Sign in to follow this
Followers
0
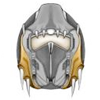
Php Email Validation A PHP data validation class with many functions
By
sonesay, in Programming
By
sonesay, in Programming
Terms of Use | Privacy Policy | Guidelines | We have placed cookies on your device to help make this website better. You can adjust your cookie settings, otherwise we'll assume you're okay to continue.