDisplayFunc(display); glutKeyboardFunc(keyboard);//(NEW) This tells our program which function to send keyboard events to. glutMainLoop();} In the keyboard function you can use other keys than just the 'r' and 'l' keys, just use the quotes around the key you want to use. For special keys such as f3 or control I have listed what you should enter there below, you do not use quotes around the following items when using them. I have not tested all of these and some may not work, I will post all of the possible values you can use. Again, I do not know which ones work and which ones don't, but if you want to test them and let me know which ones don't work I will take them down so I'm not giving out false information. I found these values in the windows code I did not test each of these and I do not know which keys they refer to unless the name is very obvious. VK_LBUTTON VK_RBUTTON VK_CANCEL VK_MBUTTON VK_BACK VK_TAB VK_CLEAR VK_RETURN VK_SHIFT VK_CONTROL VK_MENU VK_PAUSE VK_CAPITAL VK_KANA VK_HANGEUL VK_HANGUL VK_JUNJA VK_FINAL VK_HANJA VK_KANJI VK_ESCAPE VK_CONVERT VK_NONCONVERT VK_ACCEPT VK_MODECHANGE VK_SPACE VK_PRIOR VK_NEXT VK_END VK_HOME VK_LEFT VK_UP VK_RIGHT VK_DOWN VK_SELECT VK_PRINT VK_EXECUTE VK_SNAPSHOT VK_INSERT VK_DELETE VK_HELP VK_LWIN VK_RWIN VK_APPS VK_NUMPAD0 VK_NUMPAD1 .... VK_NUMPAD9 VK_MULTIPLY VK_ADD VK_SEPARATOR VK_SUBTRACT VK_DECIMAL VK_DIVIDE VK_F1 VK_F2 ..... VK_F24 VK_NUMLOCK VK_SCROLL VK_LSHIFT VK_RSHIFT VK_LCONTROL VK_RCONTROL VK_LMENU VK_RMENU VK_ATTN VK_CRSEL VK_EXSEL VK_EREOF VK_ZOOM VK_NONAME VK_PA1 VK_OEM_CLEAR Again, this was all from the code of the windows virtual key library. If you found some of the keys that don't work, please let me know and I will double check them and get rid of them. I hope this tutorial has been very informative and if there is anything I forgot to explain more please let me know, this was a bigger tutorial than the rest and the rest are just going to get bigger.
-
No, im actually using Microsoft Visual C++ 6.0, that's why I can't make a tutorial telling people how to get a free compiler and install GLUT on it, i'v never done it. I tried to post a tutorial I recieved from somebody when I had a website with these tutorials, I quoted the whole thing, but I don't know if they will let me post it.
-
This is a tutorial that someone submitted to my programming site when it was up because I didn't know how to install glut on any other compiler than my own. I though it would be helpful to post this up for everyone here so they can use GLUT as well without having to google for hours. I'm not sure if I'm allowed, but I have quoted it and have given credit where credit is due, so if this post is allowed I hope this helps those people who couldn't program in GLUT yet.
-
Love how you put that. I'm intersted in how the universe would end too, but i would be very surprised if I ever found out. Is interesting though, maybe there is no end to the univers, but just an end to where there is matter and the matter is expanding into the vast empty limitless space until gravity pulls everything back into a singularity (refer to my previous post). Very interesting topic.
