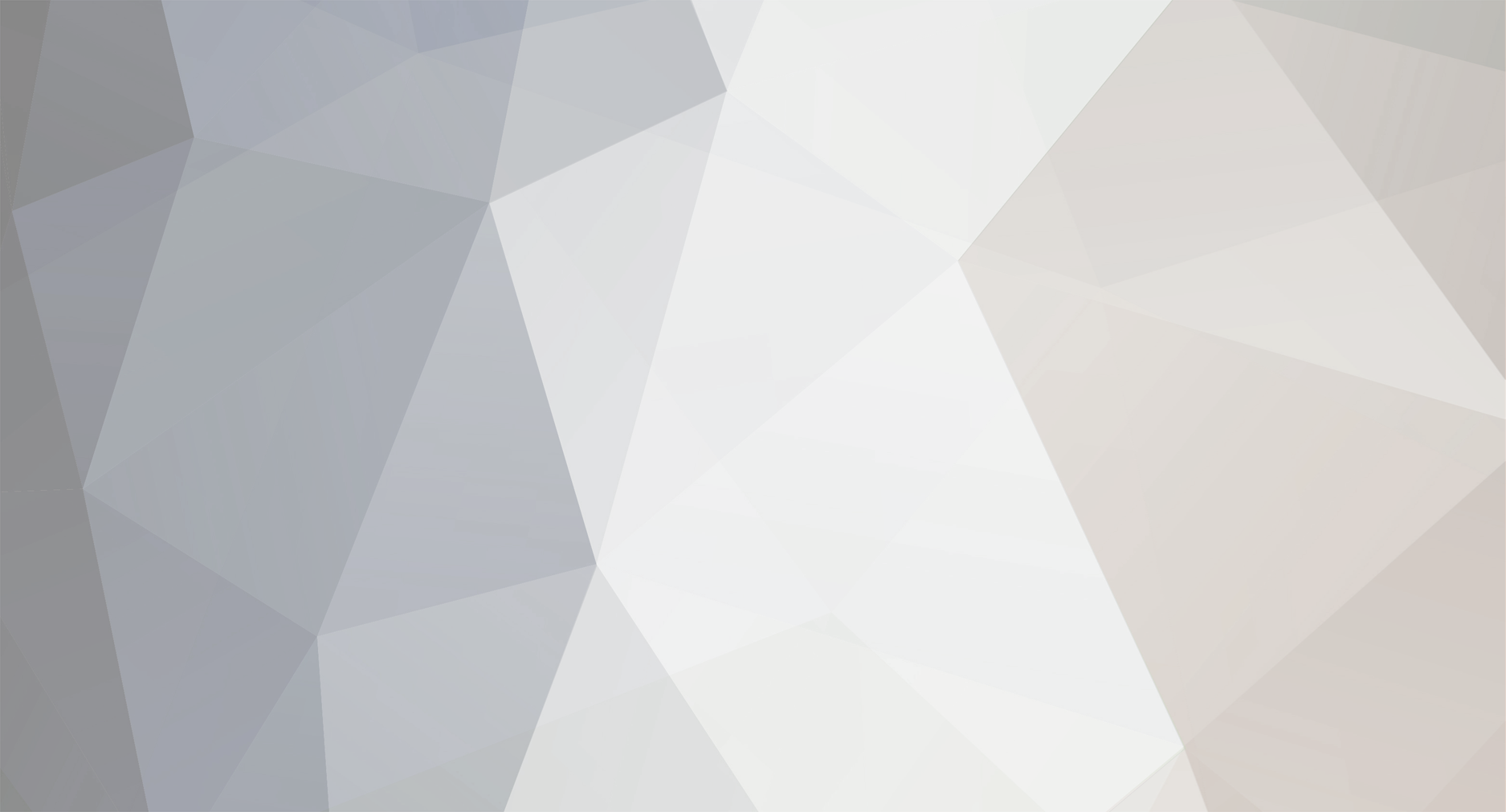
oval1405241520
Members-
Content Count
3 -
Joined
-
Last visited
About oval1405241520
-
Rank
Newbie
Contact Methods
-
Website URL
http://
Profile Information
-
Location
Texas
-
In this lesson I will cover how to use basic math operations, well addition really but you can also use subtraction[-], division[/] and multiplication[*]. I would also like to note that when doing division, if variabes of int type are used you will not get any remainders (i.e. n.345984). To be able to work with real numbers, numbers with decimals, you can use float. Below is a way to declare a float and assign it a value: float floatNumber; float Number = 28.47f; Aside from generating random numbers and basic math operations, we will also incorporate an if statement and convert the user output to an integer. If you recall from the previous lesson, when a user enters an input, it is in string format. In order to perform math calculations correctly, we need to convert it to a numeric value (such as an integer in this instance). Let's take a look at the code below. Be sure to look at the comments if something is unclear. using System;namespace lessonThree{ /// <summary> /// In this lesson we will cover some basic math /// operations, look at an if statement and a few other /// useful things that should be understood when /// programming in C# /// </summary> class thirdLesson { /// <summary> /// Ask an age. /// If under 18 -> Exit Program /// Give lucky number for the day /// Predict when person will get rich. /// </summary> [STAThread] static void Main(string[] args) { int age; //Random object createRand Random createRand = new Random(); int luckyNumber; Console.Write("Enter your age: "); //grab the input from user and convert it to //an integer, hence the 'Convert.ToInt32(); age = Convert.ToInt32(Console.ReadLine()); //skip line Console.WriteLine(); if (age > 17) { //Random generated numbers range from 0 to 1, //therefore we multiply by ten (10) below//The .NextDouble() method helps retrieve the value luckyNumber = (int)(createRand.NextDouble()*10); Console.WriteLine("Your Lucky Number is: {0}", luckyNumber); //Here you use the value of luckyNumber and add it to his age Console.WriteLine("You are {0} years old and will be rich at age: {1}", age, age+luckyNumber); //As you can see the zero in braces {0} holds the variable age //and the one in braces {1} holds the sum of the variables "age and luckyNumber" age+luckyNumber }//If the age is not 18 or above we conclude by saying Console.WriteLine("Thanks and Have a Great Day"); Console.WriteLine(); Console.WriteLine("Press [ENTER] to Exit"); Console.ReadLine(); }//end Main() }//end class}//end namespace As always, if you are testing this console application on Visual Studio or developing your own, stepping through the application with F11 is very useful.
