Sign in to follow this
Followers
0
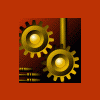
How To Make Sure Your Server Can Generate Images How to use createthumb()
By
veerumits, in Programming
By
veerumits, in Programming
Terms of Use | Privacy Policy | Guidelines | We have placed cookies on your device to help make this website better. You can adjust your cookie settings, otherwise we'll assume you're okay to continue.