-
Programming In Glut (lesson 4) Making 3D objects
t3jem1405241533 replied to t3jem1405241533's topic in Programming
Thank you, I hope to get another one up tomarrow, it will be texturing and basic keyboard interaction. I plan to take these tutorials quite a ways, not sure how far yet, but as far as I can. -
Hello, in this tutorial we will be creating a 3D pyramid. We are building this tutorial from Lesson 3, but I took out the 2D objects and placed a 3D pyramid in there instead. The 3rd axis for drawing can be a litle confusing, but after you get the hand of it you'll do fine. Now when you are setting a 3D vertex just remember that the camera is on the positive end of the z axis. So things that have a more positive z axis value are closer than verteces with a more negative value. Well let's get started We always start by including the glut library #include<glut.h> the time variable will be used to keep track of how much time has passed. float time = 0;// set time variable to 0 Our first new function is glOrtho();, this function is much like gluOrtho2D(), but glOrtho() defines how far along the z axis you can view objects as well, this is very important when drawing 3D objects. Next we want to enable depth testing to let our program test which polygons are where and draw them in the correct order for us, to do this we use glEnable() with the argument GL_DEPTH_TEST. void init(){ glClearColor(0,0,0,0); glOrtho(-5,5,-5,5,-5,5);//(NEW) set up our viewing area glEnable(GL_DEPTH_TEST);//(NEW) Enable depth testing} In our display function we will create a 3D pyramid. To set the verteces we will use glVertex3f() instead of glVertex2f() so we can define where the point is on the z axis to add depth. in our glClear() function we add a new argument that we seperate with a "|". The new argument is GL_DEPTH_BUFFER_BIT to enable the depth buffer so our program can draw 3D objects correctly. void display(){ glClear(GL_COLOR_BUFFER_BIT|GL_DEPTH_BUFFER_BIT);//(NEW) setup our buffers glPushMatrix(); glRotatef(time,0.8,5,0);//rotate our objects glBegin(GL_TRIANGLES); //This is going to be the face facing the camera //We want this face to be red glColor3f(1,0,0); glVertex3f(-1,-1,1);//(NEW) We are now defining the vertex position on all three axis glVertex3f(0,1,0);//the top vertex glVertex3f(1,-1,1);//last vertex of this face //We do not need to enter glEnd() here because we are still drawing triangles //The back left face //This face will be green glColor3f(0,1,0); glVertex3f(-1,-1,1);//Vertex closest to us glVertex3f(0,1,0);//The top vertex glVertex3f(0,-1,-1);//The vertex farthest away //The back right face //This face will be blue glColor3f(0,0,1); glVertex3f(0,-1,-1);//The farthest vertex glVertex3f(0,1,0);//The heighest vertex glVertex3f(1,-1,1);//The closest vertex //The bottom face //This is face will be white glColor3f(1,1,1); glVertex3f(-1,-1,1);//Each corner of the base of the pyramid glVertex3f(0,-1,-1); glVertex3f(1,-1,1); glEnd();//done drawing our triangle glPopMatrix(); glFlush(); glutPostRedisplay();//This function is crucial in animation, it refreshes the screen} next we create our idle function which will update our time variable void idle(){ time += 0.1;// increase our time variable if(time > 360) time = 0;// reset time variable} next is our main function. The only difference is in the gluInitDisplayMode() function, we added GLUT_DEPTH as an argument to tell the program to use the depth buffer. void main(int argc, char ** argv){ glutInit(&argc, argv); glutInitWindowSize(800,600); glutInitWindowPosition(10,50); glutInitDisplayMode(GLUT_SINGLE|GLUT_RGB|GLUT_DEPTH);//tell the program we are running the depth buffer glutCreateWindow("Lesson 4"); init(); glutDisplayFunc(display); glutIdleFunc(idle);// This function calls our idle function to update our variables glutMainLoop();} depth testing is very important when creating 3D objects, you must enable, tell the program to clear it, and you must tell it that you are using the depth buffer. If you don't do all of these than your program will draw everything in the wrong order and it will look very confusing. I hope you enjoyed yet another GLUT tutorial. The next tutorial will include texturing and keyboard interaction. Edited on December 28 2006 to make more readable.
-
Sandboxie: Excellent Browser Protection Freeware
t3jem1405241533 replied to miCRoSCoPiC^eaRthLinG's topic in Software
Oh ok, well, I guess i'm just too much of a panzy to actually thoroughly test that theory, but maybe when I get tired of how my computer is running right now i'll check it out. Thanks for this, this sounds very cool -
Sandboxie: Excellent Browser Protection Freeware
t3jem1405241533 replied to miCRoSCoPiC^eaRthLinG's topic in Software
Ok, well I just downloaded it and installed it. I ran IE under it and went to google to find a website I knew would have something on it. Well I get on the website, but my antivirus program caught a virus, so I don't know if this means that sandboxie didn't work or not, but I know I wasn't running my Antivirus program under sandboxie. -
Half-Life 2 all the way, my comp may not run it very good, but the story line is unmatched by any game I have ever seen. The gameplay is absolutly amazing and the models are all very realistic. The AI is unbelievable too.
