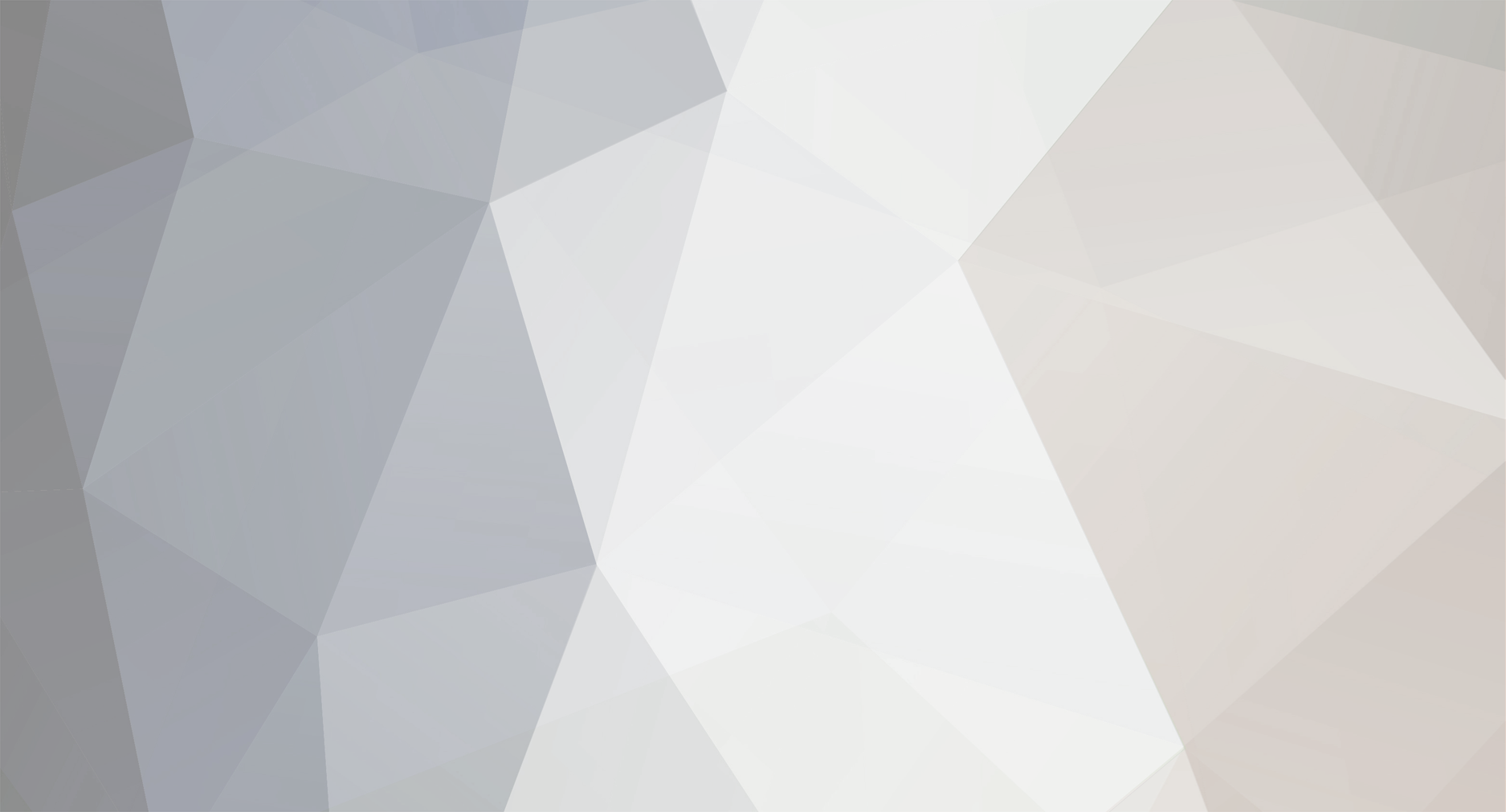
methane
-
Content Count
6 -
Joined
-
Last visited
Posts posted by methane
-
-
G'day D'day, first time posting and even my first time at the site (found after a quick google) so i hope i've followed the correct protocol. I'm stuck using borland C in Windows at home and i've come across a simple problem. My knowledge of C spans from the basics up to Stacks, Queues, Lists, Linked/Double versions, Binary tree and hash tables but its the simple things that always get me stumped.
