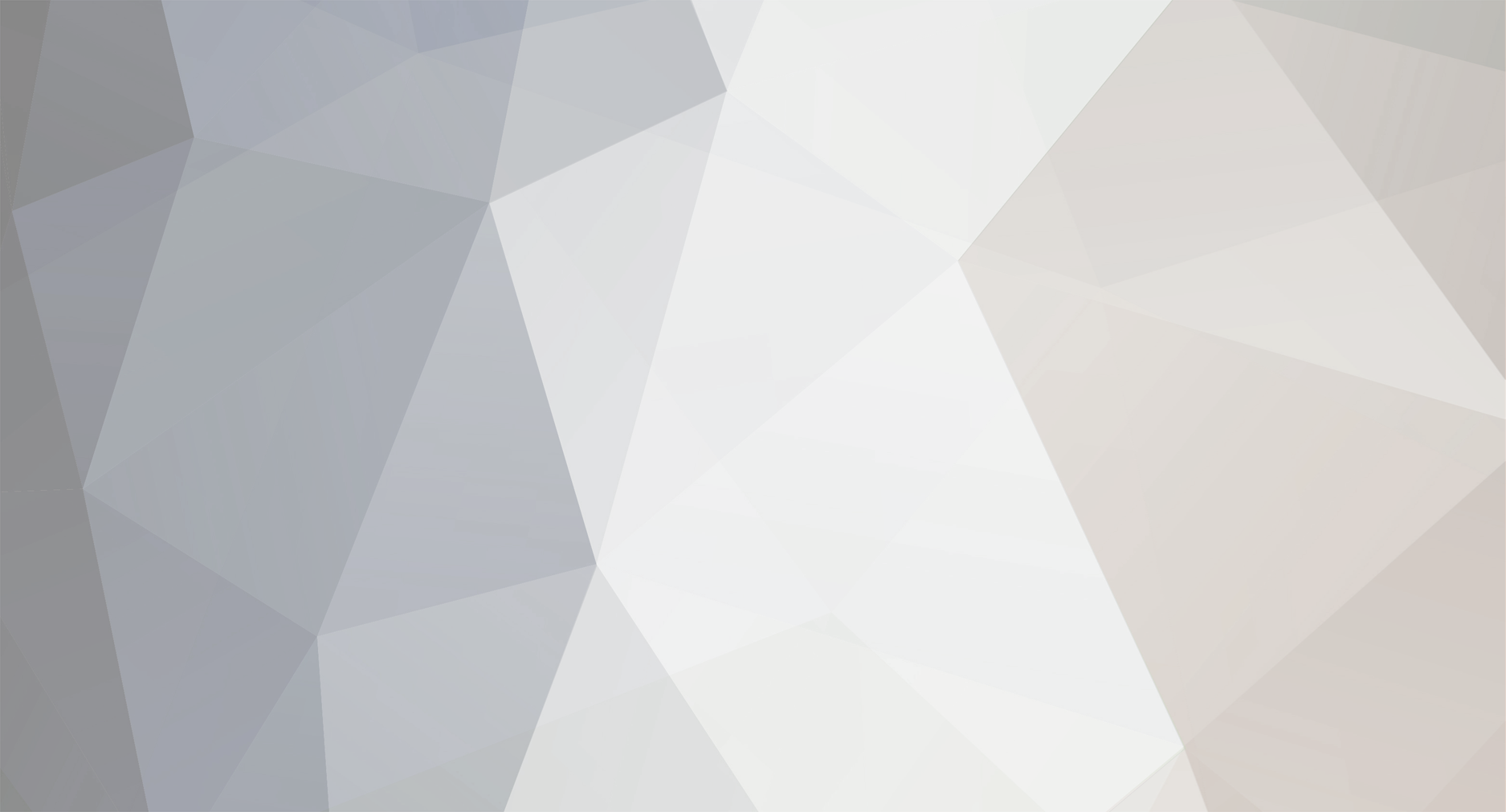
FearfullyMade
Members-
Content Count
44 -
Joined
-
Last visited
About FearfullyMade
-
Rank
Newbie [Level 3]
-
FearfullyMade started following Can't Log In, Languages Worth Learning What languages should I learn?, Suse And Dual Monitors it isnt working and and 3 others
-
The purpose of this tutorial is to explain how to create a Java applet. A Java applet is simply a Java program that runs on a webpage. This tutorial assumes that you have a basic understanding of Java. If you don't, I would suggest reading a tutorial on Java first. In this tutorial I will be using Java 1.5 (or 5.0). If you don't have it you can download it from Sun. The classes and methods used in this tutorial may work for other versions of Java, but I don't know for sure. During this tutorial I would suggest opening up Java's online documentation, found here, so that you can look at the classes and methods I use and see what they do for yourself. One of the best ways to learn about Java is the explore the documentation. The JApplet Class Like every Java program, an applet requires you to have at least one class. Luckily for us, the guys at Sun made creating applets pretty easy by designing a simple framework class for us to use: JApplet. This class gives all the functionality that you need. All you need to do is create a class that extends JApplet and you are well on your way. Be sure to import the package that contains JApplet, javax.swing. import javax.swing;public class Bouncy extends JApplet{ //the code for your applet goes here} The JApplet class has many, many different methods, but there are only a few main ones that are really important. These methods are called by Java when certain events occur. Here they are along with an explanation: init() - This method is automatically called when a user first accesses the page that contains your applet. You can think of it the applet's version of the main() method. Here is where you put all of your one-time initialization code, hence the name. start() - While your applet is only initialized once, it can be started and stopped many times. When the user leaves your applet's page the applet stays in memory. The stop() method is called (see below) but nothing else happens. Normally your applet will not actually be shut down until the browser is completely closed. The start() method is called both the first time your applet is loaded, right after init(), and whenever the user returns to your page. You can use this method to start your applet's action or do any initialization that needs to happen every time the users goes to the page. stop() - This is called whenever the user leaves the applet's page. You don't want to uninitialize everything in this method because they could return to your applet. Use this method to stop any actions that your applet may be doing. destroy() - This is the last method that Java calls. It is called just before the applet is shut down. Here is where you need to uninitialize and close anything you have running. You are not required to included all of these in your class, only include the ones you need. You will need to have at least one of them, otherwise your applet will never do anything! The Timer class The timer class doesn't really have anything to do with applets, but since I need it in my example applet, I'll take a moment to explain the basics of how it works. If you already know how it works or just don't care feel free to skip this section. The Timer class allows you to schedule tasks to occur. There are several