-
In this tutorial I am going to talk about how to get animation in your program. I will be working directly off of Lesson 2 and will now take out the notes left behind from Lesson 1, but I will leave the notes from Lesson 2. #include<glut.h>//include our glut library First we are going to initialize a variable called "time" which will record how much time has passed so we can use it to determine how to animate the objects. Then we have our init function that initializes some stuff in GLUT float time = 0;//(NEW) variable to record the how much time has passedvoid init(){ glClearColor(0,0,0,0); gluOrtho2D(-5,5,-5,5);//Setup our viewing area} Next is our display function, this time we will have three objects with their colors, but there will be five new functions introduced, all having to do with transformations. glPushMatrix(); creates a new matrix layer where you can do transformations, you use this function combined with glPopMatrix(); to keep from setting transformations that will effect your entire application. glPopMatrix(); ends transformations that you have made to the matrix you were using. glRotatef(); rotates objects around the origin. The first argument is the amount of degrees to rotate, the other three tell the program what axis to rotate it on. If you use (90,1,0,0); it will rotate 90 degrees on the x axis. glScalef(); scales objects around the origin. The values you enter into the arguments multiply each vertex, so (2,2,2) will multiply each vertex argument by 2 so the vertex (3,2) will be moved to (6,4) glTranslatef(); "slides" the verteces around on the axii. With the arguments (3,2,0); will move all verteces 3 units to the right, 2 up, and 0 back. The rest of the code is the same. void display(){ glClear(GL_COLOR_BUFFER_BIT); glColor3f(1,0,0);//Set color to red glPushMatrix();//(NEW) Create a new matrix glRotatef(time,0,0,1);//(NEW) Rotate the triangle on z axis based on how much time has passed glBegin(GL_TRIANGLES);//Start drawing triangles glVertex2f(3,-4);//1st vertex glVertex2f(3.5,-3);//2nd vertex glVertex2f(4,-4);//last vertex glEnd();//stop drawing glPopMatrix();//(NEW) stops current transformations to the matrix glColor3f(0,1,0);//set the color to green glPushMatrix();//(NEW) create new matrix glTranslatef(time/50,0,0);//(NEW) slide the object on the x axis based on time glBegin(GL_QUADS);//Start drawing quadrilaterals glVertex2f(-4,-4);//1st vertex glVertex2f(-4,-2);//2nd vertex glColor3f(0,0,1);//Change color to blue halfway through glVertex2f(-2,-2);//3rd vertex glVertex2f(-2,-4);//last vertex glEnd();//stop drawing glPopMatrix();//(NEW) stop all current transformations glPushMatrix();//(NEW) create a new matrix glScalef(time/200,time/200,time/200);//(NEW) scale the object on all axiis based on time glColor3f(1,0,0);//First vertex is red glBegin(GL_POLYGON);//start drawing a polygon glVertex2f(-2,2);//first vertex glColor3f(0,1,0);//Second vertex is green glVertex2f(-1,3);//second vertex glColor3f(0,0,1);//Third vertex is blue glVertex2f(0,2);//third vertex glColor3f(1,0,1);//Third vertex is purple glVertex2f(-0.5,0);//fourth vertex glColor3f(1,1,0);//last vertex is yello glVertex2f(-1.5,0);//last vertex glEnd();//tell the program we are done drawing our polygon glPopMatrix();//(NEW) End transformations currently used glFlush(); glutPostRedisplay();//refresh the screen} Next we have a new function called idle. This function will be called to update our time variable. With the idle function set in GLUT you can keep your display code clean and have all the background work in an easy to find function. We will reset the time variable to 0 every now and then so the objects dont get too big and they come back after they slide away. void idle(){ time += 0.1;//(NEW) increase the time variable if(time > 360) time = 0;//(NEW) reset the time variable} there is only one new call in the main function and that is glutIdleFunc(); this is used to call our idle function that we created just above. void main(int argc, char ** argv){ glutInit(&argc, argv); glutInitWindowSize(800,600); glutInitWindowPosition(10,50); glutInitDisplayMode(GLUT_SINGLE|GLUT_RGB); glutCreateWindow("Lesson 