Sign in to follow this
Followers
0
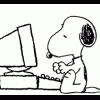
How Video Cards Render What You See
By
rayzoredge, in Hardware Workshop
By
rayzoredge, in Hardware Workshop
Terms of Use | Privacy Policy | Guidelines | We have placed cookies on your device to help make this website better. You can adjust your cookie settings, otherwise we'll assume you're okay to continue.