different methods used to schedule tasks, but the one we're interested in right now is the one that repeats the task every x milliseconds, schedule(TimerTask timer, long delay, long period). The delay is how long to wait before beginning and the period is the frequency that the task is carried out. TimerTask is a class that describes what to do when your task needs to be done. Whenever the Timer class sees that it is time to do a task it calls the run() method of the corresponding TimerTask class. Since the only important part of the TimerTask class is the run() method you can define the class right when you create an object of it, like I do. So, here is the code that would create a timer and schedule a task to begin immediately and to go off every 50 milliseconds: Timer timer= new java.util.Timer();TimerTask task= new TimerTask() { public void run() { /* do stuff in here*/ } };timer.schedule( task , 0, 50); When you are done with the task call its cancel() method and when you are done with the entire timer call its cancel() method. task.cancel();timer.cancel(); Putting it together Now that you understand how applets work it is time to make one. The example below is just a simple applet where a ball bounces around. It is pretty straightforward. You can see it in action here. I use the four methods I talked about earlier along with one of my own, move(), which moves the ball. I used the timer code I showed earlier, split up and put into the correct methods. import javax.swing.*;import java.util.*;import java.awt.*;public class Bouncy extends JApplet{ //position of the ball int x,y; //direction the ball is moving int deltaX, deltaY; final int RADIUS = 20; //size of ball final int SPEED = 10; //speed the ball is moving //used to update the balls position java.util.Timer timer; TimerTask task; public void init() { //these are the things that only need to be started once //set balls position x=200; y=150; //set the balls direction deltaX=2*SPEED; deltaY=SPEED; //create the timer timer= new java.util.Timer(); } public void start() { //the timer needs to be started everytime the page is loaded //the run() method of this class will be called by the timer at the specified interval task= new TimerTask() { public void run() { move(); } }; timer.schedule( task , 0, 50); //start timer } public void stop() { //stop the task when the user leaves the page task.cancel(); timer.purge(); } public void destroy() { //get rid of the entire timer when we are done timer.cancel(); } public void move() { ///move ball x+= deltaX; y+= deltaY; //cange direction if it hits the wall if ((x-RADIUS) <= 0) deltaX*= -1; else if ((x+RADIUS) >= getWidth()) deltaX*= -1; if ((y-RADIUS) <= 0) deltaY*= -1; else if ((y+RADIUS) >= getHeight()) deltaY*= -1; //draw Graphics g= getGraphics(); //clear background g.setColor(Color.GREEN); g.fillRect(0,0,getWidth(),getHeight()); //draw ball g.setColor(Color.RED); g.fillOval(x-RADIUS, y-RADIUS, RADIUS*2, RADIUS*2); }} Put it on the web Now that you have the applet, how do you stick it on a web page? The HTML is really pretty easy. There is an applet tag that lets you specify the .class file your applet is in and the size of your applet. Just insert that into a web page and you're ready to go. Don't forget to upload your .class file along with the web page. <html> <applet code="Bouncy.class" width=300 height=300></html> I hope you found this tutorial useful. Please let me know if you have any comments or suggestions. Also, if you have any questions about Java applets that this tutorial didn't cover let me.