3"); init(); glutDisplayFunc(display); glutIdleFunc(idle);//(NEW) calls our idle function glutMainLoop();} The function "glRotatef()" is used to rotate objects around the origin. The reason the triangle spins around the screen is because it was not placed at the origin of the screen, but at the edge; therefore, making the triangle run around the screen. The function "glTranslatef()" moves objects to a certain spot. It just adds the translation arguments to all the verteces that apply. It does not matter where the object was originally placed The function "glScalef()" scales objects according to the arguments provided. all verteces are multiplied by the arguments. If you put 2 in the x argument of glScalef() then all applicable vertices will be multiplied by 2 on the x axis. This will have an odd effect on objects not placed at the origin as seen in this tutorial. I hope you now know how to run animations, The next tutorial is on it's way Editted on December 20th 2006 to make it easier to read
-
Boast Your Computers Specs. Feel free to brag
t3jem1405241533 replied to chronogamer28's topic in Hardware Workshop
I have the dell xps m140, got it for christmas last year.CPU: Intel Pentium M processor 1.73 GHz 795 MHzRAM: 504 MB (exactly what my comp tells me, shuld be 512)Video card: Intel 915GM/GMS, 910GML Express Chipset 128MBHarddrive: 50GBIt also has built in wireless cardIt can't play counterstrike: source that great, but I'm thinking of upgrading to 1GB of ram after christmas maybe. -
This is a question I have been pondering for a little while. How would time warp if one was to be moving near the speed that light travels, or one was posititoned near a black hole. I have come up with a small theory for both; however, they are most likely incorrect . I would appreciate any feedback on my thoughts. Gravity warping time Some scientists believe the time slows down the closer you are to the event horizon of a black hole; however, I don't believe the time can be warped by a black hole, but rather it gives the illusion that time is being warped. Let's say the observer A is watching Observer B moving nearer to the event horizon of a black hole (assuming of course the gravity does not wrench either observers into a billion pieces). Both observers are watching each other, now according to theory Observer B will start to move slower and may eventually freeze in place from the viewpoint of observer A. While observer B will look at observer A and see him moving insanely fast. I believe what is actually happening here is that the gravitational force of the black hole is not affecting space, but rather the light that is being bounced off of both observers (visual perception of an object is only possible when light hits your eye. to see an object light must first bounce off of the object then hit your eye, kinda like sonar only with colors and stuff). Well, the light bouncing off of observer B towards observer A is being slowed down by the black hole's gravitational pull and therfore slowing down how fast observer B seems to be moving. When the light can no longer escape it will appear that observer B has in fact been frozen; however, over time the remaining light particles that can still escape, but haven't will eventually get free and you will no longer be able to see observer B. Observer B sees observer A moving much faster because the light is coming at him much faster. Observer B will be able to see observer A moving faster and faster until his eyes cannot see the intense movement of observer A. Those are my thought on gravitational time warping Time warping by speed My thoughts on how time is warped by moving faster are closely related to my ideas about how gravity warps time. The only difference is that instead of having gravity bring the light faster to you, or slower away from you, you are actually moving towards the light or away from the light depending on which way you look. So if you look behind you while moving close to the speed of light, everthing behind you will look like it has slowed to a very slow pace; likewise, if you look in front of you everything will seem to have sped up.
