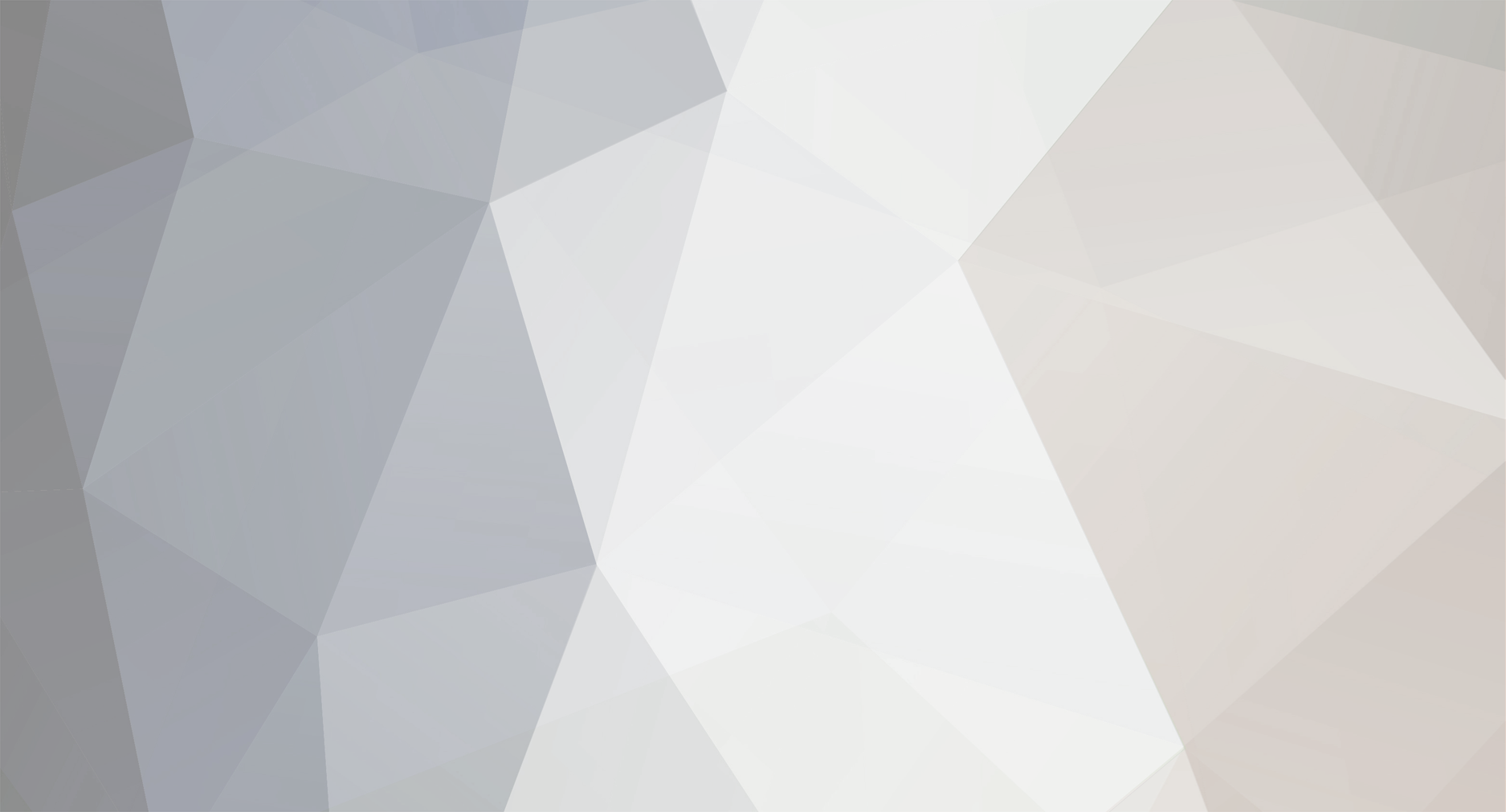
xavier1280
-
Content Count
11 -
Joined
-
Last visited
Posts posted by xavier1280
-
-
Hi All,I am very new to E-Commerce, I've recently acquired a web design project. My client requirements is to Store Customer Details, Product Listing, Manage Categories and products, Manufacturers, Specials and develop reports for best viewed products, products purchased, Customer Orders and they should be able to add it to cart and checkout and accept different methods of payments including credit cards (Visa, Master Card, Direct Debit) and keep track of recurring payments.. Is there a pre configured script for PHP? or does the hosting plan needs to have ecommerce support???Any help would be appreciated. I need to start from scratch and very new to this process.