The issue i'm having is that i use a fgets to recieve and store a string, followed by a strcmp with the saved string and "generic preset command" resulting in failed conditions. Right now the program i'm writing is a bit large so i've just written a small sample one to illustrate the problem:
Code:
PHP Code:
char array[20]; printf("Enter a string\n\n"); fgets(array,1024,stdin); if(strcmp(array,"bob the builder")==0) { printf("%s is a valid command",array); } else { printf("Error: Unknown Command, try again"); }
It will always result in the error part of the loop and i'm fairly certain that the issue arises from fgets recieving one additional character, but i can't for the life of me remember how to go about it. I had a quick look around the FAQ (Which i applaud the posters for as it resolved many issues i've had) but couldn't find anything regarding this. Once again, awesome site full of answers to questions and issues i had a year ago with my comp sci courseWhat you said is correct, there is an additional character receive from fget. That is the newline character. Do you remember what is the last key when you input the command? That is the 'Enter' Key. So you have to include the newline character in your compare string. i.e. the compare string should be 'bob the builder\n' instead of 'bob the builder'
char array[20]; printf("Enter a string\n\n"); fgets(array,1024,stdin); if(strcmp(array,"bob the builder\n")==0) { printf("%s is a valid command",array); } else { printf("Error: Unknown Command, try again"); }
Actually, this problem can be solved if you run it in debug mode. Try to watch the variable and compare the strings manaully, you will found that 2 strings are somehow different.Please also be noted that your array size is only 20 while fgets read in 1024 bytes, it may cause memory error if you input string is longer than 19.
-
For me I have got,1 x 16 MB SD2 x 256 MB SD1 x 512 MB Mini-SD1 x 1 GB SD1 X 128 MB MS1 X 64 MB Flash thumb Drive1 X 256 MP3 Player
-
Besides Google Earth, there is another site Google Moon https://www.google.com/moon/
Let's see when we will have google Solar System -
Okay i got a little further take a llook at this code and tell me what you think do i need a while loop in there?
#include<iostream.h>//------------------------------------------------------------------------------void Reduce(int Num, int Denom)int Div = Num;if (Num % Div == 0 && Div % Div == 0)GFC = int DivelseDiv--;//------------------------------------------------------------------------------int main(){int Num, Denom;cout << "Enter the numerator: ";cin >> Num;cout << "Enter the denominator: ";cin >> Denom;Reduce(Num, Denom);cout << "The reduced fraction is " << Num << "/" << Denom << endl;}return(0);}
To find out the GCD/GCF of two integers, you can make use of euclidean algorithm. That is, GCD(a,= GCD(a%b,
if a>b. You can use a recursive function to find out the answer in fewer steps. The function should look like this
int GCD(int a , int
{
if (a ==
{
return a;
}
if (a * b == 0)
{
return a + b;
}
return ( a%b , b%a);
}
Hope it can help.Okay i got a little further take a llook at this code and tell me what you think do i need a while loop in there?
#include<iostream.h>//------------------------------------------------------------------------------void Reduce(int Num, int Denom)int Div = Num;if (Num % Div == 0 && Div % Div == 0)GFC = int DivelseDiv--;//------------------------------------------------------------------------------int main(){int Num, Denom;cout << "Enter the numerator: ";cin >> Num;cout << "Enter the denominator: ";cin >> Denom;Reduce(Num, Denom);cout << "The reduced fraction is " << Num << "/" << Denom << endl;}return(0);}
To find out the GCD/GCF of two integers, you can make use of euclidean algorithm. That is, GCD(a,b ) = GCD(a%b,b ) if a>b. You can use a recursive function to find out the answer in fewer steps. The function should look like thisint GCD(int a , int b){ if (a == b) { return a; } if (a * b == 0) { return a + b; } return ( a%b , b%a);}
Hope it can help.
Multiple Drop Down Menus W/ Submit Button
in Programming
Posted · Report reply
Actually, javascript can do you need. You should write a function called by on submit form. In the javascript function you can use switch cases to check the inputs and then redirect the page to what you need it to go.