-
This is the second lesson in my series of tutorials on how to use GLUT to create graphics. In this tutorial I am going to be teaching you how to create different types of polygons. I am going to be adding on to last tutorial's code and will leave the notes in to help you remember what all the function are. I will also be noting the new functions that we will be using and how to use them. #include<glut.h>//Include the GLUT functions This time we are going to not only set the background color, but set the area that we are viewing as well. gluOrtho2D(); sets left, right, bottom, and top borders of the viewing area, in this case we are viewing 5 units to the left, right, down, and up. void init(){ glClearColor(0,0,0,0);//Define our background color gluOrtho2D(-5,5,-5,5);//(NEW) Define our viewing area} We are now going to draw our polygons using the function glBegin and glEnd. All the verteces defined between these two functions will be drawn using the rules given in the glBegin function. The rules that can be used can be found at the end of this tutorial. glColor3f(); changes the colors of objects, any verteces following this function will be changed to the color defined. The colors are defined just as they are in glClearColor(); glVertex2f(); defines a 2D vertex as an (x, y) vertex. We will use this function to define our polygons. In the next function we will create three polygons, a triangle, square, and pentagon. Each polygon will have it's own color. void display(){ glClear(GL_COLOR_BUFFER_BIT);//Clear the screen glColor3f(1,0,0);//Change the object color to red glBegin(GL_TRIANGLES);//Start drawing a triangle glVertex2f(3,-4);//draw our first coordinate glVertex2f(3.5,-3);//Our second coordinate glVertex2f(4,-4);//Our last coordinate glEnd();//Stop drawing triangles glColor3f(0,1,0);//Change the object colors to green glBegin(GL_QUADS);//Start drawing quads glVertex2f(-4,-4);//first coordinate glVertex2f(-4,-2);//second coordinate glColor3f(0,0,1);//Change the color to blue halfway through to create a neat color effect glVertex2f(-2,-2);//third coordinate (now blue) glVertex2f(-2,-4);//last coordinate glEnd();//Stop drawing quads glColor3f(1,0,0);//Change color to red glBegin(GL_POLYGON);//Start drawing a polygon glVertex2f(-2,2);//first vertex glColor3f(0,1,0);//Change color to green glVertex2f(-1,3);//second vertex glColor3f(0,0,1);//Change color to blue glVertex2f(0,2);//third vertex glColor3f(1,0,1);//Change color to purple glVertex2f(-0.5,0);//fourth vertex glColor3f(1,1,0);//Change color to yellow glVertex2f(-1.5,0);//last vertex glEnd();//Stop drawing our polygon glFlush();//Draw everything to the screen glutPostRedisplay();//Start drawing again} Now we finish the code off with our main function again. void main(int argc, char ** argv){ glutInit(&argc, argv);//Initialize GLUT glutInitWindowSize(800,600);//define the window size glutInitWindowPosition(10,50);//Position the window glutInitDisplayMode(GLUT_SINGLE|GLUT_RGB);//Define the drawing mode glutCreateWindow("Lesson 2");//Create our window init();//initialize our variables glutDisplayFunc(display);//tell Glut what our display function is glutMainLoop();//Keep the program running} As you can see there are many different modes for the function "glBegin()". The modes that can be used and what they are used for are listed below. GL_POINTS This is used to just draw points, instead of polygons or lines. You only need to define one vertex to see a point GL_LINES -- This mode lets you draw lines. You need to define 2 verteces at a time. GL_LINE_LOOP -- This mode creates a loop of lines. You define the verteces much like GL_POLYGON and when you finish it will link the last and first two verteces together with a line. GL_LINE_STRIP -- This mode does the same as GL_LINE_LOOP except it does not connect the first and second lines GL_TRIANGLES -- This mode creates triangles and takes 3 verteces at a time GL_TRIANGLE_STRIP -- This mode creates a strip of triangles. The second triangle uses 2 verteces of the first, and you just define the last vertex of the next triangle GL_TRIANGLE_FAN -- This function creates a fan of triangles. All the triangles use one same point and 1 point from the triangle before them, you define the last vertex GL_QUADS -- This creates quadrilaterals and take 4 verteces at a time GL_QUAD_STRIP -- This mode creates a strip of quadrilaterals. After the first quad, it uses 2 verteces from the preveious quad then you define the other 2 GL_POLYGON -- This mode lets you create a polygon will n verteces I hope you enjoyed the tutorial and found it helpful. Many more are on their way. Again go ahead and fiddle with the settings and modes, you can learn alot more from playing with the code then just reading it. Editted on December 18, 2006 to make it more readable
-
I cannot even guess what the universe is expanding into, but we know the universe is expanding by observing the red shift in stars and galaxys millions of light years away. I believe in the big bang theory as the way the universe was created; however, I believe that the big bang resulted from the crunch of a previous univers. When the mass of all the stars, planets, black holes, etc.. eventually overcame the kinetic force that was driving them away from each other, they began attracting each other and eventually they all collided creating phenominal energy (just think of Einstiens equation energy = mass * speed of light squared, with all the mass in our universe 8|). The energy then created the big band and started the sequence again. After things started to cool down, closer particles dust and rocks would collect creating stars and planets as we know them today. I believe that the universe will expand until gravity overtakes it again and it collapses on itself creating yet another big bang. Its just God's way of renewing the universe every now and then . Well, thats my view on it, hope I enlightened somebody.