-
Thanks for the information Mod. I had recently created a topic in a sub forum called webdesign. I cannot seem to trace it or find that topic. Perhaps that topic needs to be accepted by Mods.My Question was simply to ask how to implement web conferencing and video conferencing. I have a client who has requested me to develop a website for a school that teaches Maths, English, Spelling and Reading.Their requirements was that they want to have web conferencing feature to conduct live meetings with students as well as virtual classroom would have all the resources for students to download tutorials, lecture notes, lab assessments and virtual classroom simulation labs from a web based application. Also lecturer needs to interact with students one on one remotely and creation of group discussion forum for students to interact with teachers and post relevant topics. Other simple requirements are students can upload their assignments from digital drop box that will automatically post to lecturer's email. I did some research and came across few packages called Electalive, Dim Dim, Moodle. But Still uncertain where to begin with???Thanks. Any help would be much appreciated.
-
Trap 17 seems like a very interesting place to ask questions. I am just enquring to find out whether the basic hosting plan supports Php My Admin to create database and is it e-commerce integrated? Thanks for all the suggestions and assistance i had with forum members.
-
I am currently working as a freelancer to develop websites for companies. I got a Question to ask, I've asked many forums and they seemed uncertain on answering my query.
I am currently developing a site for a school who requires me to implement web conferencing and video conferencing to conduct live meeting with participants. students in this instance, as well as a virtual classroom with lab simulations from a web based application and interaction with students remotely. They want to be able to view/download Assessment Labs, Lecture Notes, virtual labs.. A little similar to Blackboard.
They also want to have shopping cart with appropriate packages with paypal integrated to purchase products and make payments. They want to use Secure Payment (SSL Certificate) With different methods of online payment. i.e. credit card, direct debit payment. It also needs recurring payment.
I did some research on google. I come across dimdim and electalive. I'm still uncertain on the process of how to go about impementing these features.
Thanks.
-
Thanks for the important Information Kasperooney. I will be active in forums and will contribute to tutorials. I also appreciate that you explained me the costs of basic hosting package. I will spend some time going through the forums. Also If you dont mind me asking just another specific question, if suppose i do earn the amount of credits needed for basic hosting package i.e. $3, does that support php& Mysql and do i need to have sufficent credit on the following month to again top up the hosting package by writing good quality posts..Thanks for your effort in replying to my questions.
-
Thanks for the kind welcome Kasperooney. I have had some read through some pages that explains about MyCent and frequently asked questions. I am just trying very hard to understand with how to earn credits (Mycent) easier and how many posts do i need to make to buy basic hosting package??? From my understanding does this mean 100 posts equivalent to $1. I appreciate Trap 17 is a great place to test or experiment with site design, people who don't want to 'go live' on their REAL domain, or that are just happy to have a site on the WWW for free and able to test it and run scripts.
-
Hi All,
I am an IT Graduate from University of Auckland, I have a great passion for web development, I currently assisted group of developers with small projects to build websites such as http://forums.xisto.com/no_longer_exists/ and have good grasp of PHP & MYSQL. I also enjoy developing CSS websites with Flash.
I find Trap 17 a great tool to communicate with other web developers and stregthen our knowledge and skills and appreciate all the hard work.
Kind regards,
Alkaif -
Hi Admin,Can we possibly have a thread in the main page, so users can request on specific topics for instance. I'm doing web development and there are few areas that i would need assistance with. For example. I am interested to know how to create a contact us page so when the users fill out that form and click submits, provided that it has form validation and submits to particular email. My Question in this case would be can we have the requests thread created and if someone is able to answer any specific query on the following topic and would assist many users in the forum.Thanks.
-
Trap 17 is a great site. I am just enquiring to find out what are the hosting plans that we can purchase provided that we have enough credit (my cents) to choose the appropriate needs of our website. Sorry if its already been posted or any link to guide me through would be much appreciated.
Php/mysql Login/register Tutorial for login with databases.
in General Discussion
Posted · Edited by jlhaslip (see edit history) · Report reply
Quote tags added