Sign in to follow this
Followers
0
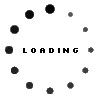
Php: Object Oriented Programming Prerequisites: A good understanding of PHP
By
Rigaudon, in General Discussion
By
Rigaudon, in General Discussion
Terms of Use | Privacy Policy | Guidelines | We have placed cookies on your device to help make this website better. You can adjust your cookie settings, otherwise we'll assume you're okay to continue.