-
The Mentos And Diet Coke Reaction
t3jem1405241533 replied to Darkwolf11235's topic in Science and Technology
idk if the Potassium being "Potassium permaganate" would make a difference, but when you mix potassium and water, it does create an explosion, maybe adding the other stuff destablized the potassium a little, but not enough to cause a large explosion. Anyways, there was something on mythbusters a few months back with mentos and soda and they actually came up with the whole formula and exactly why it would cause such a violent reaction. Unfortunatly I can niether remember what it was or find it on their site. But their site does have a link to a video of a chain reaction of like 1000 2 liters with mentos you might be interested in. -
Hello, I'm starting a series on how to program in OpenGL using the OpenGL Utility Toolkit, a.k.a. GLUT. I chose GLUT because it is quick and easy to write, and very easy to learn. In this tutorial I am going to teach you how to create a basic window which we will build off of in later tutorials. Throughout the tutorial I will leave notes to let you know what each command does, and how you can modify it to fit your needs. Everything in the code section can be copied and pasted into your compiler and it should compile proporly, if it does not, please let me know, and I may be able to help you. Note: It is recommended that you have at least some basic knowledge of C++ before learning OpenGL; though, it is not required, it will help with understanding the tutorials. Note: I have tested this code myself in Microsoft Visual C++ 6.0; however, it is possible that it may not work on other compilers. If you do find a mistake or you have questions I would be happy to try and answer them. First we will have to include our header file that contains all of our OpenGL commands #include<glut.h>//This header file contains all the commands for the OpenGL Utility Toolkit The init() function will be called after the window is created to initialize the settings for the OpenGL window. Right now all we will be doing is set the background color to black. The glClearColor() function defines what the background color will be, the values for each one range from 0 to 1 in Red, Green, Blue order. If you put (1,0,0,0) in it will be solid red, and (1,1,1,0) will be white. //function to initialize GLUT settingsvoid init(){ glClearColor(0,0,0,0);//(NEW) we define the background color here} The function is the main function in GLUT. We will use this function do draw all of our object; however, in this tutorial there are no objects to draw, but you still need to have some functions in there for the window to refresh. The glClear() function clears the screen to the default background color (in this case black). Without it your window will be clear and it will not refresh. The glFlush() function draws all of the objects we defined on to the screen. In this tutorial we have no objects so it doesn't do anything. glutPostRedisplay() tells the program to start drawing the screen again. This is a very important command for animation, without it your objects will not move. //The function where all the drawing occursvoid display(){ glClear(GL_COLOR_BUFFER_BIT);//Clear the screen glFlush();//Draw everything to the screen glutPostRedisplay();//Tell the program to refresh} The main function is the backbone of your program. This is where it begins and where you define your window, initialize GLUT, and define what your vital function are. glutInit() initializes glut and tells the program that we are using it glutInitWindowSize() defines the width and height of the window in pixels glutInitWindowPosition() defines where the window will be placed, in pixels, from the upper right corner of the screen glutInitDisplayMode() initializes what modes we are using, in this program we are only using a single buffer and red,green, blue coloring. glutCreateWindow() obviously creates a window. The one parameter for it is the name that will appear in the title bar. After we create our window we call our init function to set some GLUT variables (in this case the background color), then we tell GLUT where to find our display function glutDisplayFunc() tells glut which function does all the drawing glutMainLoop() tells GLUT that we are not done with our program and to keep going through the program void main(int argc, char ** argv){ glutInit(&argc, argv);//initialize GLUT. glutInitWindowSize(800,600);//define the window size as 800 pixels wide and 600 pixels high glutInitWindowPosition(10,50);//Set the window position at (10,50) glutInitDisplayMode(GLUT_SINGLE|GLUT_RGB);//Set the initial display mode glutCreateWindow("Lesson 1");//Create our window init();//call init() glutDisplayFunc(display);//tell GLUT what our display function is glutMainLoop();//Tell the program we are not done yet} I hope you learned something from the tutorial. Go ahead and mess around with settings and figure out how to work them. I will be adding new tutorials soon, but right now im just too tired, possibly tomarrow though. Edited Dec 11 2006 by T3jem to make it more readable (thanks twitch)