-
In the previous lesson we demonstrated did a basic input output application which asked your your name and replied with a general greeting using the name you entered. In this lesson we are going to learn about a conditional statement called the switch statement. Usually if and if else statements are taught before switch statements. Although these are very useful, I find the switch to be easier and more fun to use. The program written below is very similar to the previous program where the user is asked to enter their name, however now the program will look for four distinct string values and ti will generate output according to the string entered. Here are the things to observe when reading and replicating this program. 1.) 2 string variables are declared (greeting and fullName) 2.) A value is assigned using our Console.ReadLine() method. (Point where we insert the value.) 3.) the value of the variable greeting depends on the value entered for the variable fullName 4.) The program is case sensitive. Basically this is because different case letters have different values. So a lower case t will not evaluate the same as an upper case T. So ulitmately what does this mean. Well let's if you entered george bush as opposed to George Bush, you would get the default greeting because the program would not recognize your lower case entry of george bush as George Bush. If it does not make sense, run the program and change the case. You will see what I mean. 5.) Lastly, the default option is included in the case statement for the instances where neither of the output meets any of the criteria of the case statement. With that said let's look at the code, replicate it and modify it to your liking. using System;namespace ConsoleApplication{ /// <summary> /// Testing out the case Statement /// </summary> class switchStatement { /// <summary> /// The main entry point for the application. /// </summary> [STAThread] static void Main(string[] args) { string fullName, greeting; Console.Write("Please enter your name: "); fullName = Console.ReadLine(); switch (fullName) { case "YOUR NAME HERE": greeting = "You are the best!!"; break; case "Bill Gates": greeting = "You are super wealthy!"; break; case "George Bush": greeting = "hmmm????"; break; case "Kenneth Lay": greeting = "How is Court Going?"; break; default: greeting = "I'm sorry I did not recognize you!"; break; }//end switch statement Console.WriteLine(); Console.WriteLine(greeting); Console.WriteLine(); Console.WriteLine("Press [ENTER] to Exit"); Console.ReadLine(); }//end Main }//end class "switchStatement}//end namespace As I might have mentioned on my previous post I am using Visual Studio. I believe the Express version is out on the web for free. However you might need to install NetFramework before you proceed to install VS. Also, just like the previous program, an adobe file will be attached with the source code. variables and comments are all color coded. oval
-
This tutorial will help get you started with C# Sharp. These tutorials will assume that you are using the Visual Studio environment. If you are just curious to test out some code, then you can copy and paste this code into your CS file, however, before doing so, make sure to name your project the same as the namespace on the code. But since this first program is fairly easy, it will not hurt to type it out. It is fairly easy and short. Also, this program is highly commented so it should be easy to follow along. Comments will have 2 forward slashes before them '//' trailing with the comment. Well before looking at the code, I just want to summarize what the following lines of code will do. Console.WriteLine(); <----- Writes a string of characters on the console in it's own line. So writing Console.WriteLine("Hello World"); would result in Hello World displaying on your console and have the cursor move to the next line down. Console.Write("Hello World"); would also display Hello World however the cursor would be next to the word and not the line below. Console.ReadLine(); <------ This nifty method will allow you to take in user input. Whether the user input is numeric or alphabetic, it will take the input as a string or text. So you cannot use it in any mathematical operations right away. That is if the user was to enter 5 and you want to add 4 to that 5, you could not, right off the bat anyway. However we will go into that later. Okay, now observer the code below: using System; ==================== BEGIN C# CODE ===================================== namespace testProg { ///<summary> /// Test Programming ///</summary> class helloProg { [STAThread] static void Main(string[] args) { //Declare a String variable. Name it userName string userName; Console.Write("Console Asks - Please Enter your Name: "); //Place the user input into the variable userName with //Console.ReadLine() userName = Console.ReadLine(); //Skip a line for nicer formatting Console.WriteLine(""); //On the next line, display the input. //{0} is where the variable value will go. It //is followed by a comma and variable name userName Console.WriteLine("Hello {0}!", userName); Console.WriteLine("Press Enter to Continue"); //The Console.Readline will pause the program until you //press [ENTER]. Then it will exit. Console.ReadLine(); } //end main } //end class } //end namespace=========================== END C# CODE ================================ Okay aside from the information I provided before the code, I think the comments explain pretty much what is going on within this program. However, I want to further elaborate how we placed the user input into the variable userName. By setting first we declared userName as a string (i.e. string userName;) Later in the program, we said userName is equal to what the user enters, userName = Console.ReadLine(); This is what did the trick. now we just output user name in one of our Console.WriteLine's and Voila. Modify the variables, add lines and variables and get acquainted with it yourself. I'll be back soon to provide another lesson. By the way, I hope I am able to upload my attachment. The code on this one looks kinda sloppy. It's in .pdf format.