-
Rebbeca, I have a question about signal strength. If I lived in a house by myself then I wouldn't worry too much about it. However I leave in an apartment complex. I was wondering how far the signal would normally go. Would it stop at my building (it isn't very large, only 4 2-bedroom apartments) or would it go even farther? I've heard of people being able to drive down streets and pick up signals from nearby houses.
-
I'm not an expert on this, but here is my understanding of what you would need to create a language.Like vizskywalker said, all you need to create your own language is make up the rules and syntax and then write a compiler that will take the code and turn it into something that can be run by the computer. For example, a C++ compiler takes your code and breaks it into assembly, and eventually bytecode. Your compiler would have to something similar. I guess you could have your compiler translate your code to C++ and then use a C++ compiler to do the rest. That might work and it could be a little easier since you wouldn't have to work with assembly (I think). As far as the rules and syntax goes, you can do whatever you want, just remember the more complicated you make it, the harder it will be to write your compiler.I always thought it would be cool to create my own language, but I don't know if I'll ever get around to it. Good luck, and I hope you succeed.
-
What Language Is Best For Game Programming? I need some help
FearfullyMade replied to bigd1's topic in Programming
I would suggest giving Java a try. It is very similar to C++ as far as syntax and what you can go with the language goes. However, I think it is a little bit easier for beginners to learn. If Java is still to much for you at first, then try either some form of basic or a language like what warbird suggested. They should be even easier to learn. I like Java (and C++) because of all the power and freedom you have. If you have enough expierence you can do pretty much anything you want. Unfortunately, all that power and freedom does make them kind of hard to learn.My best piece of advice for you is to not give up. Regardless of what language you chose it is going to take some time before you become good with it. And it will even longer before you master the art of making games. But if stick with it and don't give up then I think you will eventually succeed regardless of what language you chose. -
I'm wondering if anyone can help me with my problem. I have Suse 9.2 on my computer and I recently added a second monitor. For some reason I can't get it set up correctly. My video card is a Radeon 9800. I think that might be some of my problem since Ati doesn't seem to have good driver support for linux.Anyway, I have Ati's drivers installed, not the ones that came with Suse. I ran the configuration utility that came with the drivers, and as far as I can tell I entered all the right settings. However, the X server refuses to start when I have both monitors configured. When I have just one monitor configured the desktop will come up, but for some reason it won't all fit on the screen. You have to scroll up and down to see all of the desktop. I'm not sure what I need to do to fix this problem.If I need to clarify more, let me know. I hope someone can help.
-
I use Gaim for my instant messaging. It supports alot of different clients (MSN and Yahoo are what I use it for). It works well for what I need. It doesn't support voice or video, but I don't use either of those so it doesn't bother me. I like Gaim because it is open source, so it is completely free, and it works under Linux. I don't know much about Trillian, but it is Windows-only isn't it? I've never used Trillian so I can't say which is better but Gaim is another one to try if you are tired of using multiple clients.
-
Right now the only computer I have is a desktop. There are several reasons why I decided on a desktop. First, it was cheaper than a laptop with the same specs. Since I like playing games, I needed a pretty fast computer, which put laptops out of my budget. Also, I wanted to be able to build my computer, which is kindof hard to do with a laptop. I may someday buy a laptop in addition to my desktop, but I don't know if I'll ever abandon desktops.However, I do believe there are situations where laptops are better suited than desktops. If you have to travel and need access to your computer, then definitely go with a laptop. Also, if you only have limited space then a laptop might be the better choice. But if you are just going to sit the laptop on a computer desk most of the time then I think a desktop would be better.
-
Cool. Thanks alot for your replies. It sounds like I shouldn't have much trouble with setting my wireless network up. I've never done it before so I want to be sure not to miss anything important. From you replies it sounds like I'll have everything I need, I just need to enable the security and set it up right.BigmanB, I had planned on getting a Linksys router because they seem to have a good reputation. I'm looking at getting the Wireless-G Broadband Router. I assume that it will have the security features that you were talking about. If you have a different suggestion for which one I should buy let me know.
-
Right now my home network is really simple, mainly because I have just one computer. I have it plugged into the cable modem with ethernet, and that's it. However, in a month I'm getting married. My fiancee has her own computer so I'm going to have to upgrade my network to get her on. I thought about just getting a traditional router, but since a wireless one is only $10 more I'll probably get a wireless one. I'll still connect our two desktops with cable since they will be in the same room. I have a PDA I'll use wirelessly and I may be getting a laptop at some point.My question is what do I need to do to make my wireless network as secure as possible? I've heard that wireless networks are really easy to get on and that the built-in security isn't very good. I want to set it up so that only the two of us can get onto the network. What are the different options for wireless security and what is the best solution? Also, is there anything I need to do to protect our desktops just incase someone can get in? I'm not too worried about that, I don't have anything on my computer worth the time it would take to break in. My main concern is I don't want someone using my internet connection for free.
-
I use Thunderbird and it does take it a second to get started. What I do is have it load when Windows boots up and then automatically minimize to the tray. Then it is both out of the way and it only takes a single click to get it back. I like Thunderbird becuae I can get both my Hotmail and Yahoo mail with it.
-
Languages Worth Learning What languages should I learn?
FearfullyMade replied to FearfullyMade's topic in Programming
Thanks everyone for your input. I'll keep your suggestions in mind when I'm bored and need something to do.signatureimage,Thanks for suggesting SQL. That is something I forgot about but I am sure will be important. Database programming doesn't sound very exciting but it is something that I need to learn. Part of my problem with learning something like SQL is I don't know what to make with it. I could just read about it and do some cheesy tutorials, but I like to actually make something while I'm learning. That's how I learned how to program in the first place, by making games. For me it is easier to see the importance of what you are learning when you are making a real program as opposed to some little pointless example.I did know that each processor has its own assembly language, but that is about all I know about assembly. If I do study assembly my goal would be to gain a basic understanding of it. That way if I ever need to actually use it woun't take long to figure out. -
Hello all.Right now I'm a Computer Science major in college. After I graduate (hopefully in three years) I'm wanting to get a job programming. At the moment I'm not too picky about the type of job, as long as it involves programming. So what I'm wanting to know is what languages would be worth learning while I'm in college. There are a lot of languages out there, and I'm not sure which ones are extensively used. I'm wondering what languages any programmers out there use at your job or have heard of people using. I want to be sure to have the knowledge needed to get a good job out of college. I don't want to miss out on an opportunity just because I didn't take the time to learn a language.Right now I feel comfortable using C++, C#, and Java. I've heard that COBOL is still around in many companies so I'm thinking about taking a class in it. I might learn some assembly, but I'm not sure how much and where it is used anymore. Other than those two I have no idea what might useful to learn.So if anyone has any suggestions let me know. Also, if you do suggest a language it would be nice if you could point me in the direction of any good books or online resources you know of for learning that language.
-
I understand what you are saying, but I'm not sure if there is enough activity to warrent splitting the forum up. I think it works fine like it is. I don't feel that it is too hard to sort through the noivce and advanced topics, but that's just me. I see where it could get tiresome if there were a lot of threads to go through.
-
I am currently just using ad-aware. It works for me because I don't seem to get a lot of spyware. If you are getting quite a bit then I would suggest using both ad-aware and spybot. Together they will catch most spyware.I noticed that I haven't been getting much spyware since I switched from ie to firefox. It may just be a coincidence but it appears that firefox does a better job of keeping that junk off your computer. So you might give firefox a try as well if you are having problems with spyware.
-
Like the above posters said, http://nehe.gamedev.net/ has really good opengl tutorials. Also, check out the main site, http://www.gamedev.net/page/index.html. It has more good tutorials. If you can't find many opengl tutorials, Directx tutorials will work as well. Opengl and Directx are close enough that generally a tutorial could work for either one without much modification. With advanced topics I'm not sure if this is the case, but for learning the basics Directx tutorials should do in a pinch. When I first started learning Directx I couldn't find many Directx tutorials, so I had to use opengl ones occansionally. They were a little harder to figure out, but they worked.